
I'm trying to prevent team creation for any user.
I have a test code that simply connects with a third party service and tries to create a test team.
account.createOAuth2Session("microsoft", url_success, url_failure);
teams.create("test", "test").then((response) => {
console.log(response);
}).catch(
(error) => {
console.log(error);
}
);
And I got the following error:
AppwriteException: User (role: guests) missing scope (teams.write)
at Client.<anonymous> (file:///Users/.../node_modules/appwrite/dist/esm/sdk.js:385:27)
at Generator.next (<anonymous>)
at fulfilled (file:///Users/.../node_modules/appwrite/dist/esm/sdk.js:22:58)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5) {
code: 401,
type: 'general_unauthorized_scope',
response: {
message: 'User (role: guests) missing scope (teams.write)',
code: 401,
type: 'general_unauthorized_scope',
version: '1.2.1'
}
}
But on the console the team is well created
(see picture)
If you have any advice or explanations to give me, I'm interested.
Thanks in advance
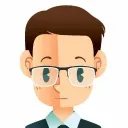
When you're stating and createOAuth2Session
it will go to the provider for authentication.
Which means the line teams.create
is being called with no user, aka guest.
You can try a flow like this (for example)
account.get().then((user)=>{
// Is logged in
addToTeam();
}).catch(()=>{
// not logged in
OAuth2Session();
})
function addToTeam() {
teams.create("test", "test").then((response) => {
console.log(response);
}).catch(
(error) => {
console.log(error);
}
);
}
function OAuth2Session(){
account.createOAuth2Session("microsoft", url_success, url_failure);
}
There is more to it, but this will make sure the team operation happens with a current signed user

the second function is on another page (the success url), it's called only after a successful login
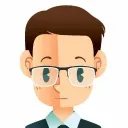
I gather them for convince sorry for the miss confusing
What I'm trying to say is that the team.create()
should run only after the user is logged in

The user is logged in, ´cause the team is well created on appwrite (see picture ) but I still get an error
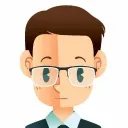
The same error is here? about user being in (role: guests)
?

yes
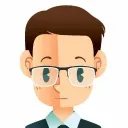
Are you still calling these two functions together?
account.createOAuth2Session("microsoft", url_success, url_failure);
teams.create("test", "test").then((response) => {
I think that the teams.create
should be separate from the createOAuth2Session
one

I have to pages,
one who call
account.createOAuth2Session("microsoft", url_success, url_failure);
and the other one is the url_success
page who call
teams.create("test", "test").then((response) => {
so the second one is called only if the first one is succesful
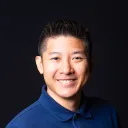
- What's your code around initializing Client, Account, and Teams?
- Do you only have 1 instance of Client in your codebase?
- Can you test calling
account.get()
before callingteams.create()
?

I only have 1 instance on Client in a appwrite.ts then in the same file I create an appwrite variable
const client = ...
const appwrite = {
account: new Account(client);
teams: new Teams(client);
};
export default appwrite;
then I just import appwrite in to different page index.tsx
and success.tsx
and if I call account.get()
I got the same error as mentionned above
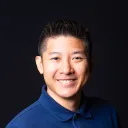
What framework are you using on your front end?
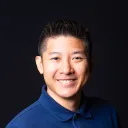
Oooh sorry oauth...you might need to make sure your Appwrite endpoint and your front end share the same front end registerable domain so that the cookie is set properly and are passed properly

I'm just debugging my app so it's localhost basicly
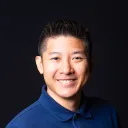
Appwrite and your front end are on localhost?

Yes
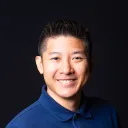
And what are you using for your front end?

Nextjs + react
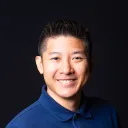
NextJS! Can you make sure that account.get() and teams.create() is being executed client side and not server side?

That's probably the reason why

And is there any way to disable teams creation or collection / document creation for any users ?
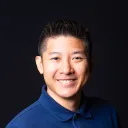
You can disable services. I think it's in the settings for your project. The service will be disabled for clients, but should still work with an API key.
For collections, you would have to limit who has create access

Ok perfect

thanks for all the help
Recommended threads
- Appwrite Cloud Custom Domains Issue
I’m trying to configure my custom domain api.kondri.lt (CNAME pointing to appwrite.network., also tried fra.cloud.appwrite.io with no luck ) but encountering a ...
- Persistent 401 Unauthorized on all authe...
Hello, I'm facing a critical 401 Unauthorized error on my admin panel app and have exhausted all debugging options. The Problem: When my React app on localhos...
- OpenAI Whisper on Appwrite Sites
Hey guys, just wondering if I can serve an OpenAI Whisper AI on appwrite / appwrite sites. tiny model is like ~40-50MB
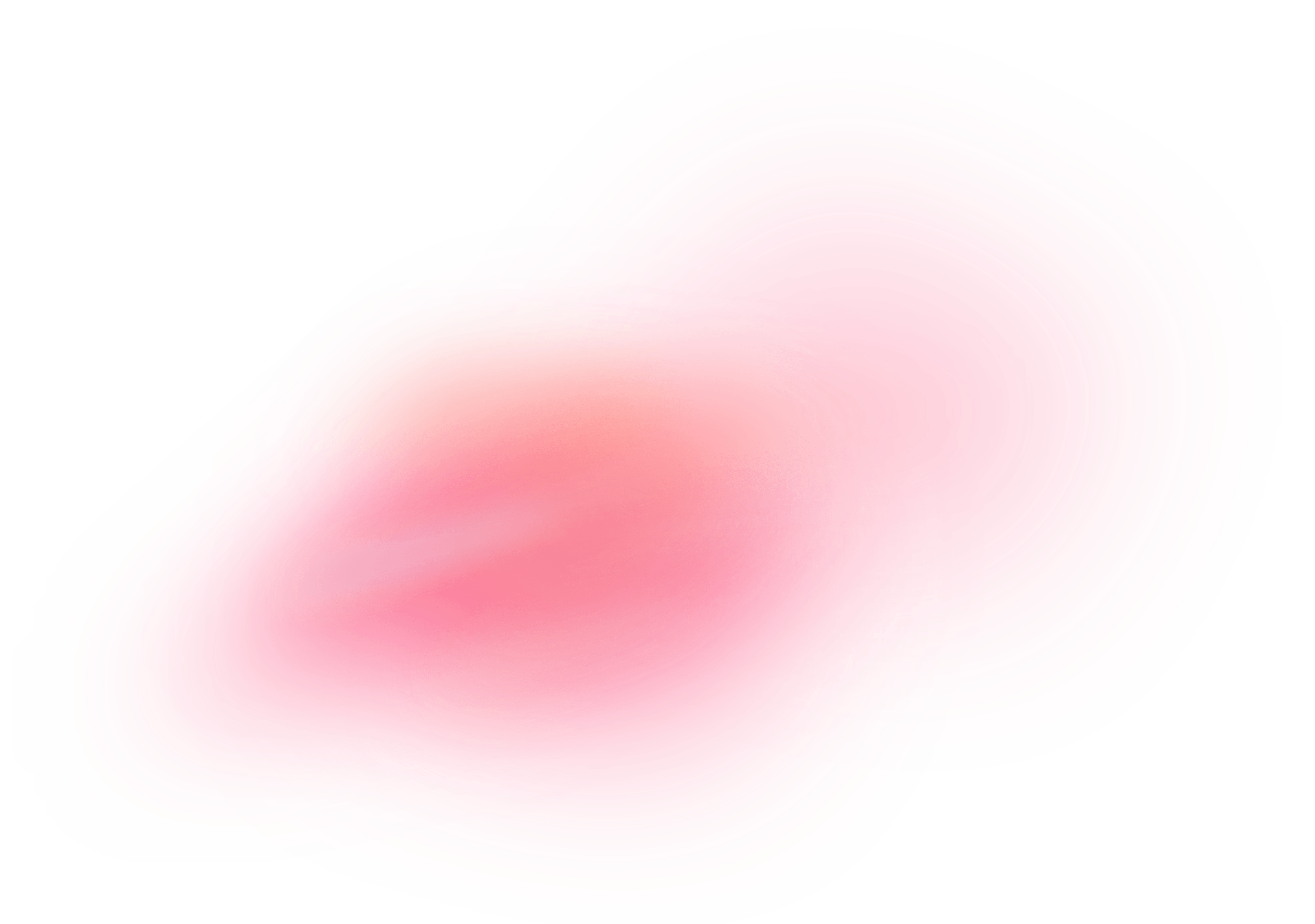