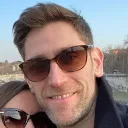
Hello all, I am completely new to Appwrite and have just spun up my first instance via Docker as per the "Getting Started" guide:
docker run -it --rm \
--volume /var/run/docker.sock:/var/run/docker.sock \
--volume "$(pwd)"/appwrite:/usr/src/code/appwrite:rw \
--entrypoint="install" \
appwrite/appwrite:1.2.1
I am on a Mac with M1 chip running macOS Ventura 13.2.1. I accepted all of the default settings using Ports 80 and 443 and am running on localhost.
I have Appwrite running on http://localhost:80 and have signed up and created my first App using the 'Web' preset.
I have made a next.js app where I am attempting to create a User via the Accounts API linked to my AppWrite App.
I have created a client and account instance using my project ID:
// located in libs/appwrite/appwriteConfig.ts file
import { Client, Account } from 'appwrite'
const client = new Client()
.setEndpoint('https://localhost/v1') // Your API Endpoint
.setProject('64188acd7499dca23b77') // Your project ID
export const account = new Account(client);
I import and use this when my signup form is submitted:
import { ID } from 'appwrite'
import { account } from '../../../../libs/appwrite/appwriteConfig'
const signupUser = async (e) => {
e.preventDefault()
const promise = account.create(
ID.unique(),
user.email,
user.password
);
promise.then(
function(response ) {
console.log(response)
},
function(error) {
console.log(error)
}
)
}
I am providing the required ID, email and password parameters in my POST request to https://localhost/v1/account
But I am receiving the following error message.
POST https://localhost/v1/account net::ERR_CERT_AUTHORITY_INVALID
My Next.js app is running on http://localhost:4200. I have clearly missed something but can't find what it is via the Appwrite docs.
Any guidance would be greatly appreciated.
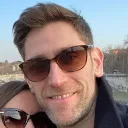
Having wracked my head over this last night, I walked away, got some sleep and then realised the solution to my issue.
In my Appwrite client I had incorrectly referenced https://localhost/v1 rather than http://localhost/v1
Corrected code is now:
import { Client, Account } from 'appwrite'
const client = new Client()
.setEndpoint('http://localhost/v1') // Your API Endpoint
.setProject('64188acd7499dca23b77') // Your project ID
export const account = new Account(client);
My silly mistake.
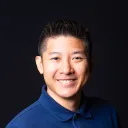
Actually, I highly suggest using https rather than http. The only thing is you have to make sure to accept the self signed warning.
Recommended threads
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
- apple exchange code to token
hello guys, im new here 🙂 I have created a project and enabled apple oauth, filled all data (client id, key id, p8 file itself etc). I generate oauth code form...
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
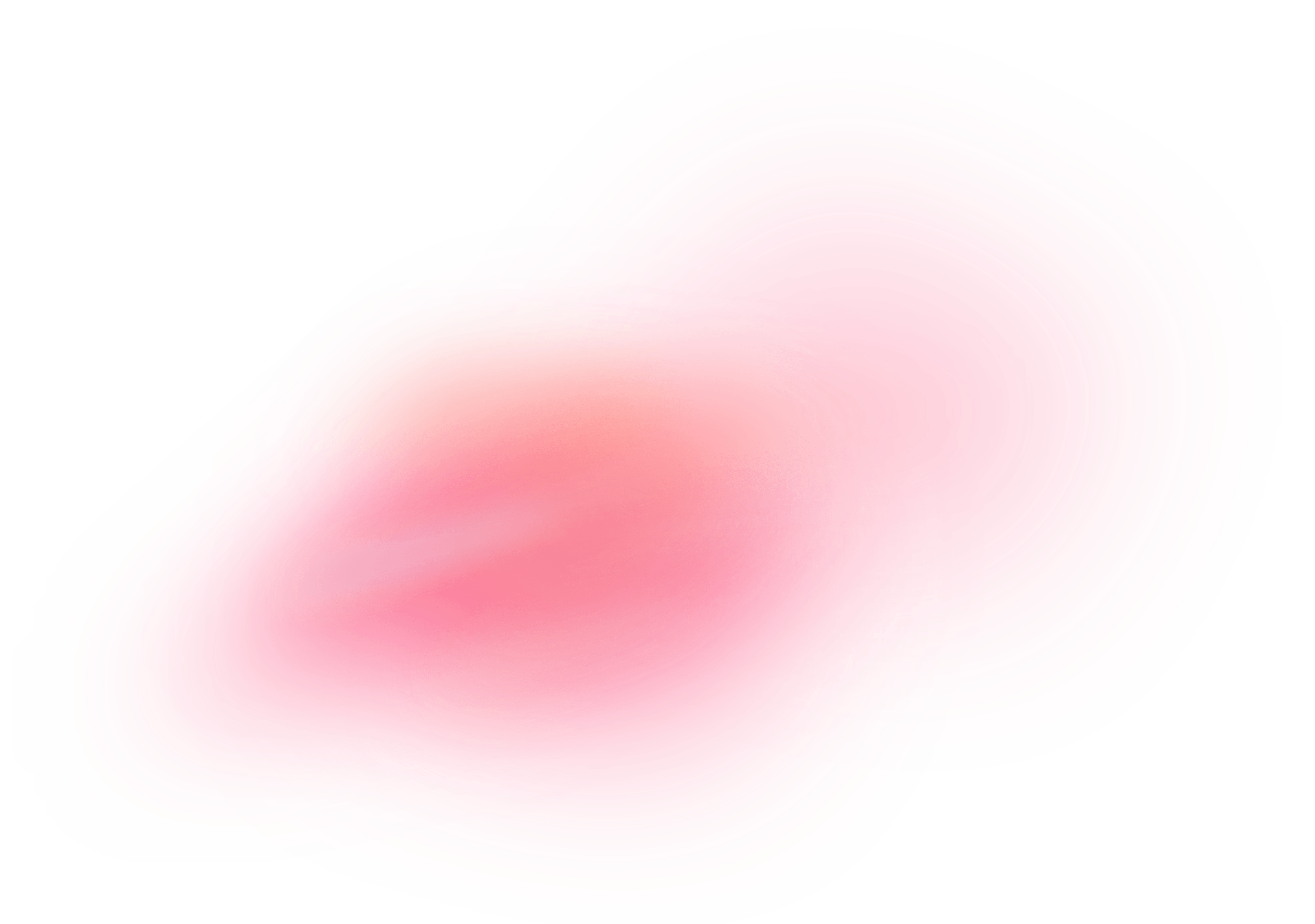