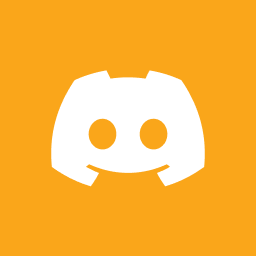
How can I choose node-18 runtime when using appwrite-cli to init function? My appwrite-cli only display this: I'm use latest docker version of appwrite: appwrite/appwrite:1.2.1
Node.js (node-16.0)
PHP (php-8.0)
Ruby (ruby-3.0)
Python (python-3.9)
appwrite -v
1.2.1
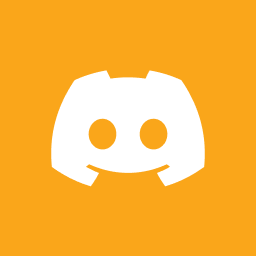
Can not see node-18 runtime when init function using appwrite-cli
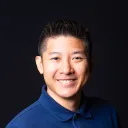
This might help you: https://github.com/appwrite/appwrite/discussions/4826
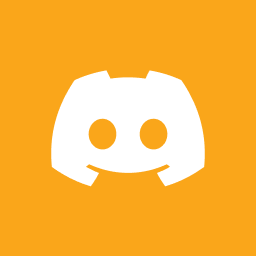
I deploy my first function but get time out. My code is try to verify purchase using googleapi. This code work fine in my local node server. How can I inspect to fix this error
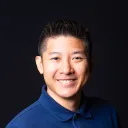
What's your full code?
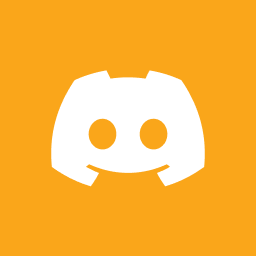
const {GoogleAuth} = require("google-auth-library");
const {androidpublisher_v3} =require("googleapis");
const credentials = require("./assets/service-account.json");
const {ANDROID_PACKAGE_ID, productDataMap} = require("./constants");
const handleSubscription = async(
productData,
token,
) => {
var androidPublisher = new androidpublisher_v3.Androidpublisher({
auth: new GoogleAuth(
{
credentials,
scopes: ["https://www.googleapis.com/auth/androidpublisher"],
}),
});
let response;
try {
response = await androidPublisher.purchases.subscriptions.get(
{
packageName: ANDROID_PACKAGE_ID,
subscriptionId: productData.productId,
token,
},
);
} catch (err){
throw err;
}
// Make sure an order id exists
if (!response.data.orderId) {
return false;
}
// If a subscription suffix is present (..#) extract the orderId.
let orderId = response.data.orderId;
const orderIdMatch = /^(.+)?[.]{2}[0-9]+$/g.exec(orderId);
if (orderIdMatch) {
orderId = orderIdMatch[1];
}
console.log({
rawOrderId: response.data.orderId,
newOrderId: orderId,
});
// Construct purchase data for db updates
const purchaseData = {
type: "SUBSCRIPTION",
iapSource: "google_play",
orderId: orderId,
productId: productData.productId,
purchaseDate: response.data.startTimeMillis ?? 0,
expiryDate: response.data.expiryTimeMillis ?? 0,
status: [
"PENDING", // Payment pending
"ACTIVE", // Payment received
"ACTIVE", // Free trial
"PENDING", // Pending deferred upgrade/downgrade
"EXPIRED", // Expired or cancelled
][response.data.paymentState ?? 4],
};
return purchaseData;
}
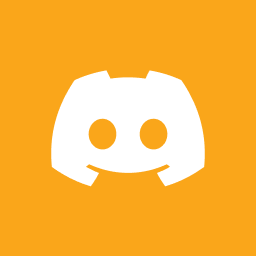
module.exports = async function (req, res) {
// const payload = JSON.parse(req.payload);
// if (!payload.productId || !payload.token){
// console.log("No payload");
// res.json({status: '1'});
// }
const productData = productDataMap["soundwave_pro"];
// if (!productData) {
// console.log("No productData");
// res.json({status: '2'});
// }
const data = await handleSubscription(productData,"thisisfixedtoken");
if (!data){
res.json({status: '3'});
}
};
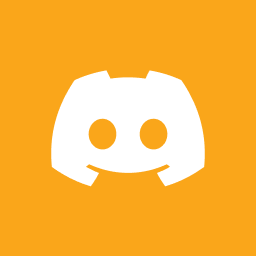
My project structure
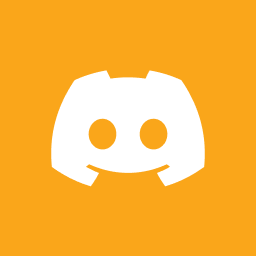
How can I check why this code timeout
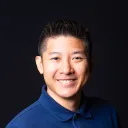
You should make sure you're calling res.json() exactly once. It seems like it's possible for you to not based on this code.
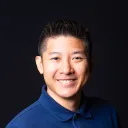
Although connection refused is an even bigger problem ..it might mean the runtime container isn't working at all. Maybe syntax problem or something.
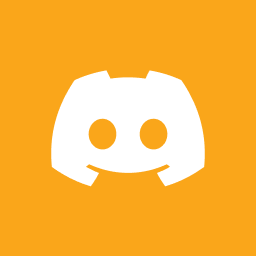
This code from codelabs tutorial and I run successful in local node server
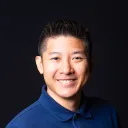
Regardless, something might be wrong in the runtime. I would recommend recreating your function from scratch adding bits slowly, starting with the imports
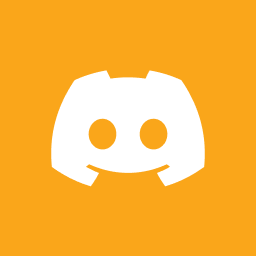
Thank you for help
Recommended threads
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
- Function in Node.JS to monitor events ar...
Hello everyone. I'm creating my first Node.JS function, but I don't have much experience with node and javascript. I'm trying to create a function, that monito...
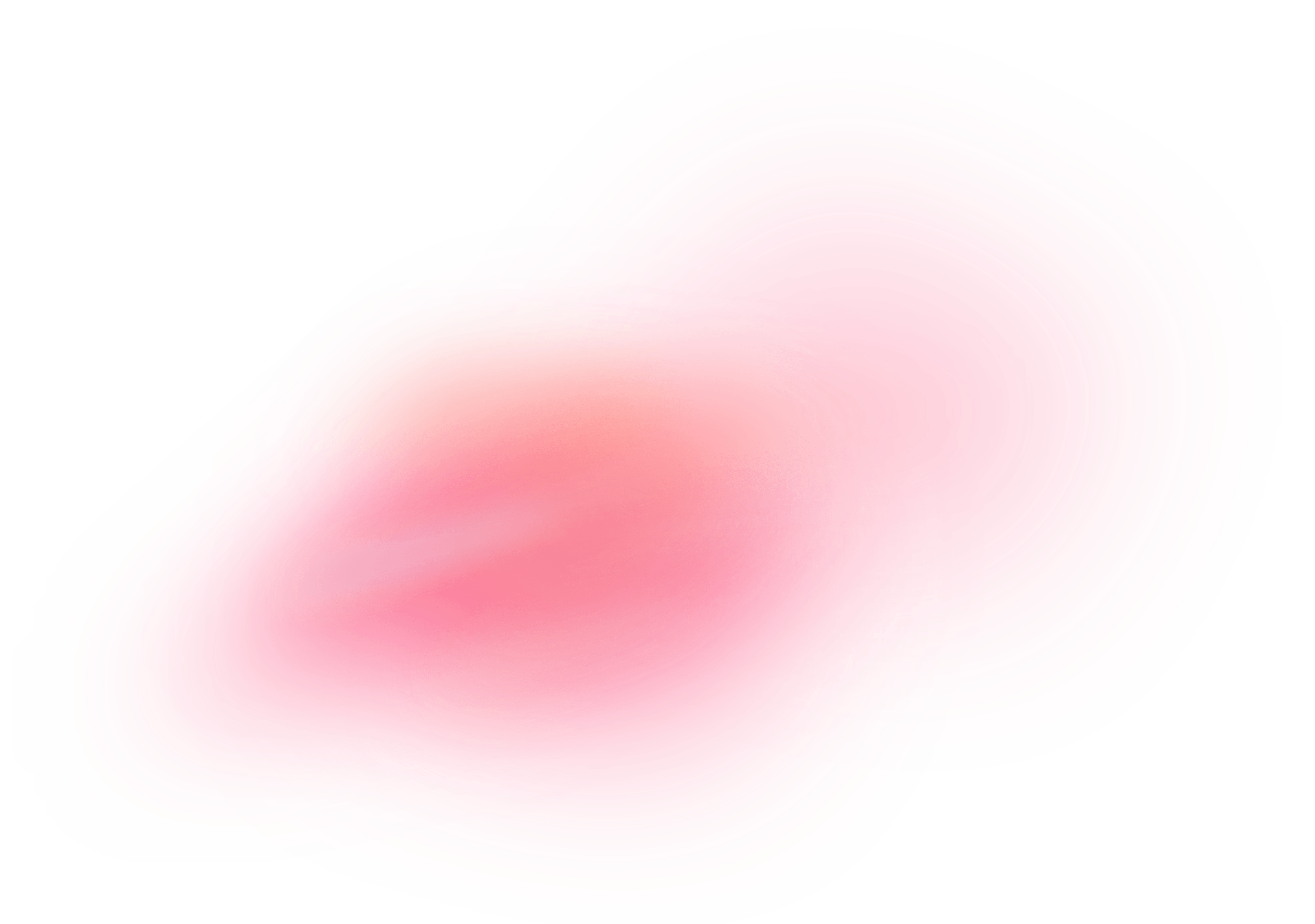