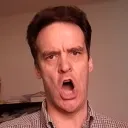
I want to integrate with AppWrite with my NextJS app, but I'm having trouble understanding the flow. What is the best way to keep the session "active", write http-only cookie with the value of the SessionObject returned from AppWrite? Here is a current POC i'm working with:
context/auth.js
const client = new Client();
client.setEndpoint('SOME_SERVE').setProject('SOME_PROJ');
const account = new Account(client);
const AuthContext = createContext();
export function useAuth() {
return useContext(AuthContext);
}
export function AuthProvider(props) {
const [user, setUser] = useState(null);
function signIn(email, password) {
const promise = account.createEmailSession(email, password);
promise.then(
function (response) {
console.log(response);
setUser(response); // Success
},
function (error) {
console.log(error);
setUser(null); // Failure
}
);
}
return (
<AuthContext.Provider value={{ user, signIn, signOut, signUp }}>
{props.children}
</AuthContext.Provider>
);
}
pages/login/index.tsx
export default function Login() {
const { signIn } = useAuth();
const username = useRef();
const password = useRef();
async function handleSubmit(e) {
e.preventDefault();
try {
await signIn(username.current.value, password.current.value);
Router.push("/account")
} catch (err) {
console.log(err);
}
}
return (
<SomeLoginForm username password />
);
}
pages/account/index.tsx this represents a private page, only accessible by someone who has an active session
export default function Account() {
const { user } = useAuth();
useEffect(() => {
if (user === null) {
Router.push("/login");
}
}, [])
return (
<Greetings user />
);
}
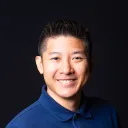
With or without server side rendering?
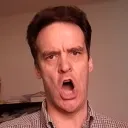
Hi Steven, server side rendering, but I’d like to know from a high level what to look out for for both SSR and CSR!
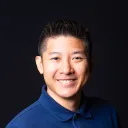
Without server side rendering, refer to https://github.com/appwrite/appwrite/discussions/3938#discussioncomment-3746725.
With server side rendering, you'll need to make sure the cookie that is sent to Appwrite is also sent to you app so you can pull out the cookie and reuse it to make API calls to Appwrite
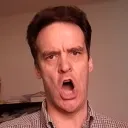
I see, thanks! Is there anything in the docs about saving the response from account.createEmailSession?
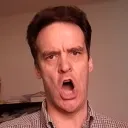
In a cookie I mean
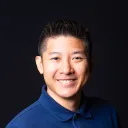
The payload from that won't help you with any sort of authentication
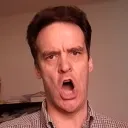
Oh ok.
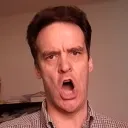
What would I store then after creating a session???
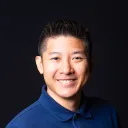
Client side, nothing because cookies are passed automatically
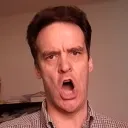
Ohhhhh… ok maybe I’m misunderstanding how to check if a user is logged in. On every page load, I was checking the context, which gets wiped. What should I be check to see if user has an active session if it’s passed automatically
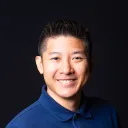
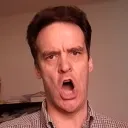
Will give it a read thanks!!
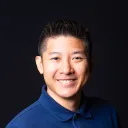
This could also be helpful: https://github.com/Meldiron/appwrite-next13-ssr
Recommended threads
- Need help setting up this error is showi...
You can't sign in to this app because it doesn't comply with Google's OAuth 2.0 policy. If you're the app developer, register the redirect URI in the Google Cl...
- Appwrite stopped working, I can't authen...
I'm having an issue with Appwrite. It was working fine just a while ago, but suddenly it stopped working for me and can't authenticate accounts. I even went bac...
- Fail to receive the verification email a...
I added my email address to prevent it from showing "appwrite," but now I'm not receiving emails for verification or password resets. The function appears to be...
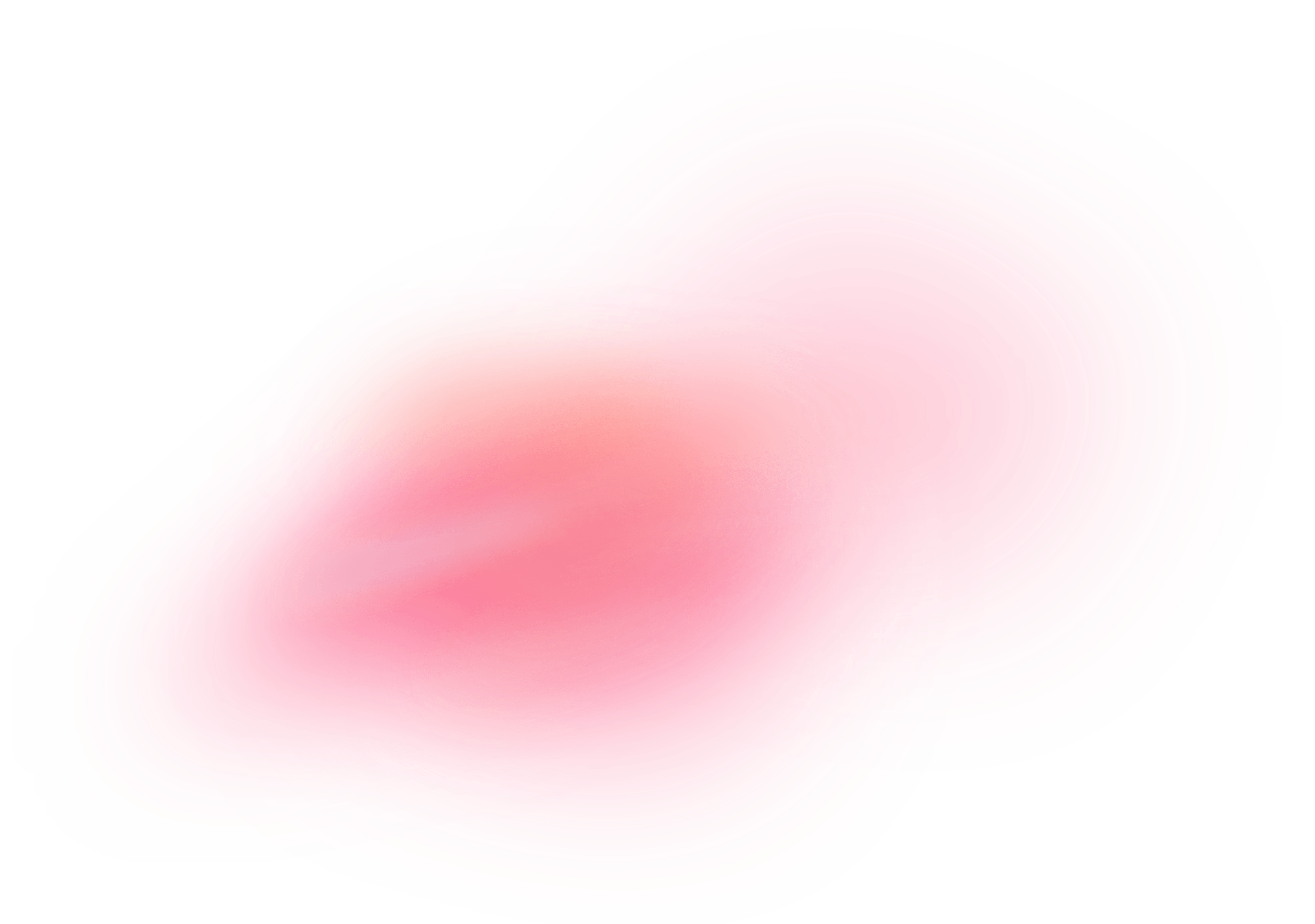