
Here's some server code:
TypeScript
import { Client, Account, Databases, Users, Permission, Role, Query, ID } from 'node-appwrite';
export default async ({ req, res, log, error }) => {
if (req.path === "/")
{
const userId = req.headers['x-appwrite-user-id'];
const client = new Client()
.setEndpoint(process.env.APPWRITE_FUNCTION_API_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const db = new Databases(client);
const event = req.headers['x-appwrite-event'];
if(event === "users." + userId + ".create")
{
let createUserDoc = await db.createDocument('db', 'users', userId, { name: "null", picture: "null"}, [ Permission.delete(Role.user(userId)) ]);
}
}
return res.json({ status: "complete" });
};```
Now, a few months ago, I was able to set the "delete" permission for that document so that only that specific user could delete it. But now I get this error on the server when I create a user:
Error: Permissions must be one of: (any, guests)
If I change it to Role.any(), there are no errors, but that's not the functionality I want. Am I missing something? I have enabled all scopes for my API key to make sure that's not an issue. How can I make it so that only the user who created that document is the only one who can delete it?
TL;DR
Developers are encountering an error when trying to set specific document permissions using an API key. The error states that permissions must be either "any" or "guests," leading to a limitation in functionality. To resolve this, developers can try manually adding the desired permissions in the Appwrite console instead of relying solely on the code.Recommended threads
- Webhooks not working?
I have this webhook in appwrite but unfortunately this webhook isn't being triggered whenever I change something in the database. I've put the right databaseID ...
- Request: When Will Appwrite Sites Waitli...
Hey team! π I recently joined the waitlist for Appwrite Sites on Cloud and got the confirmation email that says: "You've successfully joined the Sites waitli...
- Logs do not work when debugging openrunt...
Hey there. Trying to debug some openruntimes functions locally. I manage to run the server and make requests to it, but for some reason the logger is not printi...
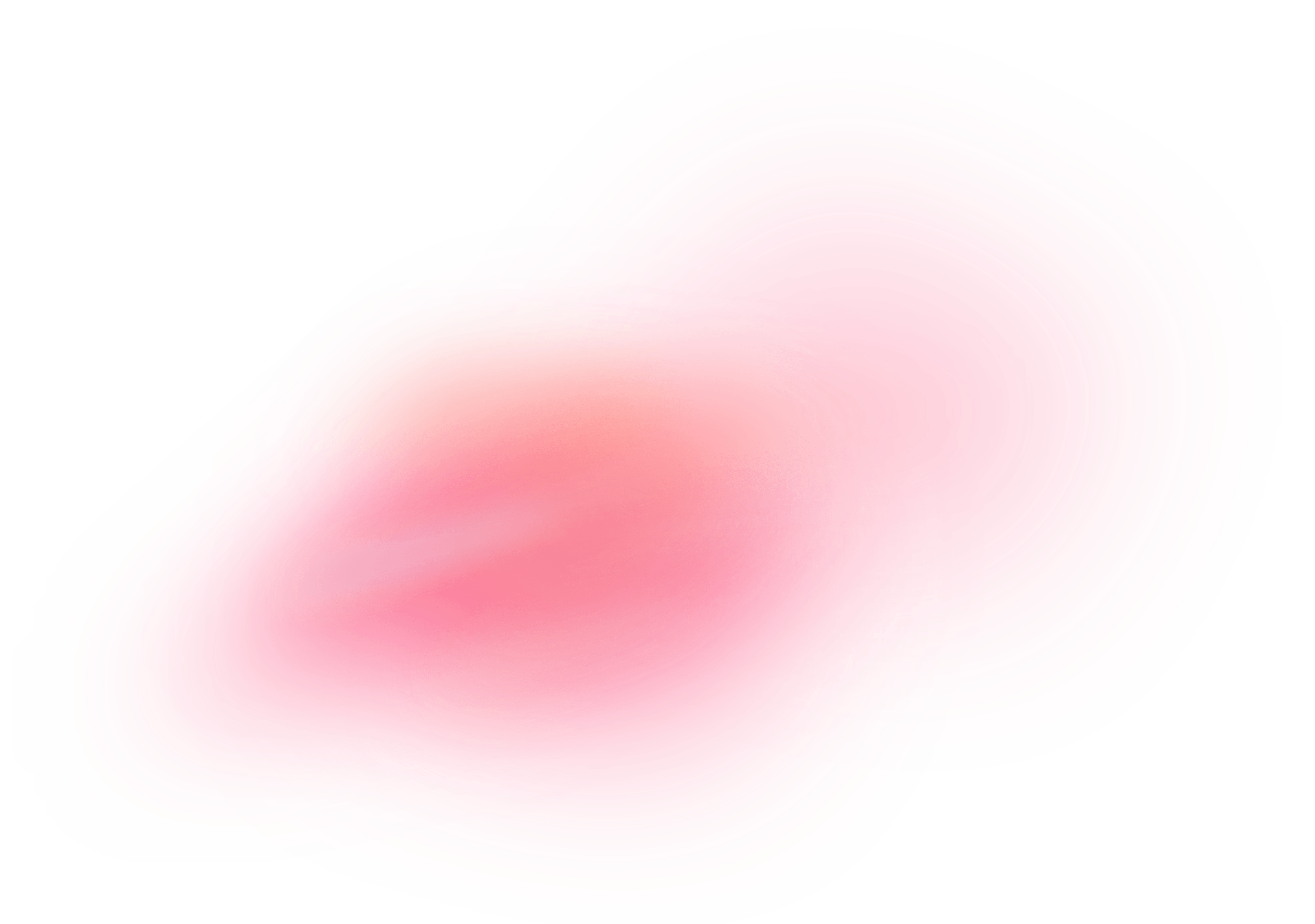