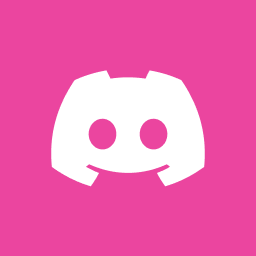
code :
`import { Account, Avatars, Client, Databases, ID, OAuthProvider, Query } from "react-native-appwrite"; import * as Linking from "expo-linking"; import { openAuthSessionAsync } from "expo-web-browser";
const DATABASE_ID = process.env.EXPO_PUBLIC_APPWRITE_DATABASE_ID!; const COLLECTION_ID = process.env.EXPO_PUBLIC_APPWRITE_COLLECTION_ID!; const SAVED_MOVIES_COLLECTION_ID = process.env.EXPO_PUBLIC_APPWRITE_SAVED_MOVIES_COLLECTION_ID!;
const client = new Client() .setEndpoint(process.env.EXPO_PUBLIC_APPWRITE_ENDPOINT!) .setProject(process.env.EXPO_PUBLIC_APPWRITE_PROJECT_ID!)
const avatar = new Avatars(client); const account = new Account(client);
const database = new Databases(client);
export async function login(urlParam:string) { try { const redirectUri = Linking.createURL(urlParam);
const response = await account.createOAuth2Token(
OAuthProvider.Google,
redirectUri
);
if (!response) throw new Error("Create OAuth2 token failed");
console.log("response", response)
const browserResult = await openAuthSessionAsync(
response.toString(),
redirectUri
);
if (browserResult.type !== "success")
throw new Error("Create OAuth2 token failed");
const url = new URL(browserResult.url);
const secret = url.searchParams.get("secret")?.toString();
const userId = url.searchParams.get("userId")?.toString();
if (!secret || !userId) throw new Error("Create OAuth2 token failed");
const session = await account.createSession(userId, secret);
if (!session) throw new Error("Failed to create session");
return true;
} catch (error) { console.log(error); return false; } } `
During Developer It's working Properly Once Create Build And Release Preview APK it's giving below error when we click continue to login button
Error 400
Invalid
success param: URL host must be one of: localhost, cloud.appwrite.io, appwrite.io, .appwrite.io,
Type : general_argument_invalid
Recommended threads
- Flutter native Google Sign Up with googl...
Hey I want to use the native login instead of the WebView. Do you have any experience on that and has Appwrite to plan this support?
- How to upload a profile picture from rea...
I need to upload profile picture from a app i am building for my project... How do i do it ? Till now i have done this much but it shows an error
- Is p-limit compatible with Appwrite?
I have a function that makes asynchronous calls to different Appwrite collections. This is how I make the call: ```js await Promise.allSettled([ ...
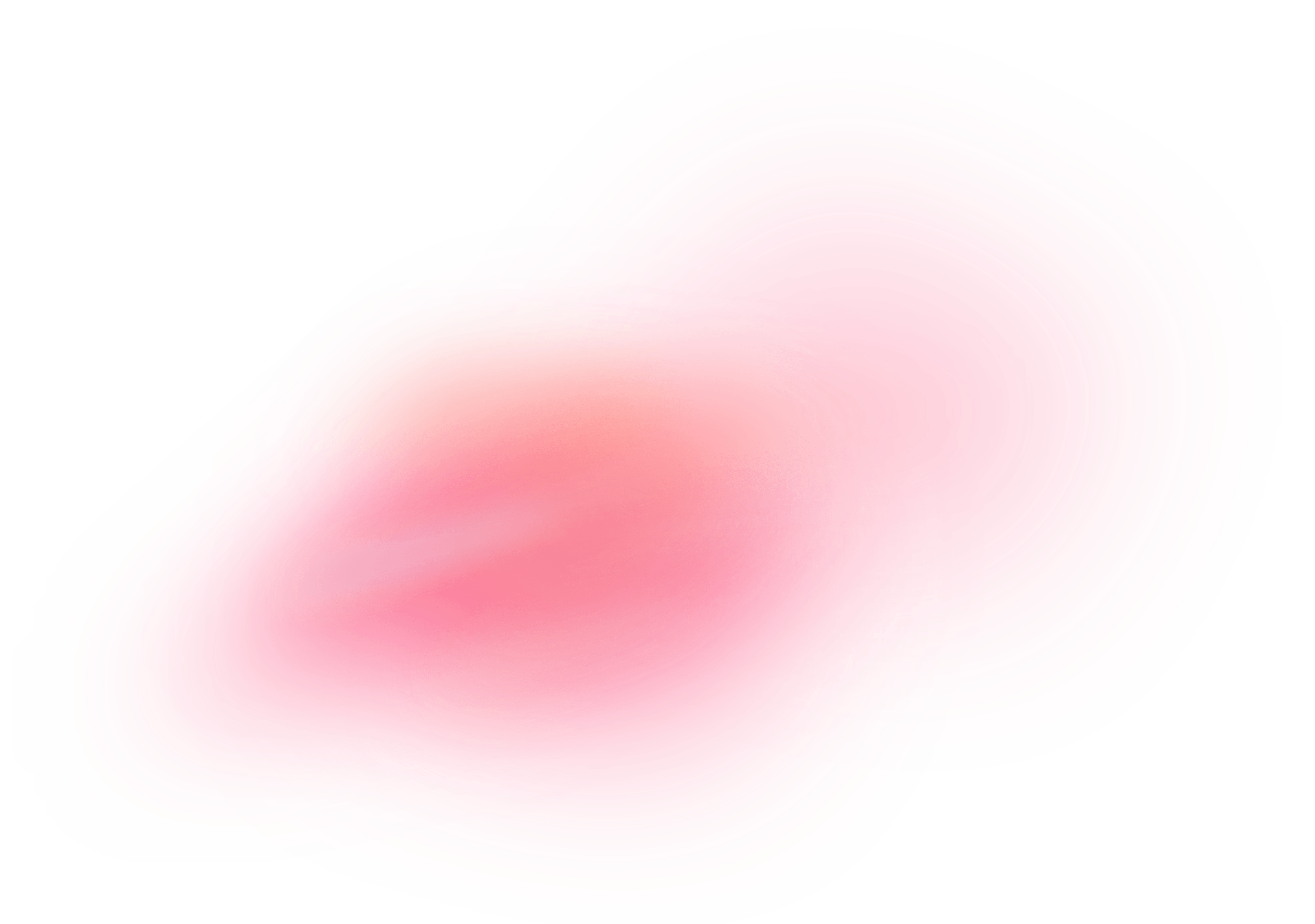