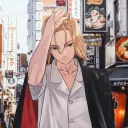
When i get the loggedInUser it returns user but when try to get a session using this ``` "use server"; import { Client, Account } from "node-appwrite"; import { cookies } from "next/headers";
export async function createSessionClient() { const client = new Client() .setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT!) .setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT!);
const session = cookies().get("my-custom-session");
if (!session || !session.value) {
throw new Error("No session");
}
client.setSession(session.value);
return {
get account() {
return new Account(client);
},
};
}
export async function createAdminClient() { const client = new Client() .setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT!) .setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT!) .setKey(process.env.NEXT_APPWRITE_KEY!);
return {
get account() {
return new Account(client);
},
};
}
export async function getLoggedInUser() { try { const { account } = await createSessionClient(); return await account.get(); } catch (error) { return null; } }
export async function getLoggedInSession() { try { const { account } = await createSessionClient(); return await account.getSession('current'); } catch (error) { return null; } }```
the session which is return has null provider access token
Session {
'$id': '66ac26d5de54d1',
'$createdAt': '2024-08-02T00:22:45.924+00:00',
'$updatedAt': '2024-08-02T00:22:45.924+00:00',
userId: '66aaa7d01f8d4af',
expire: '2025-08-02T00:22:45.910+00:00',
provider: 'oauth2',
providerUid: '',
providerAccessToken: '',
providerAccessTokenExpiry: '',
providerRefreshToken: '', } ```
Followed this guide carefully
https://appwrite.io/docs/tutorials/nextjs-ssr-auth/step-1
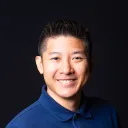
known issue: https://github.com/appwrite/appwrite/issues/8206
Recommended threads
- Unable to read session cookie
Hi, when I am hitting Appwrite **/account** API. I am getting the user account details as expected in the response. However, with that API, Appwrite also adds a...
- Database error
My code: await databases.createDocument( process.env.APPWRITE_DATABASE, process.env.APPWRITE_COLLECTION_USER, data.userId, ...
- No Headers
Hi I have 2 appwrite functions, one is working fine on localhost, second is not even working on prod with https, i inspected the api call, no headers are being ...
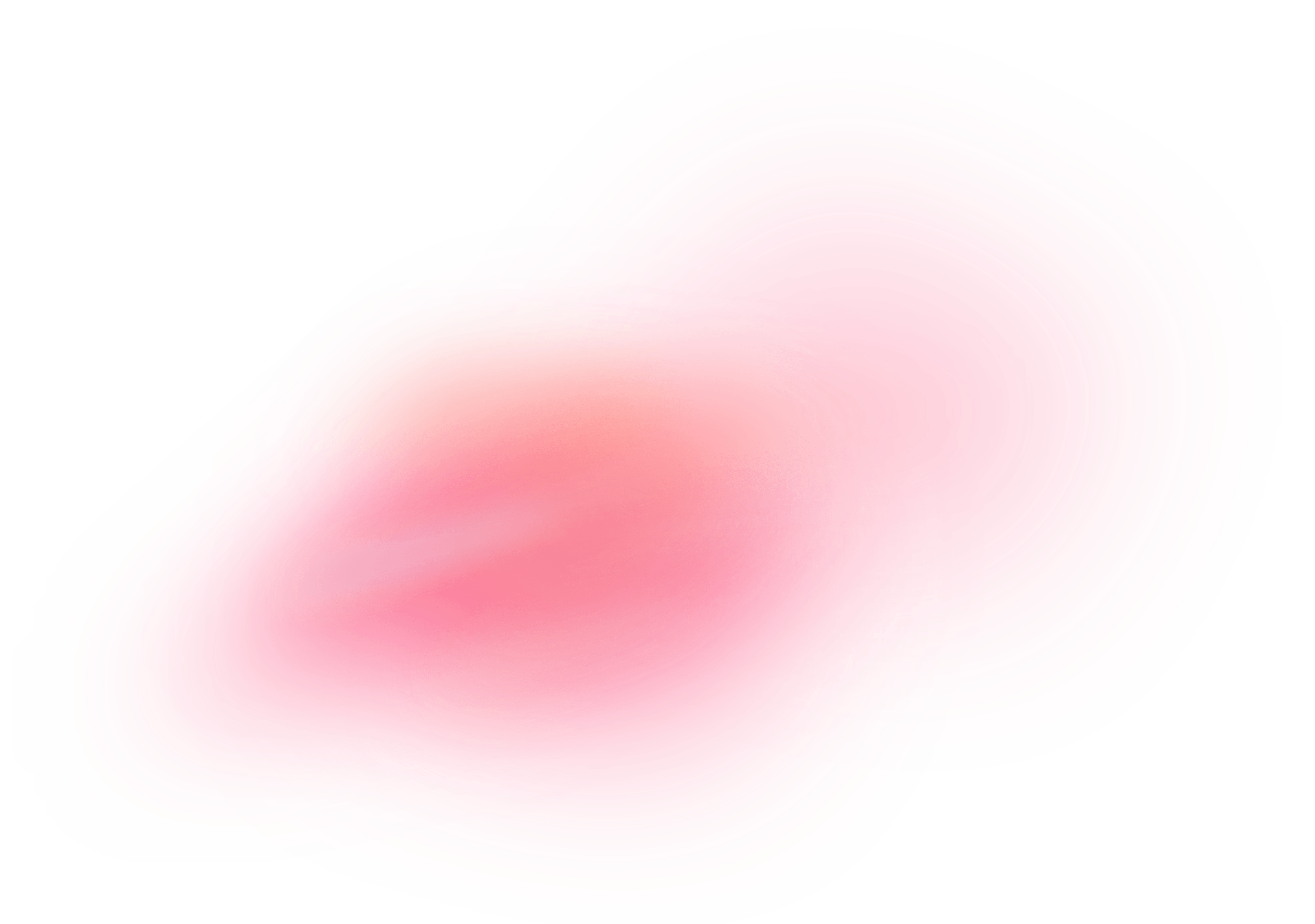