
We are playing around with REST API Authentication. I can successfully register a user and login a user. However when it comes time to get user account details (using REST) it asks for a JWT.
I tried the create JWT REST path and that gives me errors.
Not sure the correct flow for authenticating a user and storing session in browser.
Any help appreciated!

export const getJWT = async () => {
const jwt = await fetch(`https://cloud.appwrite.io/v1/account/jwt`, {
method: 'POST',
headers: {
Host: 'cloud.appwrite.io',
'Content-Type': 'application/json',
'X-Appwrite-Response-Format': '1.5.0',
'X-Appwrite-Project': projectId,
'X-Appwrite-Key': apiKey,
},
})
.then((response) => {
console.log(response)
return response.json();
})
.then((data) => {
console.log('jwt: ', data.jwt);
return data.jwt;
})
.catch((error) => {
console.error('Error:', error);
});
return jwt;
};
export const getAccount = async () => {
const jwt = await getJWT();
const response = await fetch(`${url}/account`, {
method: 'GET',
headers: {
Host: host,
'Content-Type': 'application/json',
'X-Appwrite-Response-Format': '1.5.0',
'X-Appwrite-Project': projectId,
'X-Appwrite-JWT': jwt,
},
})
.then((response) => {
return response.json();
})
.catch((error) => {
console.error('Error:', error);
});
return response;
};
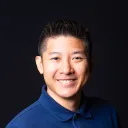
Have you read through this? https://appwrite.io/docs/apis/rest#authentication

@Steven ah, thanks! okay ... this is a really stupid question. How do I get the set-cookie from the header?

this is all that is returned in the response:
{
'$id': '66635473eaa5e1f66046',
'$createdAt': '2024-06-07T18:41:55.971+00:00',
'$updatedAt': '2024-06-07T18:41:55.971+00:00',
userId: '66634059b7c39f742502',
expire: '2024-06-07T22:41:55.961+00:00',
provider: 'email',
providerUid: 'test@me.com',
providerAccessToken: '',
providerAccessTokenExpiry: '',
providerRefreshToken: '',
ip: '108.70.33.101',
osCode: '',
osName: '',
osVersion: '',
clientType: '',
clientCode: '',
clientName: '',
clientVersion: '',
clientEngine: '',
clientEngineVersion: '',
deviceName: '',
deviceBrand: '',
deviceModel: '',
countryCode: 'us',
countryName: 'United States',
current: true,
factors: [ 'password' ],
secret: '',
mfaUpdatedAt: ''
}

nm

found it
Recommended threads
- apple exchange code to token
hello guys, im new here π I have created a project and enabled apple oauth, filled all data (client id, key id, p8 file itself etc). I generate oauth code form...
- What Query's are valid for GetDocument?
Documentation shows that Queries are valid here, but doesn't explain which queries are valid. At first I presumed this to be a bug, but before creating a githu...
- Database not found when attempting to Li...
So, I am doing this in postman to eliminate any possible weirdness from my SDK, and still unable to hit any document level endpoint for my database. I am tryin...
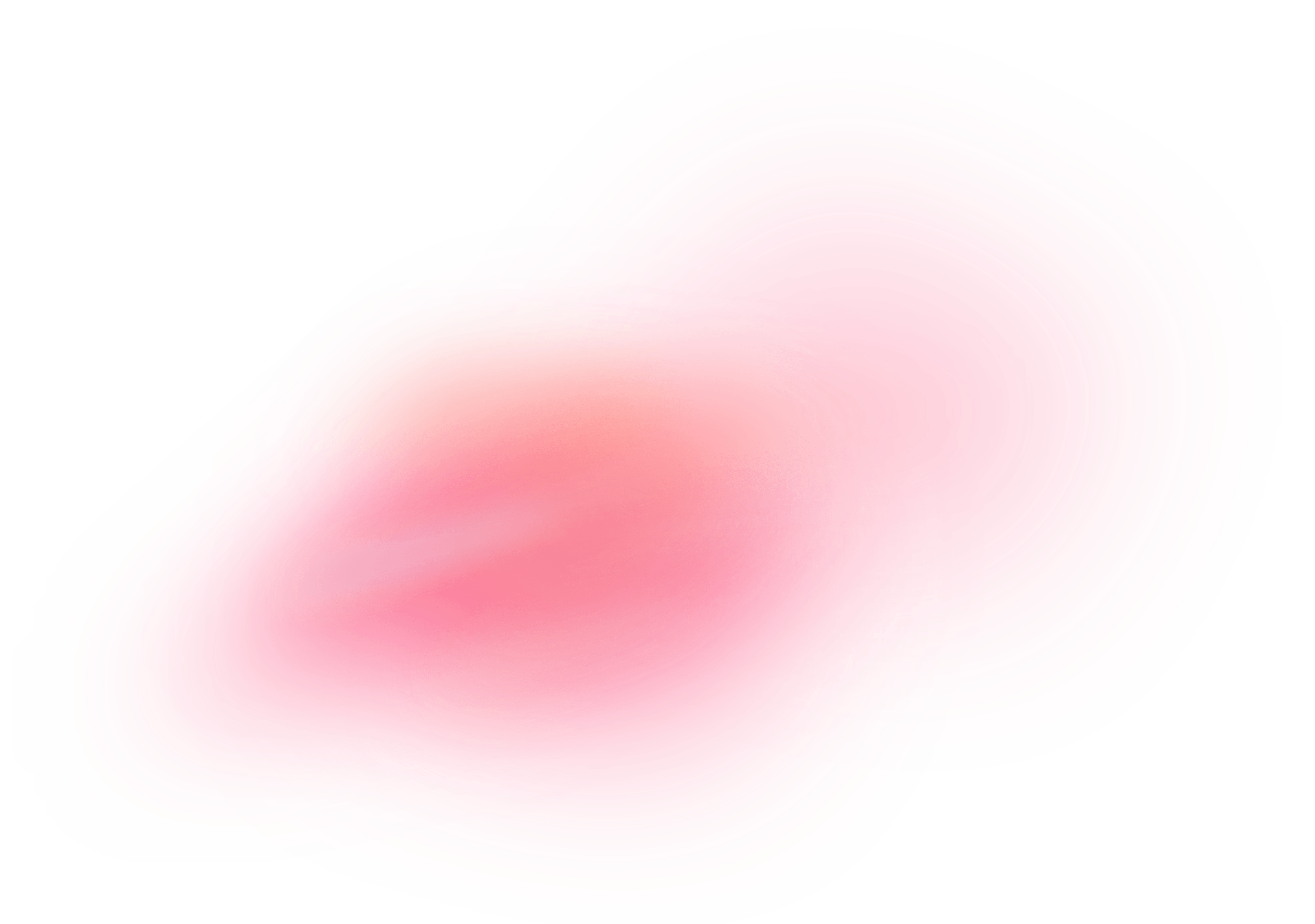