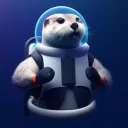
Hi,
I started playing with appwrite today and wanted to build myself a starter kit with stripe and auth via magic link, google and github.
I successfully managed to implement auth via magic links and initiated shadcn after. After that I wanted to restructure my project a little and moved the methods (e.g. createMagicLink) in the actions.ts file but now I get the error: AppwriteException [Error]: User (role: guests) missing scope (account)
.
I'm calling my action from a clientside form. This is my actions.ts:
"use server";
import { createAdminClient } from "@/lib/server/appwrite";
import { ID } from "node-appwrite";
import { cookies } from "next/headers";
export async function createMagicLink(formData: { get: (arg: string) => any }) {
const email = formData.get("email");
const { account } = await createAdminClient();
try {
console.log("createMagicURLToken", email); //todo: remove debug
const session = await account.createMagicURLToken(
ID.unique(),
email,
`${process.env.NEXT_PUBLIC_URL}/auth`,
);
cookies().set("session-secret", session.secret, {
path: "/",
httpOnly: true,
sameSite: "strict",
secure: true,
});
} catch (e) {
console.error(e); //todo: remove debug
return false;
}
console.log("200"); //todo: remove debug
return true;
}
and this is my appwrite.ts:
"use server";
import { Client, Account } from "node-appwrite";
import { cookies } from "next/headers";
const endpoint = process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT;
const projectId = process.env.NEXT_PUBLIC_APPWRITE_PROJECT;
const apiKey = process.env.NEXT_APPWRITE_KEY;
export async function createSessionClient() {
if (!endpoint || !projectId) {
throw new Error("Appwrite environment variables are missing.");
}
const client = new Client().setEndpoint(endpoint).setProject(projectId);
const session = cookies().get("session-secret");
if (!session || !session.value) {
throw new Error("No session");
}
client.setSession(session.value);
return {
get account() {
return new Account(client);
},
};
}
export async function createAdminClient() {
if (!endpoint || !projectId || !apiKey) {
console.log(endpoint, projectId, apiKey); //todo: remove debug
throw new Error("Appwrite environment variables are missing.");
}
const client = new Client()
.setEndpoint(endpoint)
.setProject(projectId)
.setKey(apiKey);
return {
get account() {
return new Account(client);
},
};
}
export async function getLoggedInUser() {
try {
const { account } = await createSessionClient();
const acc = await account.get();
console.log("acc", acc); //todo: remove debug
return await account.get();
} catch (error) {
console.log("null", error); //todo: remove debug
return null;
}
}
Somebody knows what might be wrong here? Thanks in advance!
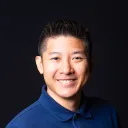
you should not be using the admin client client-side because it uses an API key
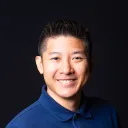
Also, createMagicURLToken()
sends an email to the user with the secret. They're supposed to click on that link and then you call https://appwrite.io/docs/references/cloud/client-web/account#createSession. The secret in there is not supposed to be used for the session secret
See https://appwrite.io/docs/references/cloud/client-web/account#createMagicURLToken
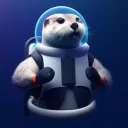
Thanks for your fast response! How to use the client api in that case properly? Like this? https://appwrite.io/docs/quick-starts/nextjs#step-4
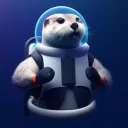
Hmm I don't get it for now. Is there maybe an example for this? Maybe I have to go to sleep 😄
Recommended threads
- Adjusting Node Heap limit on Appwrite Si...
I am running into a build issue with Appwrite Sites. I'm trying to build and deploy one of my Nuxt applications, and I am getting a failed deploy every time. Th...
- Issue: Incorrect input type for cron sch...
Description: The input field for setting the function schedule is of type email, which prevents entering a valid cron expression. Error Message: Please include...
- self host cant create relationship attri...
there is problem to self host i have upgraded to 1.6.2 to 1.7.2 but thin document i cant Create relationship attribute, and i dont get the collection name.
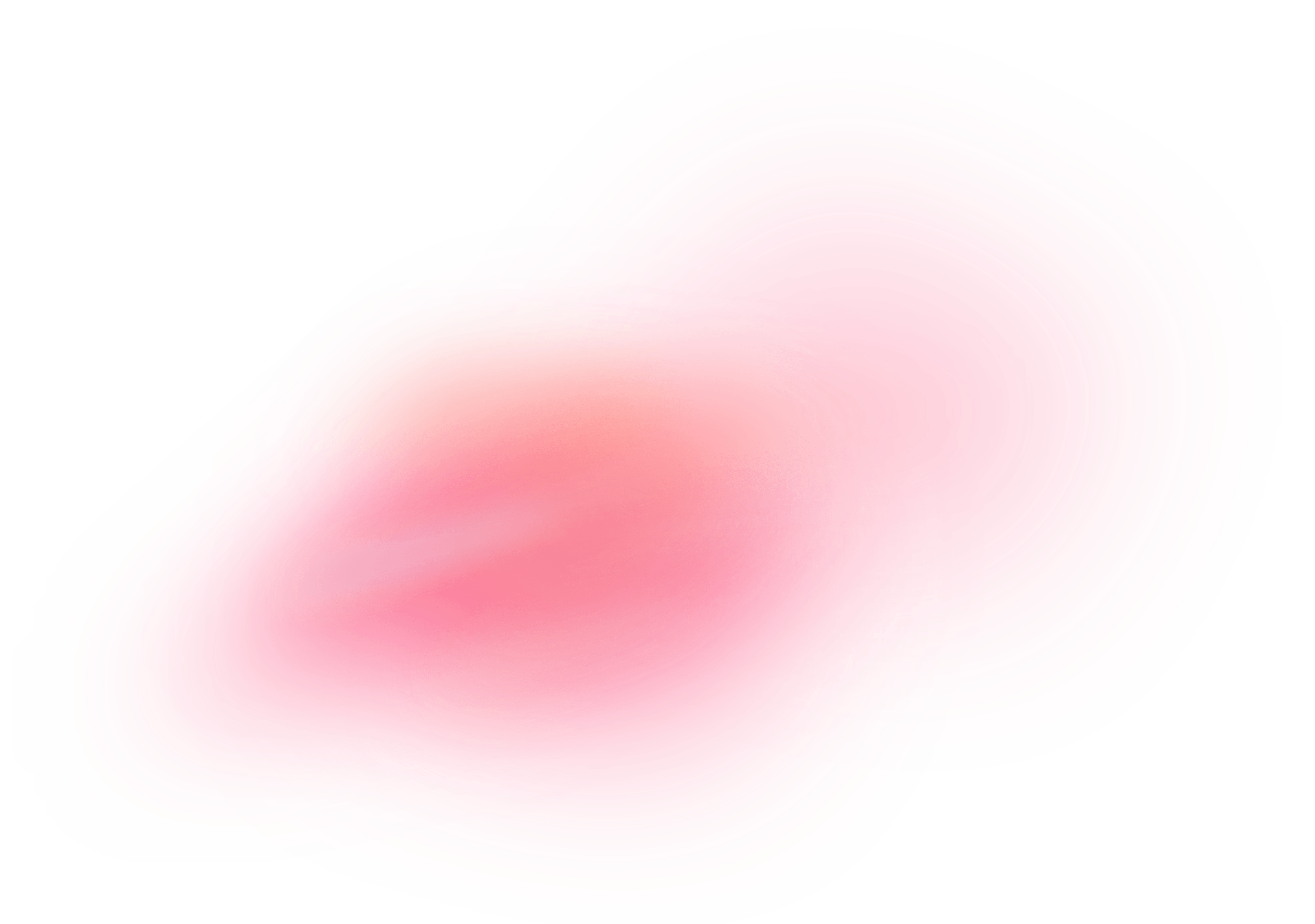