
TypeScript
import { ID, Query } from "appwrite";
import { databases } from "$lib/appwrite";
import type { FormState } from "$lib/configs/CompanyForm";
const DATABASES_ID = import.meta.env.VITE_APPWRITE_DATABASES_ID;
const COLLECTION_ID = "steps";
const createFormService = () => {
async function getFormStore(email: string) {
try {
const response = await databases.listDocuments(DATABASES_ID, COLLECTION_ID, [
Query.equal("email", email)
]);
// if fetched formStore exists
if (response.total > 0) {
return response.documents[0];
} else {
return null;
}
} catch (error: any) {
throw new Error(error);
}
}
async function updateFormStore(formStore: FormState) {
const exist = await getFormStore(formStore.user.email);
if (exist) {
await databases.updateDocument(DATABASES_ID, COLLECTION_ID, exist.$id, formStore);
} else {
await databases.createDocument(DATABASES_ID, COLLECTION_ID, ID.unique(), formStore);
}
}
return {
getFormStore,
updateFormStore
};
};
const formService = createFormService();
export default formService;
This time, I got this error when I call updateFormStore
method
Error: AppwriteException: Collection with the requested ID could not be found.
TL;DR
There is an error "AppwriteException: Collection with the requested ID could not be found" when calling the `updateFormStore` method.
To check the permissions on the collection and verify its existence, ensure that the `COLLECTION_ID` variable is correctly set to "steps" and that the database ID is being fetched correctly. Also, ensure the proper authorization and collection settings in the Appwrite console.
**Solution:** Double-check that the `COLLECTION_ID` is correct and verify permissions in the Appwrite console to ensure the collection is accessible.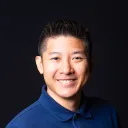
What are the permissions on the collection?

How do I check that?
Recommended threads
- Selfhosting problem
I'm migrating from cloud to self-hosted. I tried using 'Export to self-hosted instance' and use my free Ngrok domain as Endpoint self-hosted instance, but I got...
- Need help setting up this error is showi...
You can't sign in to this app because it doesn't comply with Google's OAuth 2.0 policy. If you're the app developer, register the redirect URI in the Google Cl...
- Direct Upgrade from Appwrite v1.5.11 to ...
I'm on Appwrite v1.5.11. Can I upgrade directly to v1.6.2?
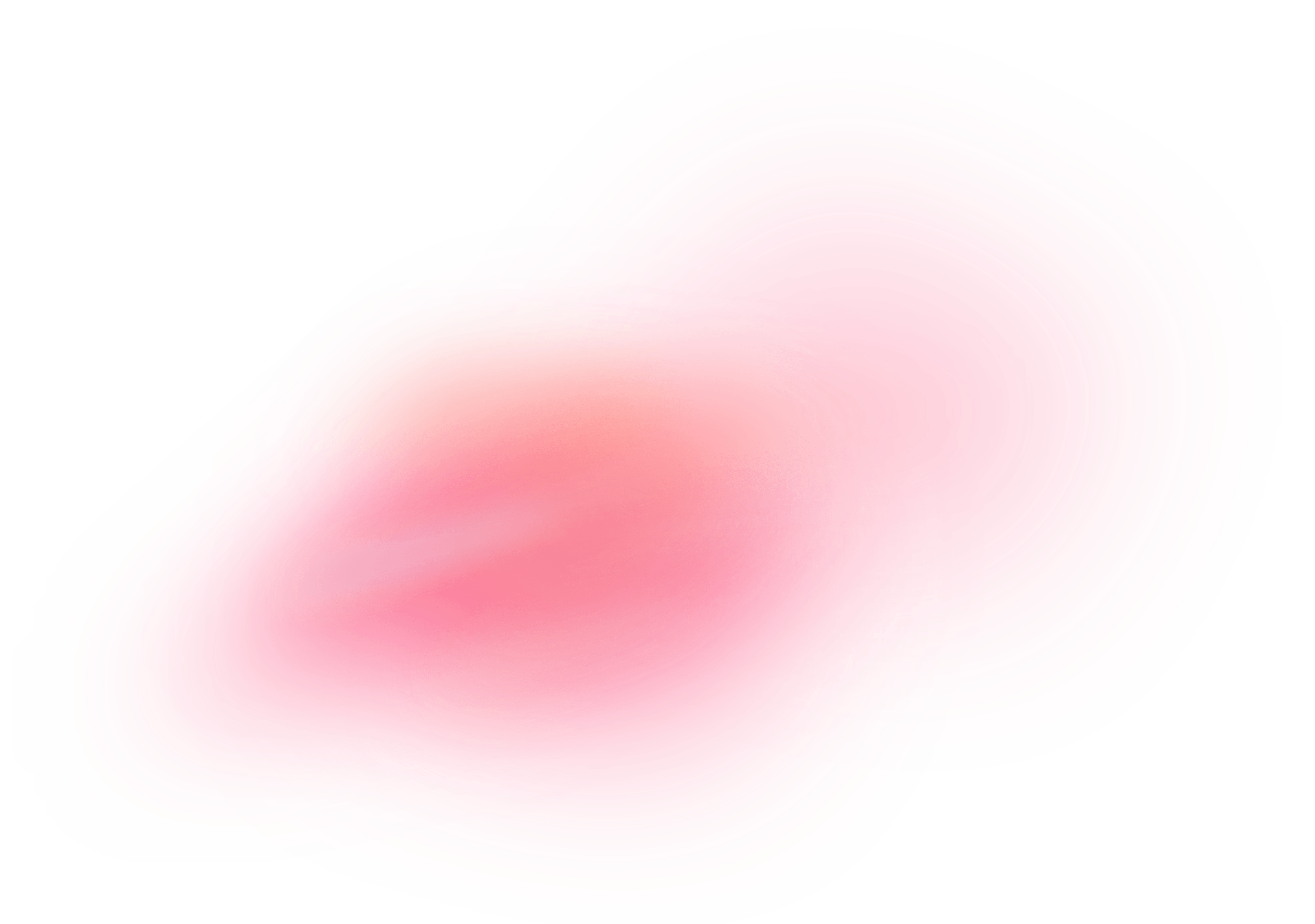