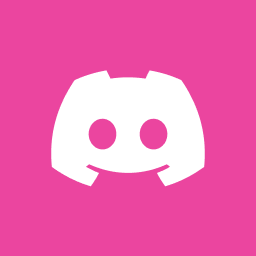
Hello,
I'm starting to learn Svelte and wanted to connect my little demo app with Appwrite. I saw the todo Svelte demo and was taking some of the logic in that demo but came across the error Argument of type 'User<Preferences>' is not assignable to parameter of type 'null | undefined'.
at the state.init(user);
line. also getting Type 'Alert' is not assignable to type 'null'
on the other line i have marked.
Not am I only new to Svelte but also typescript. Any ideas??
import { get, writable } from 'svelte/store';
import { sdk, server } from './appwrite';
export type Alert = {
color: string;
message: string;
};
const createState = () => {
const { subscribe, set, update } = writable({
account: null,
alert: null,
});
return {
subscribe,
signup: async (email: string, password: string, name: string) => {
return await sdk.account.create('unique()', email, password, name);
},
login: async (email: string, password: string) => {
await sdk.account.createEmailSession(email, password);
const user = await sdk.account.get();
-----> state.init(user);
},
logout: async () => {
await sdk.account.deleteSession('current');
},
alert: async (alert: Alert) =>
update((n) => {
------> n.alert = alert;
return n;
}),
init: async (account = null) => {
return set({ account, alert: null });
},
};
};
export const state = createState();```
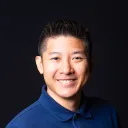
You need to define the type for your writable. Based on what you put:
const { subscribe, set, update } = writable({
account: null,
alert: null,
});
TypeScript infers account and alert can only be null so you can't assign anything to it. You would have to tell it it can be User or null...something like:
const { subscribe, set, update } = writable<{account: User | null, alert: Alert | null}>({
account: null,
alert: null,
});
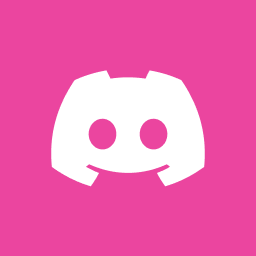
Hi Steven,
So that fixed the alert, but still having issues with state.init(user)
const user = await sdk.account.get();
user is defined as const user: Models.User<Models.Preferences>
I updated like you suggested
account: null,
alert: null,
});```
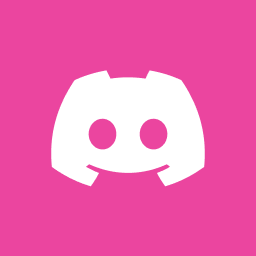
The error now is Cannot find name 'User'.ts(2304)
type User = /*unresolved*/ any
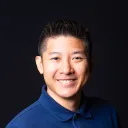
use Models.User<Models.Preferences>
isntead of just User
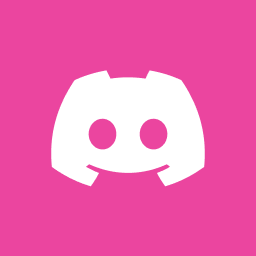
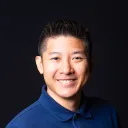
where is state?
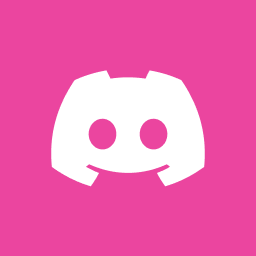
This is where I got all the logic from https://github.com/appwrite/demo-todo-with-svelte/blob/main/src/store.ts It is the very last line
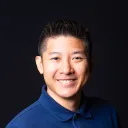
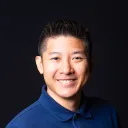
based on what i told you before, what do you think the fix is?
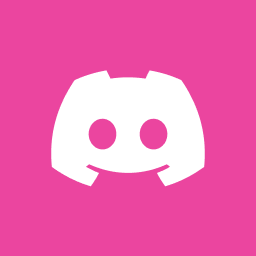
I think this is the answer? init: async (account: Models.User<Models.Preferences>) => {
return set({ account, alert: null });
},
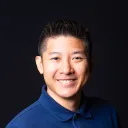
sounds about right...unless you should be allowed to pass null as well
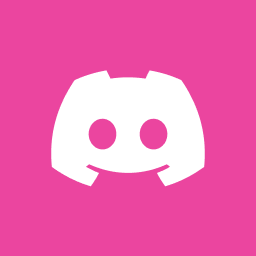
I'm trying to understand as best I can. I think I like dart much better than TypeScript haha.
I changed to:
return set({ account, alert: null });
},```
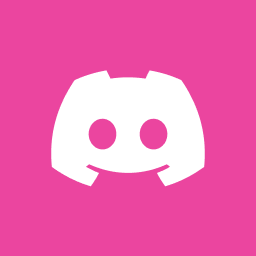
So I was able to get logged in by using state.login('user@test.com', 'password');
const { subscribe, set, update } = writable<{account: Models.User<Models.Preferences> | null, alert: Alert | null}>({
account: null,
alert: null,
});
return {
subscribe,
signup: async (email: string, password: string, name: string) => {
return await sdk.account.create('unique()', email, password, name);
},
login: async (email: string, password: string) => {
await sdk.account.createEmailSession(email, password);
const user = await sdk.account.get();
state.init(user);
},
logout: async () => {
await sdk.account.deleteSession('current');
},
alert: async (alert: Alert) =>
update((n) => {
n.alert = alert;
return n;
}),
init: async (account: Models.User<Models.Preferences> | null) => {
return set({ account, alert: null });
},
};
};
export const state = createState();```
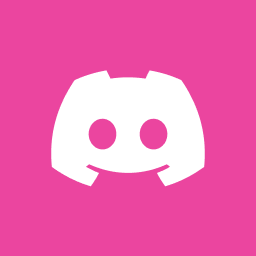
How can I get the logged in user's details?
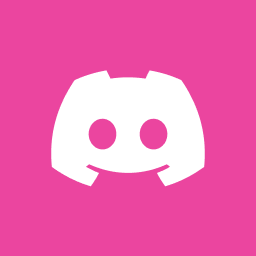
I see that init function uses set, which must be where it sets the account to the state
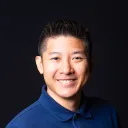
this repo might be pretty outdated...you might want to start fresh or something
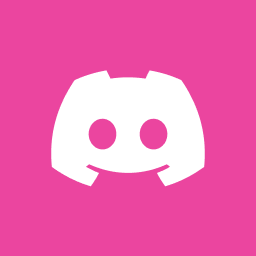
Ok. You have any links to resources or examples to get started with Appwrite and SvelteKit?
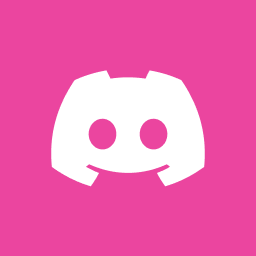
I tried searching and thats how I found this example
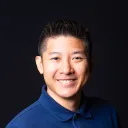
maybe focus on sveltekit by itself
Recommended threads
- Blob column?
Hi, I'm looking to use yjs/hocuspocus, and appwrite as the backend store, but I'm not finding a way to create a blob column in the database. Is there a way arou...
- Site Git deployment bug
I am facing issue when trying to create a site and deploy it into git, see attached video: ```json { "message": "When connecting to VCS (Version Control Sy...
- Cannot Create a Relationship Attribute
Related collections seems to be empty. Please help check. We are a subscriber of your platform.
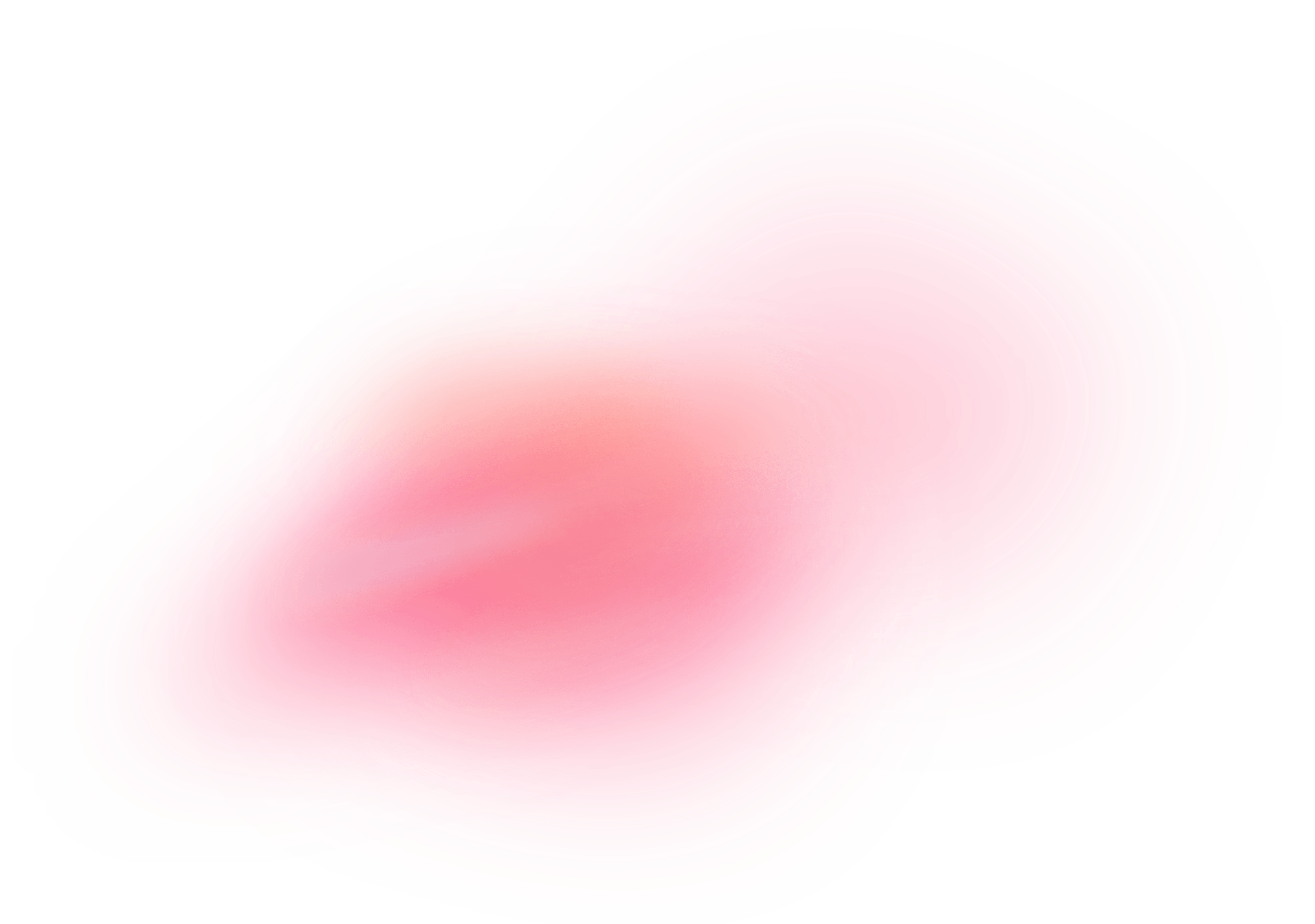