
This is my auth handler.
const createState = () => {
const { subscribe, set, update } = writable<State>({
account: null,
})
return {
subscribe,
signUp: async (email: string, password: string, name: string) => {
return await sdk.account.create('unique()', email, password, name)
},
signIn: async (email: string, password: string) => {
await sdk.account.createEmailSession(email, password)
const user = await sdk.account.get()
state.init(user)
},
signOut: async () => {
state.init(null)
await sdk.account.deleteSession('current')
},
init: async (account: Models.User<Models.Preferences> | null = null) => {
return set({ account })
},
oAuth: async (provider: string, redirectURL: string) => {
sdk.account.createOAuth2Session(provider, redirectURL)
const user = await sdk.account.get()
state.init(user)
}
}
}
This is my GitHub button.
<IconButton on:click={() => state.oAuth('github', `https://${data.hostname}`)}></IconButton>
When I click on the button, the redirect works and the page refreshes, but the app state does not change. I don't know how to get the user's details after OAuth2. Do I have to make a request to the GitHub API?

email and password works and allows me to extract the name

the github OAuth2 app seems to work fine as well
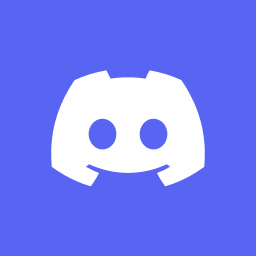
Hey
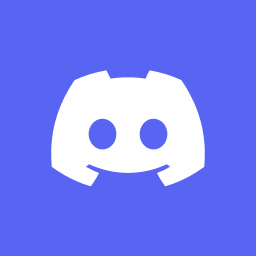
I am not well aware of Svelte but it seems that the state is not updating after the OAuth2 flow is completed.
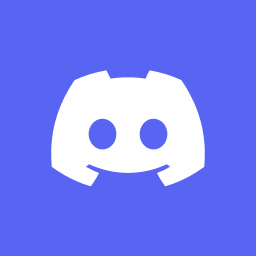
can you try something like this once? (Just an example where after the OAuth2 flow redirects back to your application, you can extract the authorization code or access token from the URL query parameters. Then, you can make a request to your backend (/api/github-oauth) or your own backend implementation with the auth code or access token. This backend endpoint should handle the authentication with the GitHub API, retrieve the user's details, and respond with the user object.)
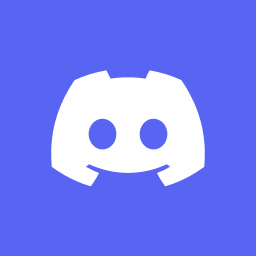
const createState = () => {
const { subscribe, set } = writable<State>({
account: null,
});
return {
subscribe,
signUp: async (email: string, password: string, name: string) => {
return await sdk.account.create('unique()', email, password, name);
},
signIn: async (email: string, password: string) => {
await sdk.account.createEmailSession(email, password);
const user = await sdk.account.get();
state.init(user);
},
signOut: async () => {
state.init(null);
await sdk.account.deleteSession('current');
},
init: async (account: Models.User<Models.Preferences> | null = null) => {
set({ account });
},
oAuth: async (provider: string, redirectURL: string) => {
sdk.account.createOAuth2Session(provider, redirectURL);
const urlParams = new URLSearchParams(window.location.search);
const code = urlParams.get('code');
const response = await fetch('/api/github-oauth', {
method: 'POST',
body: JSON.stringify({ code }),
headers: {
'Content-Type': 'application/json',
},
});
if (response.ok) {
const user = await response.json();
state.init(user);
} else {
}
},
};
};

should I be using the appwrite callback url for my github oauth app instead of my vercel url

because currently the page doesn't receive any query params after the refresh

it might sound bad but I use a modal in +layout.svelte
for auth
Recommended threads
- Auth Error
"use client"; import { useEffect } from "react"; import { getSessionCookie } from "@/actions/auth"; import { createBrowserSessionClient } from "@/lib/appwrite-...
- Prevent modifying specific attributes
How do I prevent user to only to be able to modify some of the attributes. Document level security gives full access to update whole document, what are the wor...
- Bypass Error When Creating Account With ...
Suppose user first uses email/pass for log in using xyz@gmail.com, few month later on decides to use google oauth2 with same xyz@gmail.com (or in reverse orde...
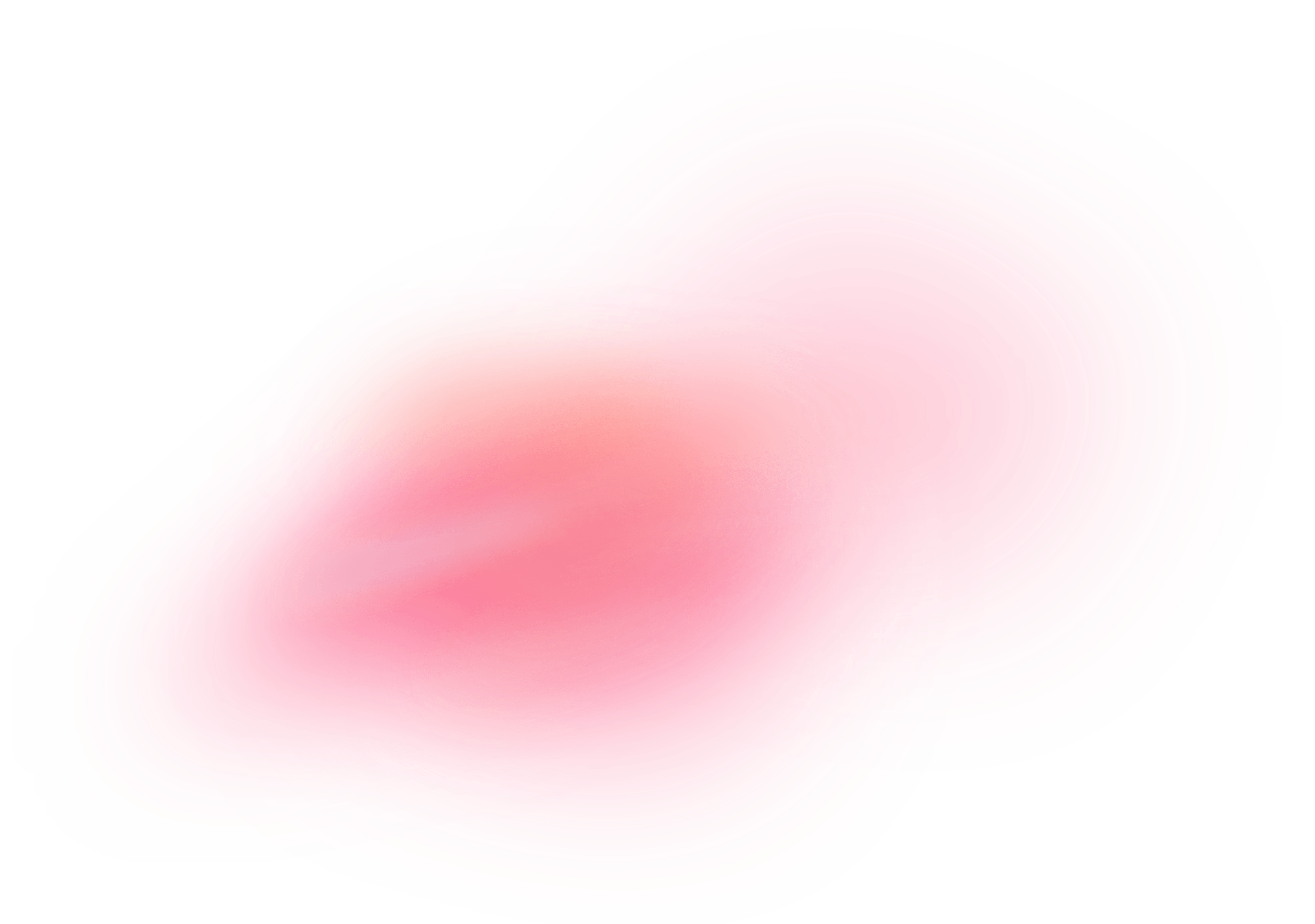