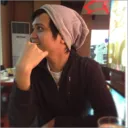
On page load I check if the user is logged in and then store that account information to a global state. The documentation says the account.get()
call throws an AppwriteException
so I wrap it in a try catch block but it still shows on the dev tools:
try {
const userSession = await account.get();
if (userSession) {
setSession(userSession);
}
} catch (error: any) {
// AppwriteException: User (role: guests) missing scope (account)
// User is not logged in - do nothing
}
Even though I wrapped this in a try catch block, I still get GET https://appwrite.mydomain.com/v1/account 401
on the dev tools. I know it is harmless but is it possible to not have that show up on the dev tools? Would it be possible to set these async calls so that you can destructure the data returned or the error if an error does occur:
const { data: userSession, error } = await account.get();
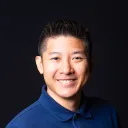
Yep, that's expected. You're doing it right. Those show up whenever there's any http call that results in > 400 status code
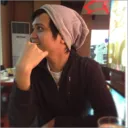
Oh I see.. hmmm.. I guess I can just leave it then. I just hate how it feels like I coded things wrong whenever I see errors like that on the console.
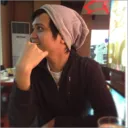
Isn't that what I'm already doing?
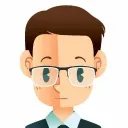
Yes, my bad

I feel that this is the right way of doing things... my only complain is with the "any" for the error type.... i just feel that it should be strongly typed... something like
catch (error: AppwriteException)
because the sdk always throws this...
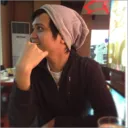
@Olivier Pavie no I think that's fine, in Javascript you can throw any errors as mentioned here https://fettblog.eu/typescript-typing-catch-clauses/ so it needs to be any
, you can then use instanceof
to check its type:
try {
const userSession = await account.get();
if (userSession) {
setSession(userSession);
}
} catch (error: any) {
if (error instanceof AppwriteException) {
if (error.message.toLowerCase() === 'user (role: guests) missing scope (account)') {
// AppwriteException: User (role: guests) missing scope (account)
// User is not logged in - do nothing
}
}
}
Recommended threads
- Need help setting up this error is showi...
You can't sign in to this app because it doesn't comply with Google's OAuth 2.0 policy. If you're the app developer, register the redirect URI in the Google Cl...
- Appwrite stopped working, I can't authen...
I'm having an issue with Appwrite. It was working fine just a while ago, but suddenly it stopped working for me and can't authenticate accounts. I even went bac...
- Fail to receive the verification email a...
I added my email address to prevent it from showing "appwrite," but now I'm not receiving emails for verification or password resets. The function appears to be...
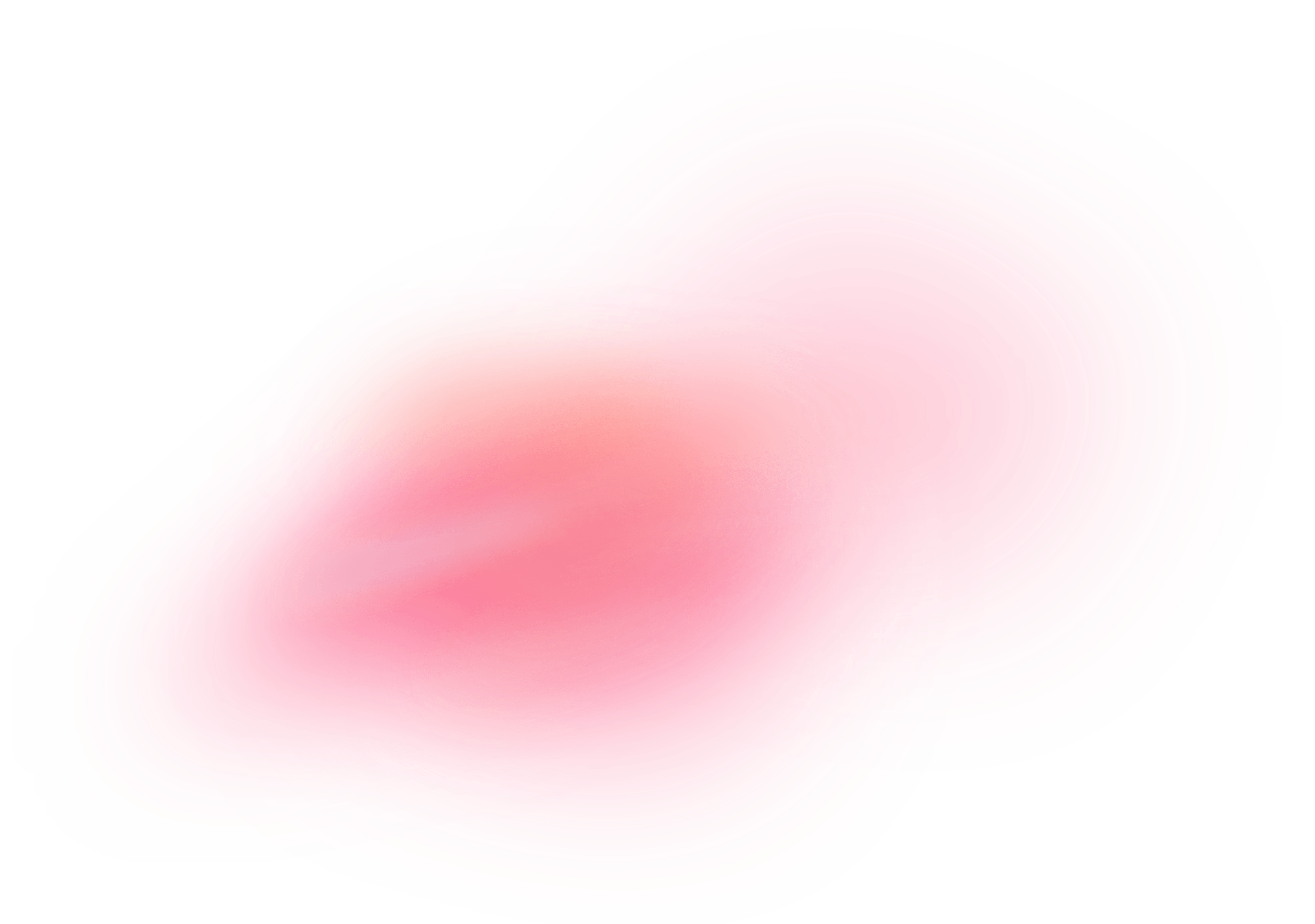