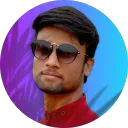
I am trying to upload a file from my backend to the storage bucket. An user will upload the file from the frontend and I am trying to upload that same to the appwrite storage bucket.
import multer from 'multer';
import sdk from 'node-appwrite';
import { appwrite } from '../../config/appwrite.config';
const router = Router();
const upload = multer();
router.post(
'/',
upload.single('photo'),
async (req: Request, res: Response, next: NextFunction) => {
try {
console.log(req.file?.buffer);
const storage = new sdk.Storage(appwrite);
const r = await storage.createFile(
process.env.APPWRITE_BUCKET_ID || '',
'122212',
sdk.InputFile.fromBuffer(
req.file?.buffer as Buffer,
req.file?.filename || ''
)
);
res.send(new ResponseHandler({r}));
} catch (error) {
next(error);
}
}
);
This is the code that I have written but while testing this I am getting back the below error
"error": {
"code": 400,
"type": "storage_file_empty",
"response": {
"message": "Empty file passed to the endpoint.",
"code": 400,
"type": "storage_file_empty",
"version": "0.10.44"
}
}
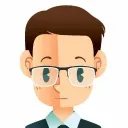
What is the log of this function?
console.log(sdk.InputFile.fromBuffer(
req.file?.buffer as Buffer,
req.file?.filename || ''
));
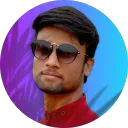
InputFile {
stream: Readable {
_readableState: ReadableState {
objectMode: true,
highWaterMark: 16,
buffer: BufferList { head: null, tail: null, length: 0 },
length: 0,
pipes: [],
flowing: null,
ended: false,
endEmitted: false,
reading: false,
constructed: true,
sync: true,
needReadable: false,
emittedReadable: false,
readableListening: false,
resumeScheduled: false,
errorEmitted: false,
emitClose: true,
autoDestroy: true,
destroyed: false,
errored: null,
closed: false,
closeEmitted: false,
defaultEncoding: 'utf8',
awaitDrainWriters: null,
multiAwaitDrain: false,
readingMore: false,
dataEmitted: false,
decoder: null,
encoding: null,
[Symbol(kPaused)]: null
},
_read: [Function: read],
_events: [Object: null prototype] {},
_eventsCount: 0,
_maxListeners: undefined,
[Symbol(kCapture)]: false
},
size: 241397,
filename: ''
}
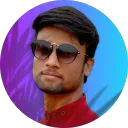
The function is returning back this
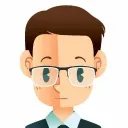
Okay, that's looks good, maybe the buffer
is empty
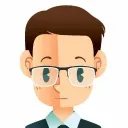
What you have here
console.log(req.file?.buffer)
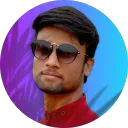
I have tried printing req.file?.buffer
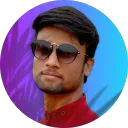
Let me show you the output
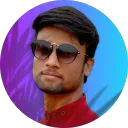
<Buffer 89 50 4e 47 0d 0a 1a 0a 00 00 00 0d 49 48 44 52 00 00 03 ac 00 00 03 14 08 06 00 00 00 b8 a2 aa 5c 00 00 00 01 73 52 47 42 00 ae ce 1c e9 00 00 00 04 ... 241347 more bytes>
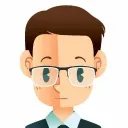
Okay, So it's good Let me check something
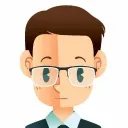
Okay
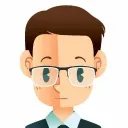
I thing you must give a filename for the file
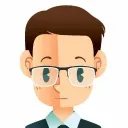
Change to this
sdk.InputFile.fromBuffer(
req.file?.buffer as Buffer,
req.file?.filename || 'filename'
)
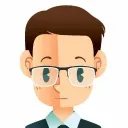
And, test it
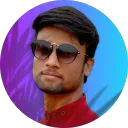
well I have not tried printing req.file.filename
let me check
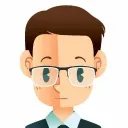
You can see here - at the end - it's empty
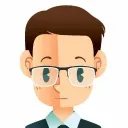
I think
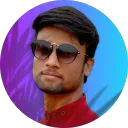
Resolved.
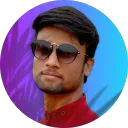
req.file.filename was empty so eventually an empty string was getting passed to the function
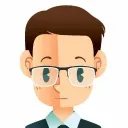
<a:agooglethumbsup:635256484682530825>
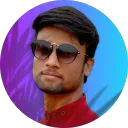
[Resolved] Facing issue while uploading file to the storage bucket
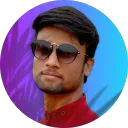
[SOLVED] Facing issue while uploading file to the storage bucket
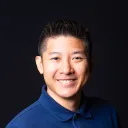
Recommended threads
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
- apple exchange code to token
hello guys, im new here 🙂 I have created a project and enabled apple oauth, filled all data (client id, key id, p8 file itself etc). I generate oauth code form...
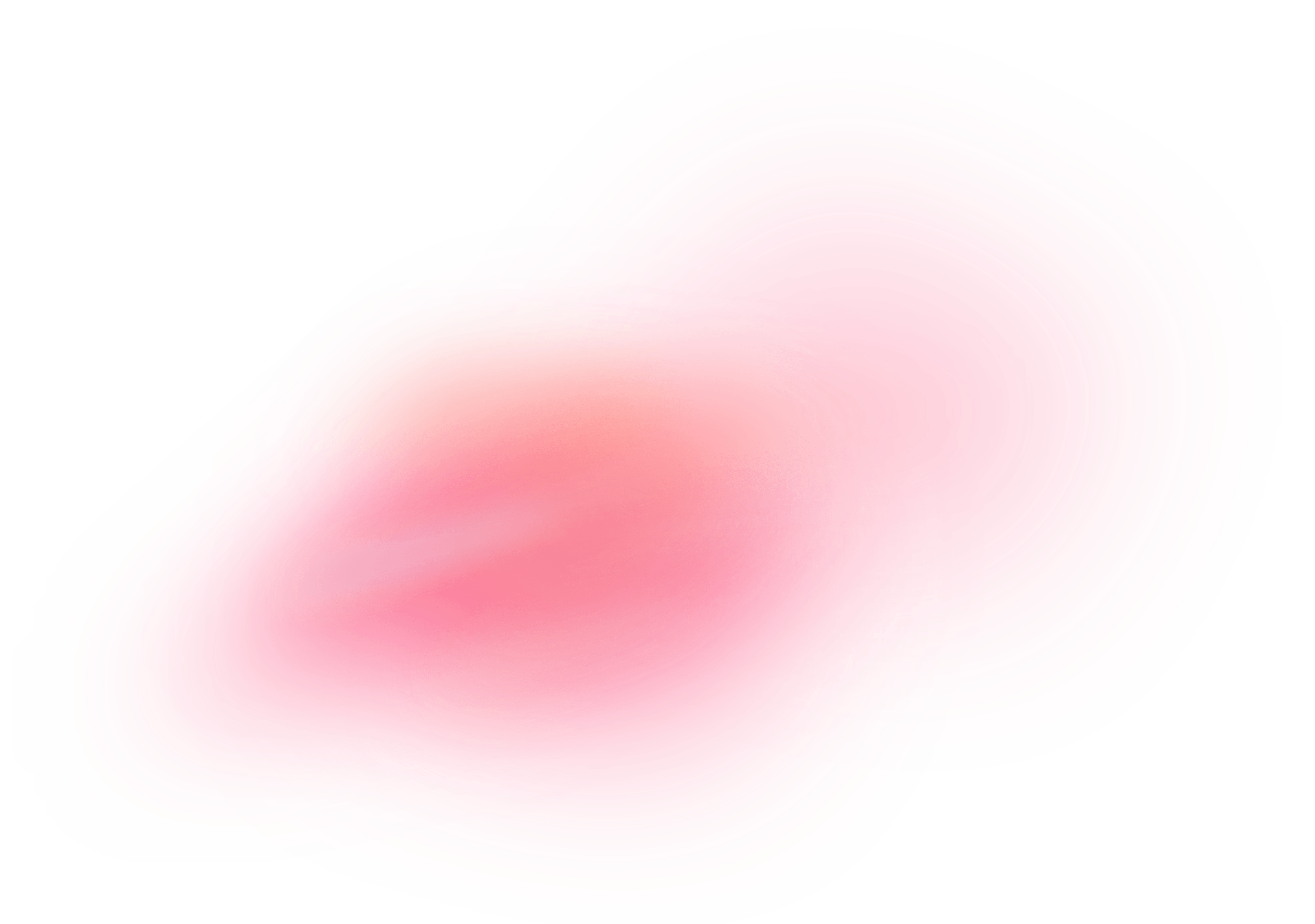