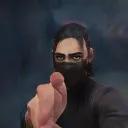
I try to dev a function for creating a new user. There is my function :
const sdk = require("node-appwrite");
module.exports = async function (req, res) {
const client = new sdk.Client();
const account = new sdk.Account(client)
const teams = new sdk.Teams(client);
if (
!req.variables['APPWRITE_FUNCTION_ENDPOINT'] ||
!req.variables['APPWRITE_FUNCTION_API_KEY']
) {
console.warn("Environment variables are not set. Function cannot use Appwrite SDK.");
} else {
client
.setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT'])
.setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID'])
.setKey(req.variables['APPWRITE_FUNCTION_API_KEY'])
.setSelfSigned(true);
}
const user = JSON.parse(req.variables["APPWRITE_FUNCTION_DATA"]);
try {
console.log('step1')
const {$id} = await account.create('unique()', user.email, user.password, user.name)
console.log('step2')
await databases.createDocument(req.variables["APPWRITE_FUNCTION_DATABASE_ID"], req.variables["APPWRITE_FUNCTION_COLLECTION_ID"],$id, {
"userId": $id,
"username": username
});
console.log('step3')
await account.createVerification(user.redirectURL)
} catch (error) {
console.log('error', error)
res.json({ error: 'Error creating user', error: error});
}
};
But the console stop at console.log('step1')
. There is an example of data that I pass to the function :
{
"email": "name@example.com",
"password":"password",
"name":"myname",
"username": "myusername",
"redirectURL":"http://localhost:3000/verifyEmail"
}
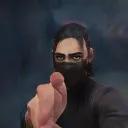
My Function API got this permissions
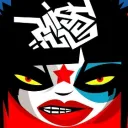
You need to use User endpoint : https://appwrite.io/docs/server/users?sdk=nodejs-default#usersCreate
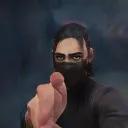
arhh im dumbb
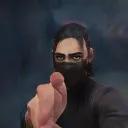
Well everything work except the .createVerification
function in my function :
const sdk = require("node-appwrite");
module.exports = async function (req, res) {
const client = new sdk.Client();
const account = new sdk.Account(client);
const users = new sdk.Users(client)
const databases = new sdk.Databases(client);
if (
!req.variables['APPWRITE_FUNCTION_ENDPOINT'] ||
!req.variables['APPWRITE_FUNCTION_API_KEY']
) {
console.warn("Environment variables are not set. Function cannot use Appwrite SDK.");
} else {
client
.setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT'])
.setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID'])
.setKey(req.variables['APPWRITE_FUNCTION_API_KEY'])
.setSelfSigned(true);
}
const user = JSON.parse(req.variables["APPWRITE_FUNCTION_DATA"]);
try {
const {$id} = await users.create('unique()', user.email, undefined, user.password, user.name)
await databases.createDocument(req.variables["APPWRITE_FUNCTION_DATABASE_ID"], req.variables["APPWRITE_FUNCTION_COLLECTION_ID"],$id, {
"userId": $id,
"username": user.username
});
await account.createVerification(user.redirectURL)
} catch (error) {
res.json({ error: 'Error creating user', error: error})
throw error
}
};
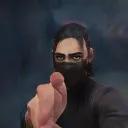
Ive got a role error : {"error":{"code":401,"type":"general_unauthorized_scope","response":{"message":"app.648cd565d979363284c1@service.cloud.appwrite.io (role: applications) missing scope (account)","code":401,"type":"general_unauthorized_scope","version":"0.10.42"}}}
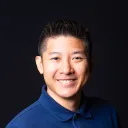
The account.createVerification
requires a user session which doesn't really exist server side. You'll need to manually make an API call to create an email session and create the email verification
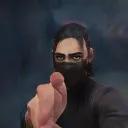
Okay so I cant createVerification without being connected ? What can I do if I don't want a user to be connected after signup ? I guess that I have to connect it automatically after the signup
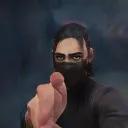
I've never used a JWT, but with this kind of token can't we authorize the function to create a verification email?
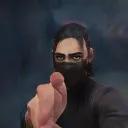
Like in this docs example :
const sdk = require('node-appwrite');
// Init SDK
const client = new sdk.Client();
const account = new sdk.Account(client);
client
.setEndpoint('https://cloud.appwrite.io/v1') // Your API Endpoint
.setProject('5df5acd0d48c2') // Your project ID
.setJWT('eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ...') // Your secret JSON Web Token
;
const promise = account.createVerification('https://example.com');
promise.then(function (response) {
console.log(response);
}, function (error) {
console.log(error);
});
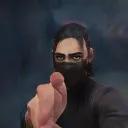
Well in any case I compared the speed of registration via an Appwrite function against the client-side, is a few seconds ago (about 5sec via the function) while it is instantaneous from the client-side. So if I have to log in to start an email check, I might as well stay on the client side
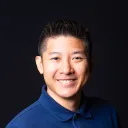
5s might be a cold start. It should be faster after that
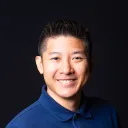
There's nothing wrong with allowing users to log in after creating an account
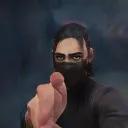
Yeah I just see a lot of website that doesnt log after signup ahah but ty a lot !
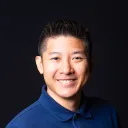
I would suggest restricting access to resources to verified users
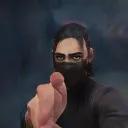
Yeah ive got 2 functions :
- user_create : add each new user in the
user
team - user_verified: add role
verified
when user verify his email address (but isnt working for now ahah)
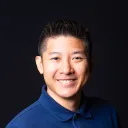
There are already built in roles for registered users and verified users 🧐
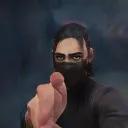
wait what ahah i wanna died
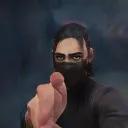
Well I just have to add that ?
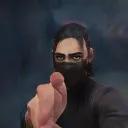
Maybe without the [] between verified
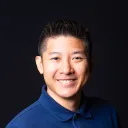
Recommended threads
- Functions page just got frozen when I tr...
Does someone know why this happens? would really appreaciate any help
- Github push not triggering function depl...
I'm using appwrite cloud instance and connected my github account to appwrite successfully. I also configured the git settings of my appwrite functions. however...
- Python Flask Project Deploy
I am trying to deploy a Python Project works with Flask on Appwrite, but this is not working, domain shows 500 Error and Logs for any execution is not loading ...
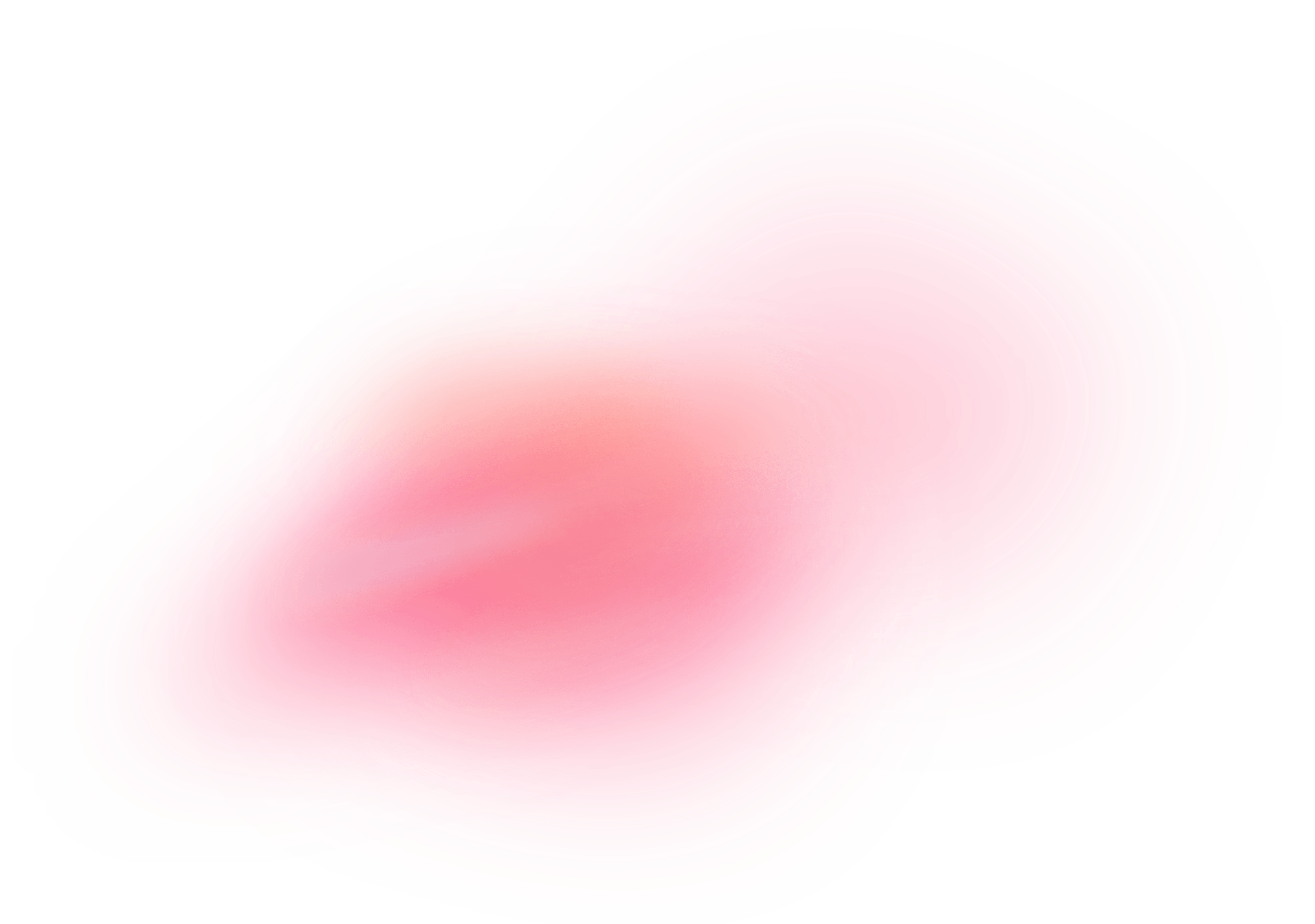