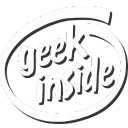
Hello,
I can create a valid session for a user account, but I cannot create a valid JWT token for the session...
Here's some sample code that illustrates the "problem":
import { Client, Account } from "appwrite";
const appwriteHost = "http://localhost:8123/v1";
const appwriteProjectId = "<projectID>";
const accountEmail = "someone@example.org";
const accountPassword = "password";
try {
const client = new Client()
.setEndpoint(appwriteHost)
.setProject(appwriteProjectId);
const account = new Account(client);
const sessionResponse = await account.createEmailSession(
accountEmail,
accountPassword
);
console.log(sessionResponse); // <- So far so good, a valid session is created
const jwtResponse = await account.createJWT(); // <- Fails, no way to link to the active session? (without eg cookies)
console.log(jwtResponse);
} catch (error) {
console.error(error);
}
The output (in my case) is similar to the following:
{
'$id': '1234567890',
'$createdAt': '2023-04-03T13:21:10.814+00:00',
userId: 'someone',
expire: '2024-04-02 13:21:10.616',
provider: 'email',
providerUid: 'someone@example.org',
providerAccessToken: '',
providerAccessTokenExpiry: '',
providerRefreshToken: '',
ip: 'xxx.xxx.xxx.xxx',
osCode: '',
osName: '',
osVersion: '',
clientType: 'library',
clientCode: '',
clientName: 'Node Fetch',
clientVersion: '1.0',
clientEngine: '',
clientEngineVersion: '',
deviceName: '',
deviceBrand: '',
deviceModel: '',
countryCode: '--',
countryName: 'Unknown',
current: true
}
AppwriteException: User (role: guests) missing scope (account)
at Client.<anonymous> (file:///path/to/source/node_modules/appwrite/dist/esm/sdk.js:385:27)
...
code: 401,
type: 'general_unauthorized_scope',
response: {
message: 'User (role: guests) missing scope (account)',
code: 401,
type: 'general_unauthorized_scope',
version: '1.2.1'
}
}
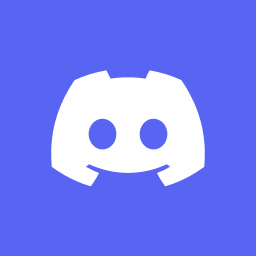
Hi
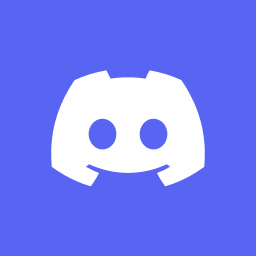
Allow me to look into this and get back to you
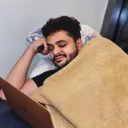
hey <@278993109852094464> are you using the nodejs sdk?
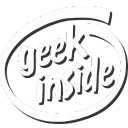
I'm using nodejs for the example app, but I'm using the appwrite
package (npm install appwrite --save
), not the node-appwrite
package
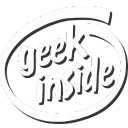
...essentially, I want to auth users on the server-side and then generate a JWT token for them - so I can make further user-scoped server-side requests. So, in essence, my use-case will be using both the appwrite
(to get
the JWT token) and node-appwrite
(to set
the JWT token) libraries. I'm thinking I should maybe ask a new question on the proposed flow of such a solution - since I cannot clearly see a method of generating a JWT token from a user session on the server-side. (Hope it makes sense.)
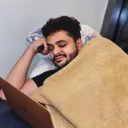
That makes sense. As far as I can see, there is no endpoint to create a JWT on server-side. I think what you can do is:
Auth on client side -> create a JWT on client side -> pass the JWT to a function that runs your operations on the server side
Recommended threads
- Can't create a function. The user interf...
I am trying to create a server-side function. I am on the free tier. **I already have three functions that work properly** that I created a few months ago. Now,...
- Data Diet Needed
I love the nested related data... but can we request limited data? I think my requests need to go on a diet. I return my courses, and the units all come with th...
- Appwrite Cloud Ui and Server Crashes rep...
I am having trouble with Appwrite cloud and server crashes and Ui crashed repeatedly. Raised a GitHub issue as well. https://github.com/appwrite/appwrite/issues...
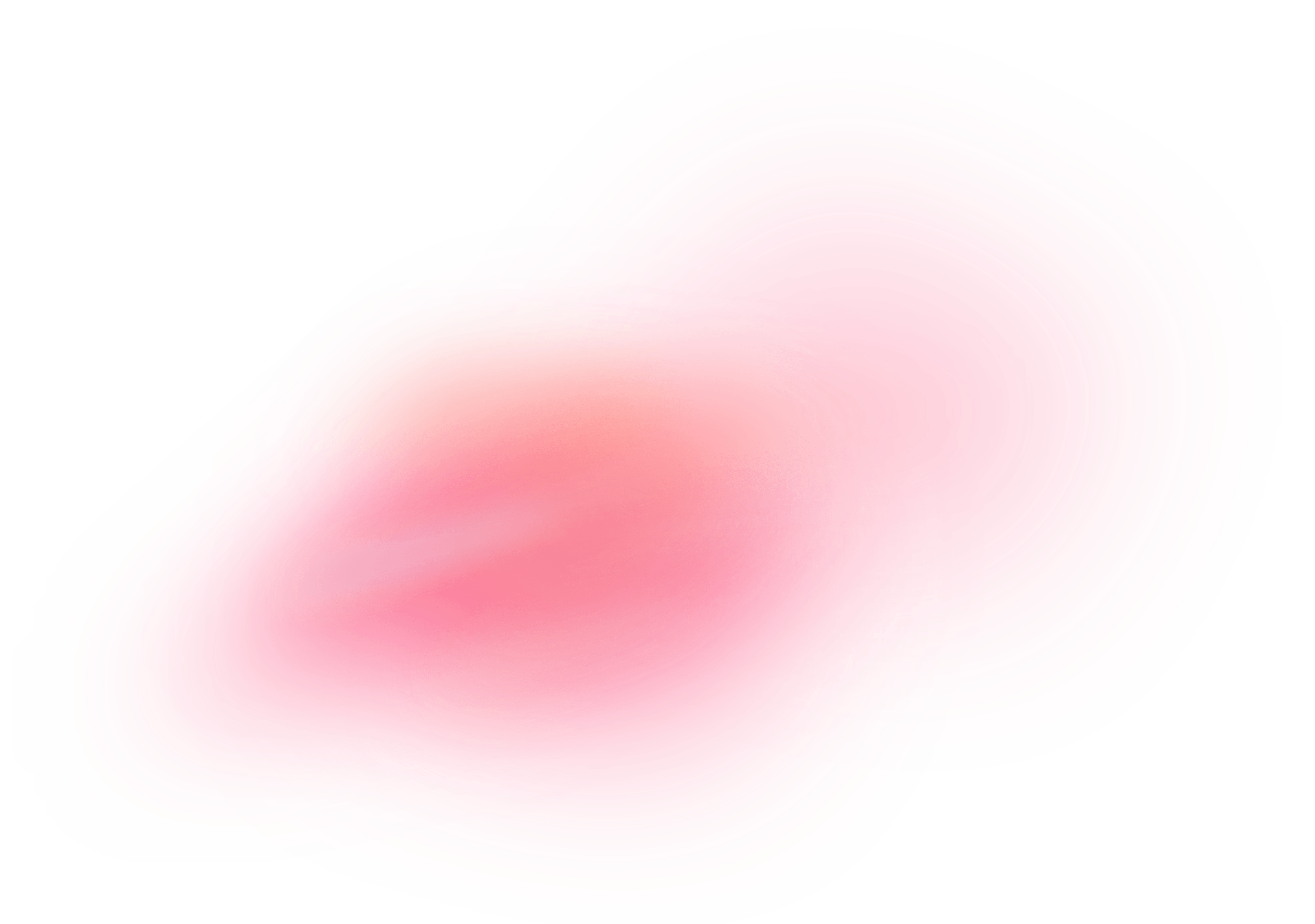