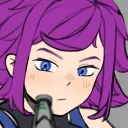
I was trying to follow the docs to activate MFA: https://appwrite.io/docs/products/auth/mfa
But when I called the account.updateMFA(true)
I got the following exception:
AppwriteException: Invalid document structure: Attribute "factors" must be an array
idk if there's a missing step in the docs or I'm doing it incorrectly
This is the code I'm using (it's vue 3, but this is just JS):
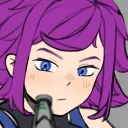
Here's the Vue code I'm using:
// STEP 1
const recoveryCodes = ref<Models.MfaRecoveryCodes | undefined>()
async function startMfaRecoveryCodes() {
try {
step.value = MFA_STEPS.RECOVERY_CODES
showMfaModal.value = true
const res = await appwriteStore.account.createMfaRecoveryCodes()
recoveryCodes.value = res
console.log('Step 1', res)
} catch (error) {
console.error(error)
if (error instanceof AppwriteException) {
alert(error.message)
return
}
alert('Error loading thing')
}
}
//STEP 2
const mfaUri = ref<string | undefined>()
const otp = ref('')
async function makeMfaAuth() {
try {
const res = await appwriteStore.account.createMfaAuthenticator(AuthenticatorType.Totp)
mfaUri.value = res.uri
console.log('Step 2', res)
step.value = MFA_STEPS.VERIFY_MFA_FACTOR
} catch (error) {
console.error(error)
if (error instanceof AppwriteException) {
alert(error.message)
return
}
alert('Error loading MFA')
}
}
// STEP 3
async function enableOtp() {
try {
const resMfaAuth = await appwriteStore.account.updateMfaAuthenticator(
AuthenticatorType.Totp,
otp.value,
)
console.log('Step 3: MFA', resMfaAuth)
const mfaFactors = await appwriteStore.account.listMfaFactors()
console.log(mfaFactors)
// THIS GENERATES ERROR
const resUpdate = await appwriteStore.account.updateMFA(true)
console.log('Step 3: Update', resUpdate)
alert('MFA activated')
authStore.reloadUserAccount()
showMfaModal.value = false
} catch (error) {
console.error(error)
if (error instanceof AppwriteException) {
alert(error.message)
return
}
alert('Error Validating OTP')
}
}
Recommended threads
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- Having issues with login via CLI
``` ~/appwrite appwrite login --endpoint https://localhost/v1 --verbose ? Enter your email myvalidemai...
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
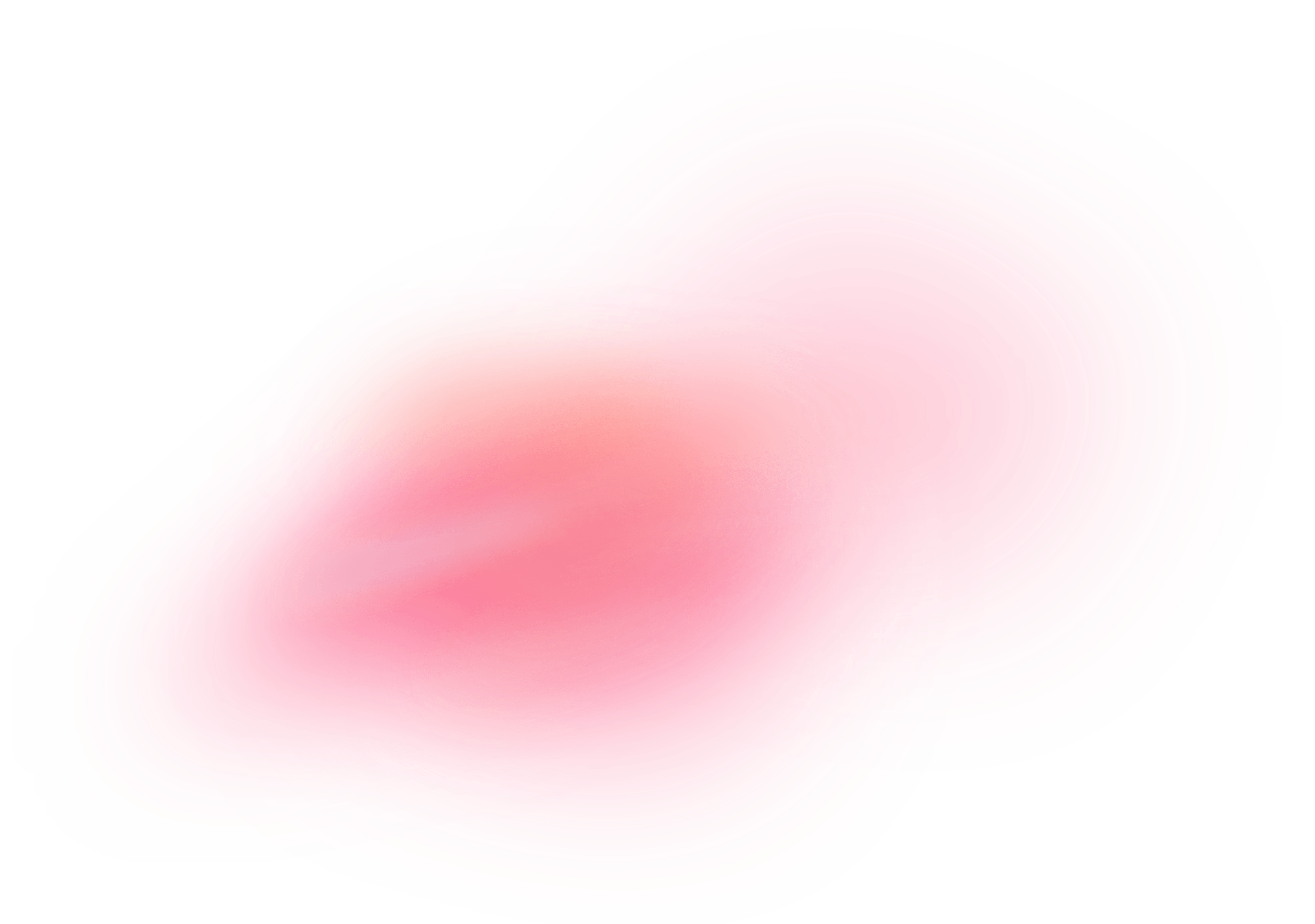