
I am working on a Node.js web function to retrieve data from an API and add it to my Appwrite database. However, when I tried debugging if the data is being retrieved, the callbacks from the http
function do not seem to work as I get an empty response when I try to execute the function
import { Client, Databases } from "node-appwrite";
import http from 'http';
export default async ({ req, res, log, error }) => {
const client = new Client();
const databases = new Databases(client);
client
.setEndpoint(process.env.APPWRITE_BACKEND_ENDPOINT)
.setProject(process.env.APPWRITE_PROJECT_ID)
if(req.method == "POST") {
let data = {};
const options = {
hostname: 'http://api.syntesio.ro',
port: 80,
path: '/SyntesioServices/api/v1/core/product/list',
method: 'GET',
headers: {
'Authorization': process.env.CHARA_API_AUTH_KEY
}
}
const request = http.request(options, (response) => {
response.setEncoding('utf-8');
response.on('data', (chunk) => {
return res.json(JSON.parse(chunk));
});
response.on('end', () => log(`Response data string end`));
})
request.on('error', (error) => error(error.message));
request.end();
return res.json(data);
}
};

Might be empty because it's moving on before it's actually returned, might want to change your logic to wait for the response before proceeding

how should i do it?

cause i wanna try to use async / await on the http.request
function but I didn't find any solutions

nevermind, our lord and saviour chatgpt solved it
Recommended threads
- Invalid document structure: missing requ...
I just pick up my code that's working a week ago, and now I got this error: ``` code: 400, type: 'document_invalid_structure', response: { message: 'Inv...
- custom domain with CloudFlare
Hi all, it seems that CloudFlare has blocked cross-domain CNAME link which made my app hostname which is in CloudFlare, unable to create a CNAME pointing to clo...
- Custom emails
What happen if I use a third party email provider to customize my emails and my plan run out of emails/month? Appwrite emails are used as fallback sending emai...
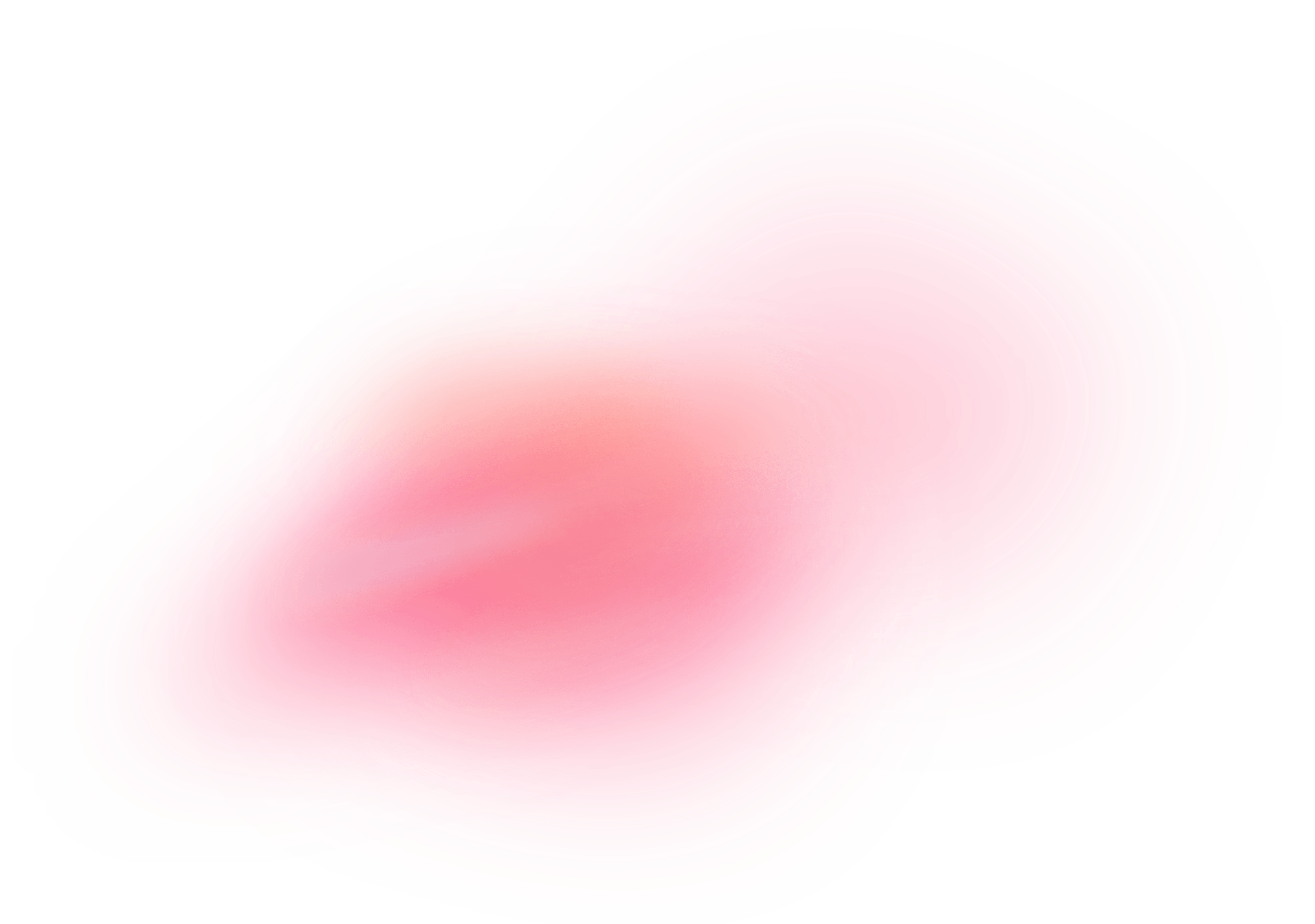