
Hello all, I need help. I'm not sure if this was just a TypeScript issue, but it relates to Appwrite so I figured I'd ask here.
I've created a function in my code to execute an Appwrite function, and I want to return the execution, but I'm running into loads of Typescript/ESLint errors. Would anybody be able to help me and tell me what I need to put in the defaultState value for the function? Here's a simplified version of my code with only relevant parts:
"use client"
import { functions } from '@/lib/appwrite';
import { AppwriteException, Models } from 'appwrite';
import {
useContext,
createContext,
ReactNode,
} from 'react';
export interface FlashcardState {
notes_to_cards: (notes: string) => Promise<Models.Execution>;
}
const defaultState: FlashcardState = {
notes_to_cards: ????
}
const flashcardContext = createContext<FlashcardState>(defaultState);
type FlashcardProviderProps = {
children: ReactNode;
};
export const FlashcardProvider = ({ children }: FlashcardProviderProps) => {
const notes_to_cards = async (notes: string) => {
let payload = {
notes: notes
}
try {
const execution = await functions.createExecution('<FUNCTION ID>', JSON.stringify(payload), false, "/", "POST")
if(execution.status == 'failed') throw new Error('Failed to execute function')
return execution
} catch(error) {
if (error instanceof AppwriteException) {
console.error(error.message);
} else {
console.error(error);
}
throw error
}
}
return (
<flashcardContext.Provider
value={{ notes_to_cards }}
>
{children}
</flashcardContext.Provider>
);
};
export const useFlashcards = () => {
const context = useContext<FlashcardState>(flashcardContext);
return context;
};
Recommended threads
- Different appwrite IDs are getting expos...
File_URL_FORMAT= https://cloud.appwrite.io/v1/storage/buckets/[BUCKET_ID]/files/[FILE_ID]/preview?project=[PROJECT_ID] I'm trying to access files in my web app...
- Got message for auto payment of 15usd fo...
how did this happen? 1. i claimed my 50usd credits via jsm hackathon - https://hackathon.jsmastery.pro/ 2. it asked me which org. to apply the credits on, i se...
- Invalid document structure: missing requ...
I just pick up my code that's working a week ago, and now I got this error: ``` code: 400, type: 'document_invalid_structure', response: { message: 'Inv...
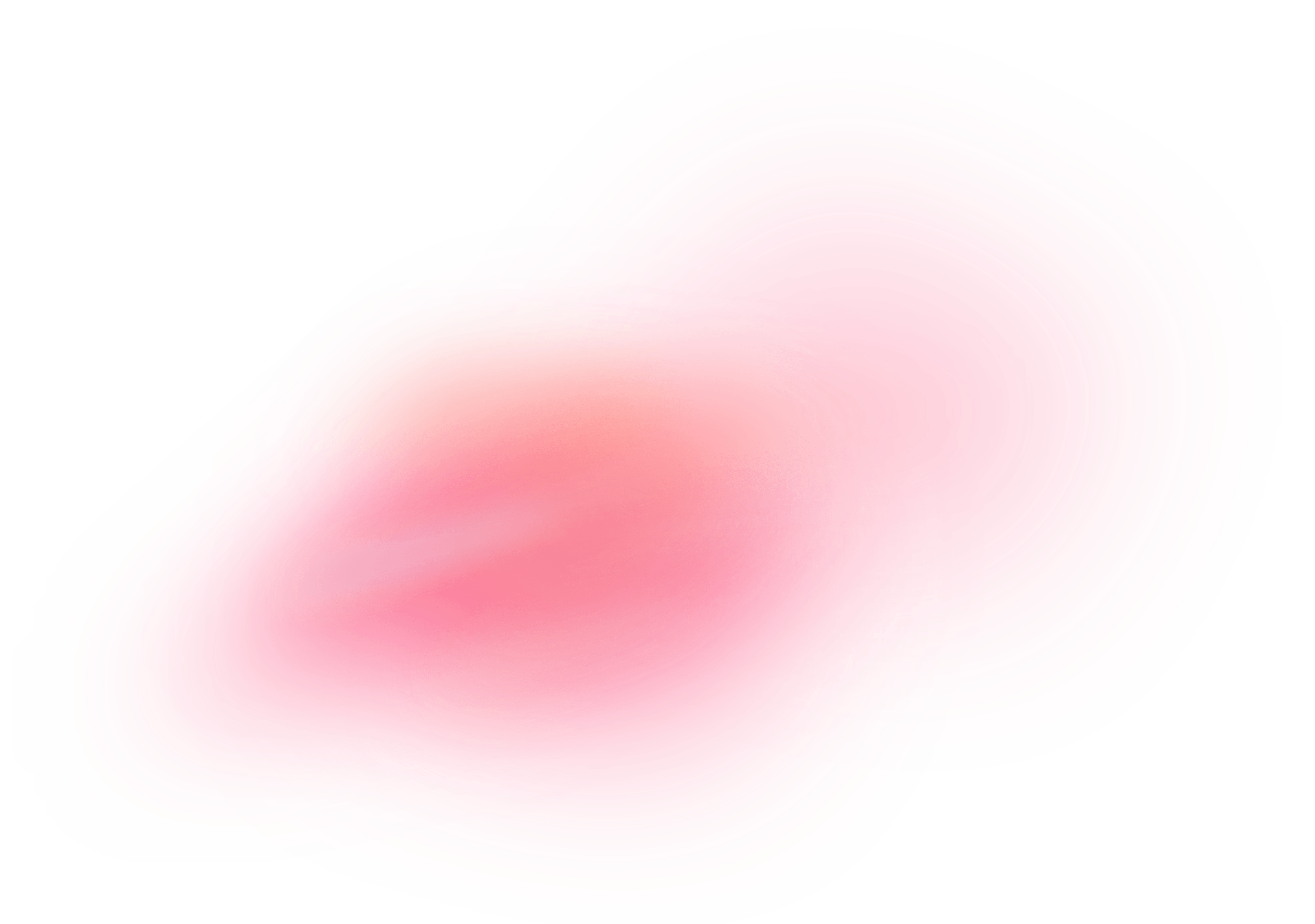