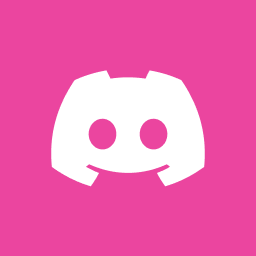
import { zodResolver } from "@hookform/resolvers/zod" import { useForm } from "react-hook-form" import * as z from "zod" import { useNavigate } from "react-router-dom";
import { Button } from "@/components/ui/button" import { Form, FormControl, FormField, FormItem, FormLabel, FormMessage, } from "@/components/ui/form" import { Input } from "@/components/ui/input" import { Textarea } from "../ui/textarea" import FileUploader from "../shared/FileUploader" import { PostValidation } from "@/lib/validation" import { Models } from "appwrite" import { useUserContext } from "@/context/AuthContext" import { useToast } from "../ui/use-toast" import { useCreatePost } from "@/lib/react-query/queriesAndMutations";
type PostFormProps = { post?: Models.Document; }
const PostForm = ({ post }: PostFormProps) => { const { mutateAsync: createPost, isPending: isLoadingCreate } = useCreatePost(); const { user } = useUserContext(); const { toast } = useToast(); const navigate = useNavigate();
// 1. Define your form schema.
const form = useForm<z.infer<typeof PostValidation>>({ resolver: zodResolver(PostValidation), defaultValues: { caption: post ? post?.caption : "", file: [], location: post ? post?.location : "", tags: post ? post.tags.join(',') : "" }, })
// 2. Define a submit handler. async function onSubmit(values: z.infer<typeof PostValidation>) { const newPost = await createPost({ ...values, userId: user.id, }) if(!newPost) { toast({ title: 'Please try again' }) } navigate('/');
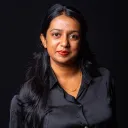
Also, please share your project id
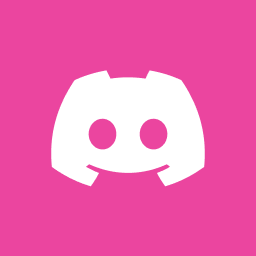
VITE_APPWRITE_PROJECT_ID='657b55c396bd6978305a'
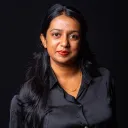
Btw, for easier readability of code, it is better to enclose it within three backticks `
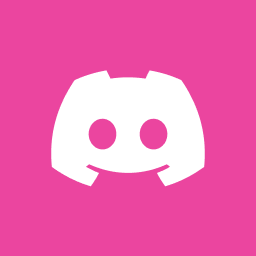
i am in the learning process... how do i do that?
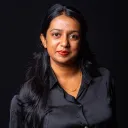
Just add this backtick thrice at the start and end of your code
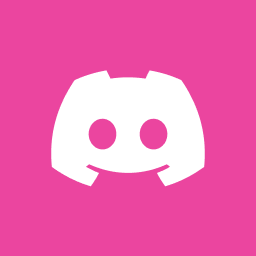
okay
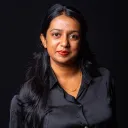
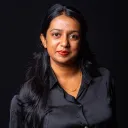
@stella can you share a screenshot of the attributes from the console?
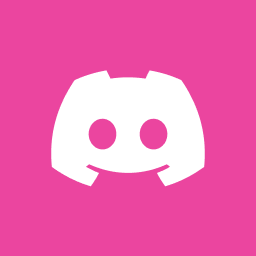
sure
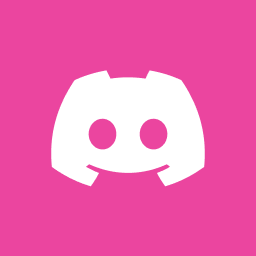
console error message?
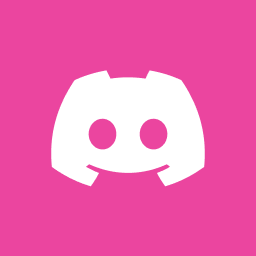
before it was status 500 but at the moment no error in console
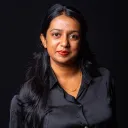
Nope, the attributes you created. Can you show a screenshot of that?
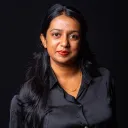
That means it is working now? 🤔
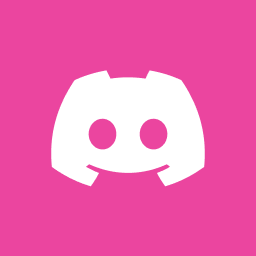
let me share
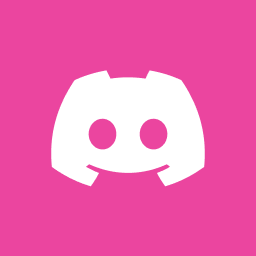
no it has not added post to appwrite
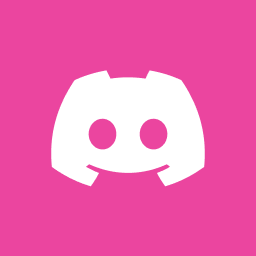
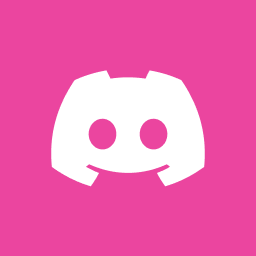
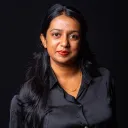
Are any attributes pending or failed?
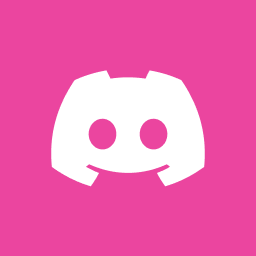
not sure😫
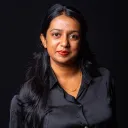
You will be able to see it in the console itself 😅
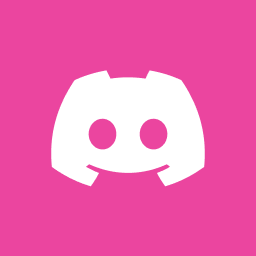
let me see
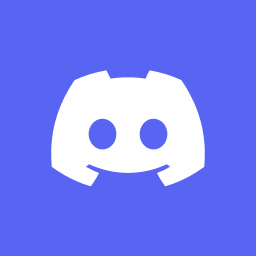
Do you have set permissions?
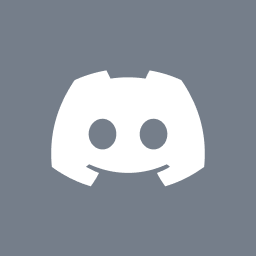
when you create a document you must precise after the data an array for permission like this [ Permission.write(Role.any()) but it depends of the role for this table. for me i put in settings of my table role : any
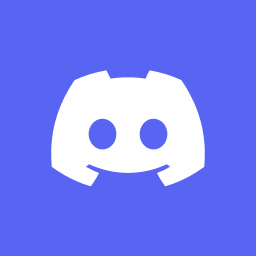
Role.any will make everyone access to it and the user maybe wants to limit access to some users
Recommended threads
- Storage files aren’t showing on mobile
hi, what can be the problem? i have a storage and there are images in it, the permissions are read for All Users, everything is fine on desktop, but on mobile t...
- Delete storage files all at once
I am using Appwrite Cloud. I want to delete all storage files in my bucket similar to how I can select all documents in a collection and delete them all at once...
- Storage images are not showing on mobile
Hi, can anyone help me? I have some images on the storage, and it is not shown on mobile, on desktop everything is fine.
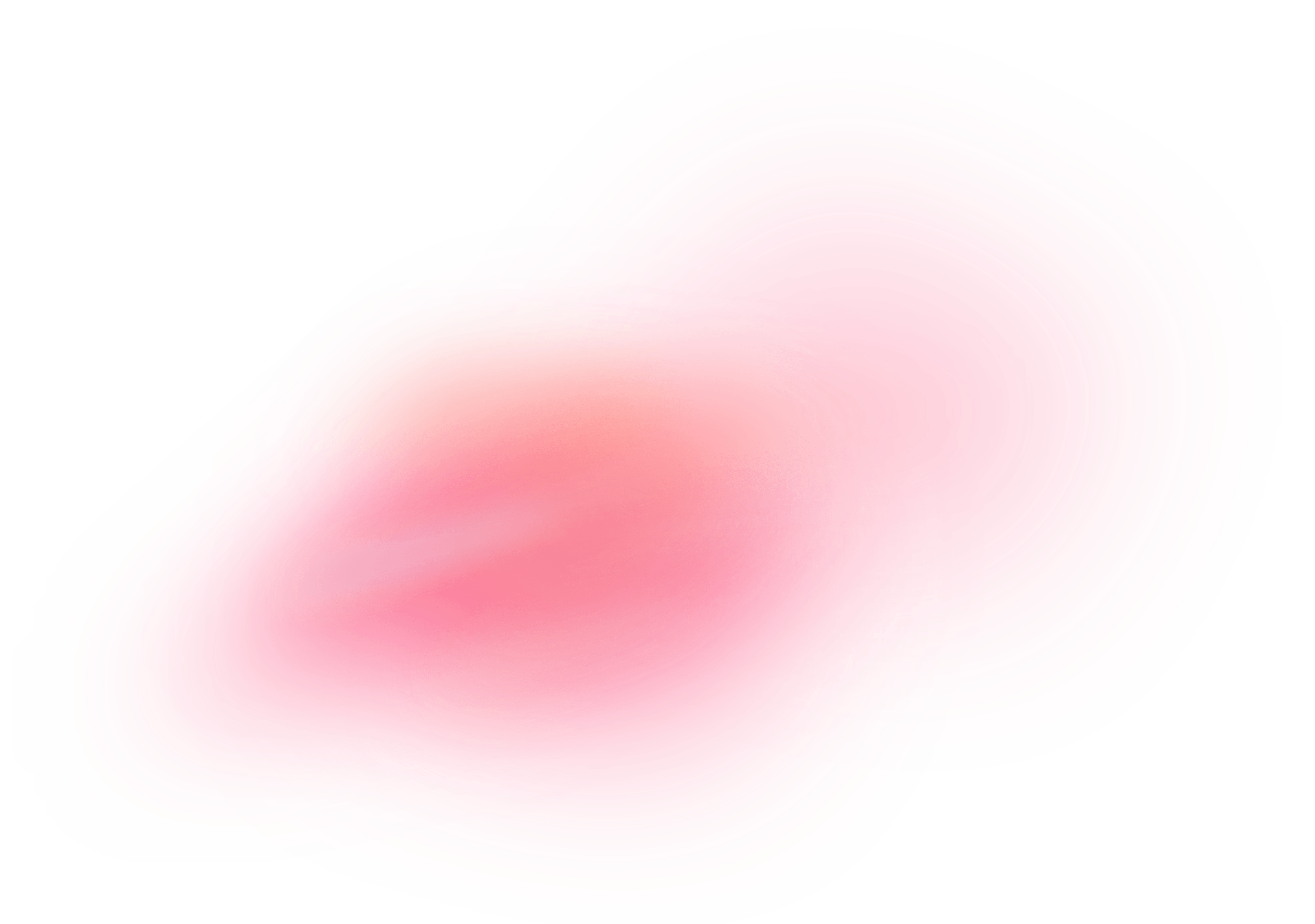