[SOLVED] AppwriteException: Invalid `email` param: Value must be a valid email address
- 0
- Self Hosted
- Web

import React, { useState } from "react";
import { account } from "../appwrite/appwriteConfig";
import { useNavigate } from "react-router-dom";
import { v4 as uuidv4 } from "uuid";
import "../styles/Login.css";
function Register() {
const navigate = useNavigate();
const [user, setUser] = useState({
name: "",
email: "",
password: "",
});
const isValidEmail = (email) => {
// Regular expression for a simple email validation
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(email);
};
const registerUser = async (e) => {
e.preventDefault();
if (!isValidEmail(user.email)) {
console.log("Invalid email address");
return;
}
const promise = account.create(
uuidv4(),
user.name,
user.email,
user.password
);
promise.then(
(res) => {
console.log(res);
navigate("/todolist");
},
(err) => {
console.log(err);
}
);
};
return (
<div className="landing">
<div className="register">
<h2>Register</h2>
<form onSubmit={registerUser}>
<label htmlFor="name">Name: </label>
<input
type="text"
onChange={(e) => setUser({ ...user, name: e.target.value })}
/>
<label htmlFor="email">Email: </label>
<input
type="email"
onChange={(e) => setUser({ ...user, email: e.target.value })}
/>
<label htmlFor="password">Password: </label>
<input
type="password"
onChange={(e) => setUser({ ...user, password: e.target.value })}
/>
<button type="submit">Register</button>
<p>Already an user?</p>
<button onClick={()=>navigate('/')}>Login</button>
</form>
</div>
</div>
);
}
export default Register;
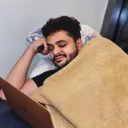
const promise = account.create(
uuidv4(),
user.name,
user.email,
user.password
);
This is not the correct format. Have a look at the docs for the correct format.
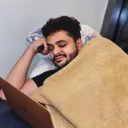
Any reason why you are using the uuid
package instead of the ID.unique()
helper that comes with the appwrite
sdk?
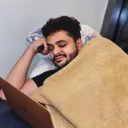
const promise = account.create(
ID.unique(),
user.email,
user.password,
user.name,
);

I was following a tutorial. In the tutorial, he was using a older version of appwrite i guess. Ok i will see the docs and give a try.

still getting the same error
AppwriteException: Invalid `email` param: Value must be a valid email address
at Client.<anonymous> (http://localhost:3000/static/js/bundle.js:49742:17)
at Generator.next (<anonymous>)
at fulfilled (http://localhost:3000/static/js/bundle.js:49376:24)
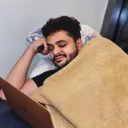
Which version of Appwrite are you using?

13.0.0 latest i guess
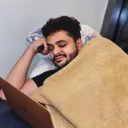
I mean the server version.

1.4.3
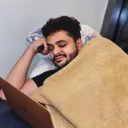
Just to be sure, can you share the updated code again?

import React, { useState } from "react";
import { ID } from 'appwrite'
import { account } from "../appwrite/appwriteConfig";
import { useNavigate } from "react-router-dom";
import "../styles/Login.css";
function Register() {
const navigate = useNavigate();
const [user, setUser] = useState({
name: "",
email: "",
password: "",
});
const registerUser = async (e) => {
e.preventDefault();
const promise = account.create(
ID.unique(),
user.name,
user.email,
user.password
);
promise.then(
(res) => {
console.log(res);
navigate("/todolist");
},
(err) => {
console.log(err);
}
);
};
return (
<div className="landing">
<div className="register">
<h2>Register</h2>
<form onSubmit={registerUser}>
<label htmlFor="name">Name: </label>
<input
type="text"
onChange={(e) => setUser({ ...user, name: e.target.value })}
/>
<label htmlFor="email">Email: </label>
<input
type="text"
onChange={(e) => setUser({ ...user, email: e.target.value })}
/>
<label htmlFor="password">Password: </label>
<input
type="password"
onChange={(e) => setUser({ ...user, password: e.target.value })}
/>
<button type="submit">Register</button>
<p>Already an user?</p>
<button onClick={()=>navigate('/')}>Login</button>
</form>
</div>
</div>
);
}
export default Register;
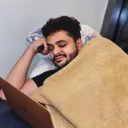
the order is wrong
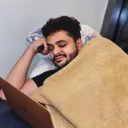
it needs to be
- id
- password
- name
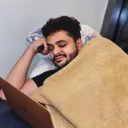
you’ve done
- id
- name
- password
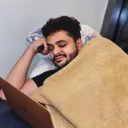
make sure the order is correct in the account.create
call

ohhhh.. thanks dude you saved the day 🥲
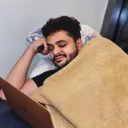
[SOLVED] AppwriteException: Invalid email
param: Value must be a valid email address
Recommended threads
- Error getting session: AppwriteException...
I get this error `Error getting session: AppwriteException: User (role: guests) missing scope (account)` when running in prod. As soon as I try running my app o...
- Unable to View / Edit Bucket Files
Hi! I am unable to view / edit Bucket Files. While Previews work just fine, clicking the actual file to view or edit it produces the errors seen in the attache...
- How to remove the Sign up link after cre...
Greetings, i just installed appwrite on a VPS and created an account but now i do not want others to have access to the sign-up page. Is there any way to hide o...
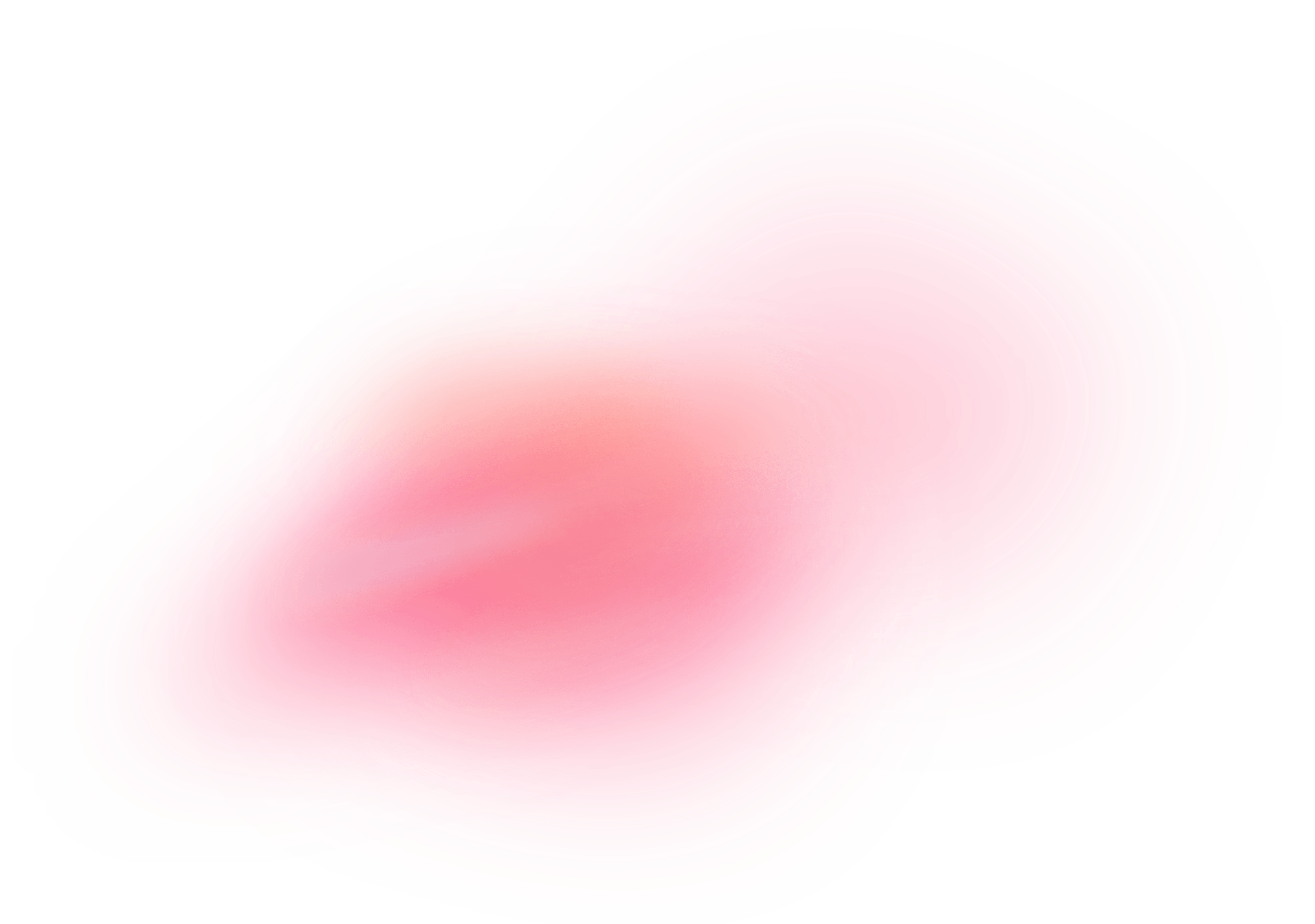