[SOLVED]Getting AppwriteException: general_unauthorized_scope (account) (401) when login.
- 0
- General
- Flutter
- Accounts

Here is my code, I've narrowed it down to currentUserId = await _account().get(); and the _getUser(); which run the same code during login.
//sign in method using email or username
@override
FutureEither signIn(
{required String email,
required String password,
required BuildContext context}) async {
try {
if (isValidEmail(email)) {
final session = await context.authNotifier
.createEmailSession(email: email, password: password);
_currentUser = await _account.get();
return right(session);
} else {
final document = await _db.listDocuments(
databaseId: AppwriteConstants.databaseId,
collectionId: AppwriteConstants.usersCollection,
queries: [
Query.search('userName', email),
],
);
final user = UserModel.fromMap(document.documents.first.data);
final responseWithUserName =
await context.authNotifier.createEmailSession(
email: user.email,
password: password,
);
_currentUser = await _account.get();
return right(responseWithUserName);
}
} on AppwriteException catch (e, stackTrace) {
return left(
Failure(e.message ?? 'Some unexpected error occurred', stackTrace));
} catch (e, stackTrace) {
return left(
Failure(e.toString(), stackTrace),
);
}
}```

.createEmailSession```
```dart
Future<bool> createEmailSession({
required String email,
required String password,
bool notify = true,
}) async {
_status = AuthStatus.authenticating;
if (notify) {
notifyListeners();
}
try {
await _account.createEmailSession(email: email, password: password);
_getUser(notify: notify);
return true;
} on AppwriteException catch (e) {
_error = e.message;
_status = AuthStatus.unauthenticated;
if (notify) {
notifyListeners();
}
return false;
}
}```

//code from _getUser()
Future _getUser({bool notify = true}) async {
try {
_user = await _account.get();
_status = AuthStatus.authenticated;
} on AppwriteException catch (e) {
_status = AuthStatus.unauthenticated;
_error = e.message;
} finally {
_loading = false;
if (notify) {
notifyListeners();
}
}
}```

Oh and isValidEmail
is just a regex email checker
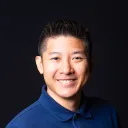
Do you only have one instance of Client?

Yes

I made this package https://pub.dev/packages/appwrite_auth_kit local since it's outdated and I want to use the latest flutter and appwrite package 11.0
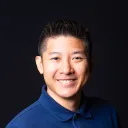
Does clearing the application data make a difference?

I haven't tried

But it's caused a lot of pain. I accidentally deleted my whole appwrite docker package last night ๐คฃ

Just a flutter clean?

Funny thing is this bug came out of nowhere
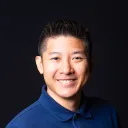
On your device, there might be something in the settings for the app. Or you can uninstall the app

Also my SMTP server doesn't work anymore not sure if related

I've uninstalled but that doesn't help let me try to clear the device

Erased all content and settings from the device, I'll keep you updated ๐

I sent the app to Apple for app review and the same thing, they said they recieved a white page when logging. Which is the same bug

For my platform integrations I selected flutter then ios. Not sure if I should add the ios one or the flutter ios one but since the real ios wanted me to add swift I used the flutter ios platform integration

Same error after clearing the device

I can only login after a hot reload

I've only seen the error I'm talking about in older github reports

Solved the error, I was right about the _getUser() method it was being called before the user even logged in. All I did was comment it out from the initializer and it works. Here's the code ```dart enum AuthStatus { uninitialized, authenticated, authenticating, unauthenticated, }
class AuthNotifier extends ChangeNotifier { late final Account _account; final Client _client; AuthStatus _status = AuthStatus.uninitialized;
User? _user; String? _error; late bool _loading;
AuthNotifier(Client client) : _client = client { _error = ''; _loading = true; _account = Account(client); //_getUser(); }
Account get account => _account; Client get client => _client; String? get error => _error; bool get isLoading => _loading; User? get user => _user; AuthStatus get status => _status;
Future _getUser({bool notify = true}) async { try { _user = await _account.get(); _status = AuthStatus.authenticated; } on AppwriteException catch (e) { _status = AuthStatus.unauthenticated; _error = e.message; } finally { _loading = false; if (notify) { notifyListeners(); } } }``` Sorry for all the trouble @Steven ๐

[SOLVED]Getting AppwriteException: general_unauthorized_scope (account) (401) when login.
Recommended threads
- Flutter Google Auth (Access blocked: Thi...
It is working fine on web platform, but on Flutter I'm getting this error. Any option to fix, or this issue with AppWrite and need to use different option? Ac...
- Appwrite Education program
I have applied for the GitHub Student Developer Pack and it was approved so that i can be eligible for the Appwrite Education program in Appwrite BUT it is sh...
- Relationships restricted to a max depth ...
When I do query like: ``` await _databases.listDocuments( databaseId: AppwriteConfig.DATABASE_ID, collectionId: AppwriteConfig.SERVICES_COLLECTI...
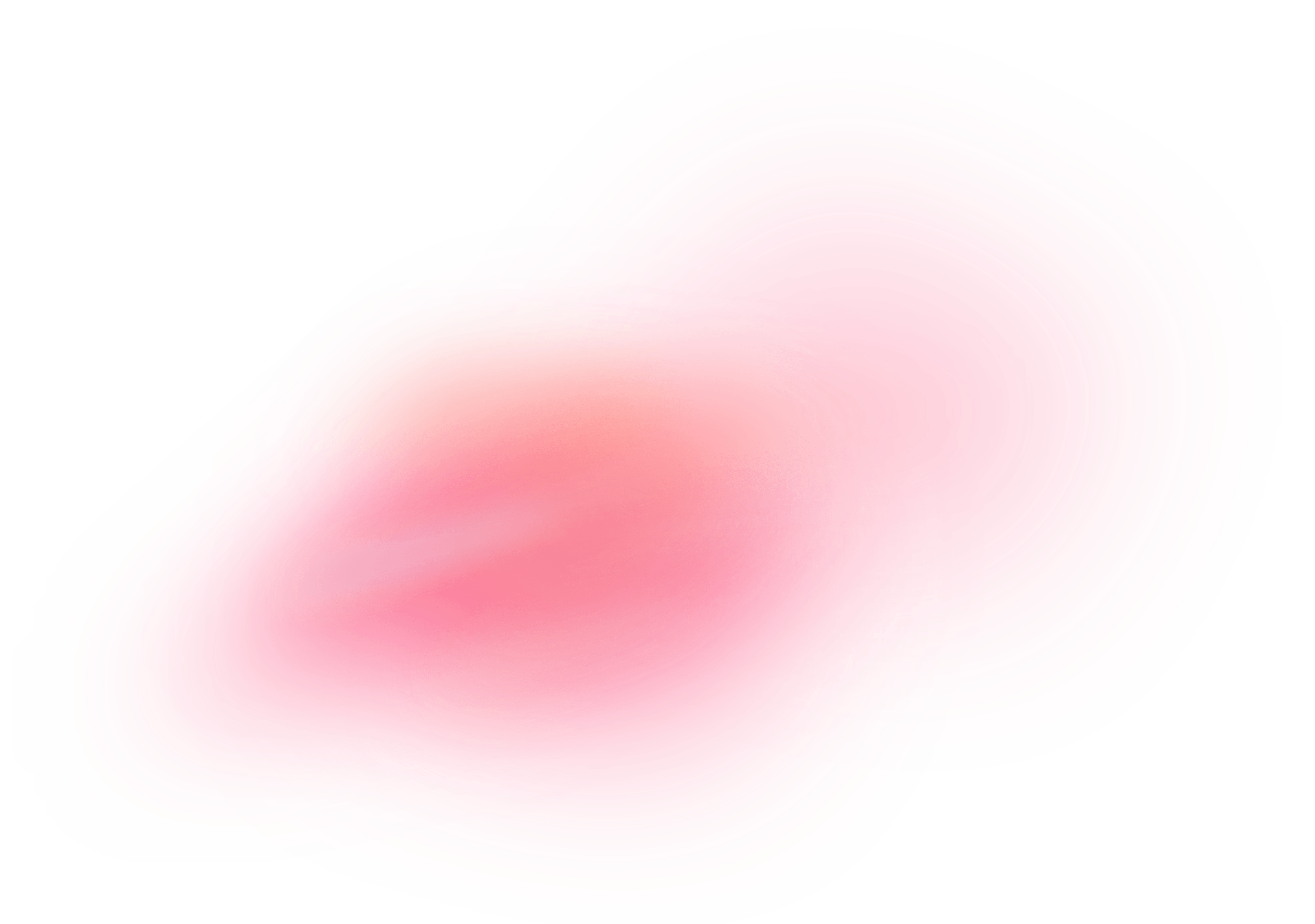