[SOLVED]Updating Object having multiple relationships create new documents instead of updating
- 0
- Databases
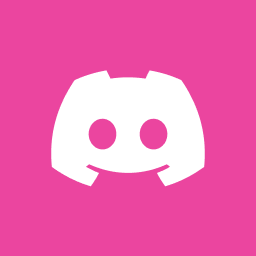
Hi guys , i use relationships to create a user details document and its contacts and address details. in flutter the class object looks like this : ```@freezed class UserDetailsModel with _$UserDetailsModel { @JsonSerializable(explicitToJson: true) const factory UserDetailsModel({ @JsonKey(includeToJson: false, ) String? id, String? firstName, String? lastName, DateTime? dateOfBirth, String? bio, required List<String> bioPicsIds, String? avatarId, ContactsModel? contacts, LocationModel? address, }) = _UserDetailsModel;
factory UserDetailsModel.fromJson(Map<String, dynamic> json) => _$UserDetailsModelFromJson(json); }``` and in appwrite it creates all documents fine . The problem is when i try to update it creates another document in the related collections instead of updating the documents already there ...how to properly do the update ?
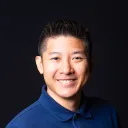
what is your code for updating the document?
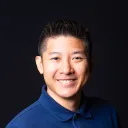
When the data is being serialized to JSON, the related objects probably don't have IDs so a new document gets created
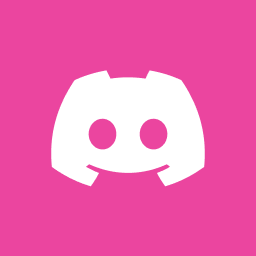
Hi @Steven sorry for the late reply here is the code for updating the document ```dart
@override FutureEitherVoid saveUserDetails(UserDetailsModel user) async { print( "in authRepository saveUserDetails method: databaseId:${Env.appwriteDatabaseId} "); try { try { //we try updating first await _db.updateDocument( databaseId: Env.appwriteDatabaseId, collectionId: Env.appwriteUserDetailsCollectionId, documentId: user.id!, data: user.toJson(), ); } on AppwriteException catch (e) { //we create a new document if (e.type == "document_not_found" && e.code == 404) { try { await _db.createDocument( databaseId: Env.appwriteDatabaseId, collectionId: Env.appwriteUserDetailsCollectionId, documentId: user.id!, data: user.toJson(), ); } on AppwriteException catch (e, stackTrace) { print(e); return Left(Failure(e.message ?? "error", e, stackTrace)); } catch (e, stackTrace) { return Left(Failure("error", e, stackTrace)); } } }
return const Right(null);
} on AppwriteException catch (e, stackTrace) {
print(e);
return Left(Failure(e.message ?? "error", e, stackTrace));
} catch (e, stackTrace) {
return Left(Failure("error", e, stackTrace));
}
}``` it is true that in the user details json i dont put the ids of the relationship.
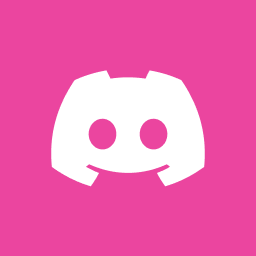
@Steven here is the json that i send to update and also create the document json
{
"firstName": "jean jacques thibaut",
"lastName": "zehi",
"dateOfBirth": "1988-08-21T00:00:00.000",
"bio": null,
"bioPicsIds": [],
"avatarId": null,
"contacts": {
"mobileNumber": null,
"officeNumber": null,
"email": "zehijean1988@gmail.com",
"url1": null,
"url2": null
},
"address": {
"countryName": "Cote D'Ivoire",
"cityName": "Abidjan",
"stateName": "Grand Abidjan",
"zipCode": null,
"fullAddress": null,
"activeDeliveryAddress": null,
"deliveryAddresses": [],
"coordinates": null,
"locationImages": []
}
}
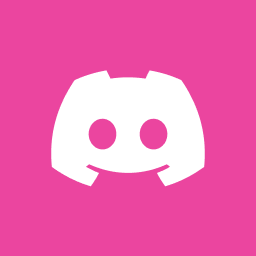
@Steven i also vaguely thought that it was because i didn't reference the ids of the relationships since i didnt see it in the doc ...based on the json above could you show me an example of updating by inserting the ids ?
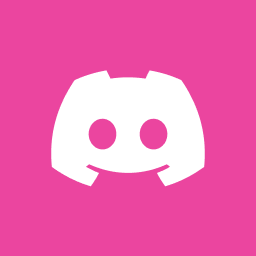
@Steven As usual Thanks a lot for pointing me into the right direction and helping me out . i was able to successfully update the documents without triggering another creation by getting the $id and putting it in the json when trying to update . json
{
"firstName": "jean jacques thibaut",
"lastName": "zehi",
"dateOfBirth": "1988-08-21T00:00:00.000",
"bio": null,
"bioPicsIds": [],
"avatarId": null,
"contacts": {
"$id": "6515b8c3cb4989c84f38",
"mobileNumber": null,
"officeNumber": null,
"email": "zehijean1988@gmail.com",
"url1": null,
"url2": null
},
"address": {
"$id": "6515b8c3cc8fd08cc76c",
"countryName": "Cameroon",
"cityName": "Banyo",
"stateName": "Adamaoua",
"zipCode": null,
"fullAddress": null,
"activeDeliveryAddress": null,
"deliveryAddresses": [],
"coordinates": null,
"locationImages": []
}
}
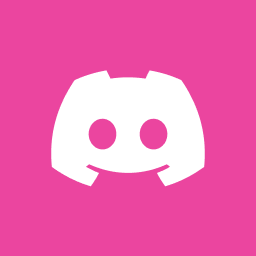
[SOLVED]Updating Object having multiple relationships create new documents instead of updating
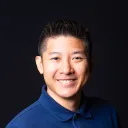
in this example, it uses an array of document IDs to only link the related documents and not update/create related documents
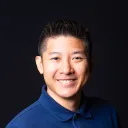
so i think you can do something like this too:
{
"firstName": "jean jacques thibaut",
"lastName": "zehi",
"dateOfBirth": "1988-08-21T00:00:00.000",
"bio": null,
"bioPicsIds": [],
"avatarId": null,
"contacts": "6515b8c3cb4989c84f38",
"address": "6515b8c3cc8fd08cc76c"
}
Recommended threads
- Appwrite database is rounding int values
Hi, i just noticed that appwrite is rounding the value 608542412536545279 to 608542412536545300 in my int array. It seems to somewhat relate to this github iss...
- Document attribute stuck on processing f...
- A feature/Fix request
Whenever I use Appwrite then to see the items of document I've to click "columns" option and select those items that I want to see then if I refresh browser/pa...
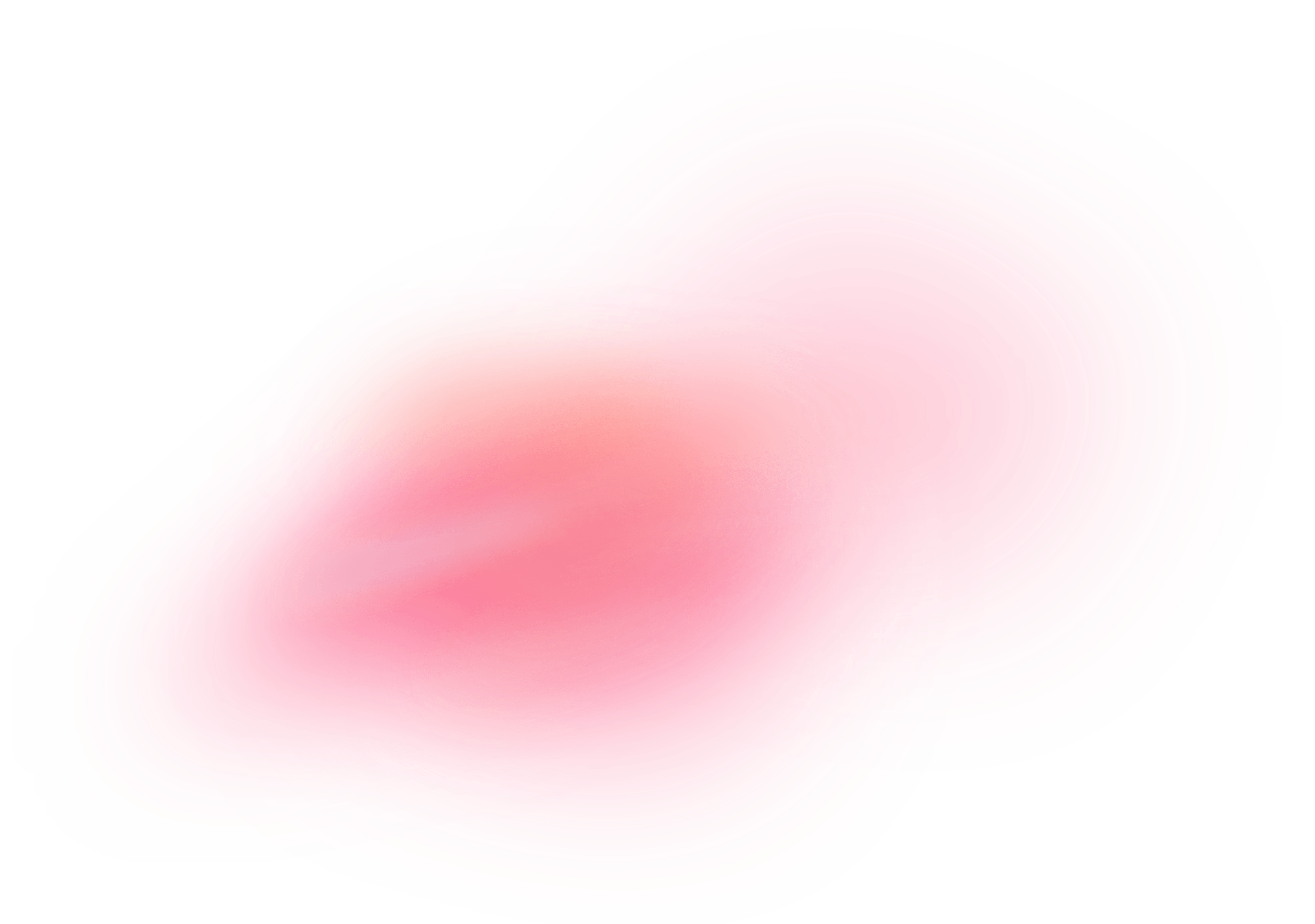