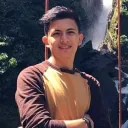
Hello, I have a problem. I have created a function in dart but when it is executed, it is not called. I have tried to run it from the appwrite console but it doesn't work either, it just throws me this error.
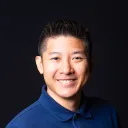
This usually happens because there's an exception thrown in your code.
Did you start with the start template created by the Appwrite CLI?
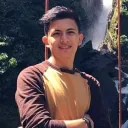
`Future<void> start(final req, final res) async { final client = Client(); print(0000); // Uncomment the services you need, delete the ones you don't // final account = Account(client); // final avatars = Avatars(client); // final database = Databases(client); // final functions = Functions(client); // final health = Health(client); // final locale = Locale(client); // final storage = Storage(client); final teams = Teams(client); // final users = Users(client); final payload = jsonDecode(req.payload == '' ? '{}' : req.payload);
if (req.variables['APPWRITE_FUNCTION_ENDPOINT'] == null || req.variables['APPWRITE_FUNCTION_API_KEY'] == null) { print( "Environment variables are not set. Function cannot use Appwrite SDK."); res.json({ 'success': true, 'message': "Environment variables are not set. Function cannot use Appwrite SDK.", }, status: 200); } else { client .setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT']) .setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID']) .setKey(req.variables['APPWRITE_FUNCTION_API_KEY']) .setSelfSigned(status: true); } final String teamId = payload['teamId']; final String userTo = payload['userTo']; /* await teams.create(teamId: teamId, name: "chat:$teamId"); */
await teams.createMembership( teamId: teamId, email: userTo, roles: [], url: req.variables['APPWRITE_FUNCTION_ENDPOINT']); res.json(success: true); } `
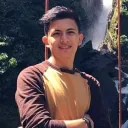
yes I edited it a bit, that's how I have it now
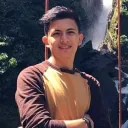
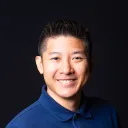
Make sure to use 3 back ticks instead of 1 for multi line code
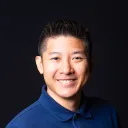
I suggest wrapping everything in a big try/catch to make sure there are no uncaught exceptions
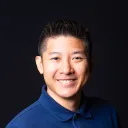
That res.json
also looks incorrect
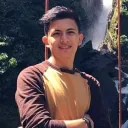
Yes, I just implemented the res.json to test if it was the error due to lack of that, but it didn't work for me either or I got another error
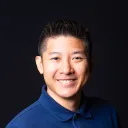
I don't know what you mean
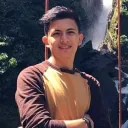
try {
final client = Client();
print(0000);
final teams = Teams(client);
final payload = jsonDecode(req.payload == '' ? '{}' : req.payload);
if (req.variables['APPWRITE_FUNCTION_ENDPOINT'] == null ||
req.variables['APPWRITE_FUNCTION_API_KEY'] == null) {
print(
"Environment variables are not set. Function cannot use Appwrite SDK.");
res.json({
'success': false,
'message':
"Environment variables are not set. Function cannot use Appwrite SDK.",
}, status: 500);
} else {
client
.setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT'])
.setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID'])
.setKey(req.variables['APPWRITE_FUNCTION_API_KEY'])
.setSelfSigned(status: true);
}
final String teamId = payload['teamId'];
final String userTo = payload['userTo'];
await teams.createMembership(
teamId: teamId,
email: userTo,
roles: [],
url: req.variables['APPWRITE_FUNCTION_ENDPOINT']);
res.json({
'success': true,
}, status: 200);
} catch (e) {
print(e);
}
}
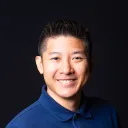
You must call res.json() or res.send() exactly once before your function finishes.
Also, make sure to pass a string to print rather than an object
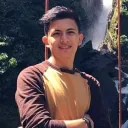
ok, but it doesn't print anything. It is as if the function does not start
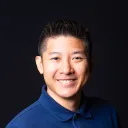
It's because your function never sent back a response so it timed out (and the output of print is in the response)
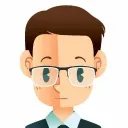
You try can add this to get your error.
} catch (e) {
res.json({"e":e});
}
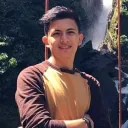
Hello again, I create a new function and paste the same code and for some reason it works
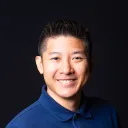
Did you create the variables?
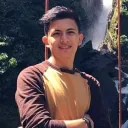
Now I'm going to try this but for the moment it already returns a successful response
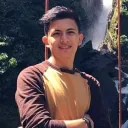
final client = Client();
final teams = Teams(client);
if (req.variables['APPWRITE_FUNCTION_ENDPOINT'] == null ||
req.variables['APPWRITE_FUNCTION_API_KEY'] == null) {
res.json({
'error': "Environment variables are not set. Function cannot use Appwrite SDK.",
});
} else {
try {
client
.setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT'])
.setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID'])
.setKey(req.variables['APPWRITE_FUNCTION_API_KEY'])
.setSelfSigned(status: true);
final payload = jsonDecode(req.payload == '' ? '{}' : req.payload);
final String teamId = payload['teamId'];
final String userTo = payload['userTo'];
await teams.createMembership(
teamId: teamId,
email: userTo,
roles: [],
url: req.variables['APPWRITE_FUNCTION_ENDPOINT']);
res.json({
'success': true,
});
} catch (e) {
res.json({
'Error': e.toString(),
});
}
}
}
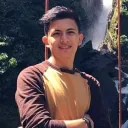
Thank you for all, problem solved, I did not understand how to send an response. Creating a function I managed to understand. I attach the code with which I get it to work for me
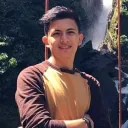
[SOLVED] Function is not executed
Recommended threads
- Attributes Confusion
```import 'package:appwrite/models.dart'; class OrdersModel { String id, email, name, phone, status, user_id, address; int discount, total, created_at; L...
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
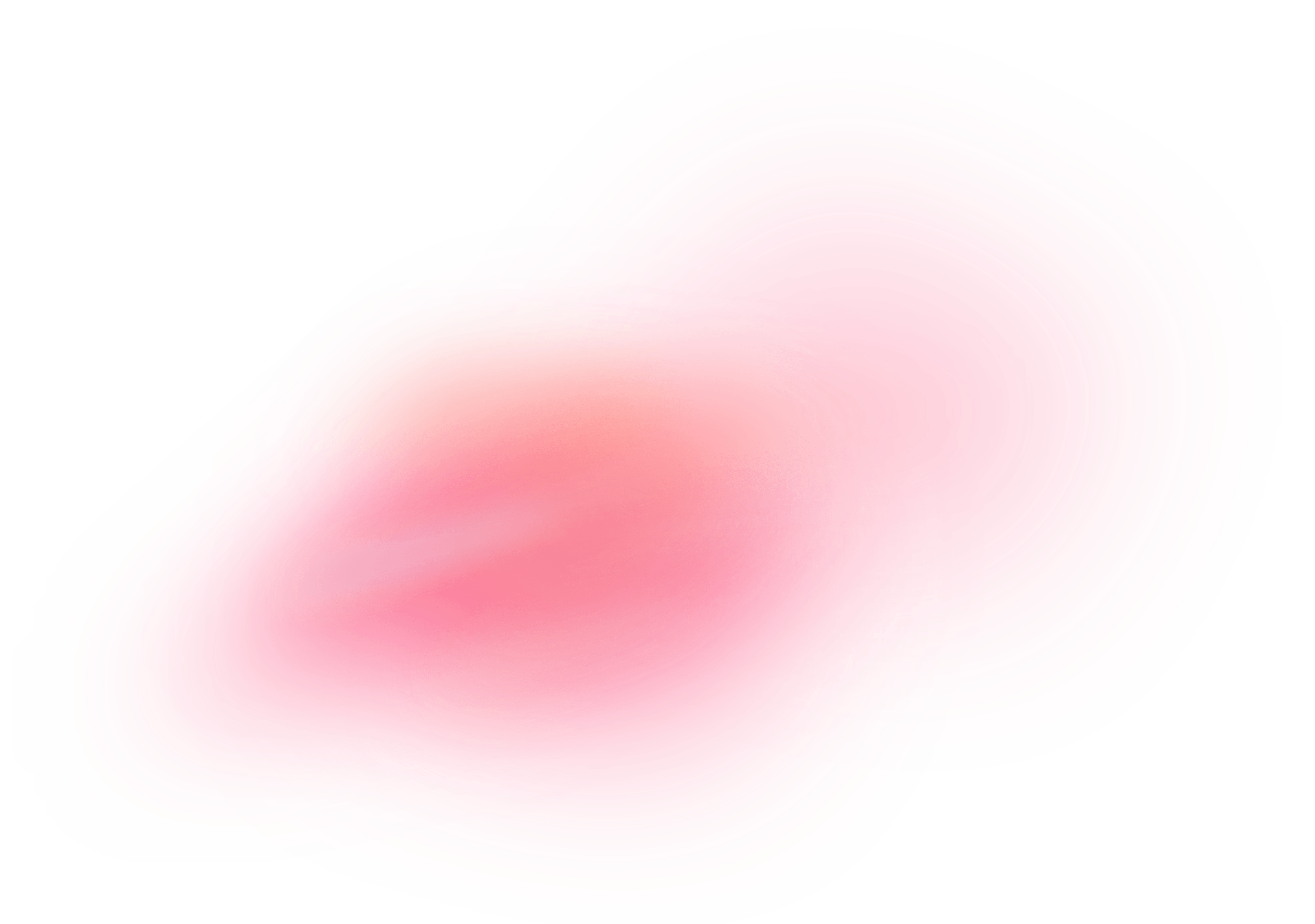