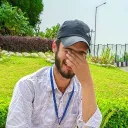
TypeScript
class OrdersModel {
String id, email, name, phone, status, user_id, address;
int discount, total, created_at;
List<OrderProductModel> products;
OrdersModel({
required this.id,
required this.created_at,
required this.email,
required this.name,
required this.phone,
required this.address,
required this.status,
required this.user_id,
required this.discount,
required this.total,
required this.products,
});
// Convert Appwrite's Document to OrdersModel
factory OrdersModel.fromJson(Document doc, [Strings]) {
final data = doc.data;
return OrdersModel(
id: doc.$id,
created_at: data['created_at'] ?? 0,
email: data['email'] ?? '',
name: data['name'] ?? '',
phone: data['phone'] ?? '',
status: data['status'] ?? '',
address: data['address'] ?? '',
user_id: data['user_id'] ?? '',
discount: data['discount'] ?? 0,
total: data['total'] ?? 0,
products: List<OrderProductModel>.from(data['products']?.map((e) => OrderProductModel.fromJson(e)) ?? []),
);
}
// Convert a list of Documents to a list of OrdersModels
static List<OrdersModel> fromJsonList(List<Document> docs) {
return docs.map((doc) => OrdersModel.fromJson(doc)).toList();
}
}
class OrderProductModel {
String id, name, image;
int quantity, single_price, total_price;
OrderProductModel({
required this.id,
required this.name,
required this.image,
required this.quantity,
required this.single_price,
required this.total_price,
});
factory OrderProductModel.fromJson(Map<String, dynamic> json) {
return OrderProductModel(
id: json['id'] ?? '',
name: json['name'] ?? '',
image: json['image'] ?? '',
quantity: json['quantity'] ?? 0,
single_price: json['single_price'] ?? 0,
total_price: json['total_price'] ?? 0,
);
}
}```
TL;DR
Developers are confused about creating a product attribute. The code provided showcases how to convert a document to OrdersModel and a list of documents to a list of OrdersModels, including OrderProductModel. 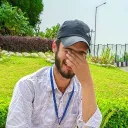
How can I create thus Product Attribute can any one tell me please!
Recommended threads
- My account is blocked so please check an...
My account is blocked so please unblock my account because all the apps are closed due to which it is causing a lot of problems
- Applying free credits on Github Student ...
So this post is kind of related to my old post where i was charged 15usd by mistake. This happens when you are trying to apply free credits you got from somewh...
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
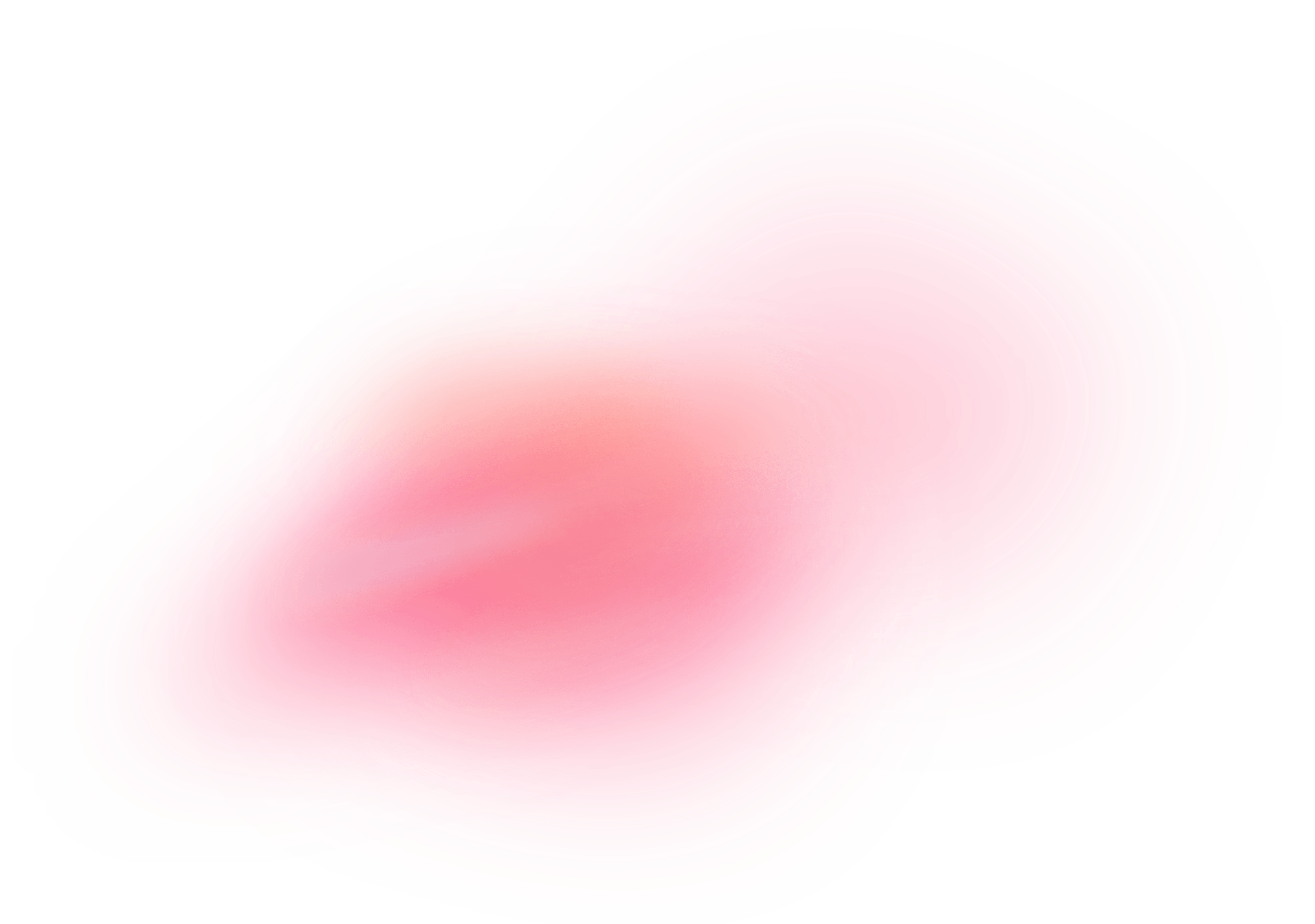