Function: An internal curl error has occurred within the executor! Error Msg: Operation timed out
- 1
- Functions
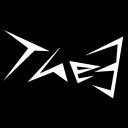
I have 2 Appwrite Functions written in TypeScript with the node-sdk which work most of the time fine, but in some cases I get the following error:
[Error] Type: Exception
[Error] Message: An internal curl error has occurred within the executor! Error Msg: Operation timed out
[Error] File: /usr/src/code/app/executor.php
[Error] Line: 544`
I found two GitHub issues, with similar problems:
- https://github.com/appwrite/appwrite/issues/2940
- https://github.com/appwrite/appwrite/issues/4626 Unfortunately there is no specific solution.
The TypeScript file is converted into JavaScript before deployment (tsconfig.json
can be provided, if necessary)
Code is posted on the next post.
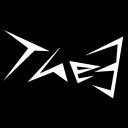
import { Client, Account, Models } from "node-appwrite";
import { MongoClient } from "mongodb";
module.exports = async function (req: any, res: any) {
const client = new Client();
const user = req.variables["MONGODB_USER"];
const password = req.variables["MONGODB_PASSWORD"];
const hostname = req.variables["MONGODB_HOSTNAME"];
// Create a new MongoClient
const mongoUri = `mongodb://${user}:${password}@${hostname}:27017/?authMechanism=DEFAULT`;
const mongoDbClient = new MongoClient(mongoUri);
let userId: string;
if (
!req.variables["APPWRITE_FUNCTION_ENDPOINT"] ||
!req.variables["APPWRITE_FUNCTION_API_KEY"]
) {
console.warn(
"Environment variables are not set. Function cannot use Appwrite SDK."
);
} else {
client
.setEndpoint(req.variables["APPWRITE_FUNCTION_ENDPOINT"])
.setProject(req.variables["APPWRITE_FUNCTION_PROJECT_ID"])
.setJWT(req.variables["APPWRITE_FUNCTION_JWT"])
.setSelfSigned(true);
}
const account = new Account(client);
let accountData: Models.Account<Models.Preferences>;
try {
accountData = await account.get();
} catch (error: any) {
console.log(error); // Failure
}
userId = accountData!.$id;
async function run() {
try {
// Connect the client to the server (optional starting in v4.7)
await mongoDbClient.connect();
let reqPayloadObj = JSON.parse(req.payload ?? "{}"); //Make string to object
console.log(reqPayloadObj);
const pipeline = //Pipeline
const coll = mongoDbClient.db("aticAR").collection("container_items");
let myContainerItems = await coll.aggregate(pipeline).toArray();
//Create Response
res.json(JSON.stringify(myContainerItems));
} catch(error: any){
res.json({'error': error.toString()});
console.log(error);
}
finally {
// Ensures that the client will close when you finish/error
await mongoDbClient.close();
}
}
run();
};
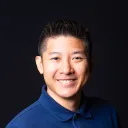
This usually happens if your function throws an error and/or doesn't call res.json() or res.send() once
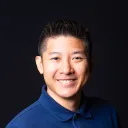
Btw I don't think you need that nested run function π§
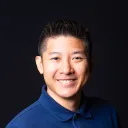
Also, it might be better to coerce the error to a string like
console.log(error.toString());
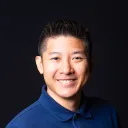
How long does it take before the timeout occurs?
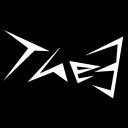
The problem is, that it is a black box for me, I can't see, what exactly is failing. There is no Error in the container logs, appwrite logs, or appwrite execution logs.
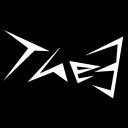
15 seconds
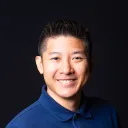
Maybe you're actually hitting the timeout. You can try increasing the timeout in your function settings
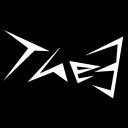
The problem is, that most of the time it succeeds with a response time of just few milliseconds.
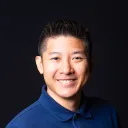
Ya if the timeout occurs, you won't get any data back.
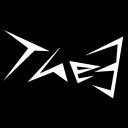
Hmm, then debugging this issue is difficult/impossible?
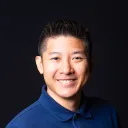
That's why I suggest increasing the timeout
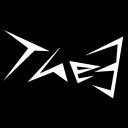
Alright, I will do that.
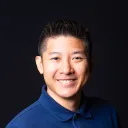
Also, the other suggestion about the run... either remove it or await it
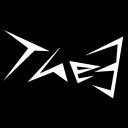
I added await
and increased timeout to 180 seconds, the result is the same. Strangely the duration is in ms even though it takes several minutes to execute/respond (maybe a bug?).
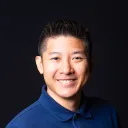
No change in the logs?
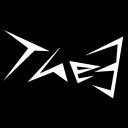
Yep, no change. π€¨
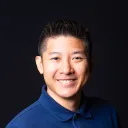
I wonder if there's an error thrown by the mongodb.close() π§
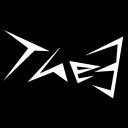
Is finally
run after or before the respond is being send by the function?
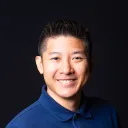
Before
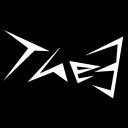
Should I wrap it in a try-catch-block? Or is a potential exception caught by the upper catch-block?
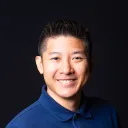
Try catch it just in case
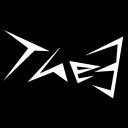
This seems to be a strange behaviour of MongoDB. I got the following error when the function fails:
Command "top" returned error "connection 1 to <server-ip> closed"
Command "currentOp" returned error "Invalid collection name specified 'admin.$cmd.sys.inprog"
Command "serverStatus" returned error "connection 3 to <server-ip> closed"
https://stackoverflow.com/questions/59942238/mongoerror-topology-is-closed-please-connect-despite-established-database-conn
So the error is most probably caused by the finally
block and await mongoDbClient.close();
.
Recommended threads
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
- Function in Node.JS to monitor events ar...
Hello everyone. I'm creating my first Node.JS function, but I don't have much experience with node and javascript. I'm trying to create a function, that monito...
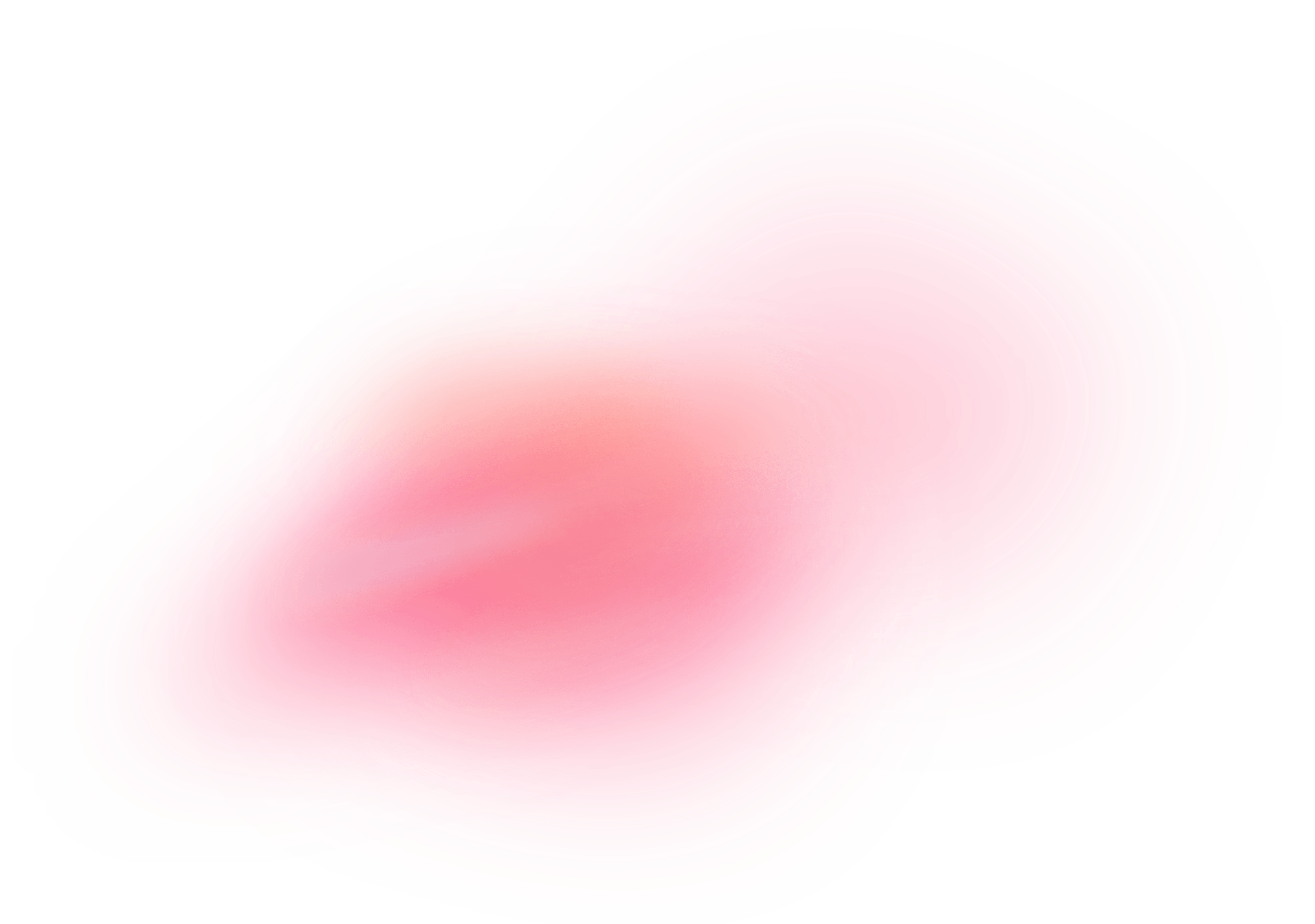