
So I am using Nextjs v15 as a full stack and im not sure what the proper flow is
I am trying to compare with supabase where i make a server client and a client client
the 1-1 equivalent i assume is node sdk (server) and web sdk (client)
i use server actions to signup / login / create session with the node sdk client
this works, i can see my user in the appwrite dashboard AND can retrieve the session
but when i am trying to get the client in my react context that I created I am presented with the following errors
GET https://cloud.appwrite.io/v1/account 401 (Unauthorized)
AppwriteException: User (role: guests) missing scope (account)
am i doing something wrong or am i unable to retrieve a session created by the server sdk with the web sdk?

// client.ts
'use client'
import { Client, Account } from 'appwrite'
const NEXT_PUBLIC_APPWRITE_ENDPOINT = String(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
const NEXT_PUBLIC_APPWRITE_PROJECT = String(process.env.NEXT_PUBLIC_APPWRITE_PROJECT)
const client = new Client()
.setEndpoint(NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(NEXT_PUBLIC_APPWRITE_PROJECT)
const account = new Account(client)
export { account }
// user-provider.tsx
useEffect(() => {
;(async function run() {
try {
const loggedInUser = await account.get()
setUser(loggedInUser)
} catch (err) {
setUser(null)
}
})()
}, [])

// server.ts
'use server'
import { Client, Account } from 'node-appwrite'
import { cookies } from 'next/headers'
const NEXT_PUBLIC_APPWRITE_ENDPOINT = String(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
const NEXT_PUBLIC_APPWRITE_PROJECT = String(process.env.NEXT_PUBLIC_APPWRITE_PROJECT)
const NEXT_APPWRITE_KEY = String(process.env.NEXT_APPWRITE_KEY)
export async function createSessionClient() {
const client = new Client()
.setEndpoint(NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(NEXT_PUBLIC_APPWRITE_PROJECT)
const session = (await cookies()).get('my-custom-session')
if (!session || !session.value) {
throw new Error('No session')
}
client.setSession(session.value)
return {
get account() {
return new Account(client)
},
}
}
export async function createAdminClient() {
const client = new Client()
.setEndpoint(NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(NEXT_PUBLIC_APPWRITE_PROJECT)
.setKey(NEXT_APPWRITE_KEY)
return {
get account() {
return new Account(client)
},
}
}
/signin.action.ts
'use server'
import { createAdminClient } from '@/utils/appwrite/server'
import { cookies } from 'next/headers'
import { redirect } from 'next/navigation'
export async function signinAction(formData: FormData) {
const values = Object.fromEntries(formData.entries())
const email = `${values.email}`
const password = `${values.password}`
const { account } = await createAdminClient()
const session = await account.createEmailPasswordSession(email, password)
;(await cookies()).set('my-custom-session', session.secret, {
path: '/',
httpOnly: true,
sameSite: 'strict',
secure: true,
})
redirect('/')
}
Recommended threads
- Error 400 general_bad_request
I am getting `general_bad_request` when trying to login Github I have follow the `Appwrite's YouTube video to setup Github OAuth` ```javascript const loginWith...
- Why currency is always an empty string? ...
```tsx const locale = new Locale(client); const userLocale = await locale.get(); ``` But always got something like this ```json {"ip":"xxx.xx.xxx.xxx","country...
- OAuth Flow Interruption Due to Redirecti...
I kindly request your assistance in resolving this issue. Specifically, I would appreciate guidance on the following: How to properly set up a redirection URI ...
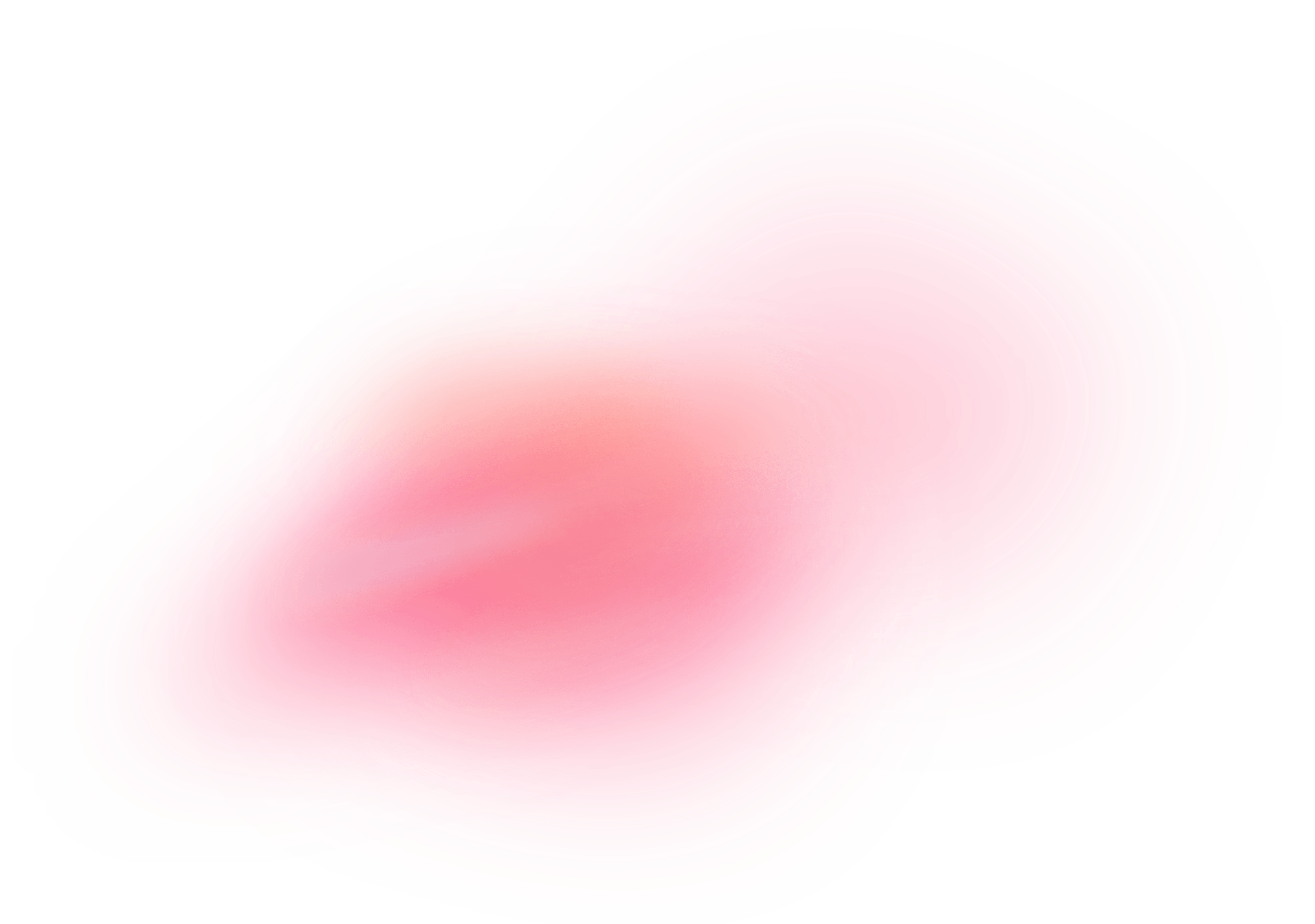