Signup failed: Invalid `userId` param: Parameter must contain at most 36 chars. Valid chars are a-z,
- 0
- Web
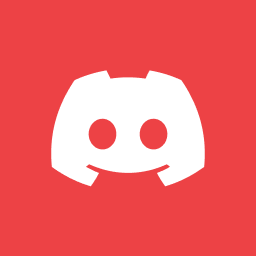
Signup failed: Invalid userId
param: Parameter must contain at most 36 chars. Valid chars are a-z, A-Z, 0-9, period, hyphen, and underscore. Can't start with a special char
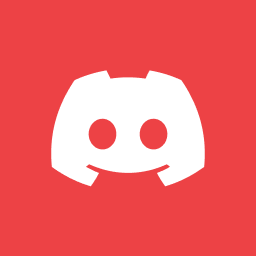
"use client"; // This directive is important
import { useState } from "react"; import { account } from "../../lib/appwrite"; import { useRouter } from "next/navigation";
const Signup = () => { const [email, setEmail] = useState(""); const [password, setPassword] = useState(""); const [username, setUsername] = useState(""); const router = useRouter();
const handleSignup = async (e: React.FormEvent) => { e.preventDefault(); try { // Create the user account
const randomString = Math.random().toString(36).substring(2, 15);
// Combine username and random string to create user ID
const userId = `${username
.replace(/[^a-zA-Z0-9.-_]/g, "")
.toLowerCase()}_${randomString}`;
await account.create(email, password, userId);
alert("Signup successful!");
router.push("/"); // Redirect to home or another page
} catch (error: unknown) {
console.error("Signup error:", error);
const errorMessage = (error as Error).message || "Something went wrong.";
alert("Signup failed: " + errorMessage);
}
};
return ( <div> <h1>Sign Up</h1> <form onSubmit={handleSignup}> <input type="text" placeholder="Username" value={username} onChange={(e) => setUsername(e.target.value)} required /> <input type="email" placeholder="Email" value={email} onChange={(e) => setEmail(e.target.value)} required /> <input type="password" placeholder="Password" value={password} onChange={(e) => setPassword(e.target.value)} required /> <button type="submit">Sign Up</button> </form> </div> ); };
export default Signup;
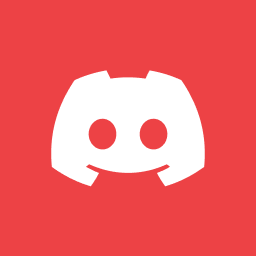
"use client"; import { useState } from 'react'; import { account } from '../../lib/appwrite'; // Ensure this import is correct import { useRouter } from 'next/navigation';
const Signin = () => { const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const router = useRouter();
const handleSignin = async (e: React.FormEvent) => { e.preventDefault(); try { // Create a session with email and password using Appwrite await account.createEmailPasswordSession(email, password); alert('Sign in successful!'); router.push('/'); // Redirect to home or another page } catch (error) { if (error instanceof Error) { const errorMessage = error.message || 'Something went wrong.'; console.error('Sign in error:', errorMessage); alert('Sign in failed: ' + errorMessage); } else { console.error('Unexpected error:', error); alert('Sign in failed due to an unexpected error.'); } } };
return ( <div> <h1>Sign In</h1> <form onSubmit={handleSignin}> <input type="email" placeholder="Email" value={email} onChange={(e) => setEmail(e.target.value)} required /> <input type="password" placeholder="Password" value={password} onChange={(e) => setPassword(e.target.value)} required /> <button type="submit">Sign In</button> </form> </div> ); };
export default Signin;
Recommended threads
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
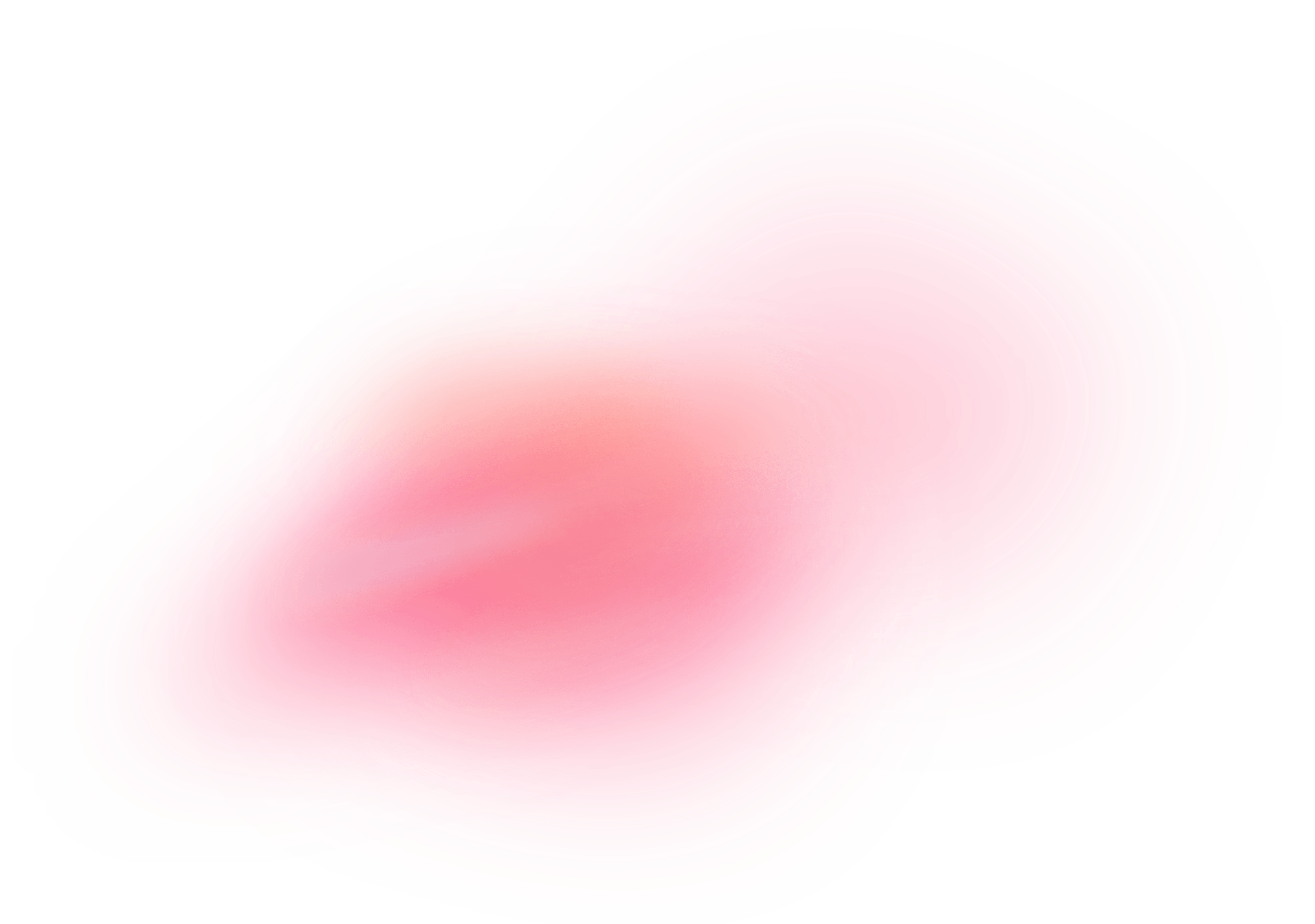