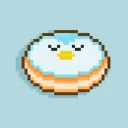
TypeScript
export async function updateAvatar({ file, userId }) {
try {
if (!file || !userId) {
throw new Error("File and userId are required");
}
// Upload the avatar file
const [avatarUrl] = await Promise.all([uploadFileUsingAppwrite(file.uri)]);
// Update the user's profile with the new avatar URL
const updatedAvatar = await databases.updateDocument(appwriteConfig.databaseId, appwriteConfig.userCollectionId, userId, {
avatar: avatarUrl,
});
return updatedAvatar;
} catch (error) {
console.error("Error updating avatar:", error.message || error);
throw new Error(error.message || "Failed to update avatar");
}
}
TL;DR
Error occurred while attempting to update avatar using ImagePicker instead of DocumentPicker in React Native. The network request failed when fetching file URI. Switching back to DocumentPicker allows successful uploads.
A possible solution can be to modify the code to handle imagepicker file uri with the same method as documentpicker. 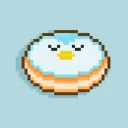
TypeScript
export async function uploadFileUsingAppwrite(fileUri) {
if (!fileUri) {
throw new Error("File URI is invalid or null");
}
try {
const file = await fetch(fileUri);
const blob = await file.blob();
const fileName = fileUri.split("/").pop();
const mimeType = blob.type || "application/octet-stream";
const response = await storage.createFile(
appwriteConfig.databaseId, // Bucket ID
fileName, // File ID or unique name
blob, // File blob
mimeType // MIME type
);
console.log("RESPONSE: ", response)
return response;
} catch (error) {
console.error("Error uploading file:", error.message || error);
throw new Error(error.message || "Failed to upload file");
}
}
This happened when we try to use ImagePicker instead of DocumentPicker in React Native. Uploading works fine with DocumentPicker.
Back then, the upload code was only this simple, but doesn't work with imagepicker file uri fetching
TypeScript
// Upload File
export async function uploadFile(file, type) {
if (!file) return;
const { mimeType, ...rest } = file;
const asset = { type: mimeType, ...rest };
try {
const uploadedFile = await storage.createFile(
appwriteConfig.storageId,
ID.unique(),
asset
);
const fileUrl = await getFilePreview(uploadedFile.$id, type);
return fileUrl;
} catch (error) {
throw new Error(error);
}
}
Recommended threads
- Is there a way to retrieve all documents...
I am trying to do a query to return all items in a given collection, but can only seem to find a way to get a single document?
- 404 Collection not found Error
I created a database on the free tier and when I click on the DB to create new collections, I get the error 404 Collection not found. Attaching the screenshots ...
- How to verify a JWT token (generated on ...
I am new to Appwrite. I have set up Appwrite authentication in React Native and am successfully obtaining a JWT. I have AWS serverless functions. How can I veri...
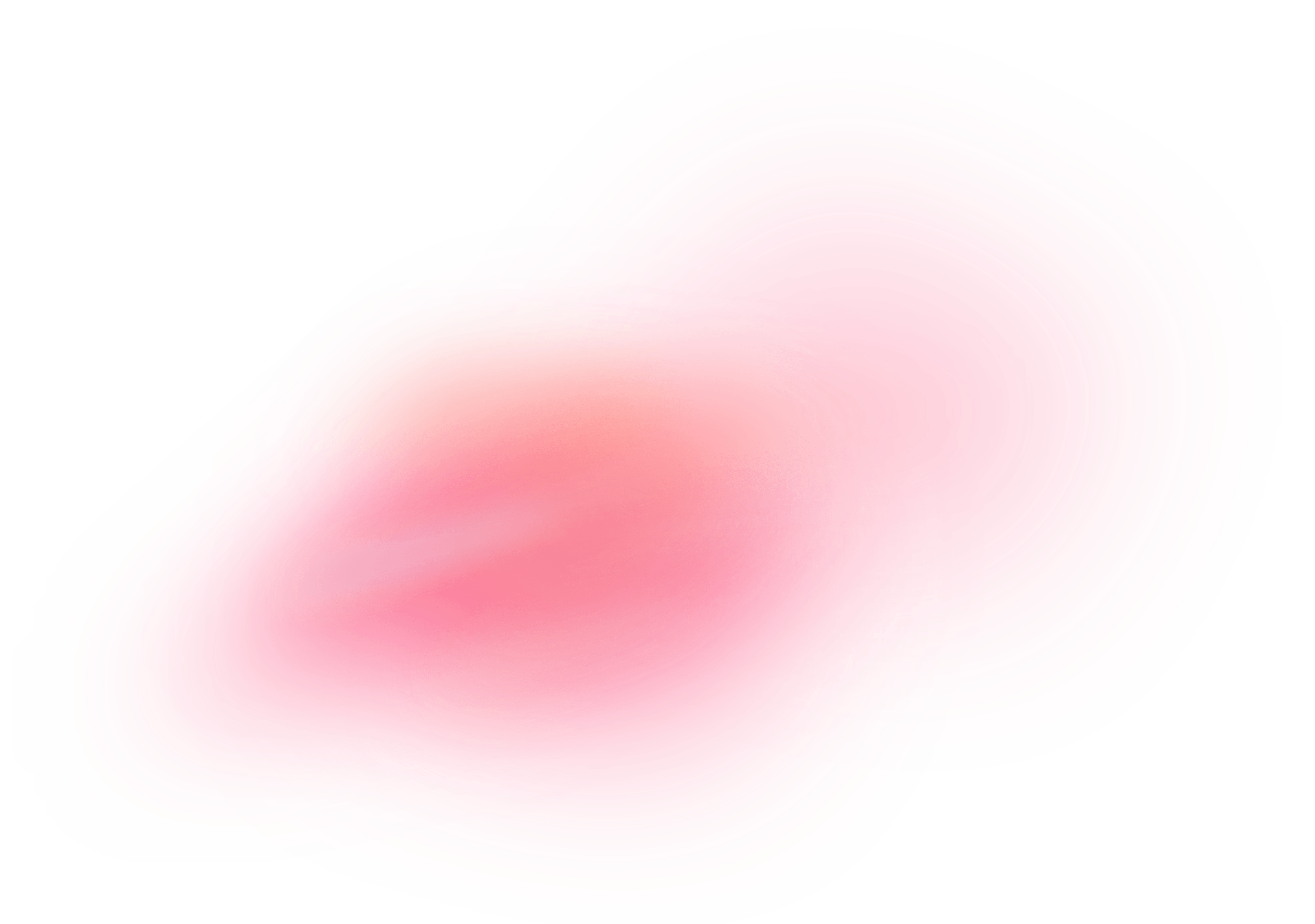