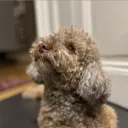
@Red I’m no expert but it seems to be an issue with your code
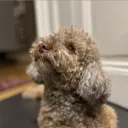
Is it the same code that you tested on cloud?
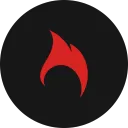
Same code on cloud just imported to local
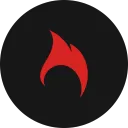
This is on cloud
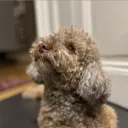
Can you share your code please
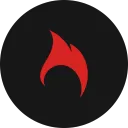
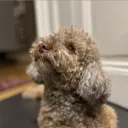
Please copy paste it here
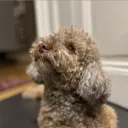
I cant do anything with a screenshot
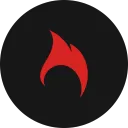
` import 'dart:async'; import 'dart:convert'; import 'dart:io'; import 'package:backoffice_shared/backoffice_shared.dart'; import 'package:dart_appwrite/dart_appwrite.dart'; import 'package:starter_template/utils/update_compensations.dart';
Future<dynamic> main(final context) async { final List payslipsData = json.decode(context.req.body)["payslips"]; final List employeeData = json.decode(context.req.body)["employees"]; final List compensationData = json.decode(context.req.body)["compensations"];
final List<PayslipModel> payslips = payslipsData.map((payslip) { return PayslipModel.fromJson(payslip); }).toList();
final List<EmployeeModel> employees = employeeData.map((employee) { return EmployeeModel.fromJson(employee); }).toList();
final List<CompensationModel> compensations = compensationData.map((employee) { return CompensationModel.fromJson(employee); }).toList();
final client = Client() .setEndpoint('https://cloud.appwrite.io/v1') .setProject(Platform.environment['APPWRITE_FUNCTION_PROJECT_ID']) .setKey(Platform.environment['APPWRITE_API_KEY']);
final Databases databases = Databases(client); final Messaging messaging = Messaging(client);
try { await updateCompensations( context: context, databases: databases, messaging: messaging, payslips: payslips, employees: employees, compensations: compensations, ); } catch (e) { context.error(e); }
return context.res.empty(); }
`
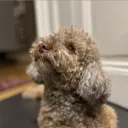
Future<dynamic> main(final context) async {
final Map<String, dynamic> body = json.decode(context.req.body);
final List payslipsData = body["payslips"] ?? [];
final List employeeData = body["employees"] ?? [];
final List compensationData = body["compensations"] ?? [];
final List<PayslipModel> payslips = payslipsData.map((payslip) {
return PayslipModel.fromJson(payslip);
}).where((payslip) => payslip != null).toList();
final List<EmployeeModel> employees = employeeData.map((employee) {
return EmployeeModel.fromJson(employee);
}).where((employee) => employee != null).toList();
final List<CompensationModel> compensations = compensationData.map((employee) {
return CompensationModel.fromJson(employee);
}).where((compensation) => compensation != null).toList();
final projectId = Platform.environment['APPWRITE_FUNCTION_PROJECT_ID'];
final apiKey = Platform.environment['APPWRITE_API_KEY'];
if (projectId == null || apiKey == null) {
throw Exception('Environment variables not set.');
}
final client = Client()
.setEndpoint('https://cloud.appwrite.io/v1')
.setProject(projectId)
.setKey(apiKey);
final Databases databases = Databases(client);
final Messaging messaging = Messaging(client);
try {
await updateCompensations(
context: context,
databases: databases,
messaging: messaging,
payslips: payslips,
employees: employees,
compensations: compensations,
);
} catch (e) {
context.error(e);
}
return context.res.empty();
}
Try this with added checks in it.
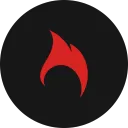
Okay let me try

Are you really sure your code is the same locally?

The error you showed us indicates that you made a typo

the method "erorr" does not exists but "error"

but in the code you showed us it seems to be correct
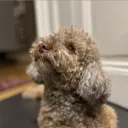
if they copy paste the code I gave them
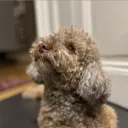
it should work

I'm really confused. He does nowhere uses the method "erorr" in his code

I'm not sure if he sent the correct code
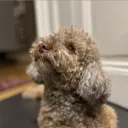
Im not sure either
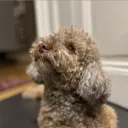
@Red is it working?
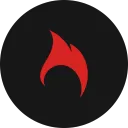
Sorry I was moving now trying to test
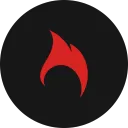
I just imported from cloud no changes whatsoever

show us the code for updateCompensation

You are passing context there and that code snippet is missing here
Recommended threads
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
- Send Email Verification With REST
I am using REST to create a user on the server side after receiving form data from the client. After the account is successfully created i wanted to send the v...
- Use different email hosts for different ...
Hello, I have 2 projects and i want to be able to set up email templates in the projects. Both projects will have different email host configurations. I see ...
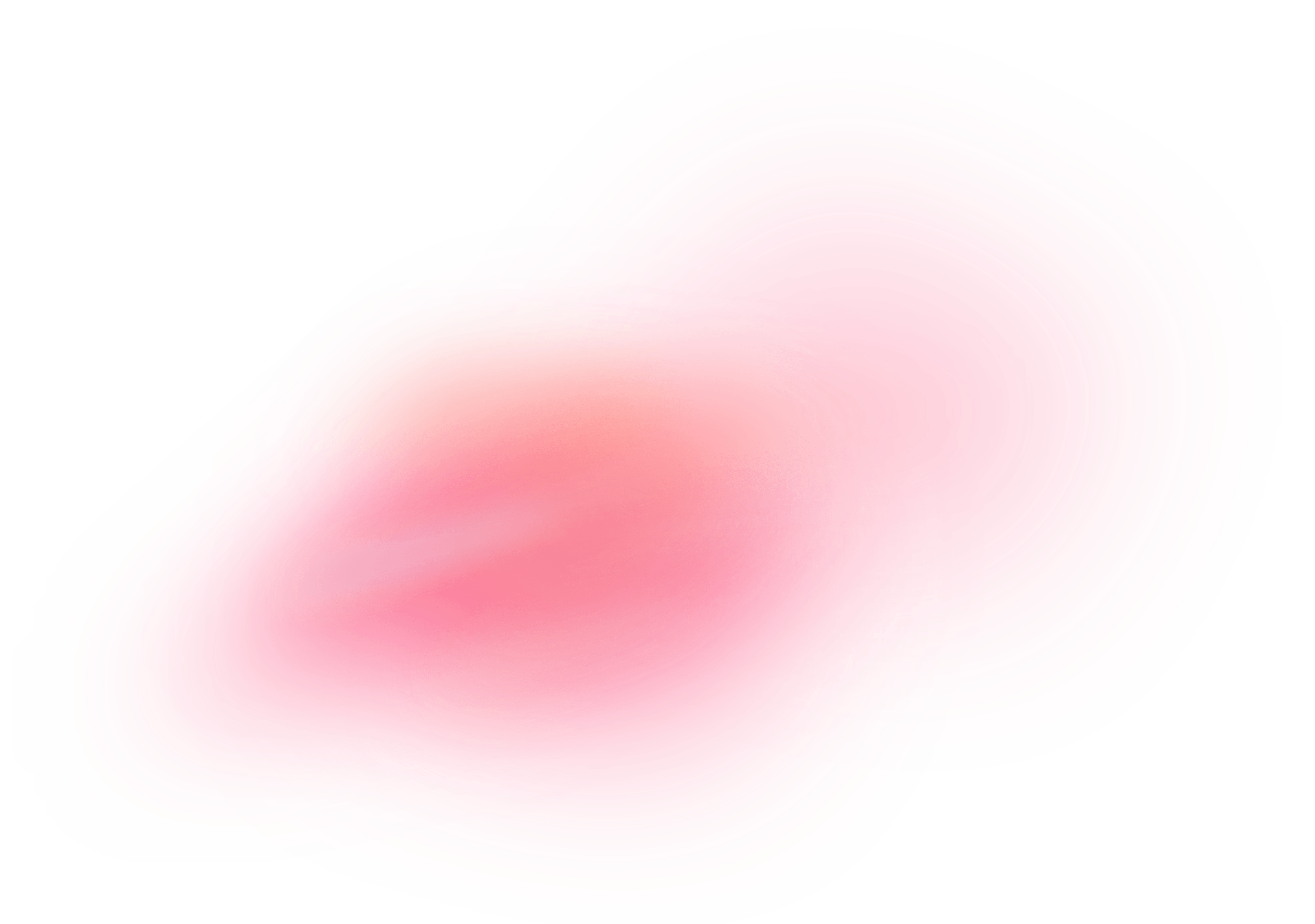