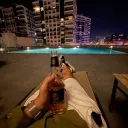
Hello, create and delete functions works well with custom domain appwrite database with the code above. but updating doesnt work.
TypeScript
import client from "@/app/appwrite";
import { Databases, ID, Query } from "appwrite";
class AddressService {
static instance;
static databases = new Databases(client);
static databaseId = "";
static collectionId = "";
static getInstance() {
if (!AddressService.instance) {
AddressService.instance = new AddressService();
}
return AddressService.instance;
}
createAddress = async (userId, addressName, fullAddress) => {
try {
return await AddressService.databases.createDocument(
AddressService.databaseId,
AddressService.collectionId,
ID.unique(),
{
user_id: userId,
address_name: addressName,
full_address: fullAddress,
}
);
} catch (error) {
throw error;
}
};
updateAddress = async (documentId, addressName, fullAddress) => {
try {
const result = await AddressService.databases.updateDocument(
AddressService.databaseId,
AddressService.collectionId,
documentId,
{
address_name: addressName,
full_address: fullAddress,
}
);
console.log("Document updated successfully:", result);
return result;
} catch (error) {
console.error("Error updating address:", error);
throw error;
}
};
deleteAddress = async (documentId) => {
try {
await AddressService.databases.deleteDocument(
AddressService.databaseId,
AddressService.collectionId,
documentId
);
console.log("Document deleted successfully");
} catch (error) {
console.error("Error deleting document:", error);
throw error;
}
};
export default AddressService;
TL;DR
The developer found a temporary solution for updating a document by creating an API route instead of calling the service directly. They shared code snippets demonstrating the creation, update, and delete functions, with the issue specifically with updating not working as expected. The solution involves reviewing and potentially revising the updateAddress function in the AddressService class. 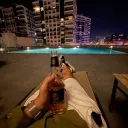
Temporary solved it with ;
TypeScript
updateAddress = async (documentId, addressName, fullAddress) => {
try {
// Mevcut belgeyi al
const existingDocument = await AddressService.databases.getDocument(
AddressService.databaseId,
AddressService.collectionId,
documentId
);
// Mevcut belgeyi sil
await AddressService.databases.deleteDocument(
AddressService.databaseId,
AddressService.collectionId,
documentId
);
// Yeni belge oluştur
const result = await AddressService.databases.createDocument(
AddressService.databaseId,
AddressService.collectionId,
documentId, // Aynı ID ile yeni belge oluşturuluyor
{
user_id: existingDocument.user_id, // Mevcut belgeden alınan veriler
address_name: addressName,
full_address: fullAddress,
}
);
console.log("Document updated successfully:", result);
return result; // Güncellenmiş belgeyi döndür
} catch (error) {
console.error("Error updating address:", error);
throw error; // Hata oluşursa fırlat
}
};
``` but really need it to be fixed.
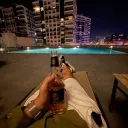
Solved it with creating API route instead of calling service directly on client client component.
Recommended threads
- Issue with relations on database
I'm creating a food ordering website for businesses. I'm having issues with the relations on my database I attached the attributes for the collections I'm hav...
- The current user is not authorized to pe...
I want to create a document associated with user after log in with OAuth. The user were logged in, but Appwrite said user is unauthorized. User is logged in wi...
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
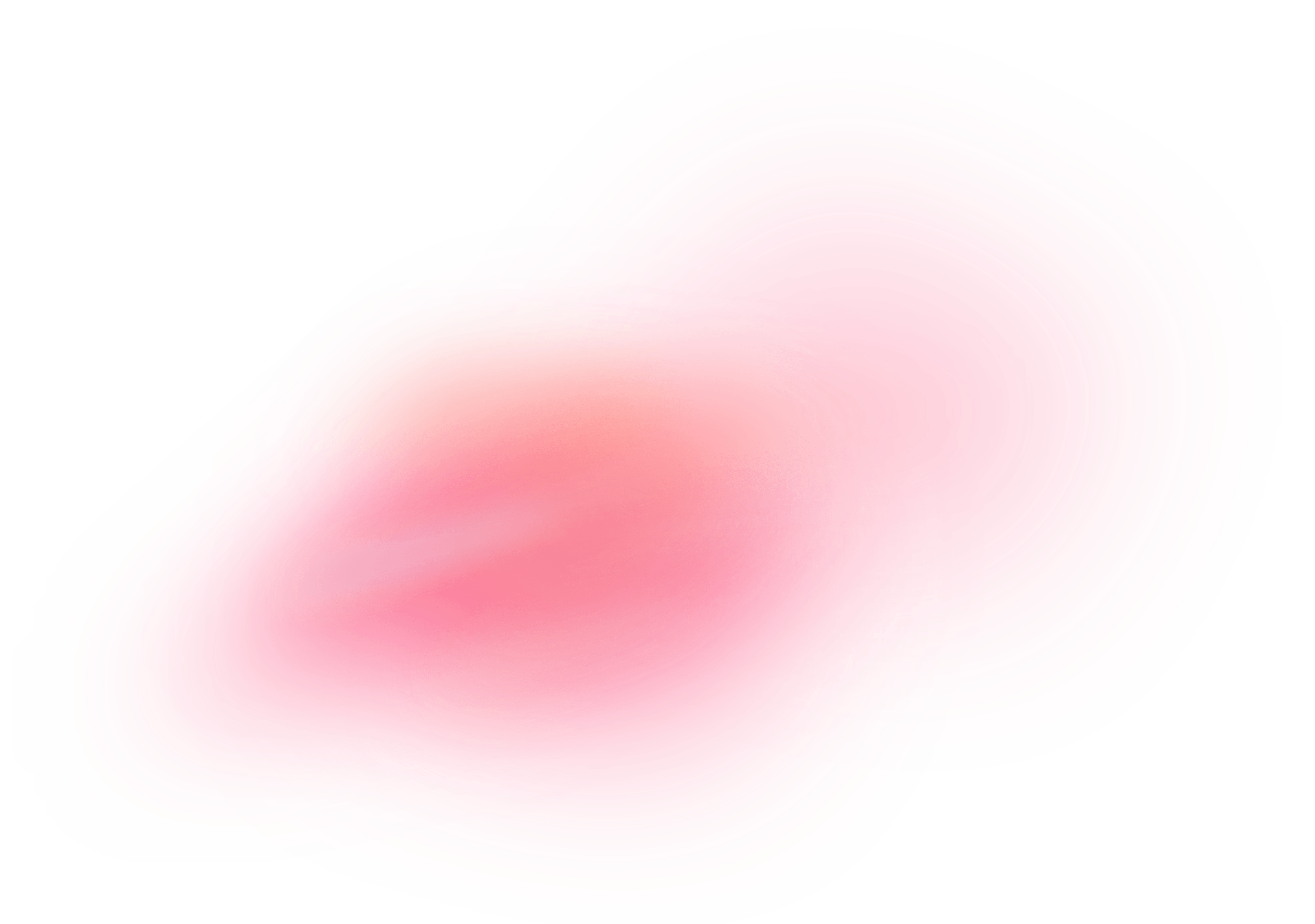