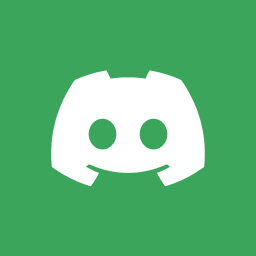
I'm trying to register a new user and then sign them in but got this error: - Error: AppwriteException: User (role: guests) missing scope (account)
Here's the code
// Register user
export async function createUser(email, password, username) { try { const newAccount = await account.create( ID.unique(), email, password, username );
if (!newAccount) throw Error;
const avatarUrl = avatars.getInitials(username);
await signIn(email, password);
const newUser = await databases.createDocument(
appwriteConfig.databaseId,
appwriteConfig.userCollectionId,
ID.unique(),
{
accountId: newAccount.$id,
email: email,
username: username,
avatar: avatarUrl,
}
);
return newUser;
} catch (error) { throw new Error(error); } }
// Sign In
export async function signIn(email, password) { try { await account.deleteSessions(); await account.deleteSession("current"); const session = await account.createEmailPasswordSession(email, password);
return session;
} catch (error) { throw new Error(error); } }
// Get Account export async function getAccount() { try { const currentAccount = await account.get();
return currentAccount;
} catch (error) { throw new Error(error); } }
// Get Current User
export async function getCurrentUser() { try { const currentAccount = await getAccount(); if (!currentAccount) throw Error;
const currentUser = await databases.listDocuments(
appwriteConfig.databaseId,
appwriteConfig.userCollectionId,
[Query.equal("accountId", currentAccount.$id)]
);
if (!currentUser) throw Error;
return currentUser.documents[0];
} catch (error) { console.log(error); return null; } }
Any insights or suggestions on how to fix this would be greatly appreciated! Thanks in advance!

please use code formatting to make it more readable.
use back ticks

AppwriteException: User (role: guests) missing scope (account)
this error means that current session user is not authenticated

in sign in function you dont have to delete sessions on server side. you can directly create new session.
can u specify where the error is coming from??
Recommended threads
- custom domain with CloudFlare
Hi all, it seems that CloudFlare has blocked cross-domain CNAME link which made my app hostname which is in CloudFlare, unable to create a CNAME pointing to clo...
- My organization's project is blocked
My organization's project is blocked so unblocked my organization then I will this
- [SOLVED] React Native Appwrite SDK not w...
So I'm trying to generate a unique ID using the ID.unique() and its generating properly, but its saying its longer than 36 characters but it isnt.. ```typescri...
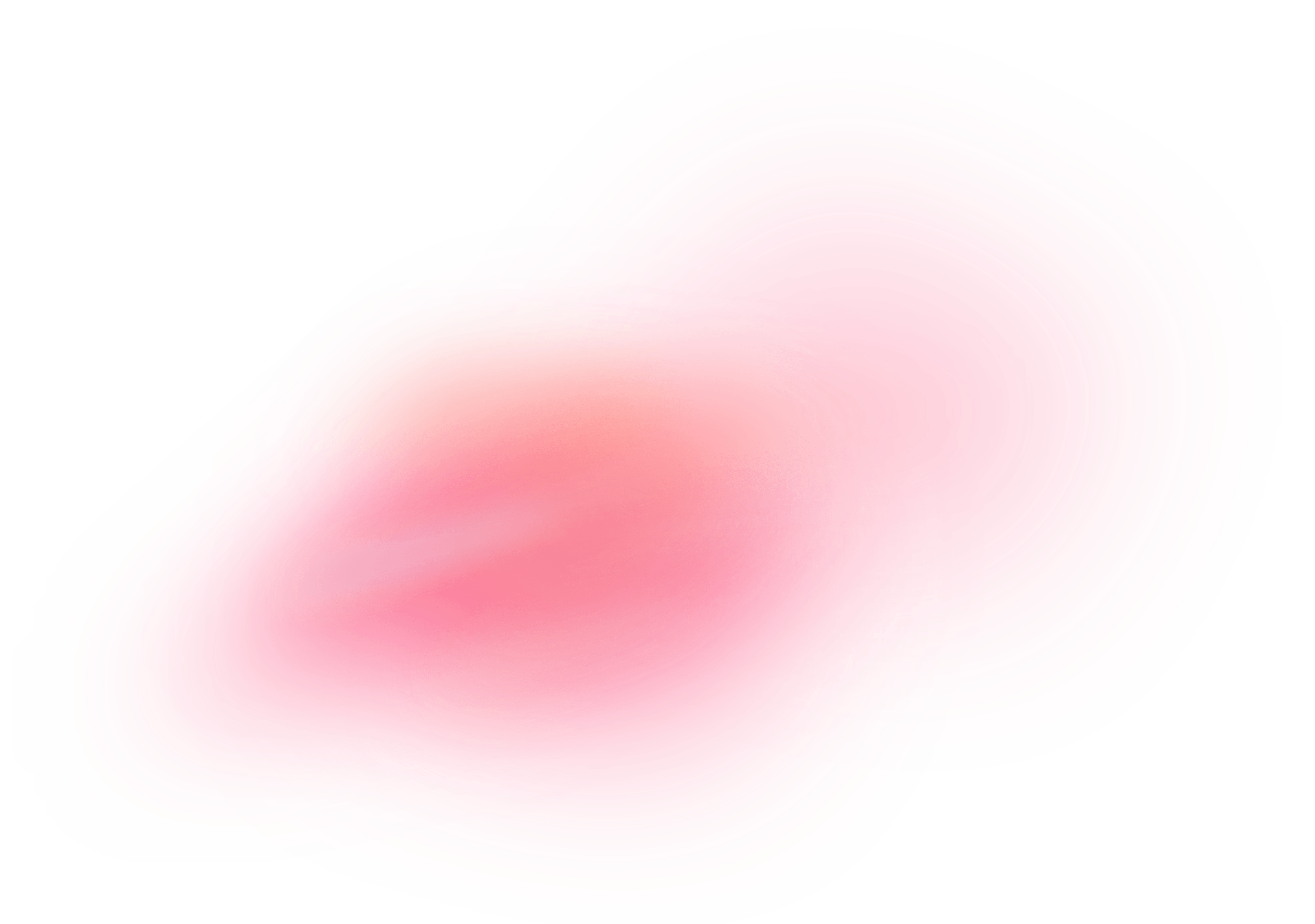