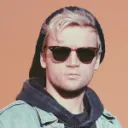
I have the api route in nextjs. then I connect via client app. what is best way to send the quries to the api route. I want to build the query string using the client sdk. is this possible

So you're wanting to make a rest call, and add queries to it?

You can do something like this if that is the case
export function generateQueryList(queries: Query[]) {
const queryList: string[] = [];
queries.forEach((x) => {
queryList.push(`queries[]=${x}`);
});
return queryList.join("&");
}
And you'd use it like this.
/**
* Retrieves a list of documents from a specific collection.
*
* @template {T} - The type of the documents to retrieve.
* @param {string} collectionId - The ID of the collection to retrieve documents from.
* @returns A promise that resolves to an array of documents of type T.
*/
async list<T extends Models.Document>(
collectionId: string,
queries: string[] = [],
) {
const queryList = generateQueryList(queries);
const url = `${ENDPOINT}/databases/${DATABASE_ID}/collections/${collectionId}/documents${queries.length > 0 ? `?${queryList.toString()}` : ""}`;
const response = await fetch(url, {
headers: {
"x-appwrite-project": PROJECT_ID,
},
cache: "no-store",
});
const result: T = await response.json();
return result;
},
};
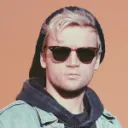
@Kenny yes something like that. then in server, how to parse it back

Not sure I understand what you mean by parse it back /:
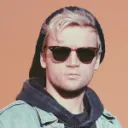
i'm not using the rest to appwrite directly. i will use node-appwrite sdk in server as normal. jusst i need to send rest from client via api
Recommended threads
- Use different email hosts for different ...
Hello, I have 2 projects and i want to be able to set up email templates in the projects. Both projects will have different email host configurations. I see ...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
- Edit ID of an existing collection
Hi there. Is it possible to edit an ID of an existing collection? Right now it looks impossible from AppWrite cloud at least.
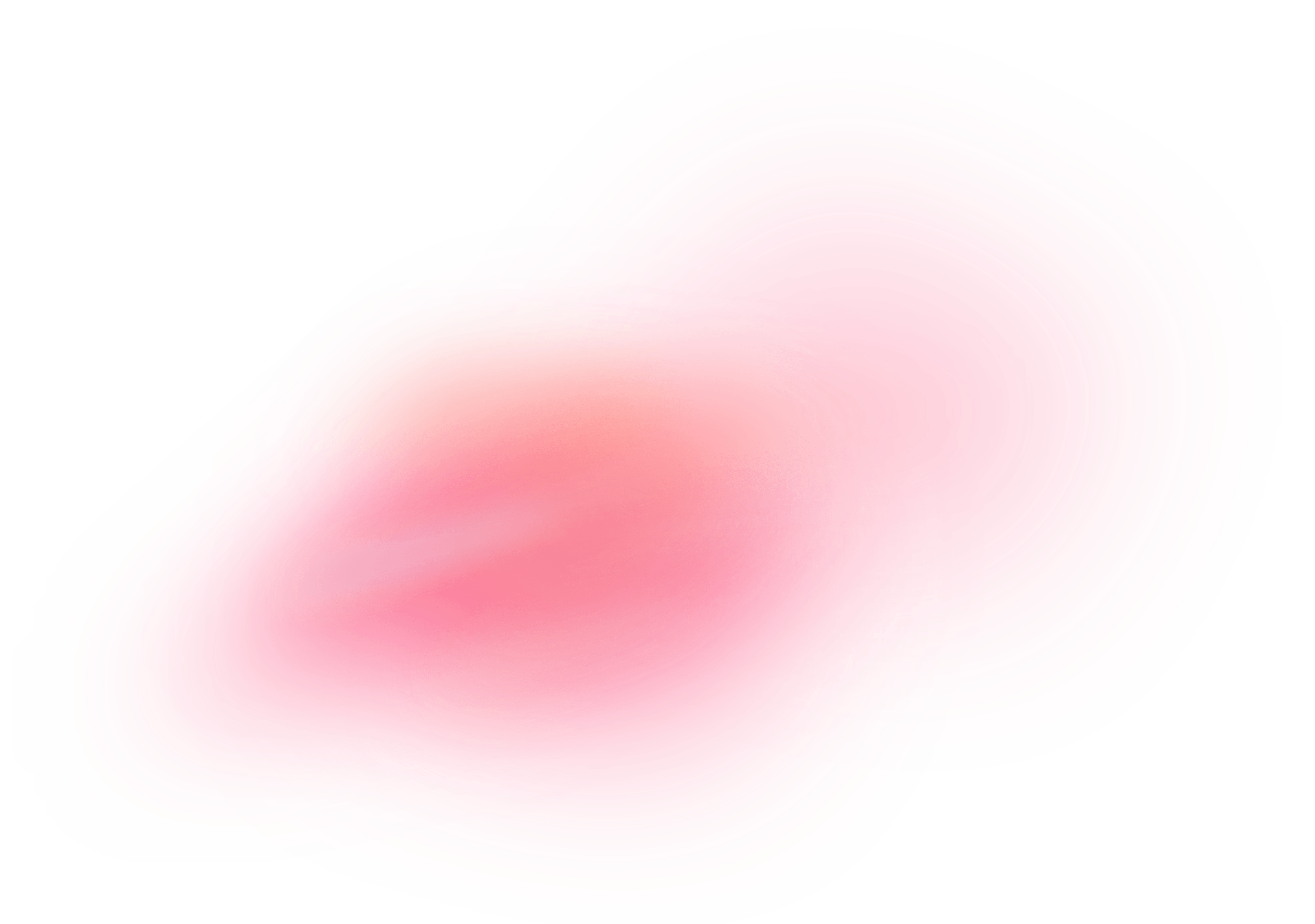