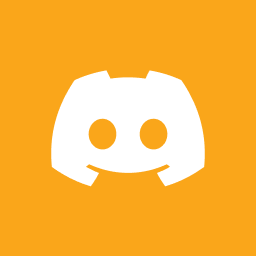
If I want to update the users' preferences, but only one of the values... you wipe out all their preferences. This doesn't make sense. You can try to get all preferences, modify the existing one, and then send it all over... but it's not only bad for network traffic but also horrible for multithreaded environments. You may be updating the whole preferences several times and stepping on each thread ending up with corrupted data.
In addition, you make a mistake and you wipe out all the preferences just trying to modify one key. My point is, when you update preferences it should only update the ones you assign.
Code:
var client = new Client() .SetEndpoint(Utils.AppWriteURL) // Your API Endpoint .SetProject(Utils.AppWriteProjectID) // Your project ID .SetKey(Utils.AppWriteAPI);
Users users = new Users(client);
Dictionary<string, object> dic= new Dictionary<string, object>(); dic["key"] = "value"; await users.UpdatePrefs(user.Id, dic); // this will wipe out all your preferences, all of them, and only place key and value.
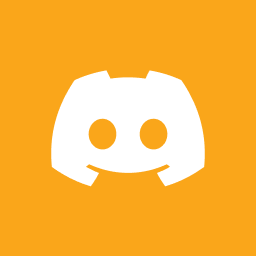
Now imagine you have 2 api calls where you have to update a "picture" key and another with a "username" key. If i use that code, one will wipe out the info of the other.
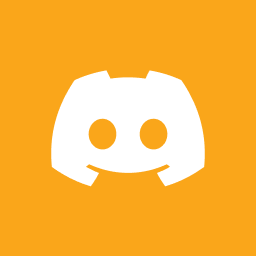
At the moment I'm forced to get the user, get the dictionary, change the value, and send ALL the data back to update.
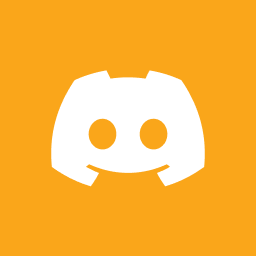
But even then, if 2 threads try to update picture and username independently, you may end up with one of the values lost because you got all the data and replaced all the data.
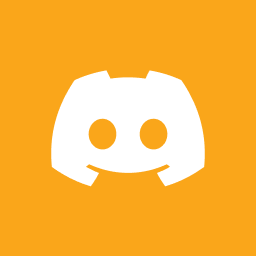
My suggestion is that it should update only the provided "key". And maybe have a different method for replace all keys if you want.
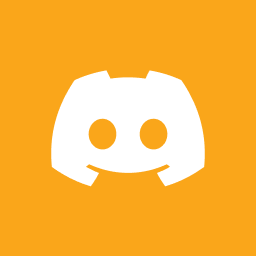
or a "replaceAll" parameter
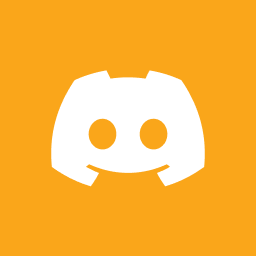
This way you would have 1) Less network traffic 2) Be thread-safer towards updating different preferences 3) Have the option to replace all of them if wanted
Recommended threads
- What Query's are valid for GetDocument?
Documentation shows that Queries are valid here, but doesn't explain which queries are valid. At first I presumed this to be a bug, but before creating a githu...
- Database not found when attempting to Li...
So, I am doing this in postman to eliminate any possible weirdness from my SDK, and still unable to hit any document level endpoint for my database. I am tryin...
- module 'appwrite.query' has no attribute...
In python im running this line: from appwrite import query database = Databases(client).list_documents(database_id= 'xxx', collection_id= 'xxx', queri...
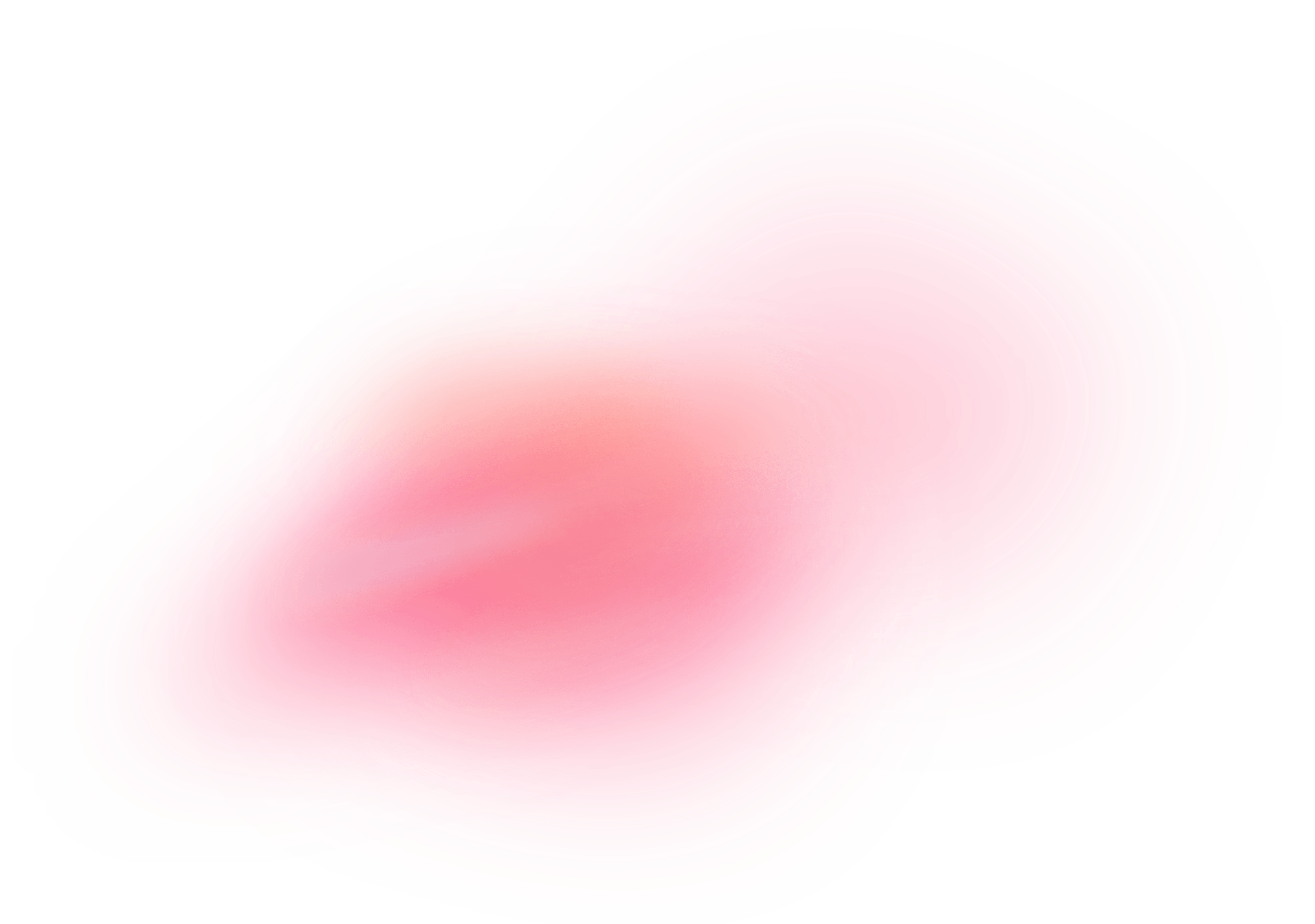