
I need to store a unique id in a const. All I get back from
const id = ID.unique();
is "unique()"
What am I doing wrong? π
Thank you
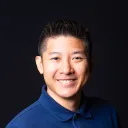
Nothing, this is expected

So, in that case should I use uuid npm package or a regular expression?
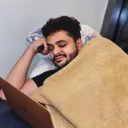
You don't need to use uuid.
ID.unique()
generates a unique ID for you
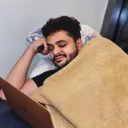
I need to store a unique id in a const What exactly do you need this unique ID for?

@safwan Hi, The main goal is to create user names when registering a new user. I am making several requests one after another to create a usernames record and then user's data and in the end, creating a new user account. All those collections share the same user's unique ID
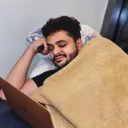
Well then you can just do:
const promise = await account.create(ID.unique(), 'email@example.com', 'password');
This is will automatically generate the unique ID for the user, and account.create
will return a User Object, which includes the generated ID in the $id
attribute

Yes of course, I know that, but because this is my last request in the chain, I don't have the id. This is the last request because I want to be able to cancel the other request if something went wrong
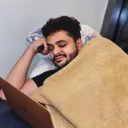
Once again, you can get the ID from the user object that is returned.
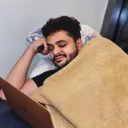
I'm assuming you just want the ID. You don't need to assign ID.unique()
to a variable.
const promise = await account.create(ID.unique(), 'email@example.com', 'password');
const userId = promise.$id;

Let me ask you something important π
I am making the account.create ...
call last because I read the documentations and did not find a way to delete a user from the client side (only server side) if something went wrong.
I can make your example the first call and get the ID. But what if the 2 calls after that are got an error? How do I "undo " account.create ...
?
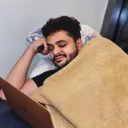
But what if the 2 calls after that are got an error? Are these two calls user-specific actions like creating a document, adding default user preferences or something like that?
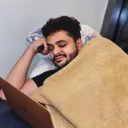
Regardless of that, the reason deleting a user is available only on the server-side is for obvious security reasons.
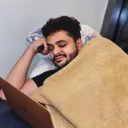
So if you want to delete a user at any point in time, you can do this:
- Create a Cloud Function that uses the Users API. Specifically the Delete User endpoint. Make sure it grabs the
userId
from the request payload. - You can execute this function from the client-side using the Create Execution endpoint of the Functions API. Make sure to send the
userId
in the request payload
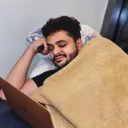
In your particular use-case, wrap all of the calls you are making after the account.create
in a try-catch
and have the functions.createExecution
in the catch
.

First, thank you very much for the help. I will try that @safwan
Recommended threads
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
- Update User Error
```ts const { users, databases } = await createAdminClient(); const session = await getLoggedInUser(); const user = await users.get(session.$id); if (!use...
- apple exchange code to token
hello guys, im new here π I have created a project and enabled apple oauth, filled all data (client id, key id, p8 file itself etc). I generate oauth code form...
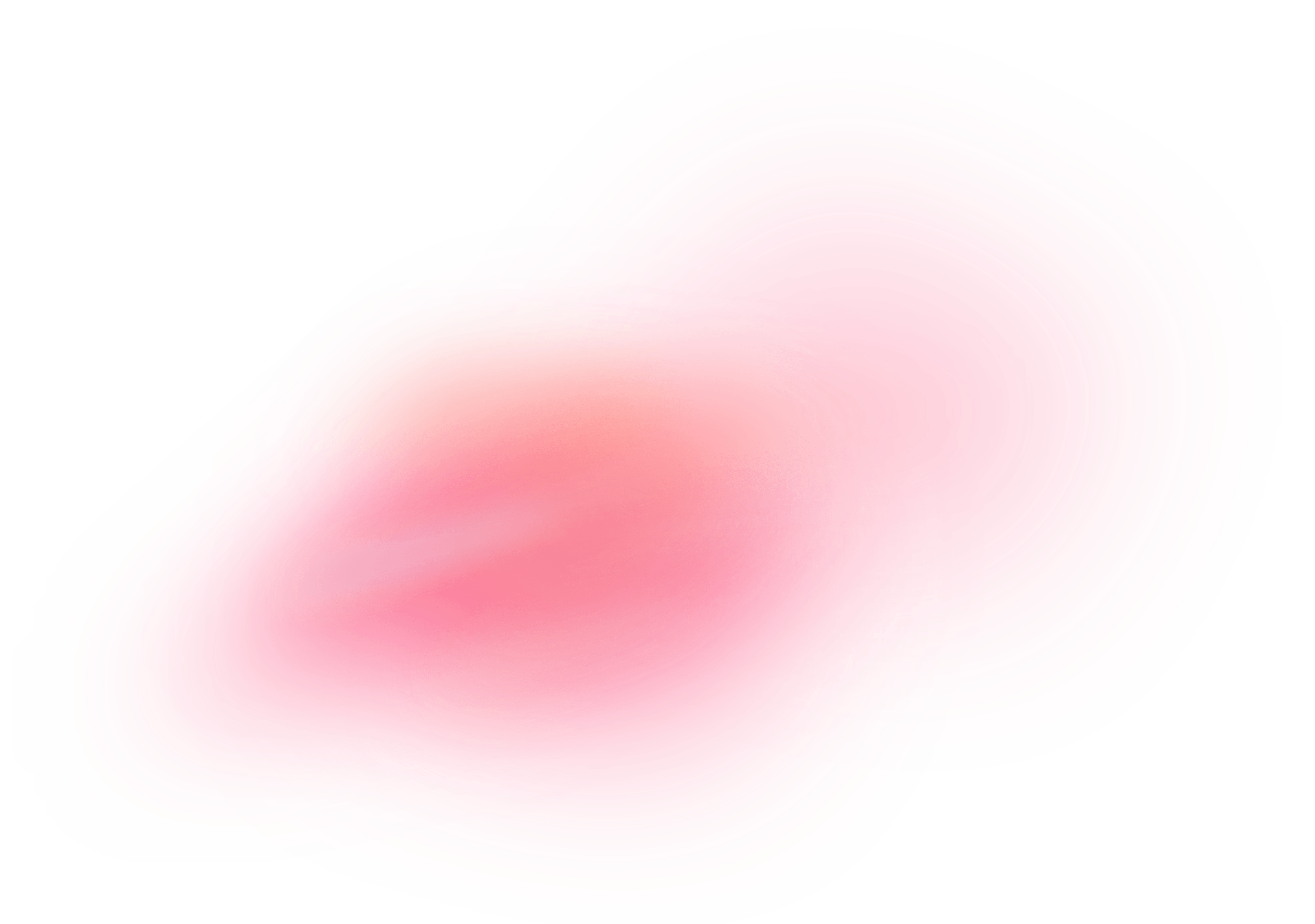