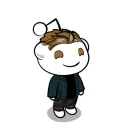
Hi, I'm using Google OAuth and Appwrite 1.4.1 and before with 1.3.8 I never got this error: Invalid success param: URL host must be one of: localhost, backend.justitis.it
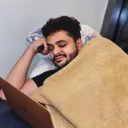
Can you show your code?
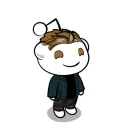
import 'dart:convert';
import 'package:appwrite/models.dart';
import 'package:flutter/material.dart';
import 'package:justitis_app/services/appwrite_service.dart';
enum AuthStatus{
uninit, auth, unauth
}
class AuthProvider extends ChangeNotifier{
late User _currentUser;
double _wallet = 0.0;
double _moneyToAdd = 0.0;
AuthStatus _authStatus = AuthStatus.uninit;
// Getter
User get currentUser => _currentUser;
AuthStatus get authStatus => _authStatus;
double get wallet => _wallet;
double get moneyToAdd => _moneyToAdd;
set moneyToAdd(double money) => _moneyToAdd = money;
// Remote Server (deprecated)
// final String userCollectionId = '64d295100d412a890d19';
// final String _successRedirectUrl = 'https://test.justitis.it/auth.html';
//Local Server
final String _userCollectionId = 'users';
final String _successRedirectUrl = 'http://test.justitis.it/auth.html';
AuthProvider(){
checkIfLogged();
}
void checkIfLogged() async{
try{
_currentUser = await AppwriteService.account.get();
_authStatus = AuthStatus.auth;
updateWallet();
}catch(e){
_authStatus = AuthStatus.unauth;
}finally{
notifyListeners();
}
}
void updateWallet() async{
try{
var result = await AppwriteService.database.getDocument(databaseId: AppwriteService.databaseId, collectionId: _userCollectionId, documentId: _currentUser.$id);
var document = result.toMap();
_wallet = (document['data']['balance'] + 0.0);
}finally{
notifyListeners();
}
}
void logIn() async{
try{
//final session =
await AppwriteService.account.createOAuth2Session(provider: 'google', success: _successRedirectUrl);
_currentUser = await AppwriteService.account.get();
_authStatus = AuthStatus.auth;
updateWallet();
}finally{
notifyListeners();
}
}
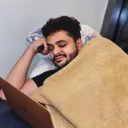
I think it’s because you didn’t provide a failure url.
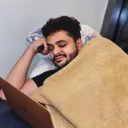
Either have both success and failure, or none
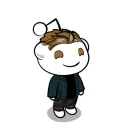
ok, i'll try
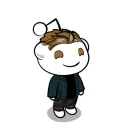
nope, same error
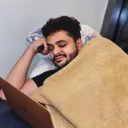
Both ways? Removing the success and failure params gave same error?
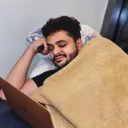
This is what I meant
void logIn() async{
try{
//final session =
await AppwriteService.account.createOAuth2Session(provider: 'google');
_currentUser = await AppwriteService.account.get();
_authStatus = AuthStatus.auth;
updateWallet();
}finally{
notifyListeners();
}
}
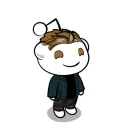
now started working
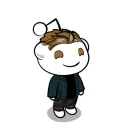
thank you!
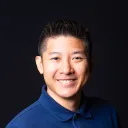
[SOLVED] Invalid success
param

Success and failure URL must be a subdomain of your Appwrite instance AND added as a Web platform.

I think that might be why
Recommended threads
- Apple OAuth Scopes
Hi Hi, I've configured sign in with apple and this is the response i'm getting from apple once i've signed in. I cant find anywhere I set scopes. I remember se...
- Sign In With Apple OAuth Help
Hi All! I've got a flutter & appwrite app which Im trying to use sign in with apple for. I already have sign in with google working and the function is the sam...
- [SOLVED] OAuth With Google & Flutter
Hi all, I'm trying to sign in with google and it all goes swimmingly until the call back. I get a new user created on the appwrite dashboard however the flutte...
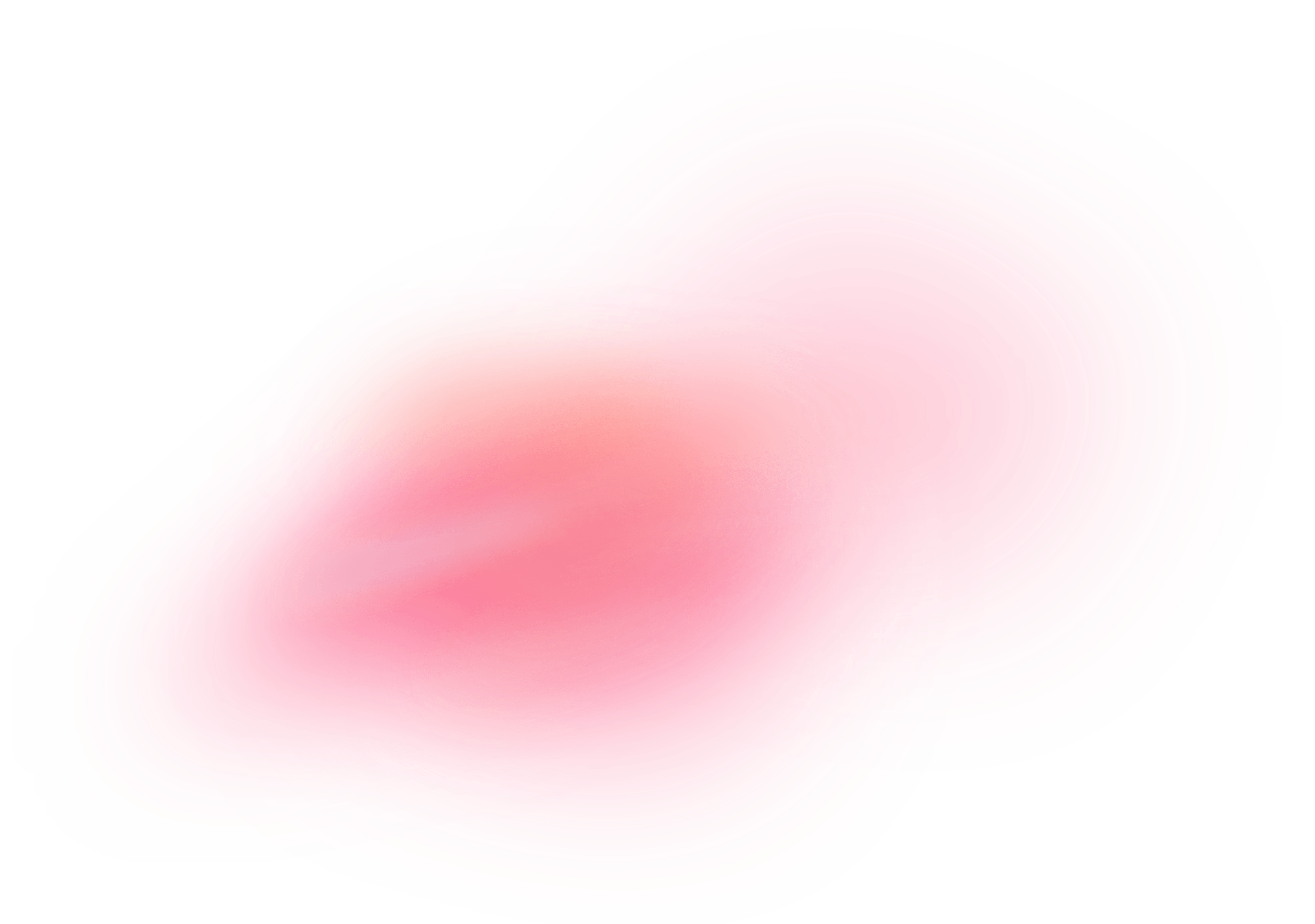