How to read the most recent 25 documents, then the next 25 documents after?
- 0
- Databases
- General
- Web
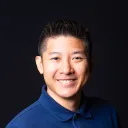
Please share your code
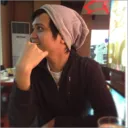
let filters = [
Query.orderAsc('$createdAt'),
Query.limit(25)
];
if (documents.length) {
filters.push(Query.cursorAfter(documents[0].$id));
}
const response = await databases.listDocuments(
Constants.APPWRITE_DATABASE_ID,
Constants.APPWRITE_COLLECTION_ID,
filters
);
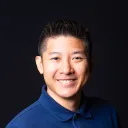
And then?
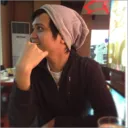
Well this is how I fetch the documents. This was supposed to give me the most recent documents but it's giving me the oldest documents. I haven't really coded the part the fetches the next page.
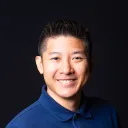
Order ascending created at gives you the oldest first. And you put cursor after the 1st document so it's going to return starting from the 2nd document
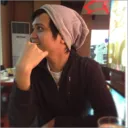
So should I sort in descending order when listing the documents, get the 25 items, then on the frontend of my application, I reverse the sorting?
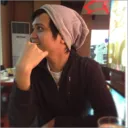
I need the documents sorted in ascending order.
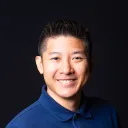
Maybe use cursor before?
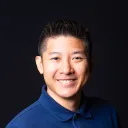
But you'd need to pass in the newest document so that it will fetch the older ones
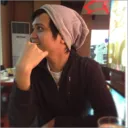
This works for when I grab the documents in an ascending order - basically from oldest to newest:
const getDocuments = async () => {
let queries = [
Query.orderAsc('$createdAt'),
Query.limit(25)
];
if (documents.length) {
queries.push(Query.cursorAfter(documents[documents.length - 1].$id));
}
const response = await databases.listDocuments(
Constants.APPWRITE_DATABASE_ID,
Constants.APPWRITE_COLLECTION_ID,
queries
);
const documentsList = response.documents;
setMessages((prevState) => [...prevState, ...documentsList]);
};
I just want to reverse this to get the most recent document first then to the oldest.
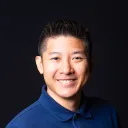
To get the most recent document, you'll need to order by created at descending...
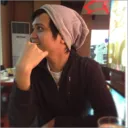
Yes that is what I initially did. I sorted in descending order. But then I had problems getting the next page. I tried both cursor before and after and I don’t think it looks correct. I’ll take a look again tomorrow and see if I can explain it better. I’m no longer on my computer so I can’t show my current code.
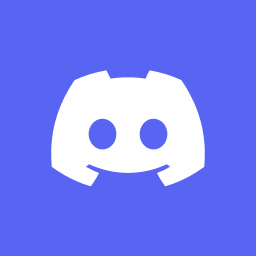
Hey, sure, let us know if it doesnt work and we can help debug
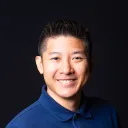
you would sort descending just to get the last document. then you would use the cursorBefore(lastDocument.$id)
without any ordering after
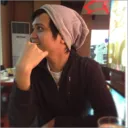
Aaaah, I see what you mean. I'll try this out later after work. Thanks for the suggestion! I think that should fix my issue!
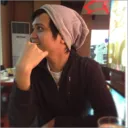
I really can't get this to work. Let me try to illustrate what I'm trying to do. To scale it down a bit let's pretend I have 15 documents in my collection:
Doc 1
Doc 2
...
Doc 15
So if I want to get the 5 most recent documents first I would call listDocuments
in descending order and a limit of 5. That would then give me:
Doc 15
Doc 14
Doc 13
Doc 12
Doc 11
When I display the results on my page, I do want the documents listed in ascending order:
Doc 11
Doc 12
Doc 13
Doc 14
Doc 15
When I scroll all the way up to the end of the container, I want to fetch the next most recent documents (documents 6-10 on the second request and documents 1-5 for the final request) and add the results to my component's state. This is the part where I'm unable to get it to work. If I use cursorBefore(lastDocument.$id)
, the cursor will be pointing to Doc 10 because my last document was Doc 11. And if I make another request for the next 5 documents, it will give me:
Doc 10
Doc 11
Doc 12
Doc 13
Doc 14
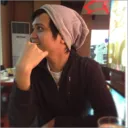
If I use cursorAfter(lastDocument.$id)
, the cursor would be pointing to Doc 12 because my last document was Doc 11. And if I make another request for the next 5 documents, it will give me:
Doc 12
Doc 13
Doc 14
Doc 15
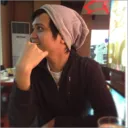
The way I see it is that I need to set the cursor 5 documents before my last document (the number of documents I fetch in each batch) for this to work. So in my example, once I fetch Doc 15, 14, 13, 12 , and 11 - I would set my cursor all the way to 6 (11 minus 5) and then do another fetch which should give me Doc 6, 7, 8, 9, and 10.
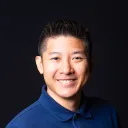
You're supposed to use cursor before and pass in doc 11 so that you'll get docs 5 - 10
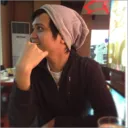
This is what I'm already doing and it gives me docs 10-14.
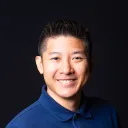
You're passing in doc 15 and not doc 11
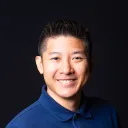
Maybe share your full code
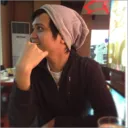
const getDocuments = async () => {
let queries = [Query.limit(Constants.DOCUMENTS_PER_BATCH)];
if (documents.length) {
// Subsequent fetches
queries.push(Query.cursorBefore(documents[documents.length - 1].$id));
} else {
// First fetch
queries.push(Query.orderDesc('$createdAt'));
}
const response = await databases.listDocuments(
Constants.APPWRITE_DATABASE_ID,
Constants.APPWRITE_COLLECTION_ID,
queries
);
// Reverse on the first fetch because it's sorted in descending order
const documentsList = messages.length ? response.documents : response.documents.reverse();
// Add the new items at the start of the array since they are older documents
setDocuments((prevState) => [...documentsList, ...prevState]);
};
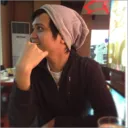
Looks like I need to use documents[0].$id
instead of documents[documents.length - 1].$id
since the oldest document is the first element on my array.
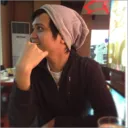
I think that fixes my issue. Although as a feedback, this process doesn't feel intuitive in my mind. When the fetching is all in ascending order, it feels great, the experience was intuitive. But when fetching in reverse, not at all intuitive.
Regardless, thanks for your help as always!!
Recommended threads
- The current user is not authorized to pe...
I want to create a document associated with user after log in with OAuth. The user were logged in, but Appwrite said user is unauthorized. User is logged in wi...
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
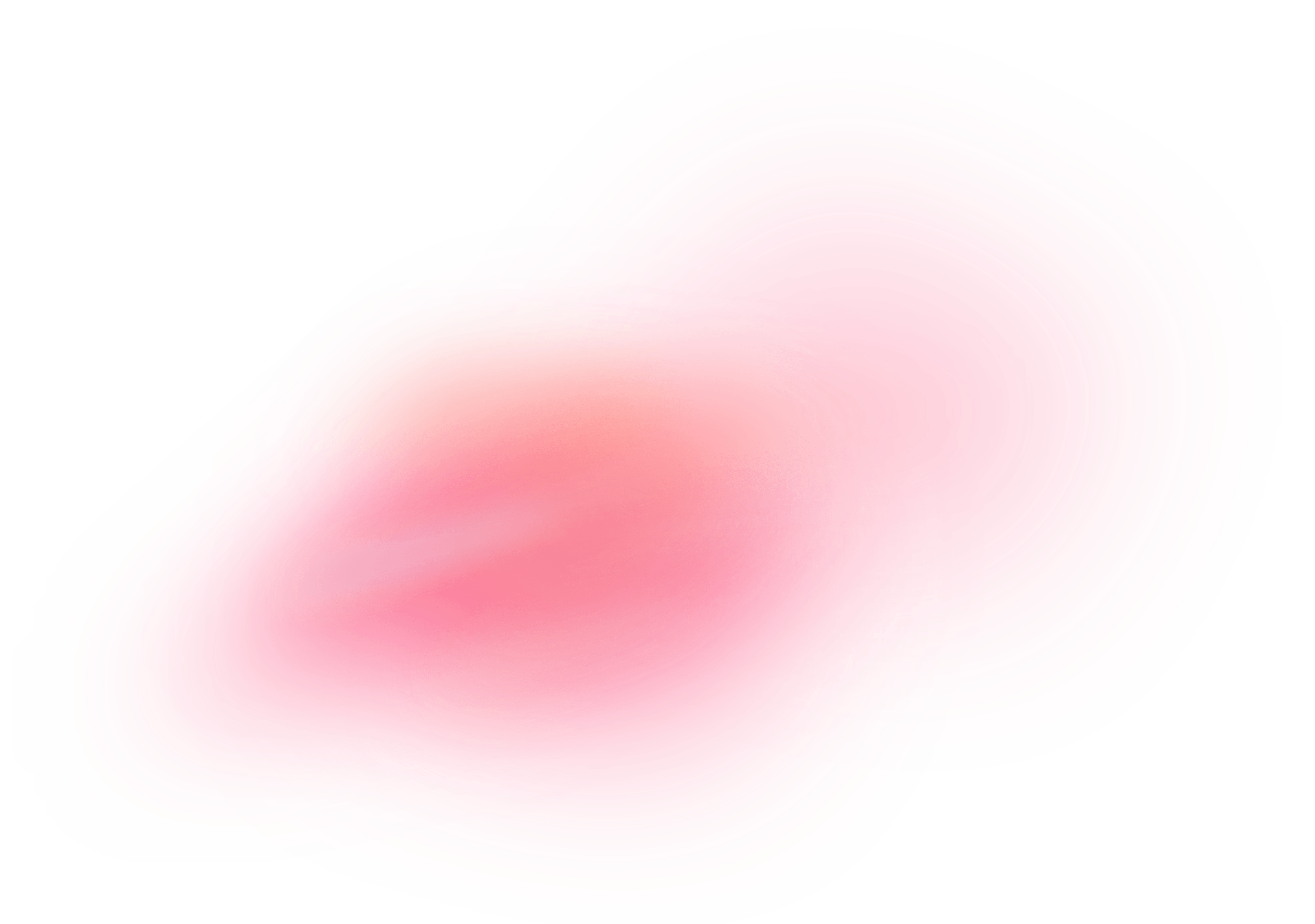