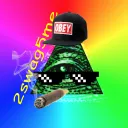
I have tried this on multiple machines with a fresh install of AppWrite: Based on example on https://appwrite.io/docs/server/databases?sdk=nodejs-default#databasesCreate
const sdk = require("node-appwrite");
module.exports = async function (req, res) {
const client = new sdk.Client();
const database = new sdk.Databases(client);
if (
!req.variables['APPWRITE_FUNCTION_ENDPOINT'] ||
!req.variables['APPWRITE_FUNCTION_API_KEY']
) {
// Changed 'console.warn()' to 'console.log()' or else I would never see this message.
console.log("Environment variables are not set. Function cannot use Appwrite SDK.");
} else {
client
.setEndpoint(req.variables['APPWRITE_FUNCTION_ENDPOINT'])
.setProject(req.variables['APPWRITE_FUNCTION_PROJECT_ID'])
.setKey(req.variables['APPWRITE_FUNCTION_API_KEY'])
.setSelfSigned(true);
}
const promise = database.create(sdk.ID.unique(), 'test');
// This part does not output anything :( (see attached images)
promise.then(function (response) {
console.log(response);
}, function (error) {
console.log(error);
});
res.json({
areDevelopersAwesome: true,
});
};
Am I missing something?
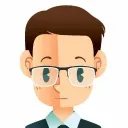
Console log logs don't appear in the logs/errors tabs It will be implented in future You can see more here https://github.com/appwrite/appwrite/discussions/5016 at the section Logging
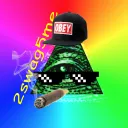
Ok! I will keep that in mind, thanks for the fast reply.
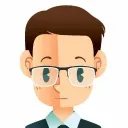
For now what I'm doing is to add the logs back to the request when I'm developing, like so
let logs = '';
// This part does not output anything :( (see attached images)
promise.then(function (response) {
logs = response;
}, function (error) {
logs = error;
});
res.json({
logs: logs,
areDevelopersAwesome: true,
});
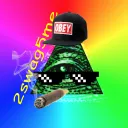
Great, I will copy your solution for now. I thought it may have been a bug :p
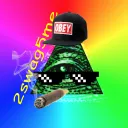
This "Issue" is resolved!
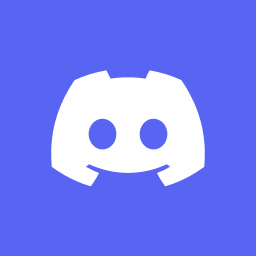
Please mark it as resolved @TheIlluminatiMember
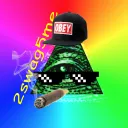
I closed it, is that ok?
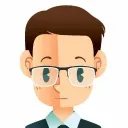
You can edit the title and [Solved] - before it
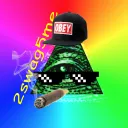
[SOLVED] Issue: no log output on error
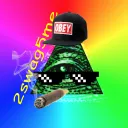
This may help anyone facing the same issue.
Avoid using .then()
and .catch()
! Use async
, await
in combination with a try/catch block:
try {
const response = await database.create(sdk.ID.unique(), 'test');
console.log(response)
} catch (error) {
console.log(error)
}
Logging like this will work. In addition: throwing errors will (intentionally) fail the function and will be listed under the "Errors" tab. See attached image for example.
if (!payload.name) {
throw new Error('No name was supplied, aborting...');
}
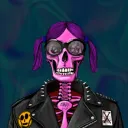
This should be included in the sample files in the docs.
Recommended threads
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
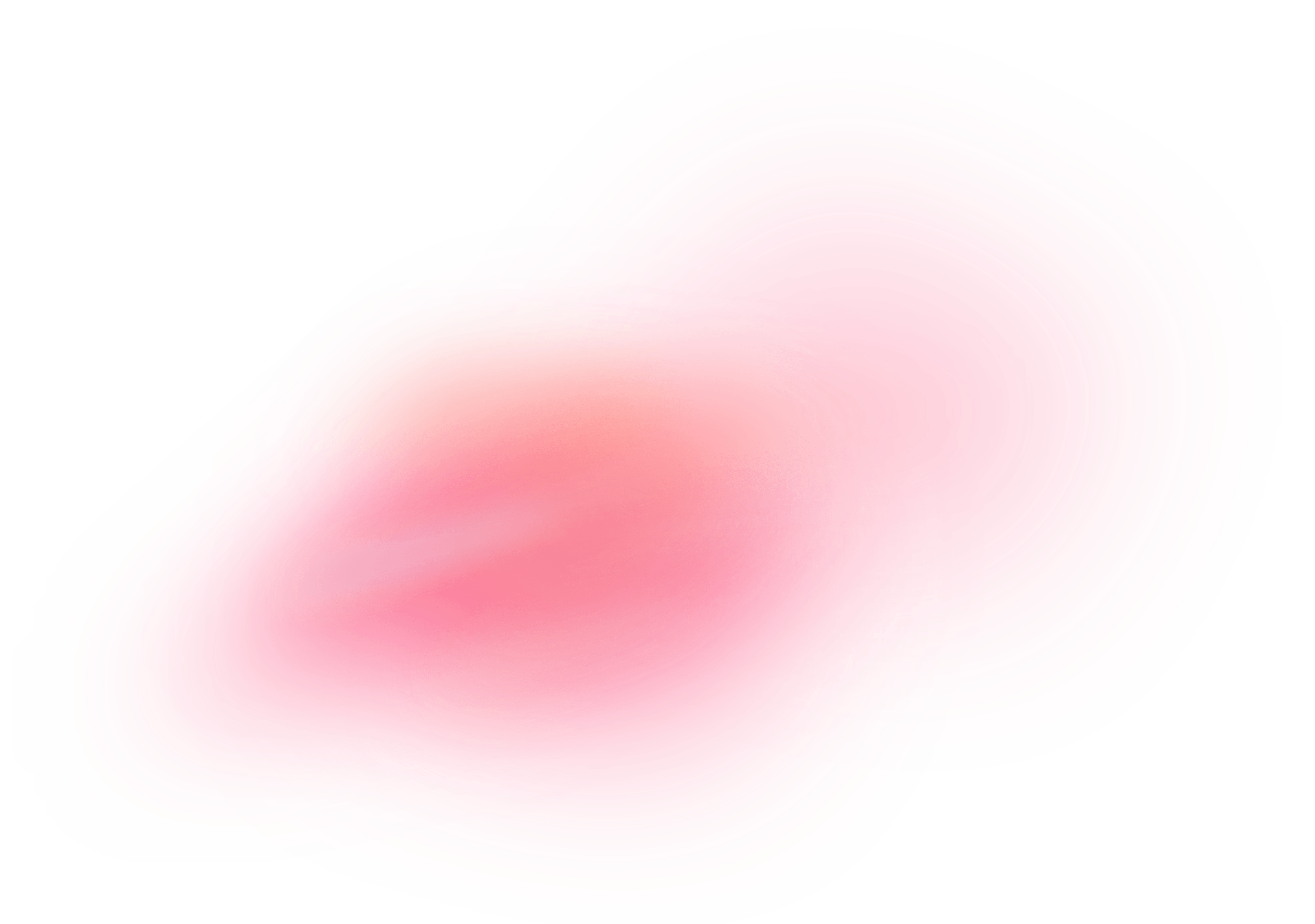