
Hello, In the cloud function, I try to make a POST API request, after which I need an appropriate response. And here is a strange situation - when I send a same request to the same API via POSTMAN or via a function from my local mobile application, I receive a correct response, while when the request is sent via cloud function, I receive the response status code 403 Forbidden. I was blocked by CORS policy when trying to send a request from a web application. Could this be the same type of problem? Could the problem is somewhere else?
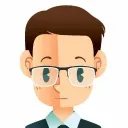
What is the context? How do you run the request with what function on which environment?

I execute a cloud function, where the API request is placed.
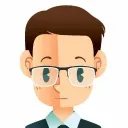
Okay.
So fetch
inside node js deployed function ?

The function is properly deployed and execution status is completed. The request made to another API works fine, but it is clearly visible that in this case (API with CORS policy) there is a problem in Appwrite cloud function.
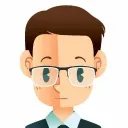
I understand But if you are using the node SDK that it's pretty not possible for it to meet CORS condition as the request is from a CLI and not from HTTP Can you share your code

I am using Dart SDK. Here is my code:

import 'package:http/http.dart' as http;
import 'dart:convert';
import 'package:dart_appwrite/dart_appwrite.dart';
Future<void> start(final req, final res) async {
var headers = {
'Authorization': apiKey,
'Content-Type': 'application/json'
};
var request = http.Request('POST', Uri.parse('https://api.myapi.com/v1/products'));
request.body = json.encode({
"type": "Product",
"name": "Gloves",
"condition": "New",
"code": "714",
"imei": "714",
"category": "",
"model": "655954",
"additionalModels": "",
"status": "Active",
"oneTimeUse": false,
"inventoryControl": true,
"TaxIncludedInCost": true,
"cost": 0,
"TaxIncludedInPrice": true,
"price": 0,
"enableOfferPrice": true,
"offerStart": "15-Feb-2023 13:10",
"offerEnd": "06-Mar-2024 13:44",
"TaxIncludedInOfferPrice": true,
"offerPrice": 5,
"tax": 34513,
"discountable": true,
"description": "desc",
"stockQuantity": 8
});
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200 || response.statusCode == 201) {
res.json({'res': await response.stream.bytesToString()});
print(await response.stream.bytesToString());
}
else {
res.json({'res': response.reasonPhrase});
}
}
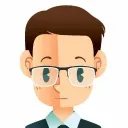
So over here you get CORS?

over here I got 403 Forbidden
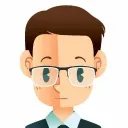
Ohh, So here you don't get CORS,
I would recommend you'll check the headers, that the
var headers = {
'Authorization': apiKey,
'Content-Type': 'application/json'
};
That they are exactly like in the postman
For start return the header in the json
res.json({
'res': response.reasonPhrase
'headers': headers
});
And compere them to the Postman one
Because so far you code looks intact
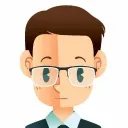
And I assume that you've redact the apiKey
for the example, right?

Headers is the same. I copied the generated code snipped from POSTMAN to my cloud function. And that's right - apiKey is for example
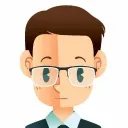
It's really weird as CORS
cannot occurs when running CLI, and the only problem is the 403
So, what I think can be the problem is that somehow your wanted API are blocking you server IP from any reason.
And just to prove it try this way instead of the one you're using (Which is how I send my requests)
import 'package:dart_appwrite/models.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
import 'package:dart_appwrite/dart_appwrite.dart';
Future<void> start(final req, final res) async {
var headers = {
'Authorization': apiKey,
'Content-Type': 'application/json'
};
final data = {
"type": "Product",
"name": "Gloves",
"condition": "New",
"code": "714",
"imei": "714",
"category": "",
"model": "655954",
"additionalModels": "",
"status": "Active",
"oneTimeUse": false,
"inventoryControl": true,
"TaxIncludedInCost": true,
"cost": 0,
"TaxIncludedInPrice": true,
"price": 0,
"enableOfferPrice": true,
"offerStart": "15-Feb-2023 13:10",
"offerEnd": "06-Mar-2024 13:44",
"TaxIncludedInOfferPrice": true,
"offerPrice": 5,
"tax": 34513,
"discountable": true,
"description": "desc",
"stockQuantity": 8
};
try {
var response = await http.post(Uri.parse('https://api.myapi.com/v1/products'),
headers: headers,
body: jsonEncode(data),
);
if (response.statusCode == 200 || response.statusCode == 201) {
print(await response.body);
// res.json({'res': await response.body});
}
} catch (e) {
res.json({'res': response.reasonPhrase});
}
}
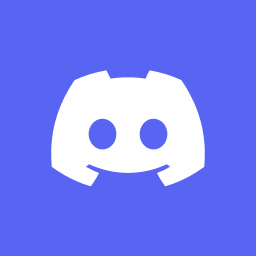
Hi @katskoru is your query resolved?

Hello @joeyouss Unfortunately not.
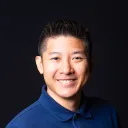
How are you initializing the apiKey? You might want to return the response body from your API.
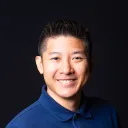
Btw, there's no need to await response.body
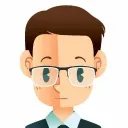
Agreed it was copied from the original one
Recommended threads
- How to Avoid Double Requests in function...
I'm currently using Appwrite's `functions.createExecution` in my project. I want to avoid double requests when multiple actions (like searching or pagination) a...
- Project in AppWrite Cloud doesn't allow ...
I have a collection where the data can't be opened. When I check the functions, there are three instances of a function still running that can't be deleted. The...
- Get team fail in appwrite function
I try to get team of a user inside appwrite function, but i get this error: `AppwriteException: User (role: guests) missing scope (teams.read)` If i try on cl...
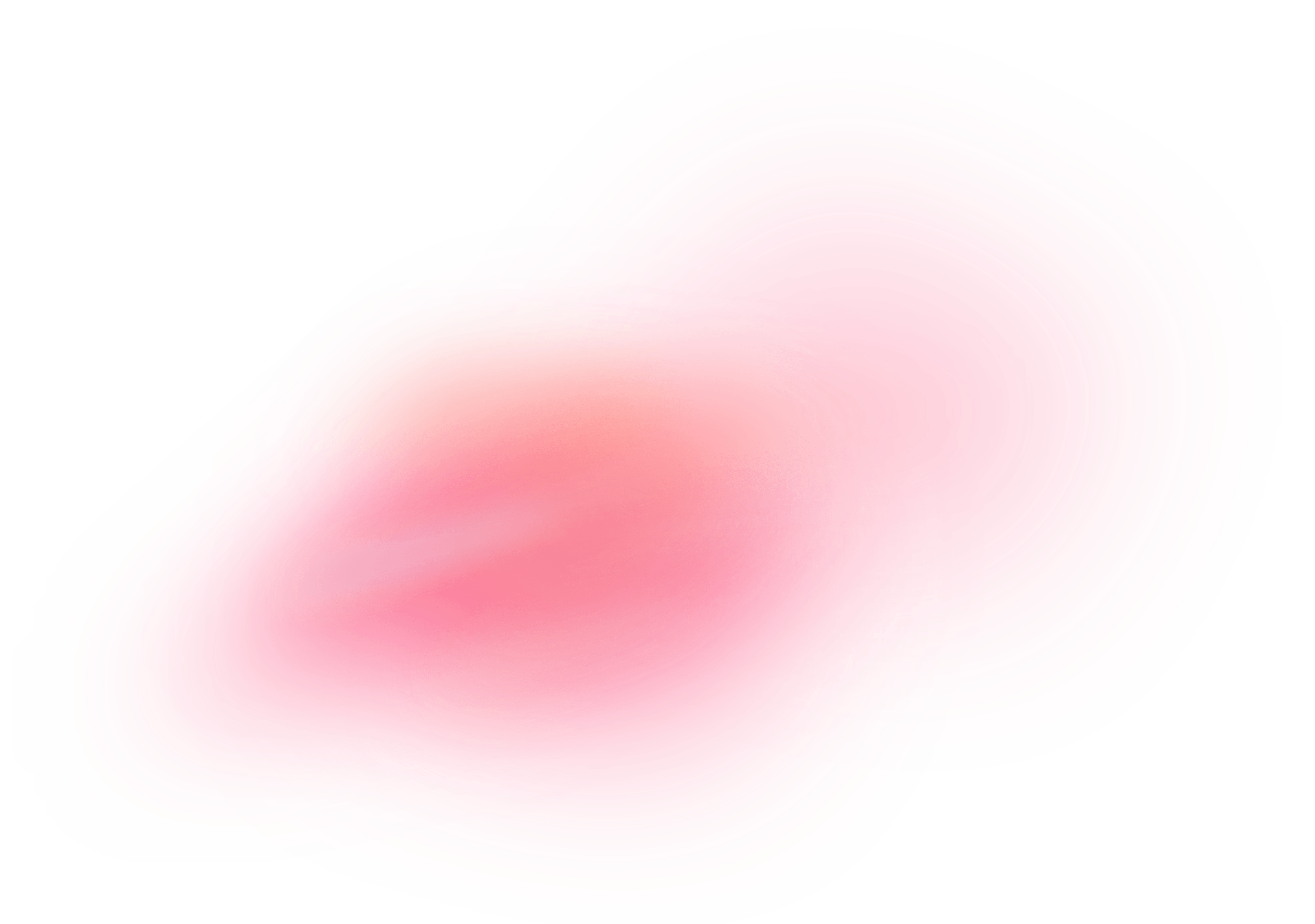