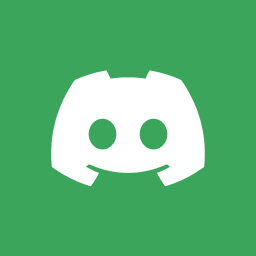
Hello Appwrite Support,
I'm experiencing an issue with the password reset process. The secret key sent with the redirect URL is 256 characters long, which exceeds the 255-character limit set by Appwrite. When using the account.updateRecovery method, I receive the following error: "AppwriteException: Invalid document structure: Attribute "key" has invalid type. Value must be a valid string and no longer than 255 chars"
Code snippet to handle the password reset: import { useState, useEffect } from "react"; import { account } from "../Backend/appwriteConfig"; // Assumes correct setup const NewPassword = () => { const [form, setForm] = useState({ password: "", confirmpassword: "" }); const [secret, setSecret] = useState(""); useEffect(() => { const queryParams = new URLSearchParams(window.location.search); const secretKey = queryParams.get("secret"); if (secretKey) { setSecret(secretKey); } else { console.error("Invalid password reset link."); } }, []); const updatePassword = async (password, secret) => { try { // Attempting to update the password with a secret key of 256 characters const trimmedSecret = secret.slice(0, 255); // Trimmed version const res = await account.updateRecovery("userId", trimmedSecret, password); // User ID used here for example console.log("Password updated successfully:", res); } catch (error) { console.error("Error updating password:", error); } }; // Form submission logic here... };
Questions:
- Is it safe to trim the secret key to 255 characters, or could this compromise security?
- Is there support for handling secret keys longer than 255 characters, possibly through a premium feature or configuration setting?
- Is this key length issue a known limitation or a bug?
- I am using the latest Appwrite SDK on a free plan. Please advise on how to proceed.
Thank you for your assistance!
Best regards! Gift Jackson codewithjacksun@gmail.com
Recommended threads
- Transfer from Anonymous to Real User
I am trying to transfer from an Anonymous User to a Real User. The only methods that I use inside my Flutter App to log in are passwordless(magicLink and oAuth)...
- Can't add CC to send email function
Hi, I was trying to create an email notification function, the code below works perfectly for single recipient, but I can't added more recipients using cc/bcc. ...
- Using OIDC Oauth2 Provider, seems to inj...
Is this typical behavior? Is there any way to prevent an email scope being added?
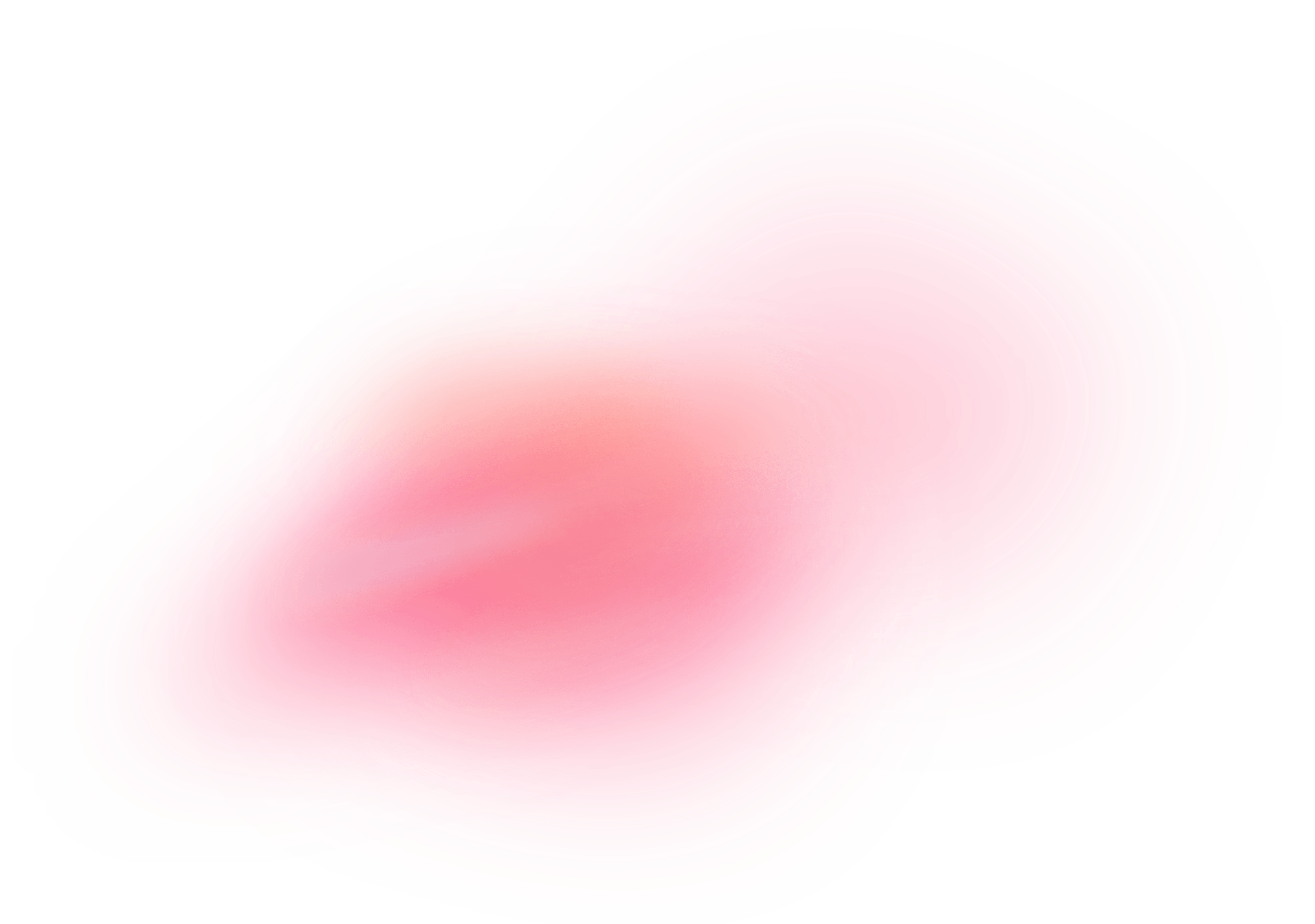