
// Create the Appwrite client.
const { account } = createAdminClient();
// Create the session using the client
const session = await account.createEmailPasswordSession(username, password);
How can I obtain the user object from this?
I use this code in my hooks.server.ts
const { account } = createSessionClient(event);
let appwriteUser = await account.get();
But running account.get() on the account variable that I get from createAdminClient will throw a error:
"(role: applications) missing scope (account)"
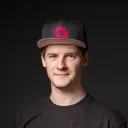
Hii 👋 Is this Svelte Kit?

Yupp
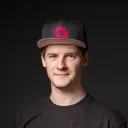
Can you please show us your createSessionClient()
function?

export function createSessionClient(event: any) {
const client = new Client()
.setEndpoint(PUBLIC_APPWRITE_ENDPOINT)
.setProject(PUBLIC_APPWRITE_PROJECT);
// Extract our custom domain's session cookie from the request
const session = event.cookies.get(SESSION_COOKIE);
if (!session) {
redirect(301, "/login");
}
client.setSession(session);
// Return the services we want to use.
return {
get account() {
return new Account(client);
}
};
}
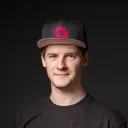
Hmm, nowhere in this method you setKey
. But the error seems to be that API Key is used when doing account.get()
. Can you please ensure account
is from this clientSession call, and not from clientAdmin?

The problem isn't in the createSessionClient()

I can successfully obtain the authed user from that function, its when I do the login that the issue occurs. I want to get the appwrite user object once I initilize a session with createEmailPasswordSession
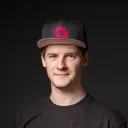
For this scenario, considering you have admin session already, you could:
session = await createEmailPasswordSession(...)
userId = session.userId
user = users.get(userId)
(users
is new Users(client)
where client
is from createAdminClient()
)

const { account } = createAdminClient();
const users = new Users(account)
Passing the value of createAdminClient into the Users is throwing an error
Argument of type 'Account' is not assignable to parameter of type 'Client'.

client doesn't exist on createAdminClient
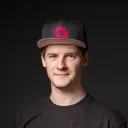
I meant something like this:
const { users } = createAdminClient();
const user = await users.get(userId);
You might need to define this inside createAdminClient
with getter, something along
// Return the services we want to use.
return {
get users() {
return new Users(client);
}
};

ah, i see

@Meldiron how do I set what perfs there are?
const user: Models.User<Models.Preferences>
Error: Property 'domain' does not exist on type 'Preferences'.
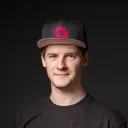
You can do Models.User<any>
to not type it at all, and it will work.
Otherwise you could define your own type of prefs that you expect, like
export type UserPrefsType = {
theme: string;
devices: number;
nickname: string;
}
And to use custom type, you can const user: Models.User<UserPrefsType>
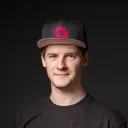
Both approaches are valid, up to you. I personally dont sture too much in prefs, so benefits from type isn't too significant. So I go the easy way of doing any
usually.

Ah

ill rock with that, i do that on other places aswell
Recommended threads
- How to implement Google Authentication a...
Hi, I implemented Appwrite Google Authentication in NextJS v14.1.3 and successfully implemented the sign in feature (on SSR) when trying it in localhost, but wh...
- Bandwidth increasing doubts
Hello , I don't want to disturb you , I just want to get my answers , My question is , I am not using any functions or storage , just using db and users auth ,...
- How to alter attributes in collections
Hi there, unluckily I made avatar as string. I should have make it URL. I forgot. Now my URLs for avatar coming more than 156 char. For hot fix, I need to use l...
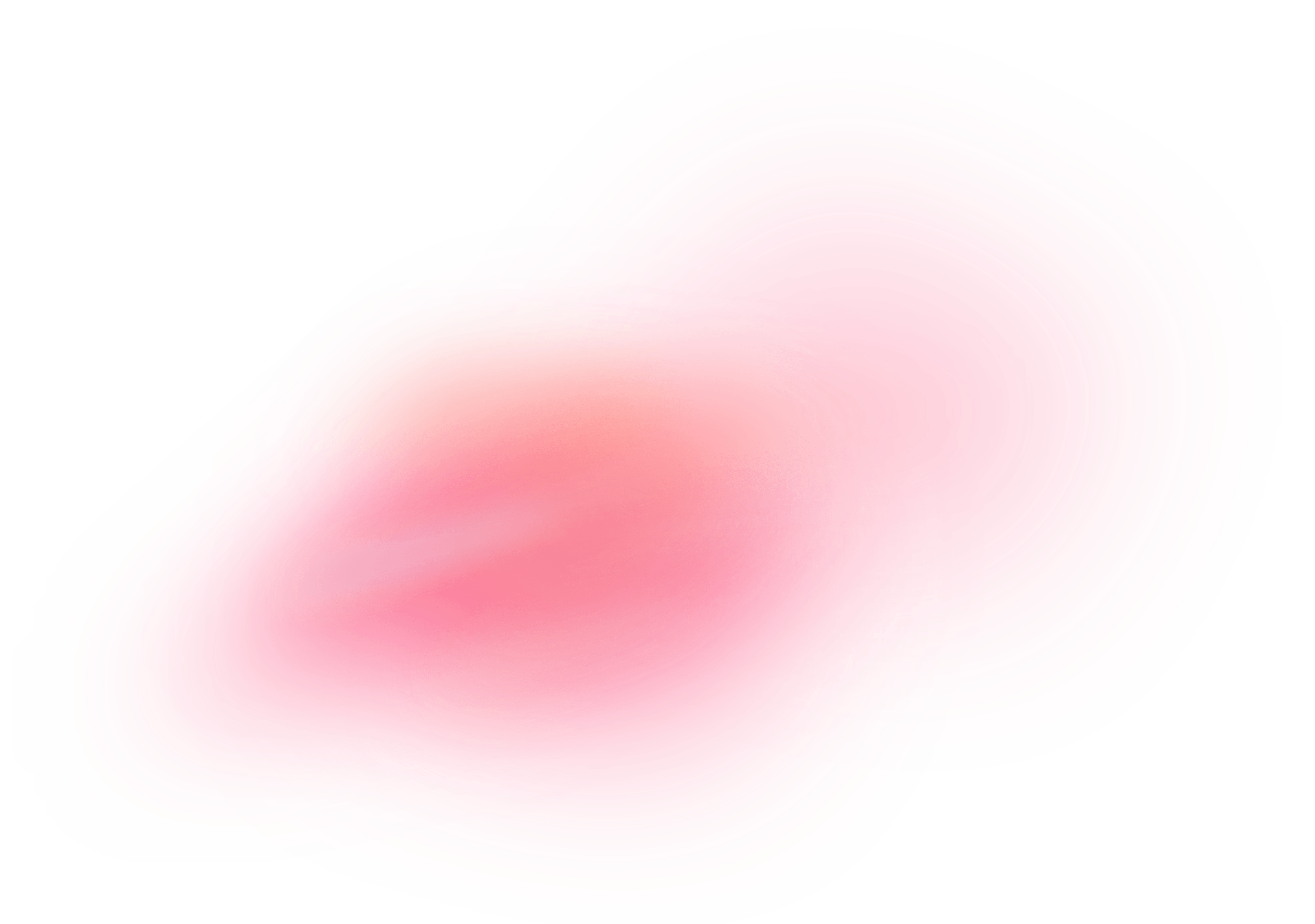