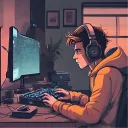
I have made it so my users can log in with Github, and it does work, however when I try and get their details with account.get() I get the error AppwriteException: User (role: guests) missing scope (account)
I tried to do account.getSession('current') but I get the same error. I am using a custom Auth which I will add the code below. I believe the error has something to do with the cookie not being read so it doesn't correctly redirect my user?
import { useNavigate } from "react-router-dom";
import React, { createContext, useContext, useEffect, useState } from "react";
import { IUser } from "@/types";
import { getCurrentUser } from "@/lib/appwrite/api";
import { account } from "@/lib/appwrite/config";
import { OAuthProvider } from "appwrite";
export const INITIAL_USER = {
id: "",
name: "",
username: "",
email: "",
imageUrl: "",
};
const INITIAL_STATE = {
user: INITIAL_USER,
isLoading: false,
isAuthenticated: false,
setUser: () => {},
setIsAuthenticated: () => {},
checkAuthUser: async () => false as boolean,
};
type IContextType = {
user: IUser;
isLoading: boolean;
setUser: React.Dispatch<React.SetStateAction<IUser>>;
isAuthenticated: boolean;
setIsAuthenticated: React.Dispatch<React.SetStateAction<boolean>>;
checkAuthUser: () => Promise<boolean>;
};
const AuthContext = createContext<IContextType>(INITIAL_STATE);
export function AuthProvider({ children }: { children: React.ReactNode }) {
const navigate = useNavigate();
const [user, setUser] = useState<IUser>(INITIAL_USER);
const [isAuthenticated, setIsAuthenticated] = useState(false);
const [isLoading, setIsLoading] = useState(false);
const checkAuthUser = async () => {
setIsLoading(true);
try {
const currentAccount = await getCurrentUser();
if (currentAccount) {
setUser({
id: currentAccount.$id,
name: currentAccount.name,
username: currentAccount.username,
email: currentAccount.email,
imageUrl: currentAccount.imageURL,
});
setIsAuthenticated(true);
return true;
}
return false;
} catch (error) {
console.error(error);
return false;
} finally {
setIsLoading(false);
}
};
const handleGitHubLogin = () => {
account.createOAuth2Session(
OAuthProvider.Github,
"http://localhost:5173",
"http://localhost:5173/sign-in",
["user:email"]
);
};
useEffect(() => {
// const cookieFallback = localStorage.getItem("cookieFallback");
// if (
// cookieFallback === "[]" ||
// cookieFallback === null ||
// cookieFallback === undefined
// ) {
// navigate("/sign-in");
// }
checkAuthUser();
}, [navigate]);
const value = {
user,
setUser,
isLoading,
isAuthenticated,
setIsAuthenticated,
checkAuthUser,
handleGitHubLogin,
};
return <AuthContext.Provider value={value}>{children}</AuthContext.Provider>;
}
export const useUserContext = () => useContext(AuthContext);
Any help would be appreciated.
Recommended threads
- document.update
Invalid relationship value! The data I'm passing is not null and all the rest I'm just updating the document and not the relationship
- Cannot create documents with encrypted a...
Hello. I wonder if nobody actually uses these things, however: If you create an string attribute through the API with encrypt: true (why only the api..?), th...
- Push target always empty on flutter
```dart await account.createPushTarget(targetId: ID.unique(), identifier: "Demo"); ``` After executing that code with an active session in flutter, in the Appw...
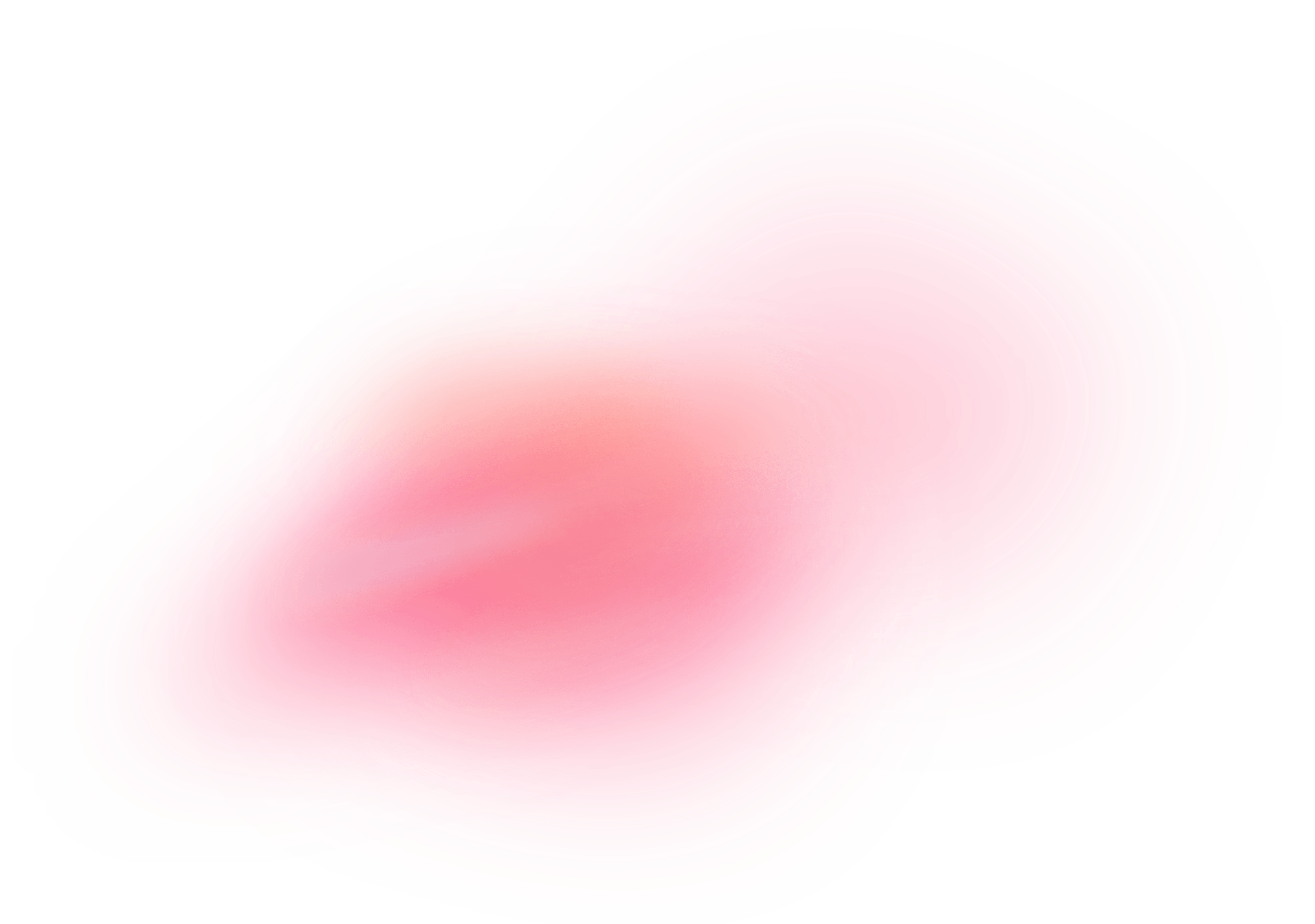