In Appwrite Messaging, you can use topics to deliver messages to groups of users at once.
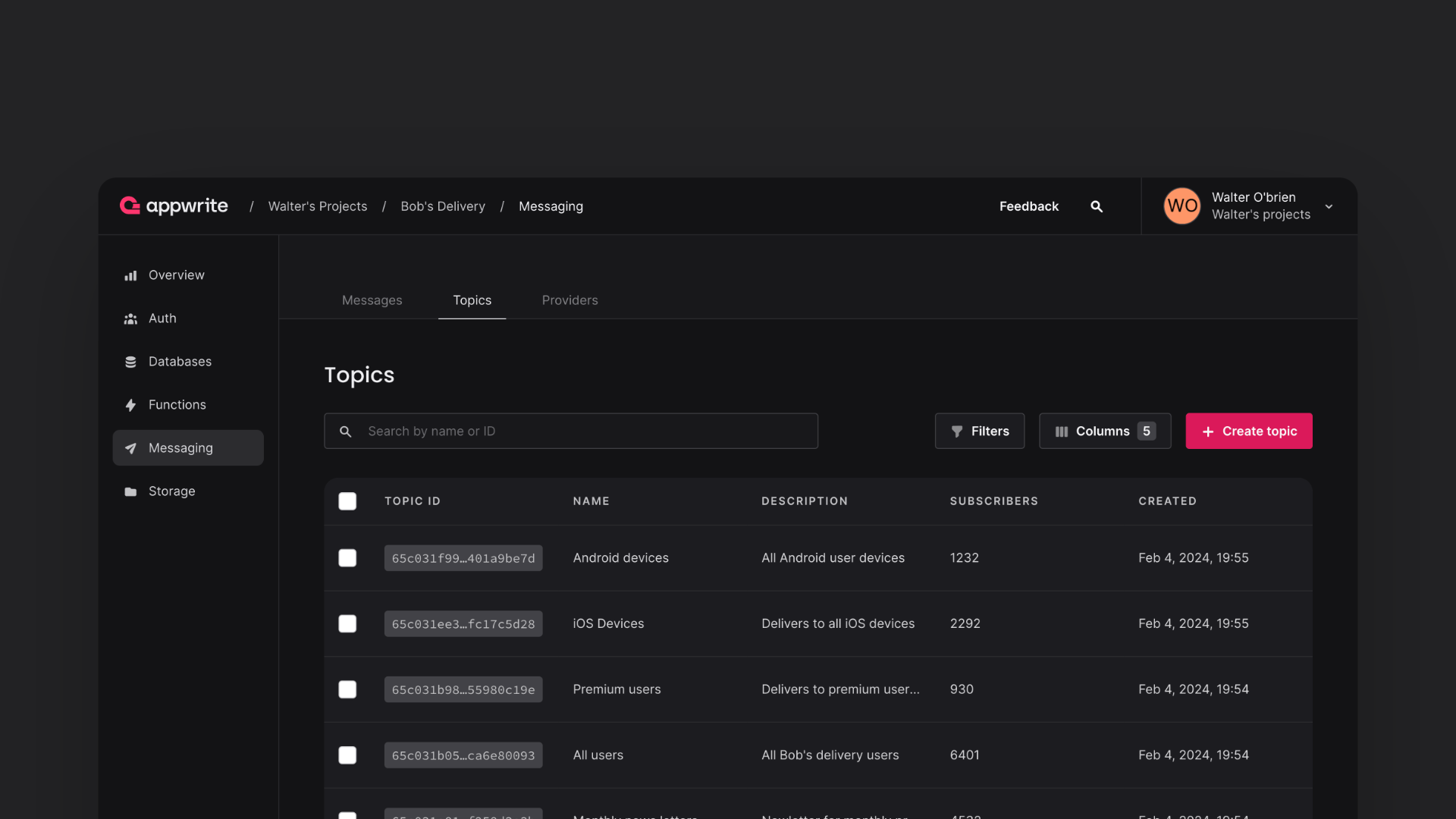
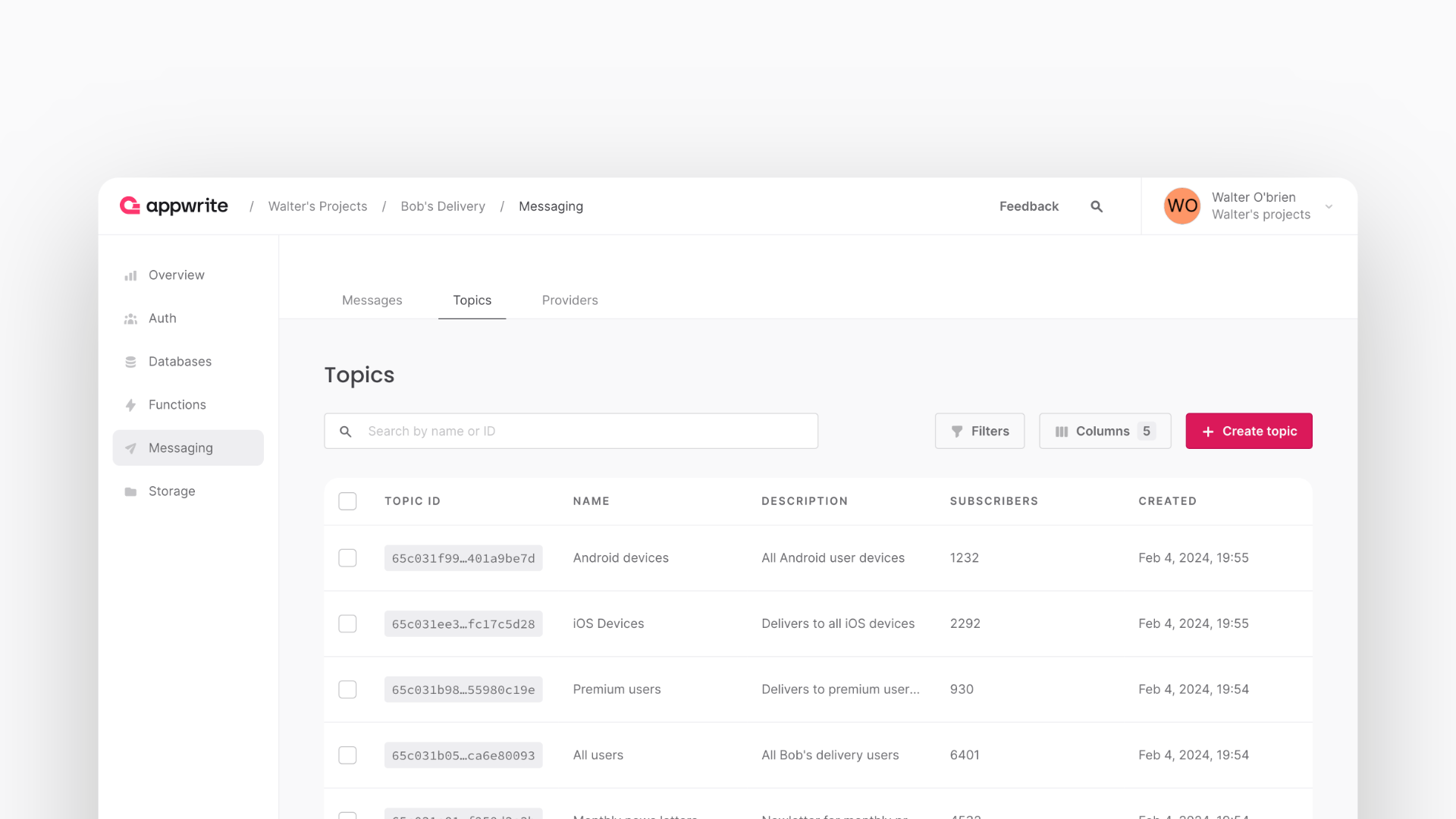
Topics and targets
A user can have multiple targets, such as emails, phone numbers, and devices with your app installed. These targets can subscribe to a topic, so when messages are published to a topic, all subscribed targets receive the message.
Learn more about targets
Organizing topics
A topic should have semantic meaning. For example, a topic can represent a group of customers that receiving a common announcemennt or publishing public updates. It's important to keep privacy in mind when using topics. Prefer sending private information like chat messages by addressing individual targets attached to a user.
Topics are optimized for delivering the same message to large groups of users. If you need to deliver messages to all devices of the same user, you can find a user's targets by calling account.get().
Create a topic
You can create topics
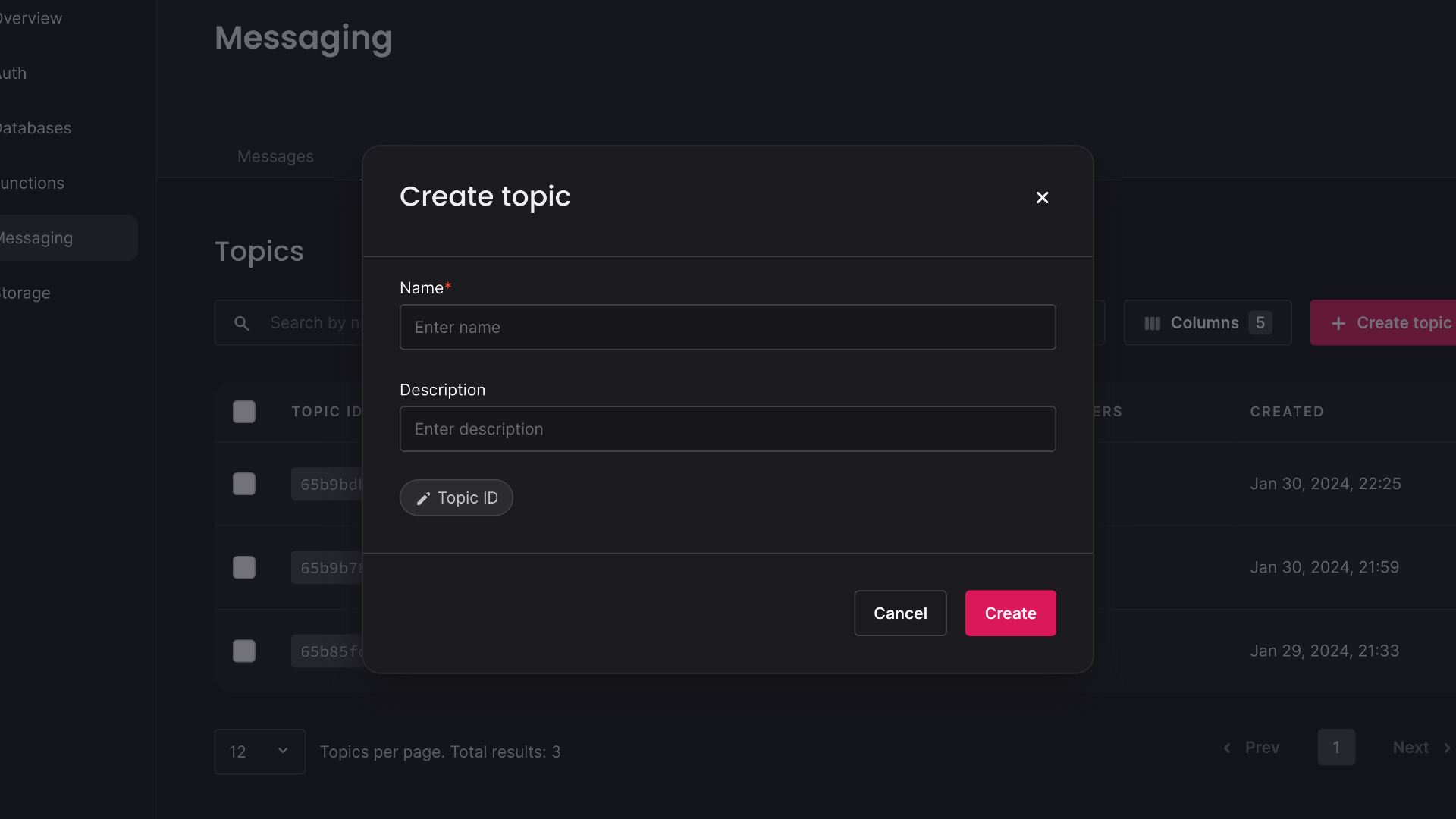
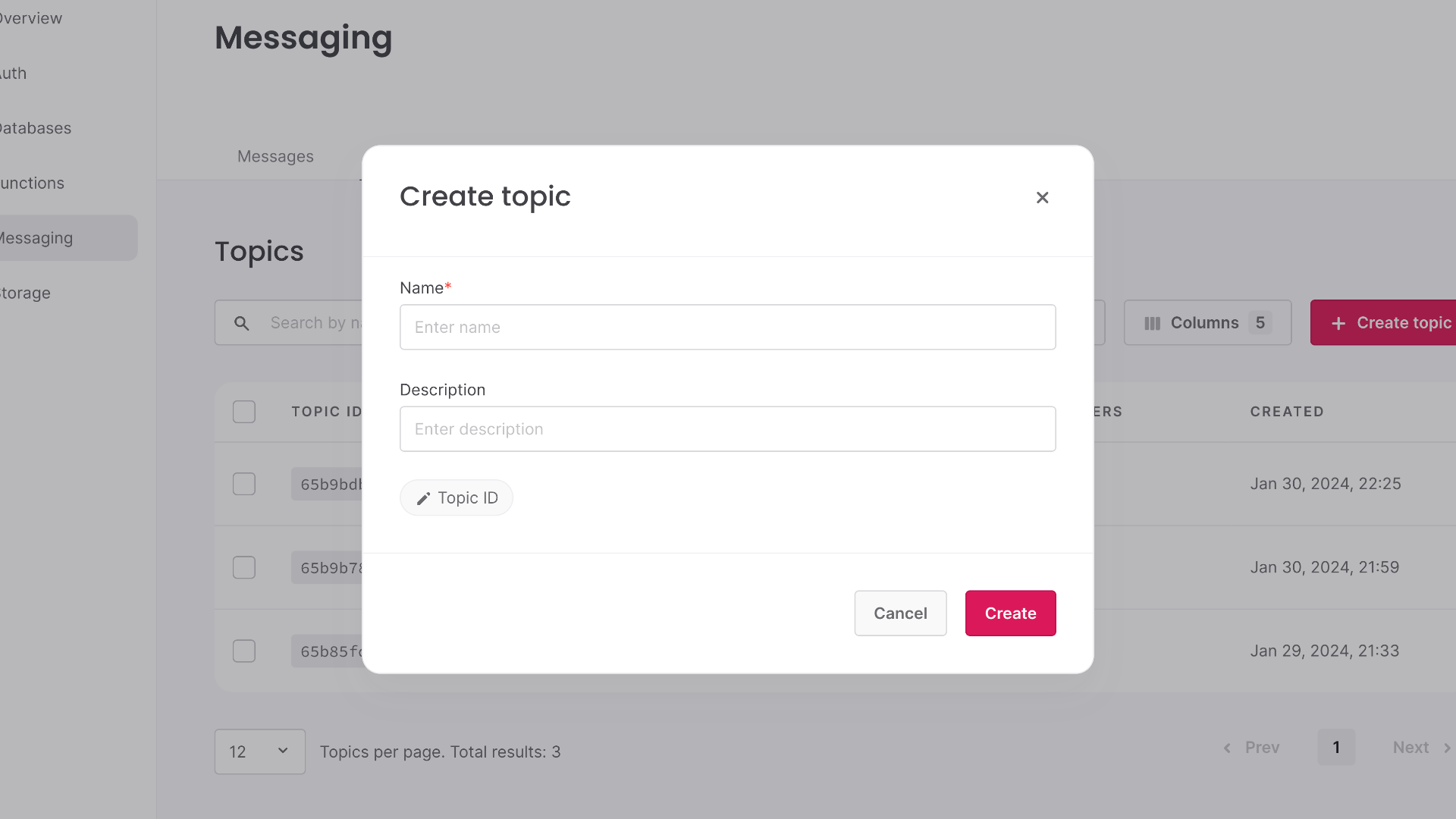
Navigate to your Appwrite Console > Messaging > Topics > Create topic.
Before proceeding
Ensure you install the CLI, log in to your Appwrite account, and initialize your Appwrite project.
You can create a topic using the CLI command appwrite init topics to initialize a topic.
appwrite init topics
You can now push your topics with the following command:
appwrite push topics
This will create your topic in the Console with all of your appwrite.json configurations.
Learn more about the CLI topics commands
Permissions
Before you can subscribe to a topic, a user needs the appropriate permission. You can set permission by navigating to Messaging > Topics > select a topic to configure > Subscription access.
Learn more about permission roles
Subscribe targets to a topic
During development, you can subscribe targets to a topic for testing right in the Appwrite console.
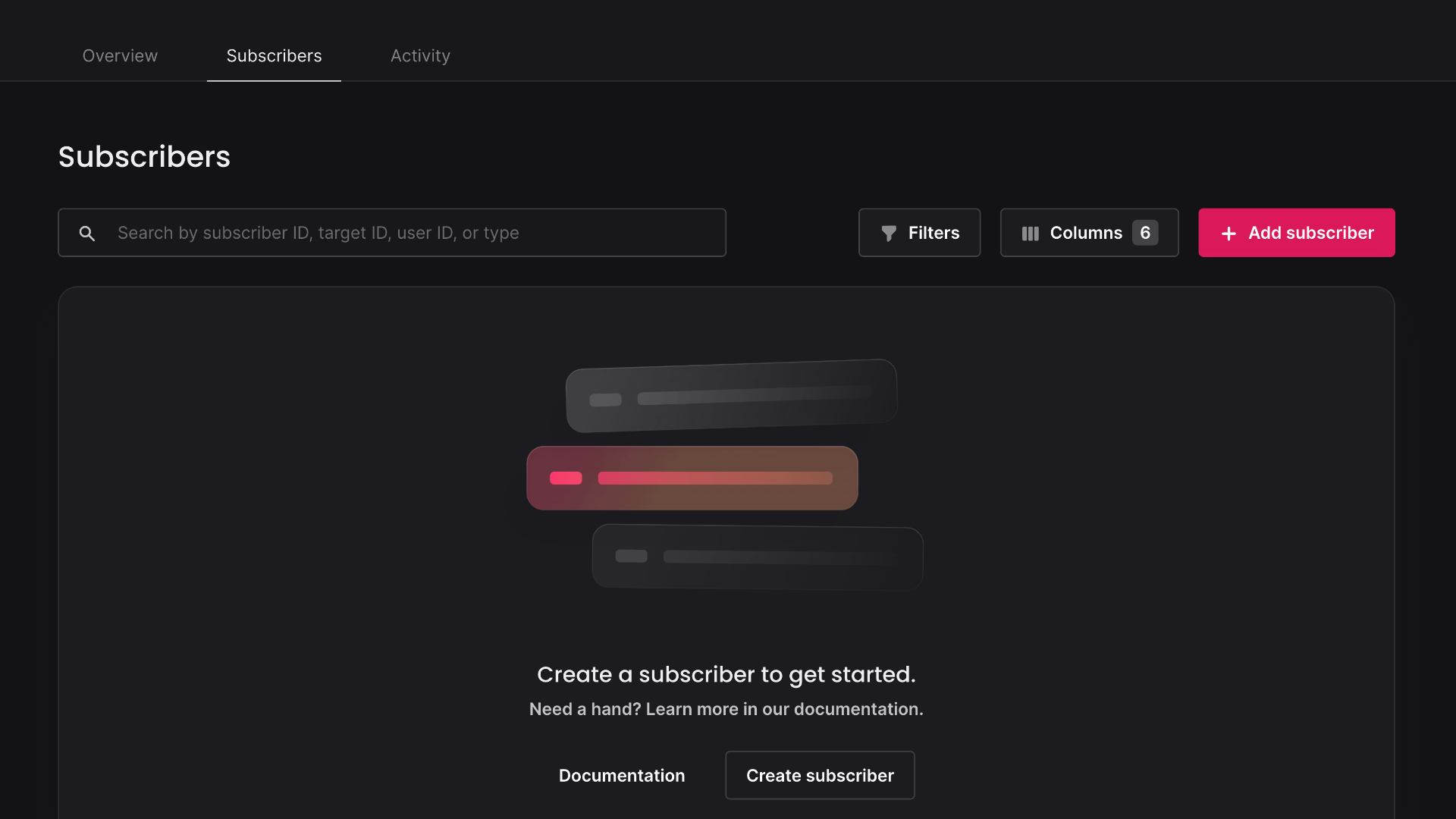
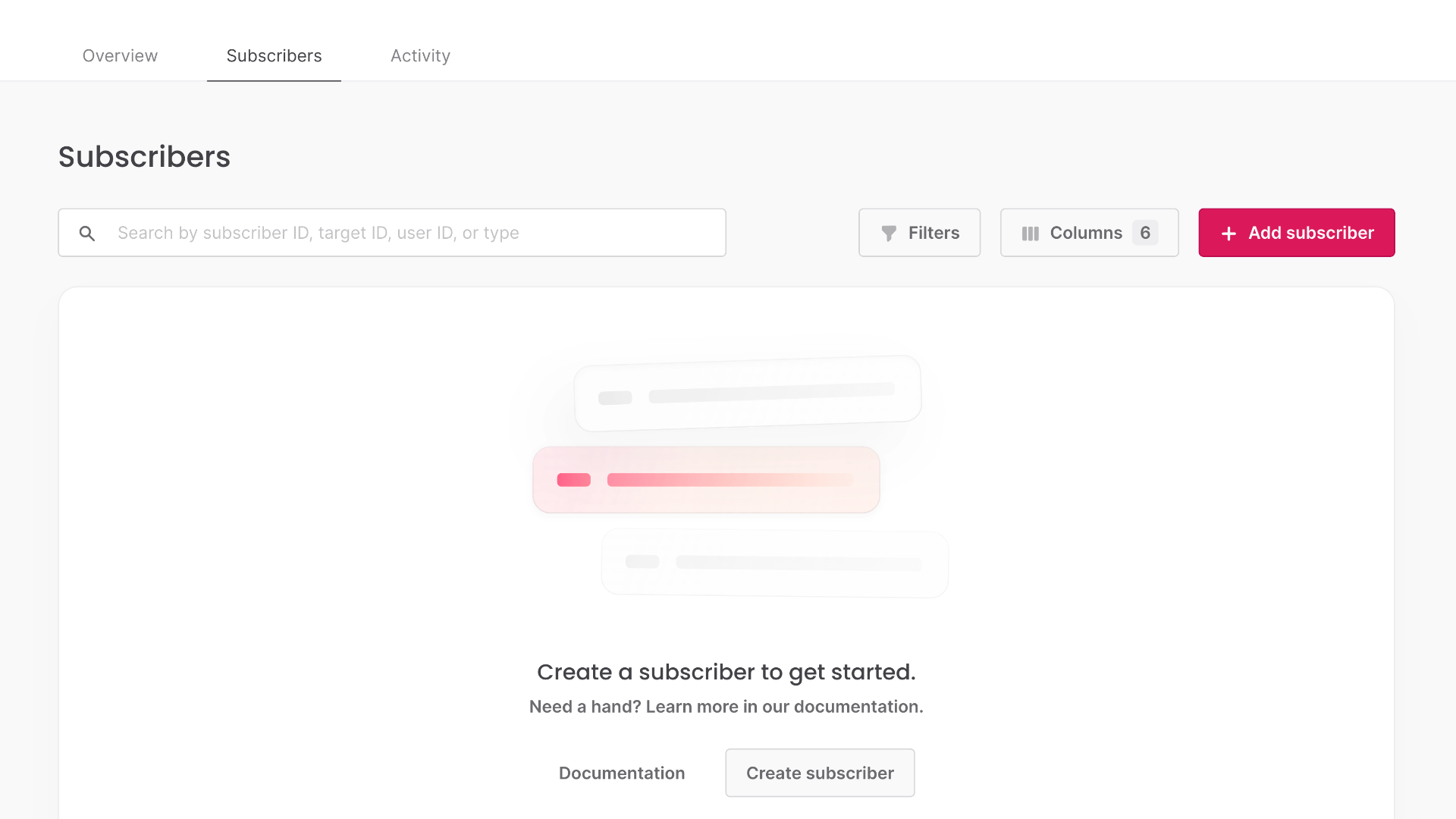
Navigate to your Appwrite Console > Messaging > Topics > click on your topic > Subscribers > Create topic > Add subscriber.
If you can't find the targets you'd like to add, see the targets page.