You can send custom email messages to your app's users using Appwrite Messaging and a connected SMTP service. This guide takes you through the implementation path of adding email messaging to your app.
Add a provider
Appwrite supports Mailgun and Sendgrid as SMTP providers. You must configure one of them as a provider.
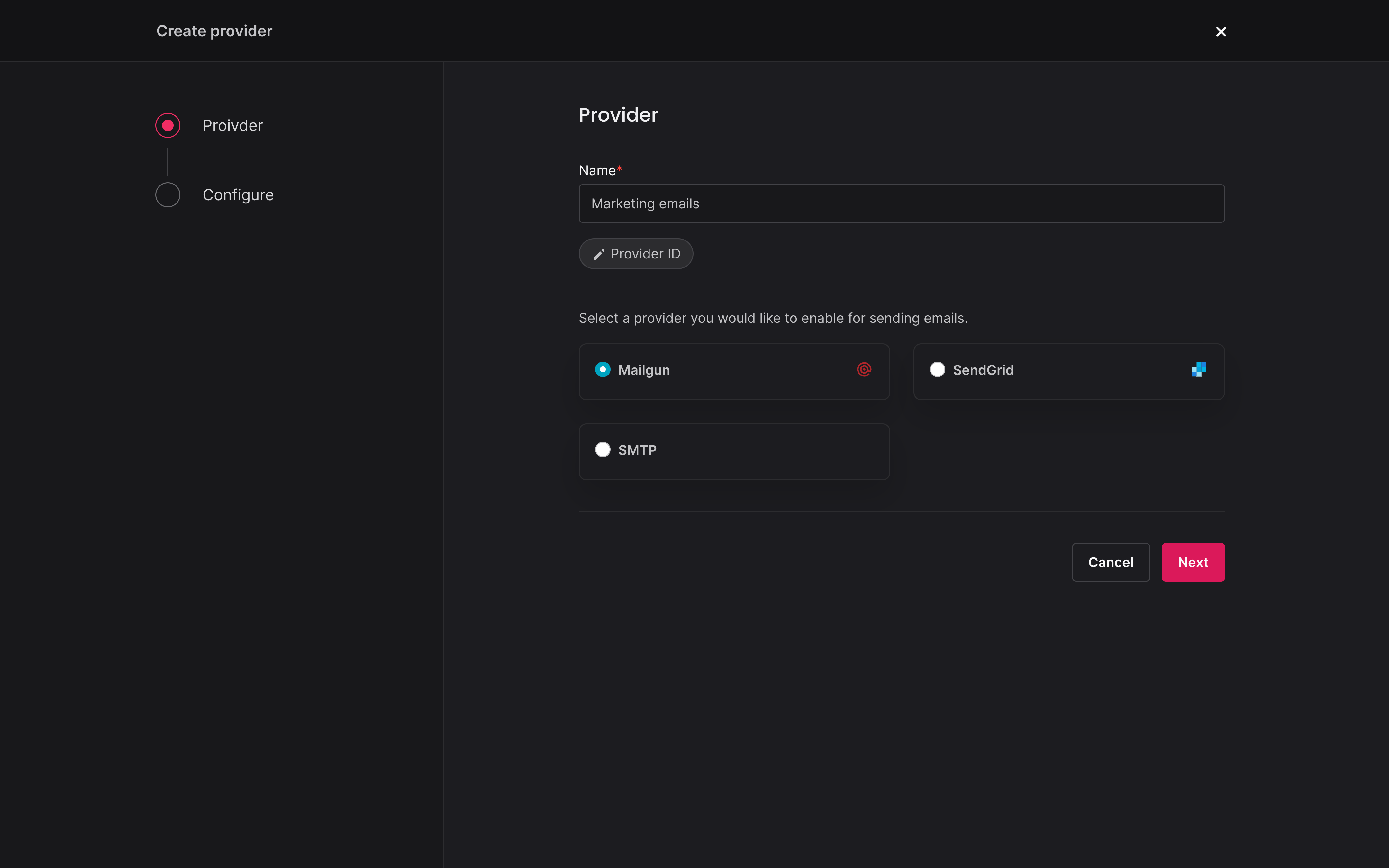
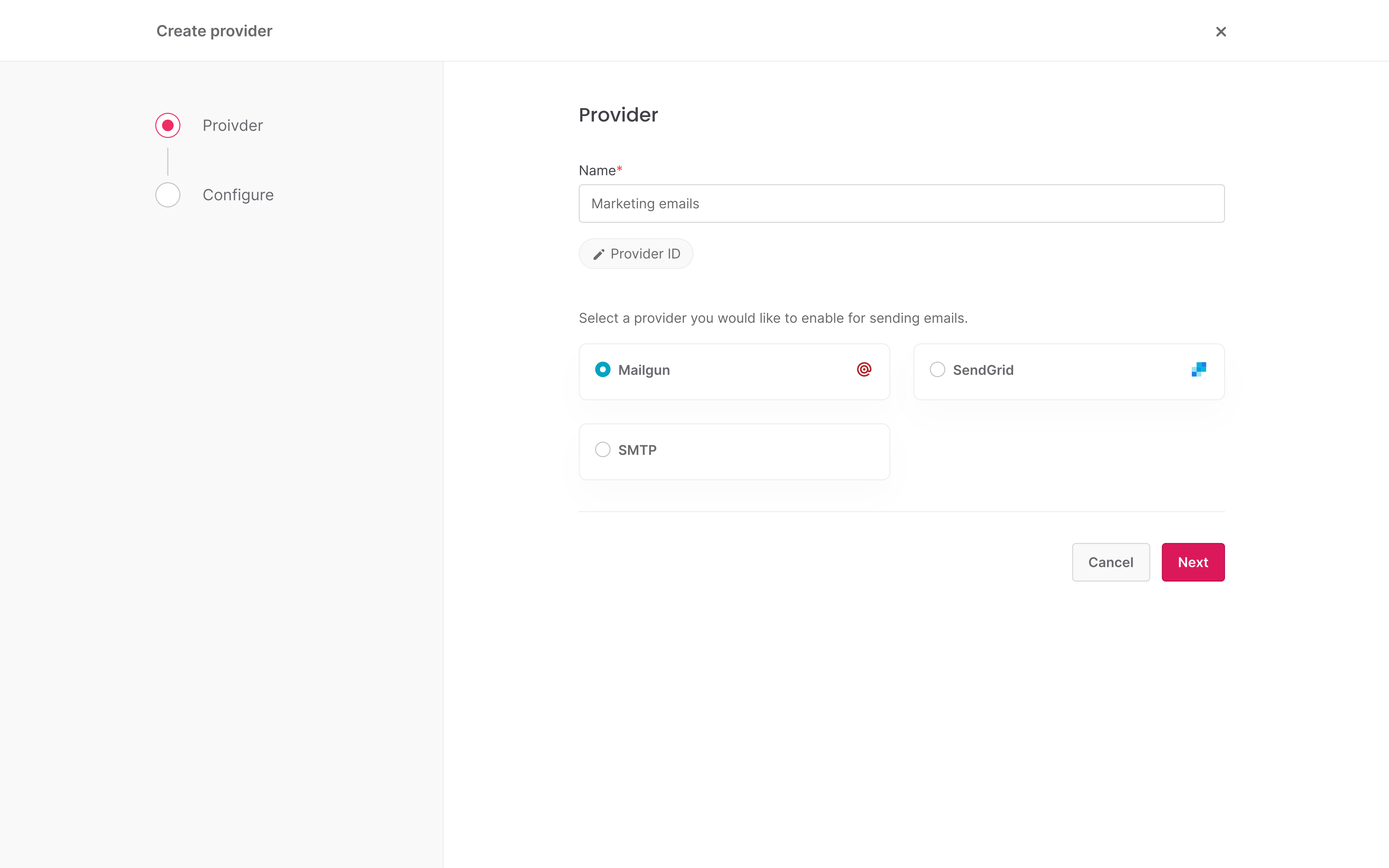
To add a new provider navigate to Messaging > Providers > Add provider > Email and follow the wizard. You can find more details about configuring in the provider guides for Mailgun and Sendgrid.
Add targets
In Appwrite Messaging, each user has targets like their email, phone number, and devices with your app installed. You can deliver messages to users through their targets.
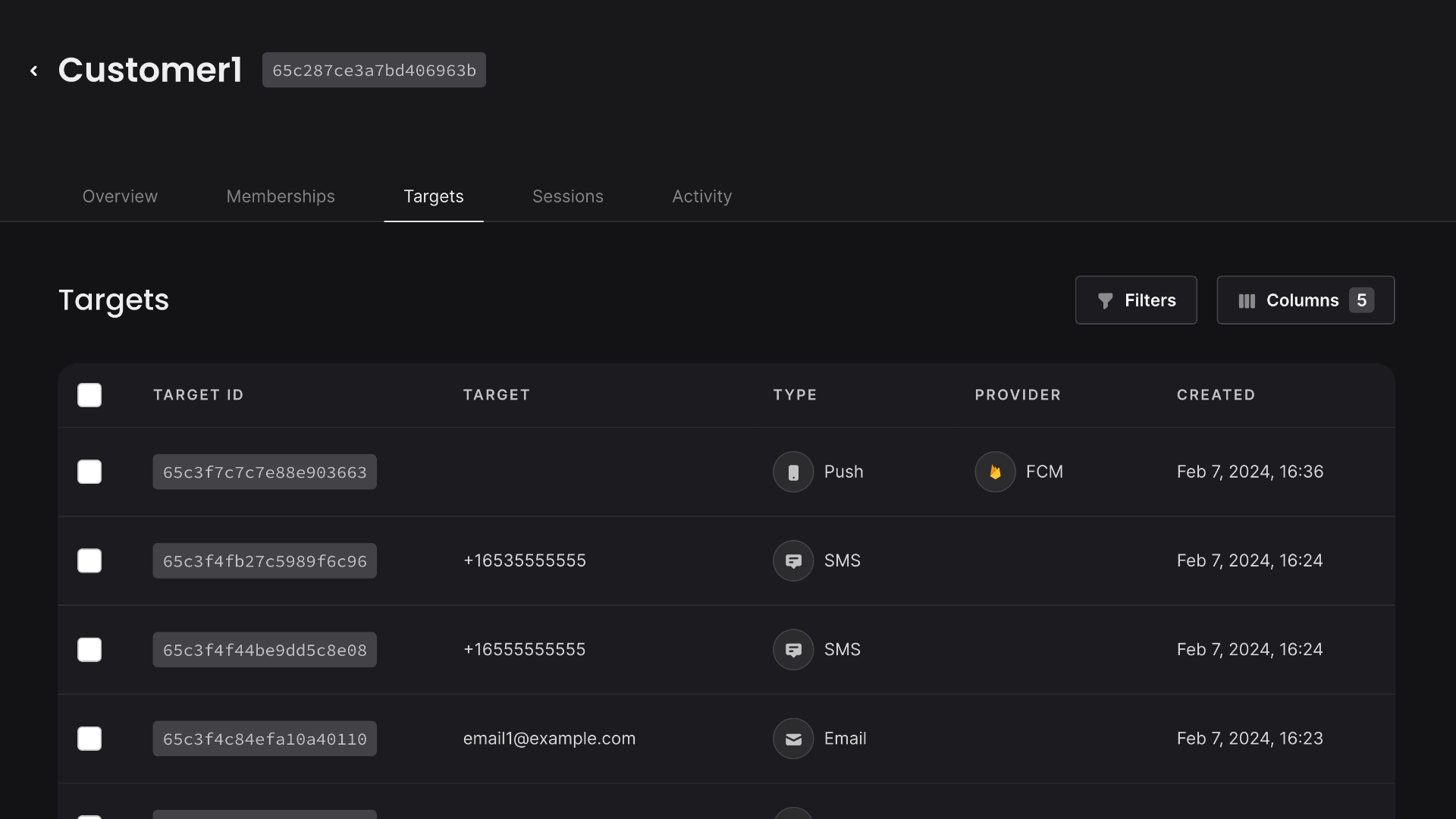
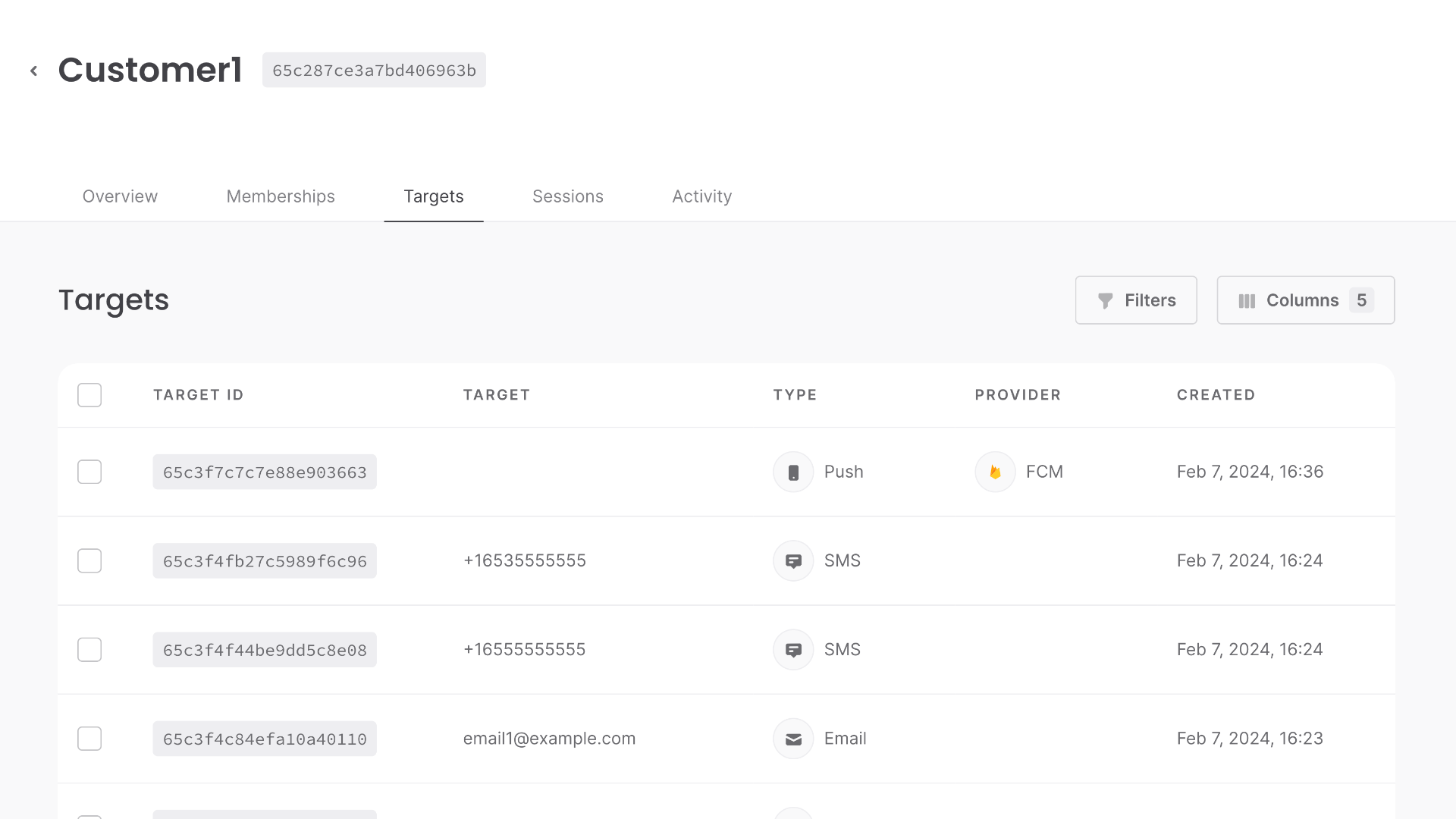
If the user signed up with email and password, their account would already have email as a target. During development, you can add targets to existing accounts by navigating to Authentication > Users > Select a user > Targets > Add a subscriber.
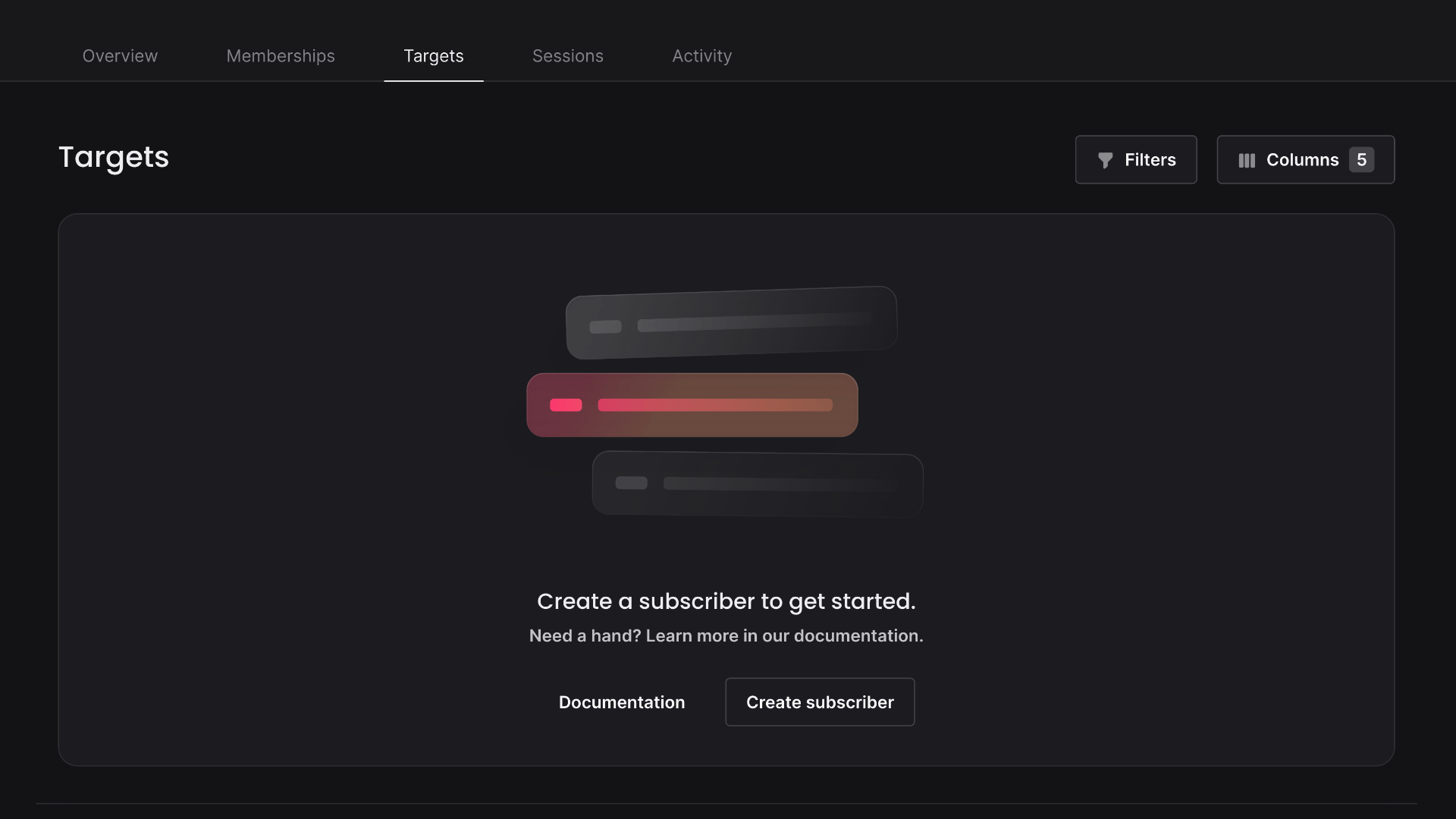
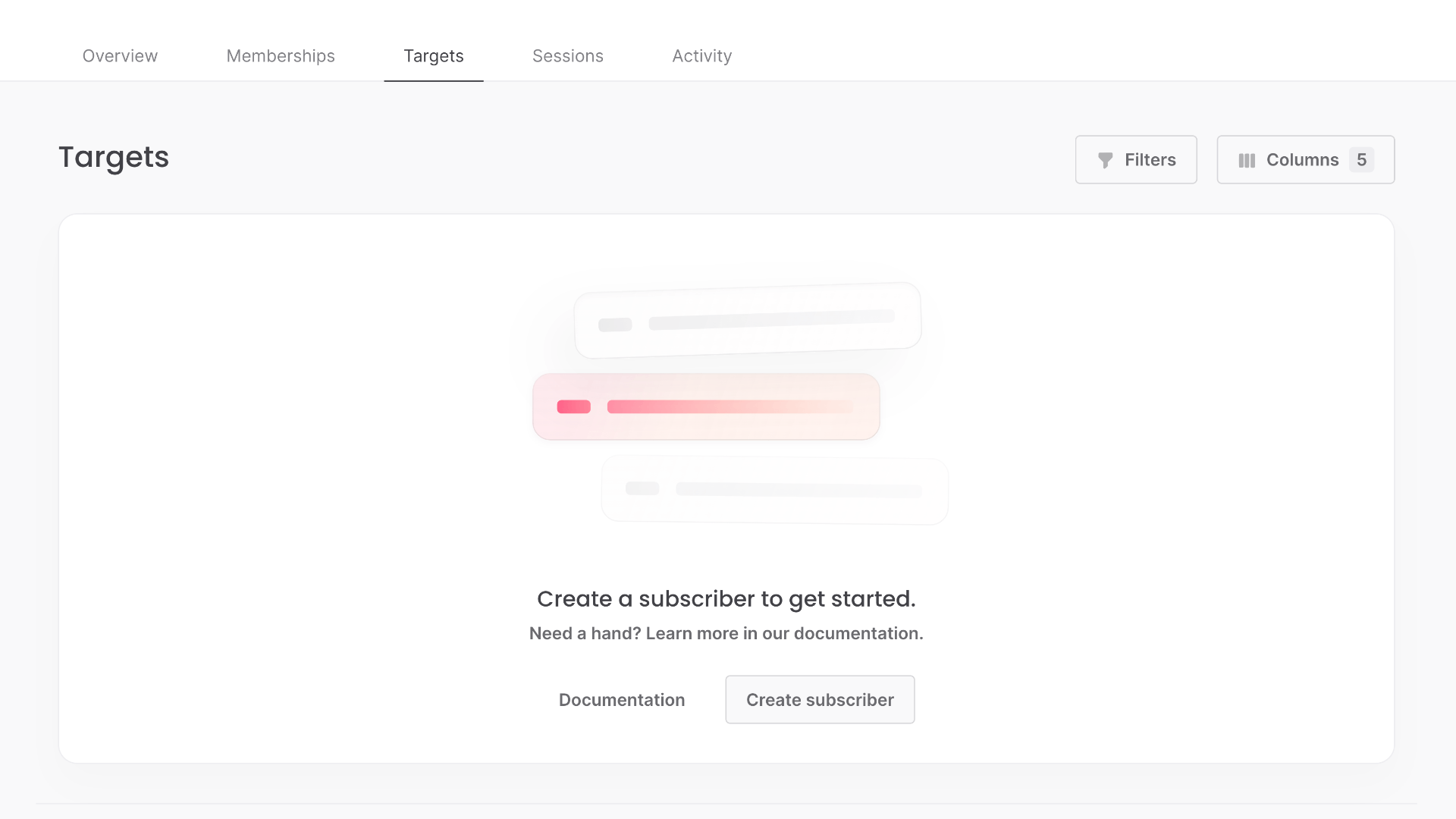
You can also implement forms in your app to collect contact information and add it as a target with the createTarget endpoint.
Create topics (optional)
You can use topics to organize targets that should receive the same messages, so you can send emails to groups of targets instead of one at time. This step is optional if you plan to only send emails to individual targets.
To create a topic in the Appwrite Console, navigate to Messaging > Topics > Create topic.
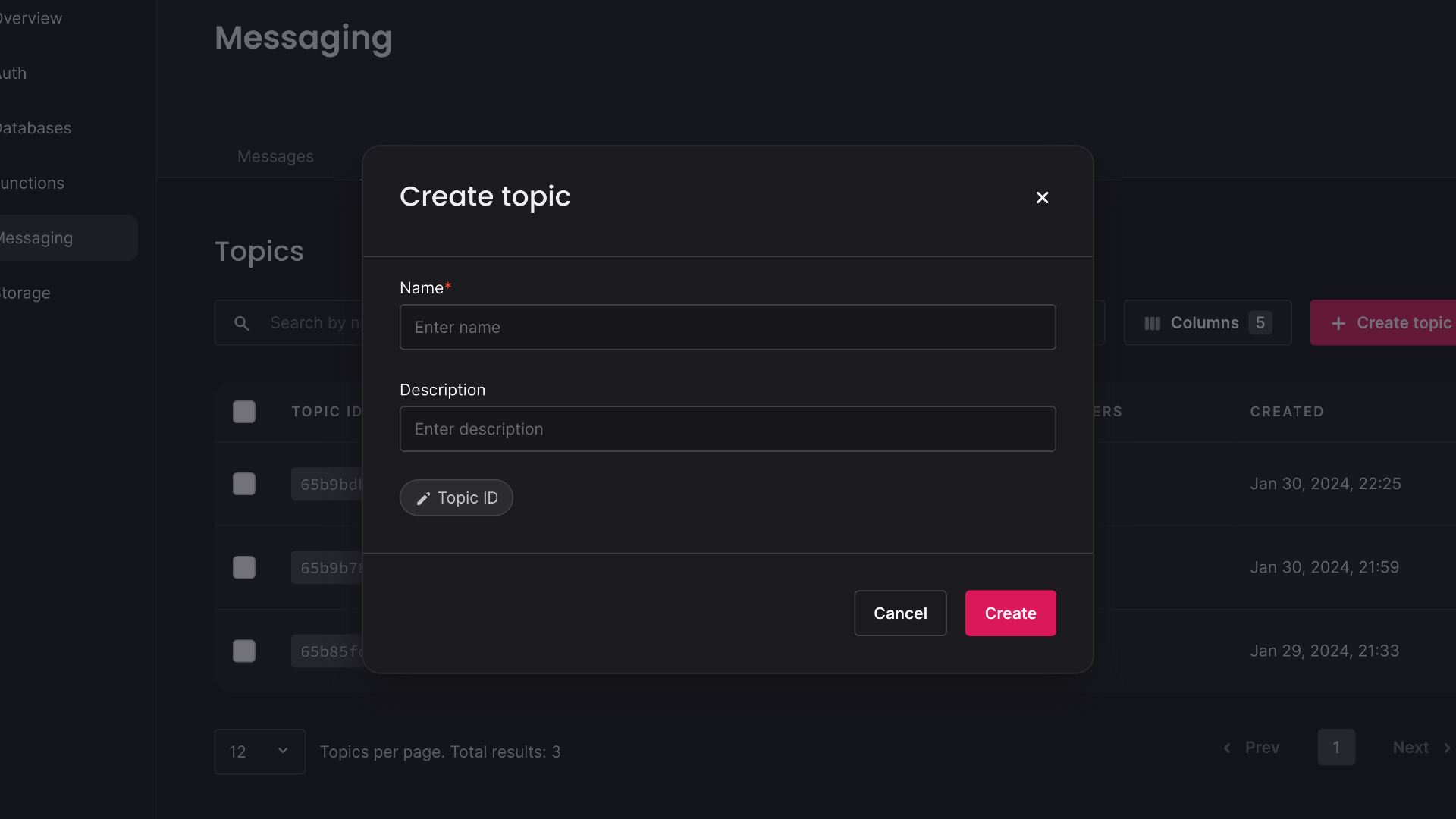
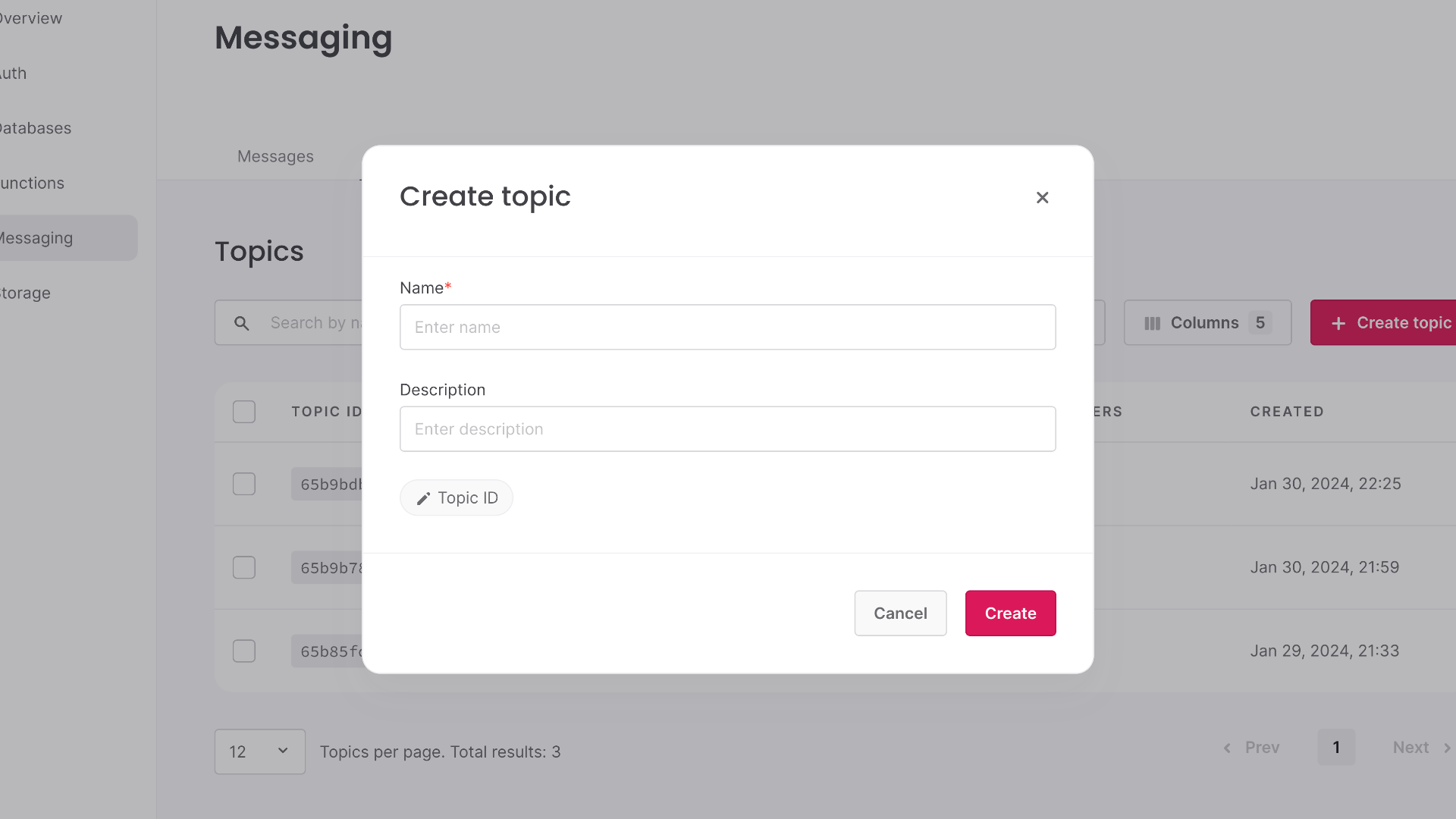
You can also create topics programmatically using an Appwrite Server SDK.
Send emails
You can send emails using a Server SDK. To send an email immediately, you can call the createEmail endpoint with schedule left empty.
Schedule emails
To send a scheduled email, you can call the createEmail endpoint with status set to 'scheduled' and schedule as a ISO 8601 date time string for the scheduled time.