Create collection
In Appwrite, data is stored as a collection of documents. Create a collection inside a database in the Appwrite Console to store our ideas.
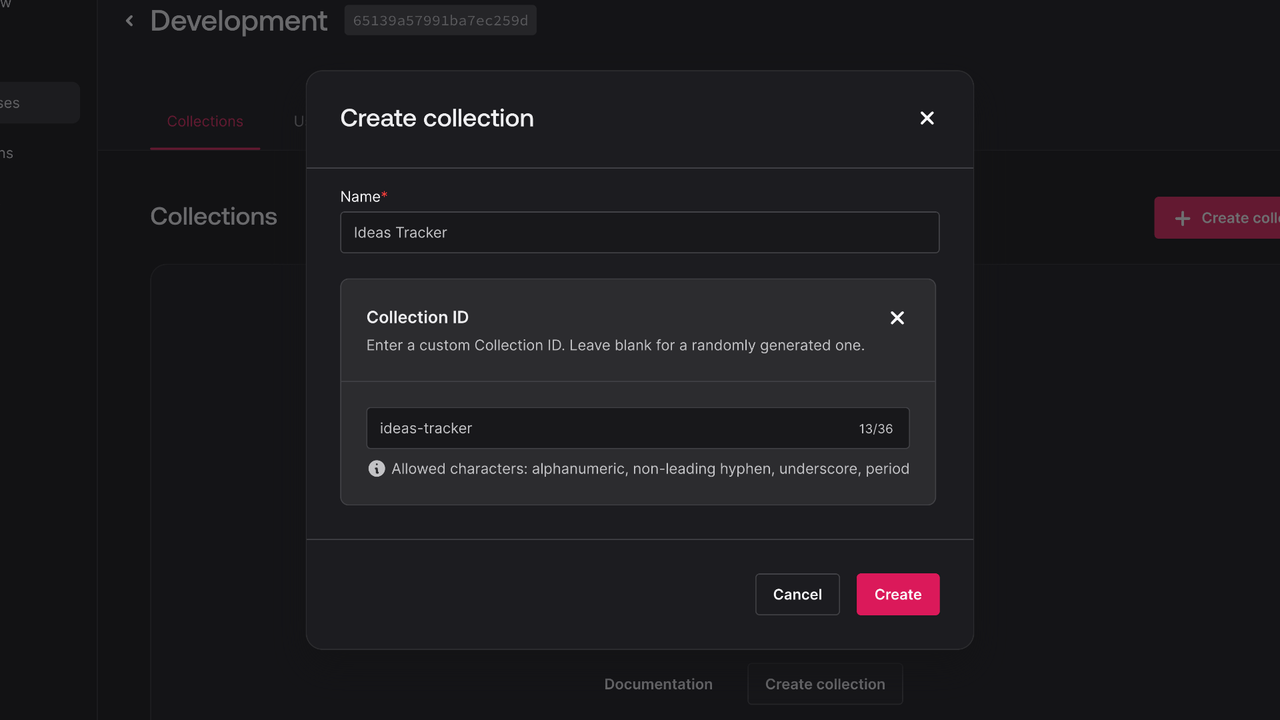
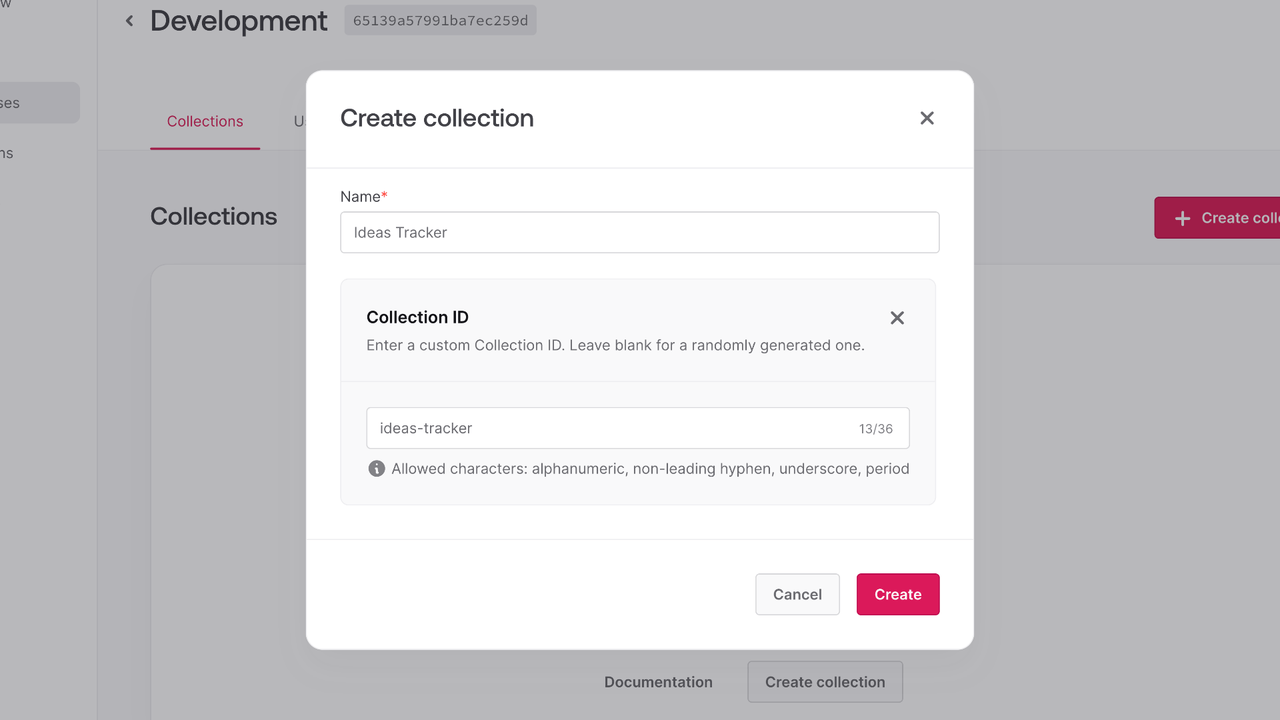
Create the following attributes within the collection:
Field | Type | Size | Required |
userId | String | 36 | Yes |
title | String | 128 | Yes |
description | String | 1024 | No |
For this tutorial, we'll also set the permissions to allow any users to read and write to the collection. In a real-world scenario, you would probably want to restrict access to a certain group of users.
Managing ideas
Now that you have a collection to hold ideas, we can read and write to it from our app.
Let's create some methods to manage ideas in our app.
Create a new file src/lib/ideas.js and add the following code:
Web
import { ID, Query } from 'appwrite';
import { databases } from '$lib/appwrite';
const IDEAS_DATABASE_ID = '<YOUR_DATABASE_ID>'; // Replace with your database ID
const IDEAS_COLLECTION_ID = '<YOUR_COLLECTION_ID>'; // Replace with your collection ID
export async function getIdeas() {
return await databases.listDocuments(
IDEAS_DATABASE_ID,
IDEAS_COLLECTION_ID,
// Use a query to show the latest ideas first
[Query.orderDesc('$createdAt')]
);
}
export async function addIdea(userId, title, description) {
await databases.createDocument(IDEAS_DATABASE_ID, IDEAS_COLLECTION_ID, ID.unique(), {
userId,
title,
description
});
}
export async function deleteIdea(id) {
await databases.deleteDocument(IDEAS_DATABASE_ID, IDEAS_COLLECTION_ID, id);
}
Remember to use a store to hold data returned from Appwrite Databases, so your components can be updated when the data changes.