Create database
To store your ideas, you need to create a database first.
Go to the Databases section in your Appwrite Console
Click Create Database
Give it a name and ID. For this tutorial, we'll use Ideas Tracker as the name and ideas-tracker as the ID.
You'll need to remember the database ID as you'll need it later.
Create collection
In Appwrite, data is stored as a collection of documents. Create a collection in the Appwrite Console to store our ideas.
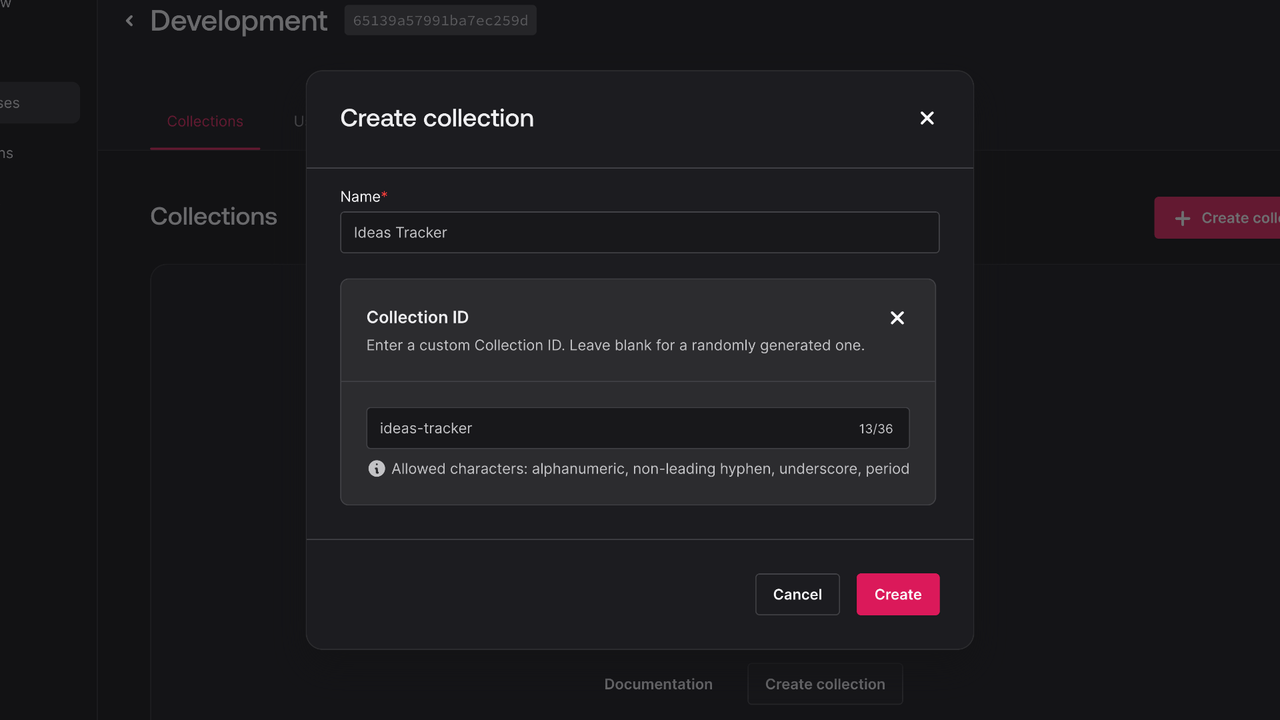
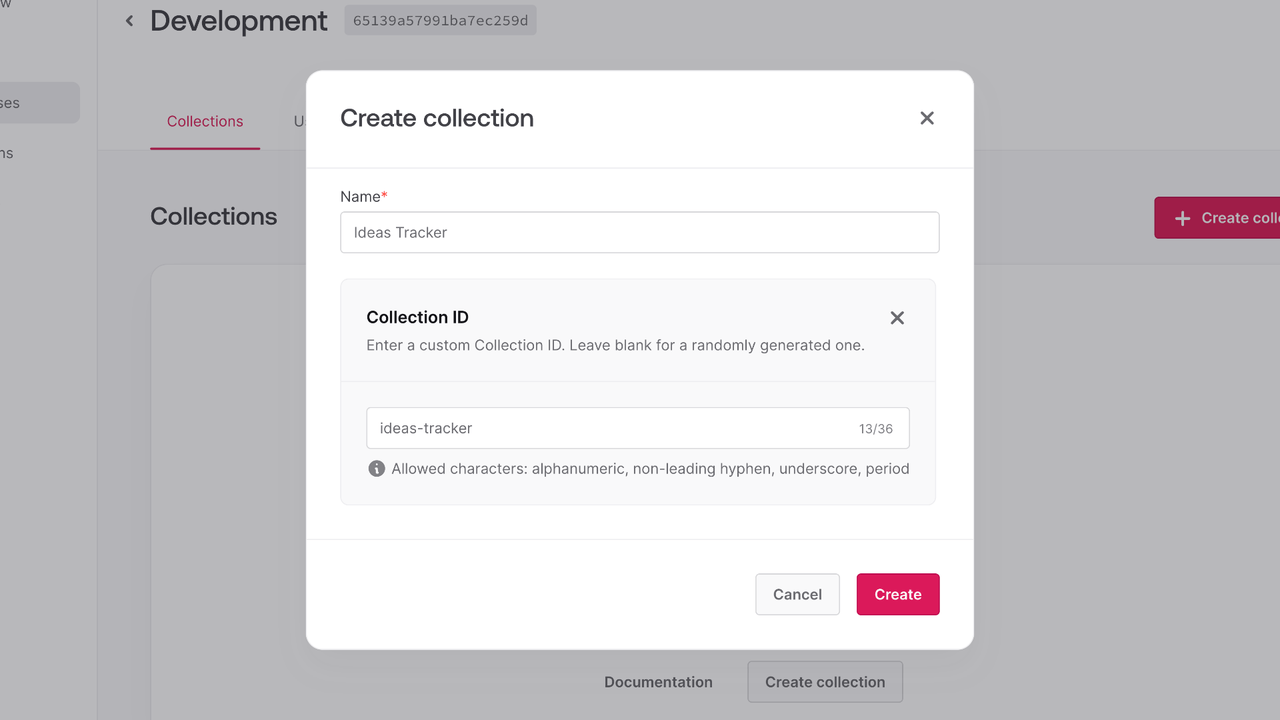
Attributes
Create a new collection with the following attributes:
Field | Type | Required | Size |
userId | String | Yes | 50 |
title | String | Yes | 25 |
description | String | No | 100 |
Configure permissions
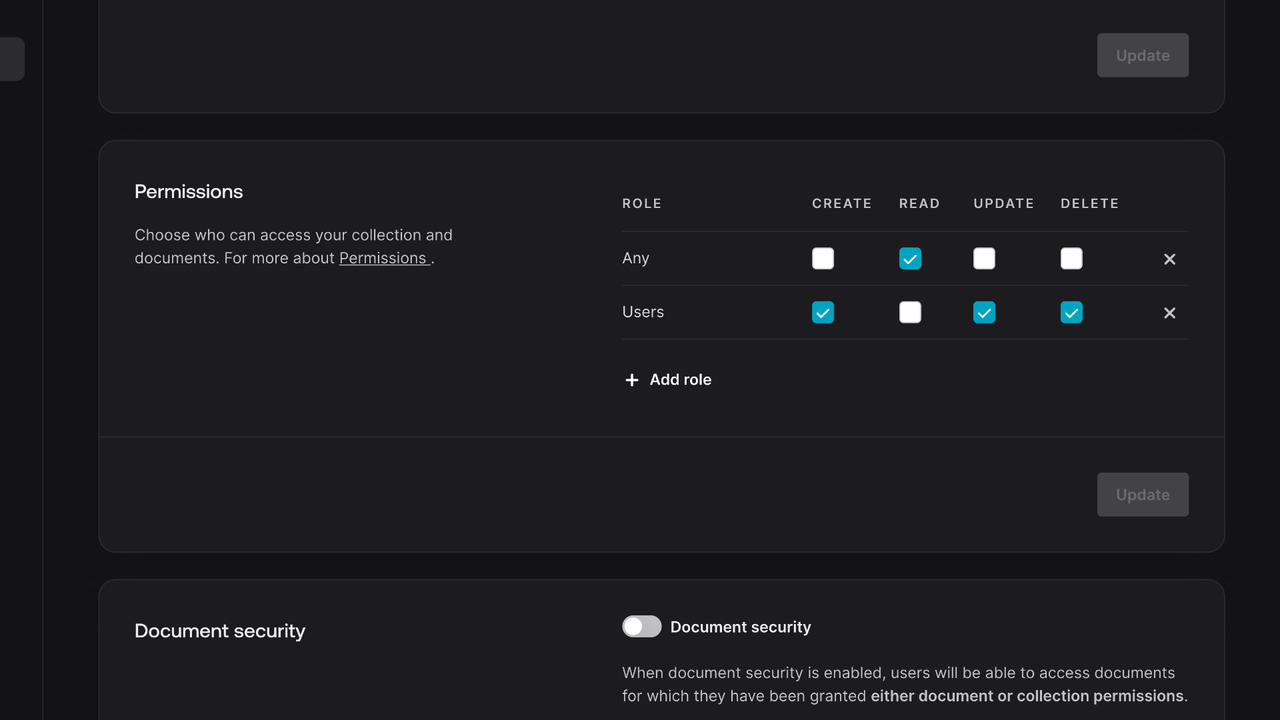
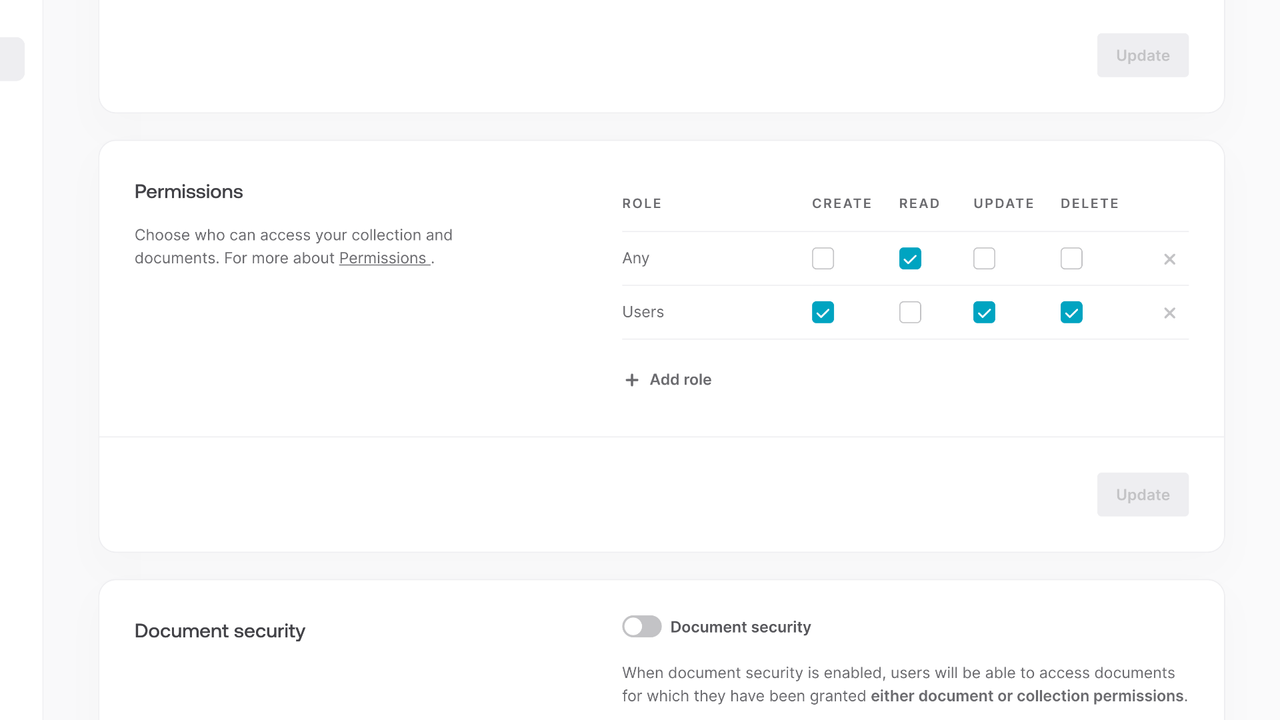
Navigate to the Settings tab of your collection, add the role Any and check the Read box. Next, add a Users role and give them access to Create by checking those boxes. These permissions apply to all documents in your new collection.
Ideas context
Now that you have a collection to hold ideas, we can read and write to it from our app. Like we did with the user data, we will create a React context to hold our ideas. Create a new file src/lib/context/ideas.jsx and add the following code to it.
import { createContext, useContext, useEffect, useState } from "react";
import { databases } from "../appwrite";
import { ID, Query } from "appwrite";
export const IDEAS_DATABASE_ID = "<YOUR_DATABASE_ID>"; // Replace with your database ID
export const IDEAS_COLLECTION_ID = "<YOUR_COLLECTION_ID>"; // Replace with your collection ID
const IdeasContext = createContext();
export function useIdeas() {
return useContext(IdeasContext);
}
export function IdeasProvider(props) {
const [ideas, setIdeas] = useState([]);
async function add(idea) {
try {
const response = await databases.createDocument(
IDEAS_DATABASE_ID,
IDEAS_COLLECTION_ID,
ID.unique(),
idea
);
setIdeas((ideas) => [response, ...ideas].slice(0, 10));
} catch (err) {
console.log(err) // handle error or show user a message
}
}
async function remove(id) {
try {
await databases.deleteDocument(IDEAS_DATABASE_ID, IDEAS_COLLECTION_ID, id);
setIdeas((ideas) => ideas.filter((idea) => idea.$id !== id));
await init();
} catch (err) {
console.log(err)
}
}
async function init() {
try {
const response = await databases.listDocuments(
IDEAS_DATABASE_ID,
IDEAS_COLLECTION_ID,
[Query.orderDesc("$createdAt"), Query.limit(10)]
);
setIdeas(response.documents);
} catch (err) {
console.log(err)
}
}
useEffect(() => {
init();
}, []);
return (
<IdeasContext.Provider value={{ current: ideas, add, remove }}>
{props.children}
</IdeasContext.Provider>
);
}