Learn how to setup your first Python project powered by Appwrite.
Create project
Head to the Appwrite Console.
If this is your first time using Appwrite, create an account and create your first project.
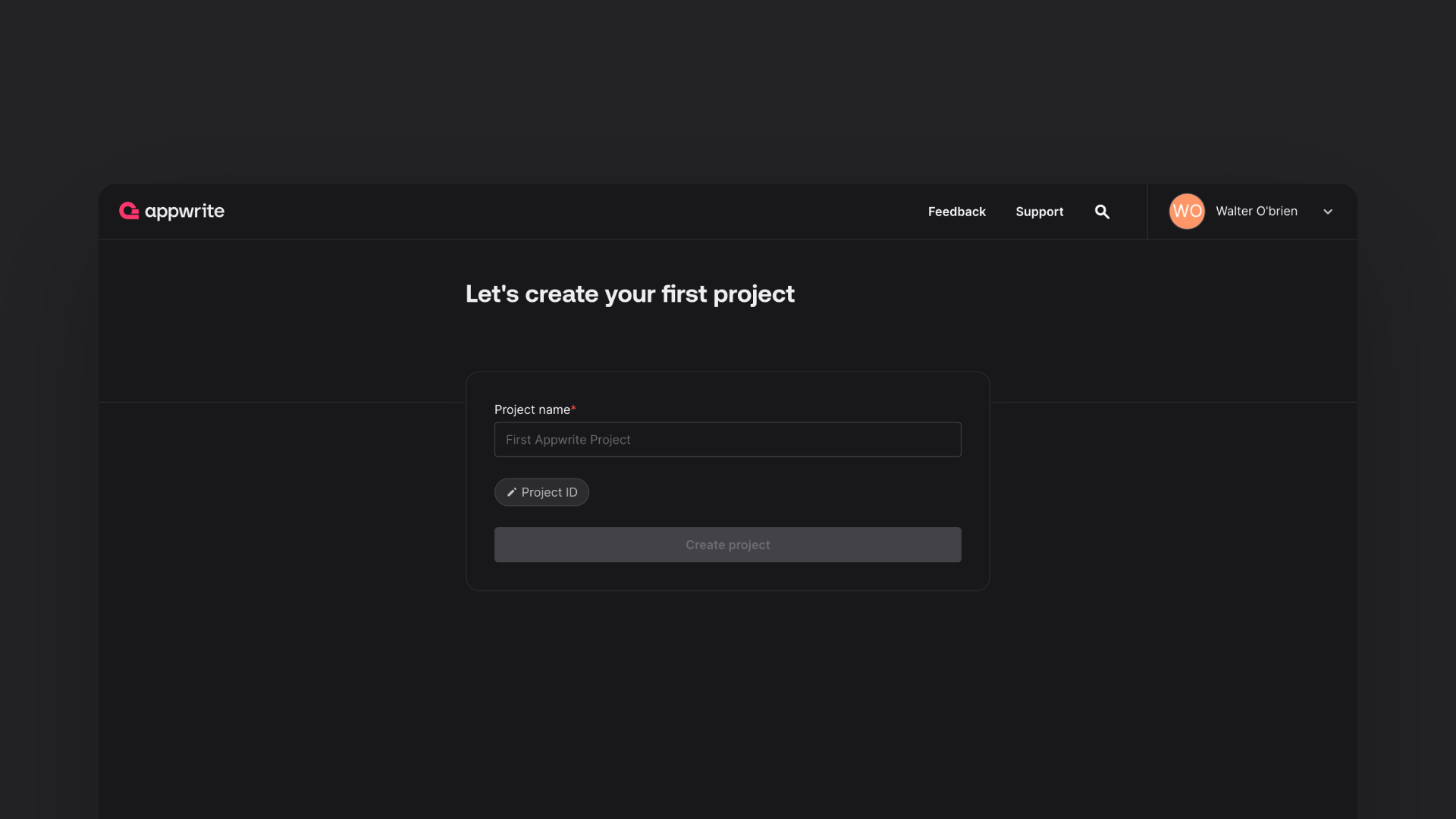
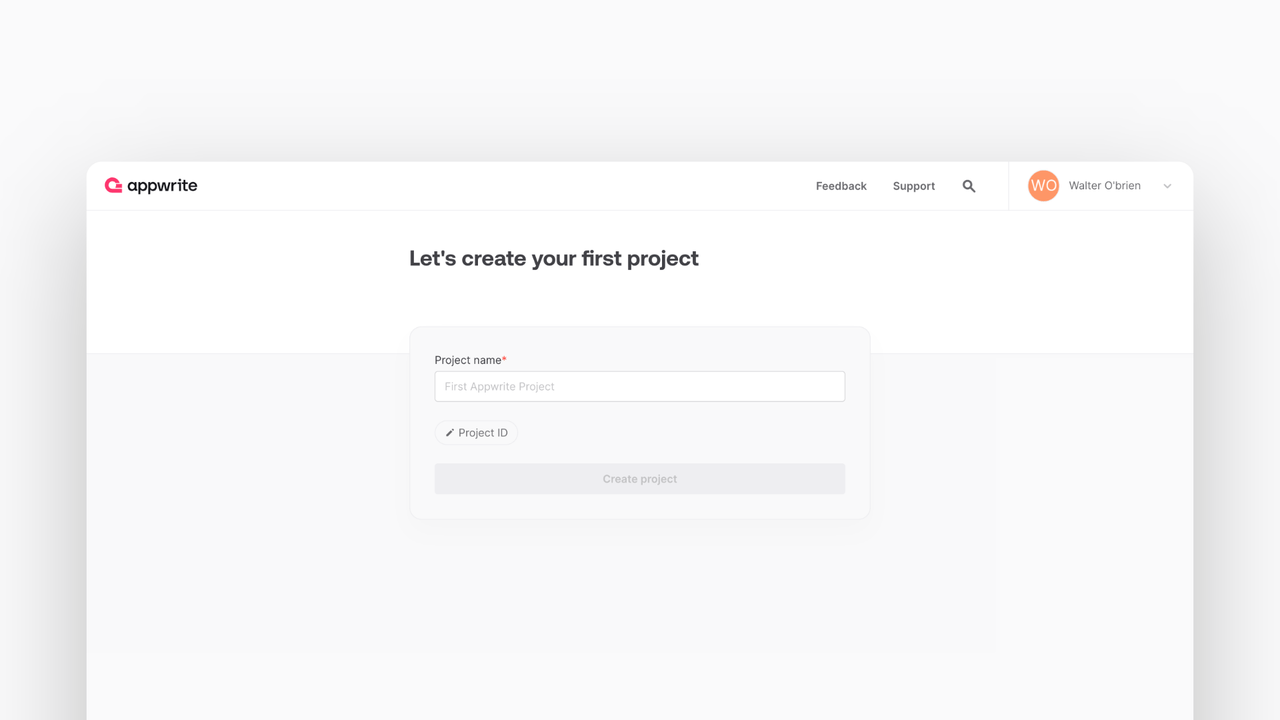
Then, under Integrate with your server, add an API Key with the following scopes.
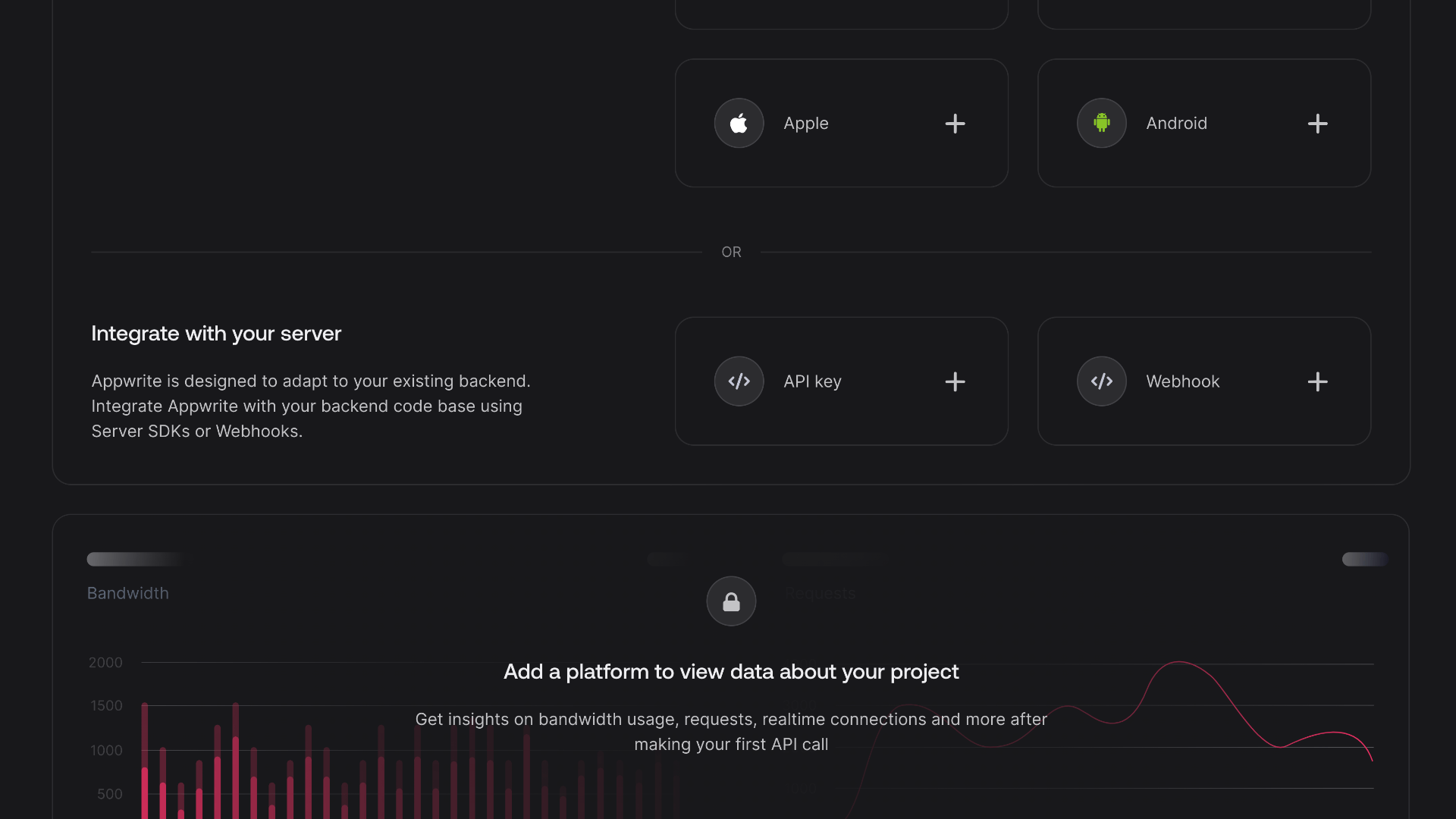
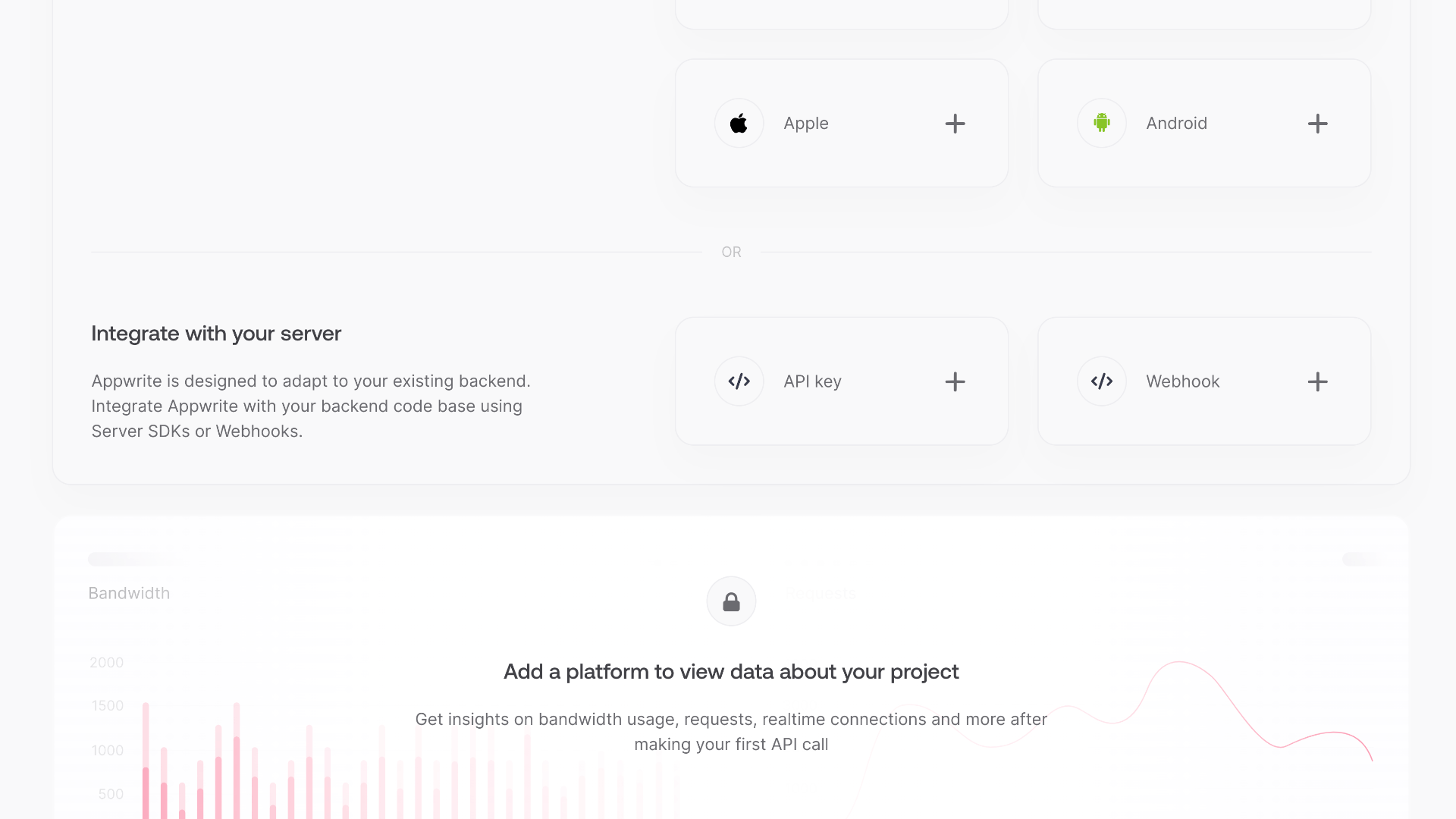
Category | Required scopes | Purpose |
Database | databases.write | Allows API key to create, update, and delete databases. |
collections.write | Allows API key to create, update, and delete collections. | |
attributes.write | Allows API key to create, update, and delete attributes. | |
documents.read | Allows API key to read documents. | |
documents.write | Allows API key to create, update, and delete documents. |
Other scopes are optional.
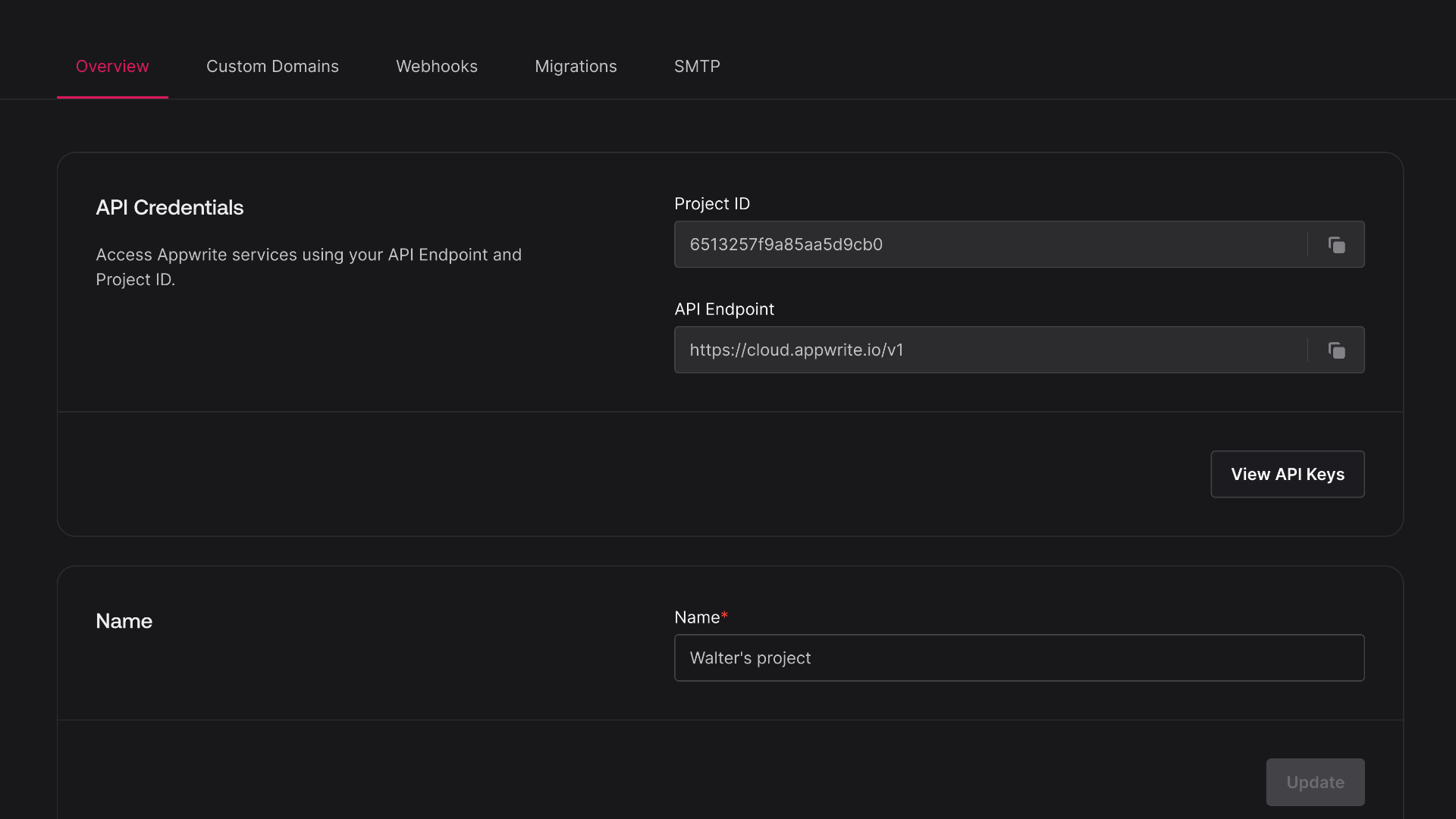
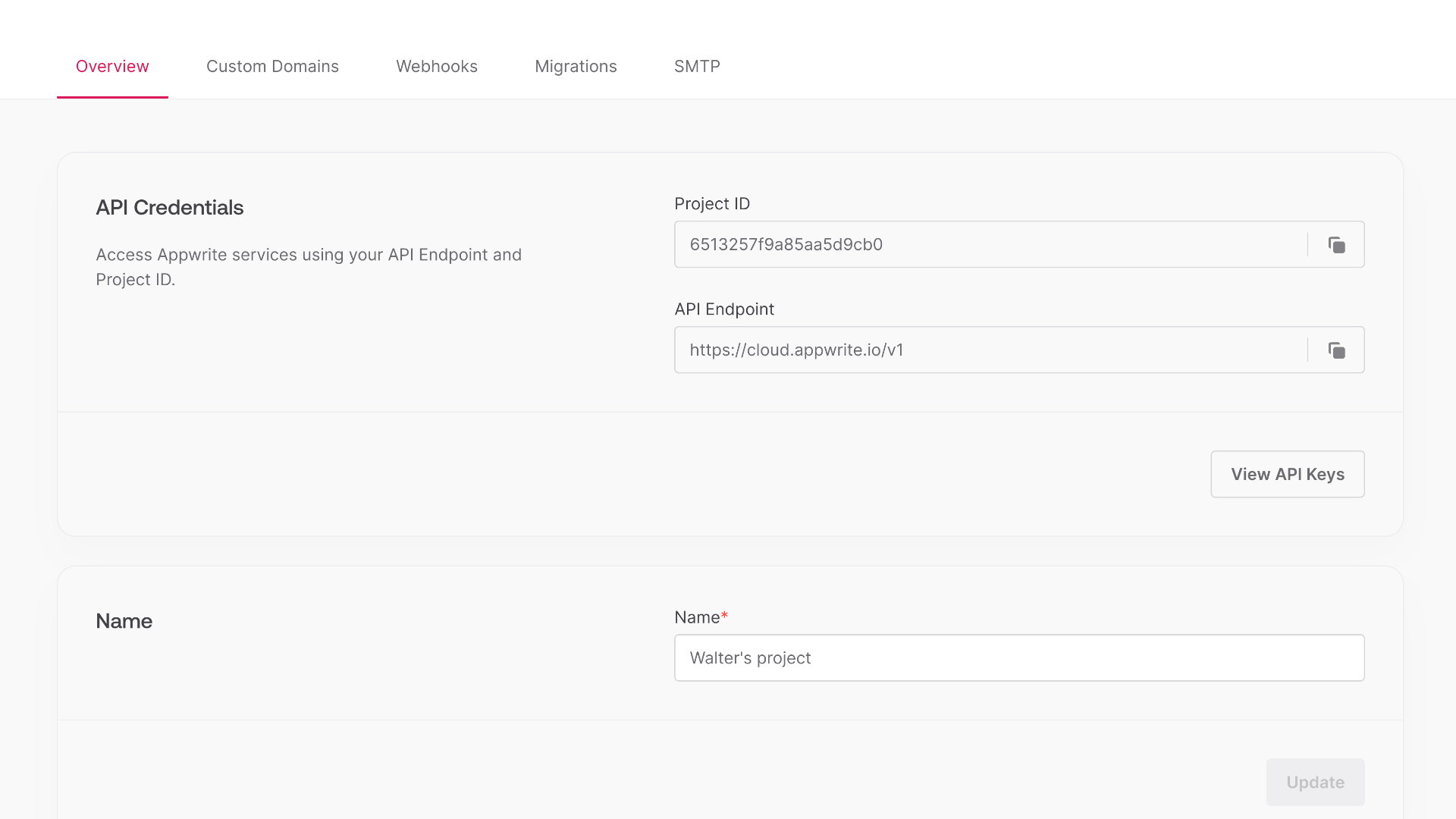
Create Python project
Create a directory for the project.
mkdir my_app
cd my_app
After that, create a virtual environment in this directory and activate it.
# Create a venv
python -m venv .venv
# Active the venv in Unix shell
source .venv/bin/activate
# Or in Powershell
.venv/Scripts/Activate.ps1
Finally, create a file my_app.py.
Install Appwrite
Install the Python Appwrite SDK.
pip install appwrite==11.0.0
Import Appwrite
Find your project ID in the Settings page. Also, click on the View API Keys button to find the API key that was created earlier.
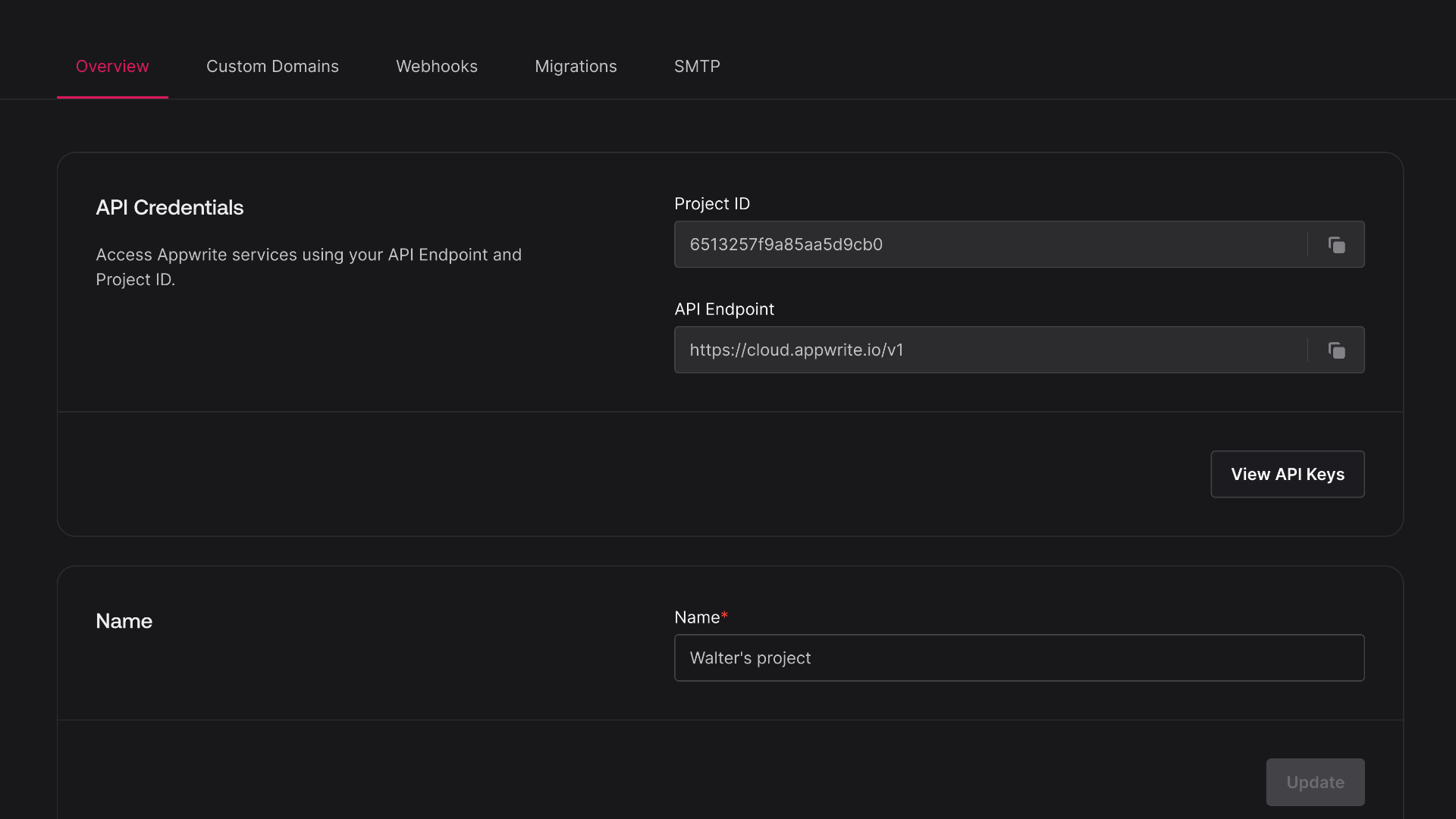
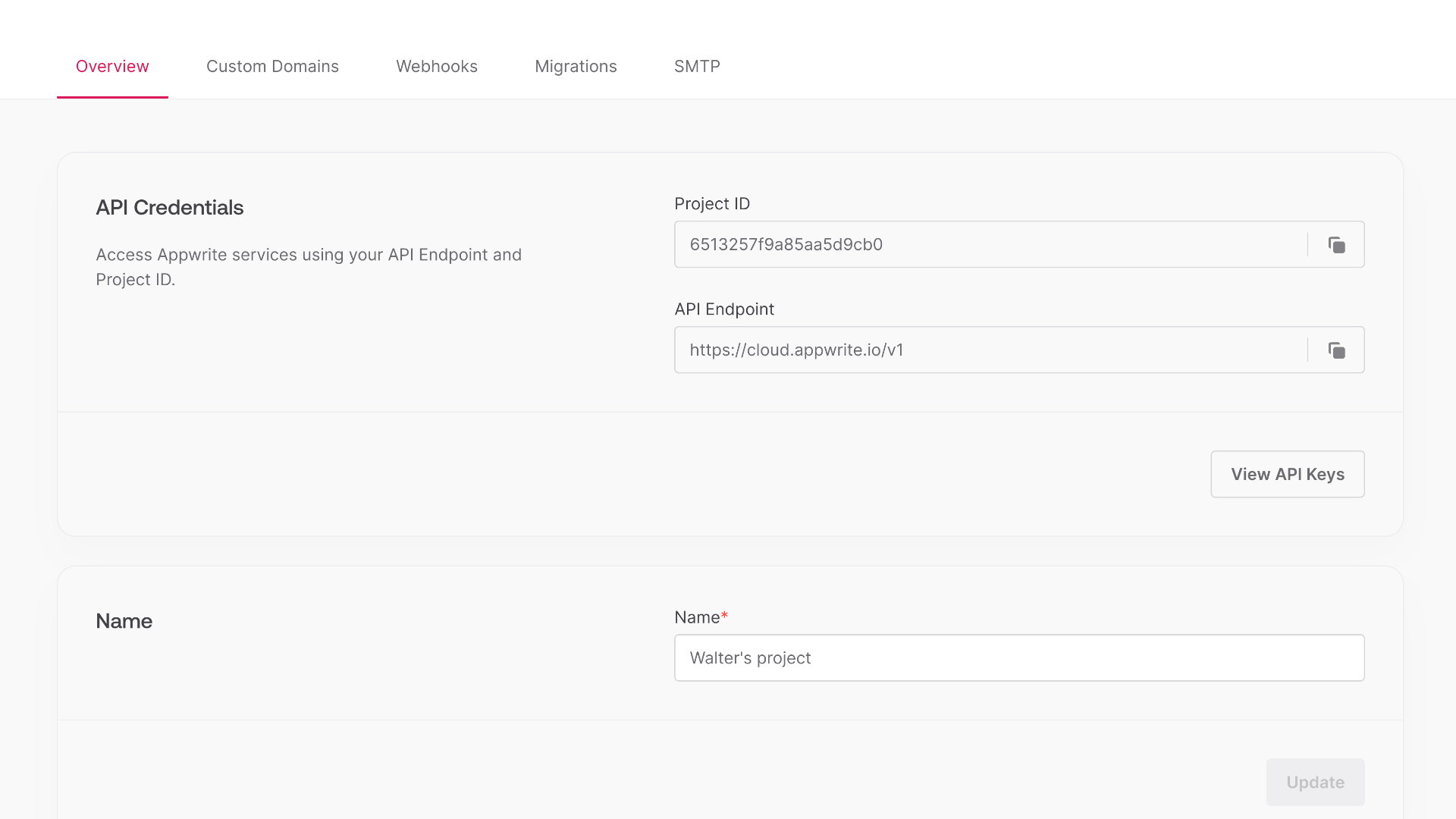
Open my_app.py and initialize the Appwrite Client. Replace <PROJECT_ID> with your project ID and <YOUR_API_KEY> with your API key.
from appwrite.client import Client
from appwrite.services.databases import Databases
from appwrite.id import ID
client = Client()
client.set_endpoint('https://<REGION>.cloud.appwrite.io/v1')
client.set_project('<PROJECT_ID>')
client.set_key('<YOUR_API_KEY>')
Initialize database
Once the Appwrite Client is initialized, create a function to configure a todo collection.
databases = Databases(client)
todoDatabase = None
todoCollection = None
def prepare_database():
global todoDatabase
global todoCollection
todoDatabase = databases.create(
database_id=ID.unique(),
name='TodosDB'
)
todoCollection = databases.create_collection(
database_id=todoDatabase['$id'],
collection_id=ID.unique(),
name='Todos'
)
databases.create_string_attribute(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id'],
key='title',
size=255,
required=True
)
databases.create_string_attribute(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id'],
key='description',
size=255,
required=False,
default='This is a test description.'
)
databases.create_boolean_attribute(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id'],
key='isComplete',
required=True
)
Add documents
Create a function to add some mock data into your new collection.
def seed_database():
testTodo1 = {
'title': "Buy apples",
'description': "At least 2KGs",
'isComplete': True
}
testTodo2 = {
'title': "Wash the apples",
'isComplete': True
}
testTodo3 = {
'title': "Cut the apples",
'description': "Don\'t forget to pack them in a box",
'isComplete': False
}
databases.create_document(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id'],
document_id=ID.unique(),
data=testTodo1
)
databases.create_document(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id'],
document_id=ID.unique(),
data=testTodo2
)
databases.create_document(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id'],
document_id=ID.unique(),
data=testTodo3
)
Retrieve documents
Create a function to retrieve the mock todo data, then execute the functions in _main_.
def get_todos():
todos = databases.list_documents(
database_id=todoDatabase['$id'],
collection_id=todoCollection['$id']
)
for todo in todos['documents']:
print(f"Title: {todo['title']}\nDescription: {todo['description']}\nIs Todo Complete: {todo['isComplete']}\n\n")
if __name__ == "__main__":
prepare_database()
seed_database()
get_todos()
All set
Run your project with python my_app.py and view the response in your console.