Learn how to setup your first Kotlin project powered by Appwrite.
Create project
Head to the Appwrite Console.
Server SDK
This tutorial is for the Kotlin Server SDK, meant for server and backend applications. If you're trying to build a client-side app, like an Android app, follow the Start with Android guide.
If this is your first time using Appwrite, create an account and create your first project.
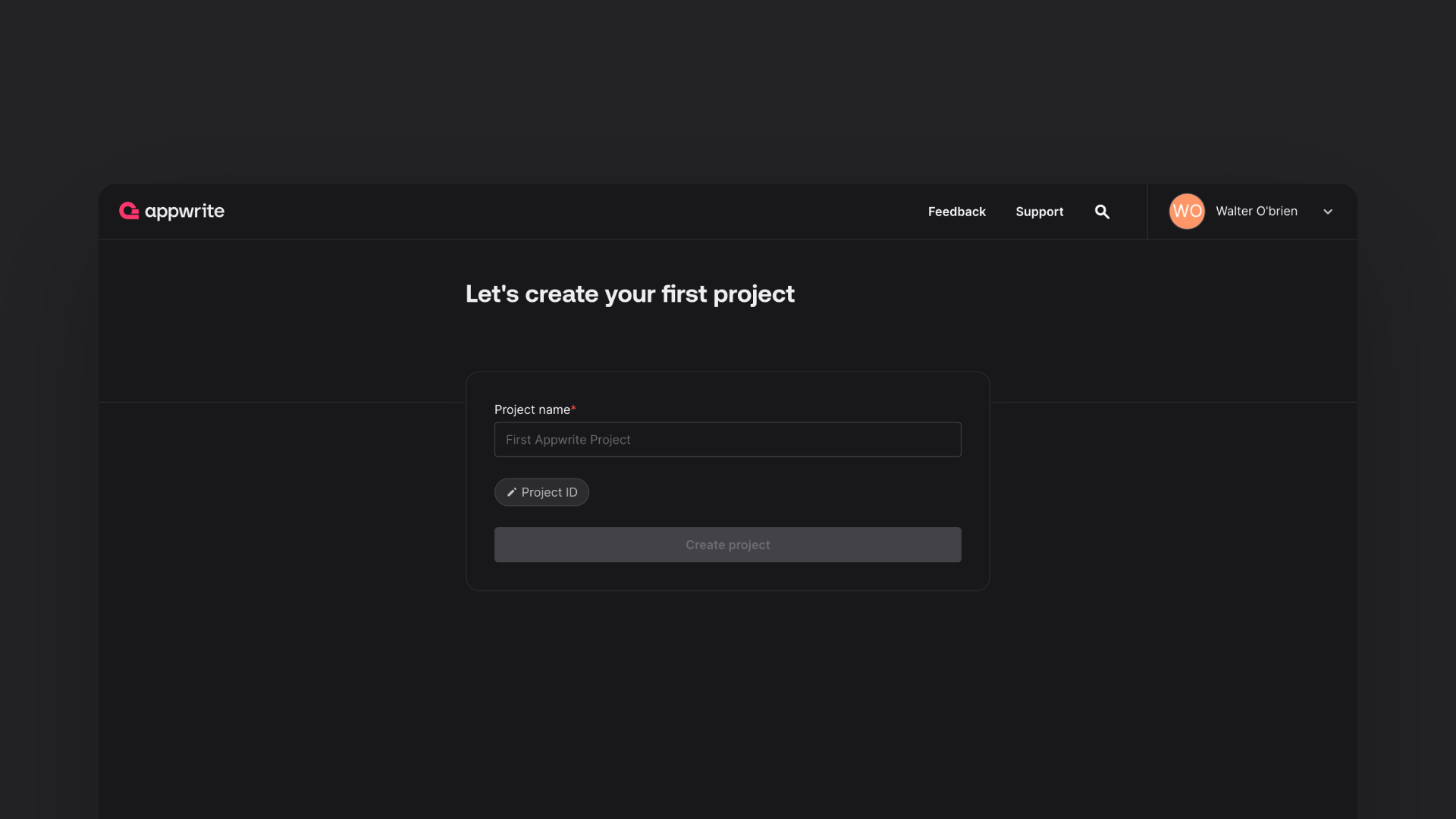
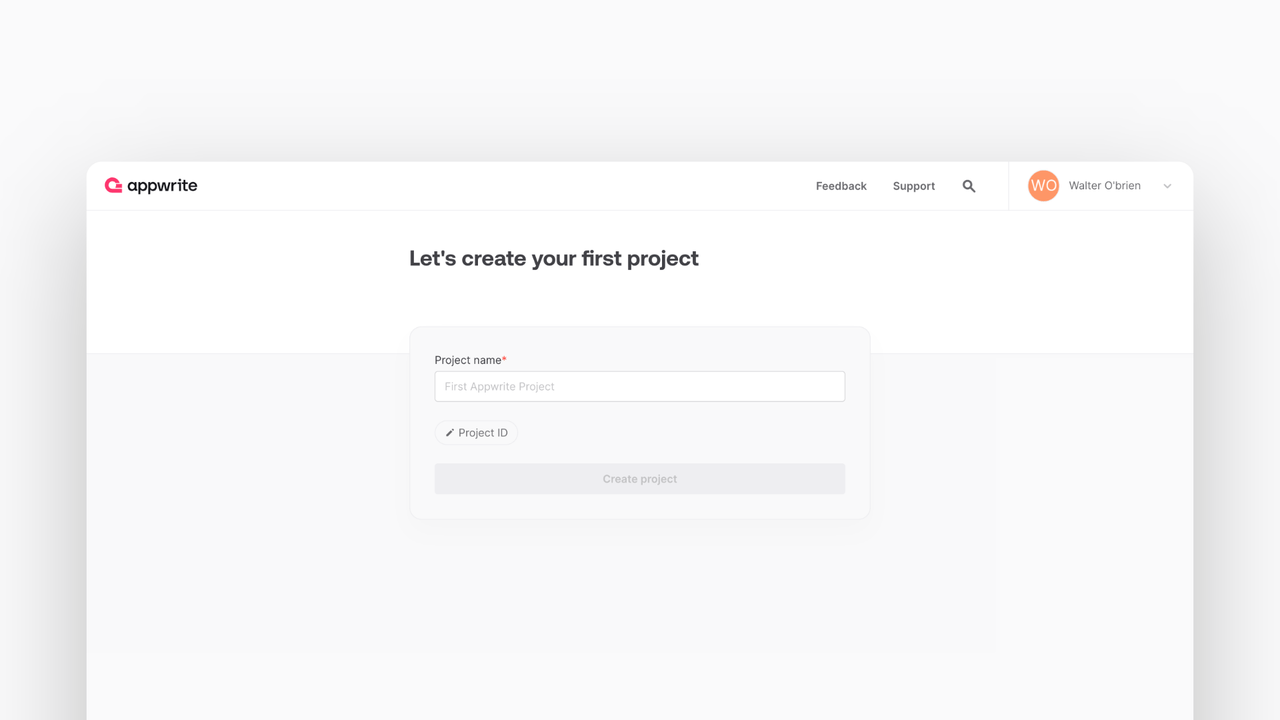
Then, under Integrate with your server, add an API Key with the following scopes.
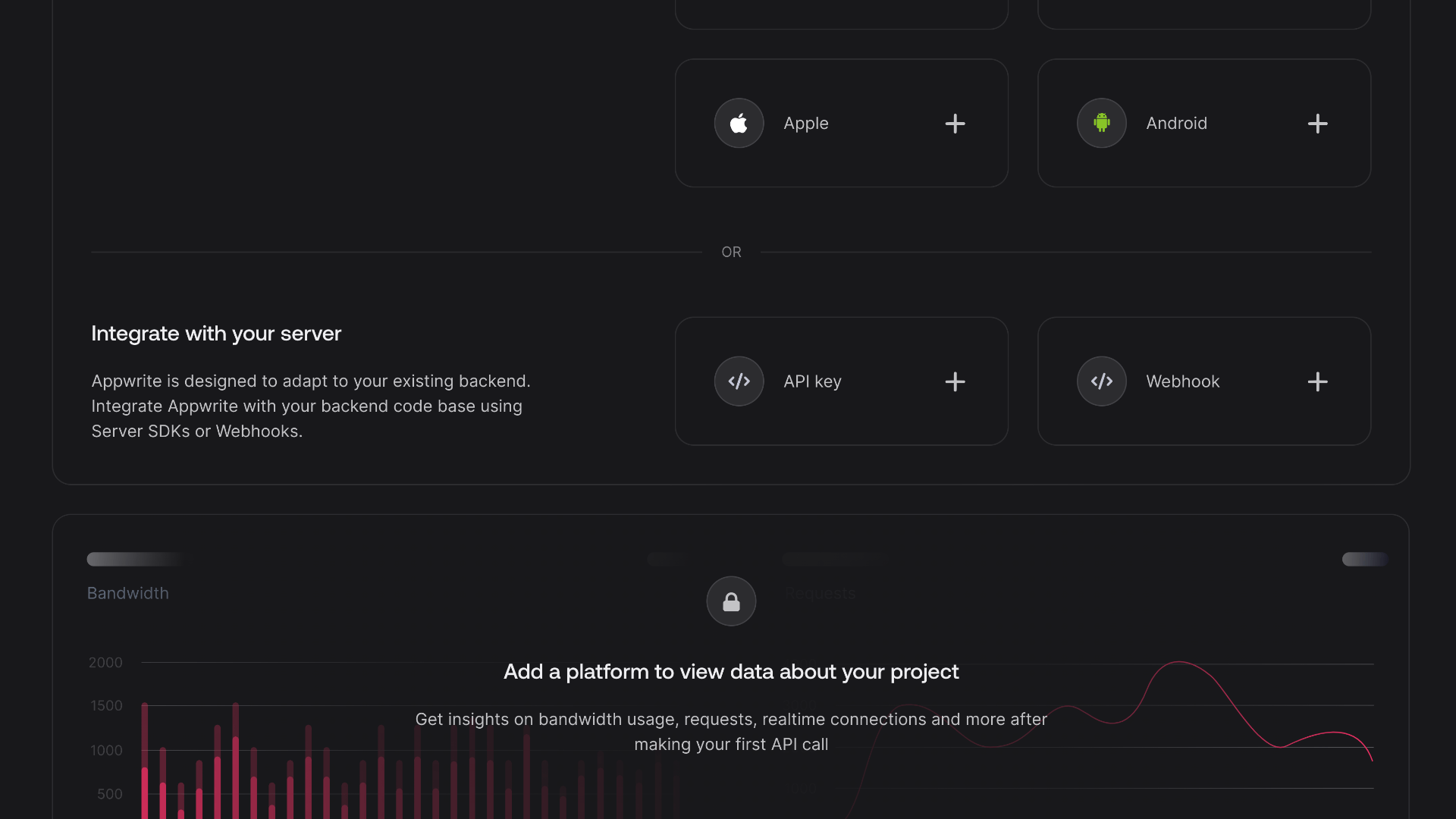
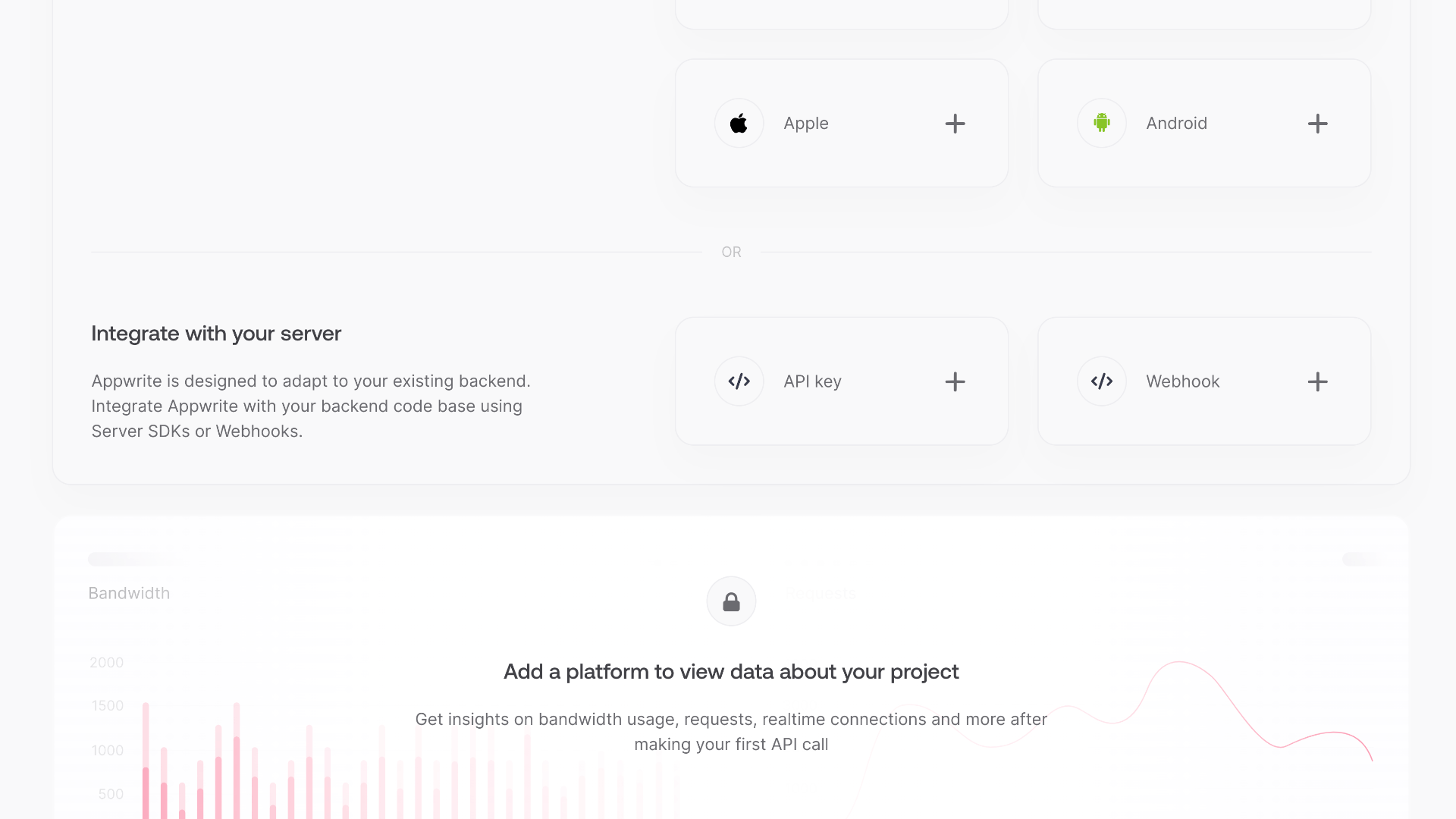
Category | Required scopes | Purpose |
Database | databases.write | Allows API key to create, update, and delete databases. |
collections.write | Allows API key to create, update, and delete collections. | |
attributes.write | Allows API key to create, update, and delete attributes. | |
documents.read | Allows API key to read documents. | |
documents.write | Allows API key to create, update, and delete documents. |
Other scopes are optional.
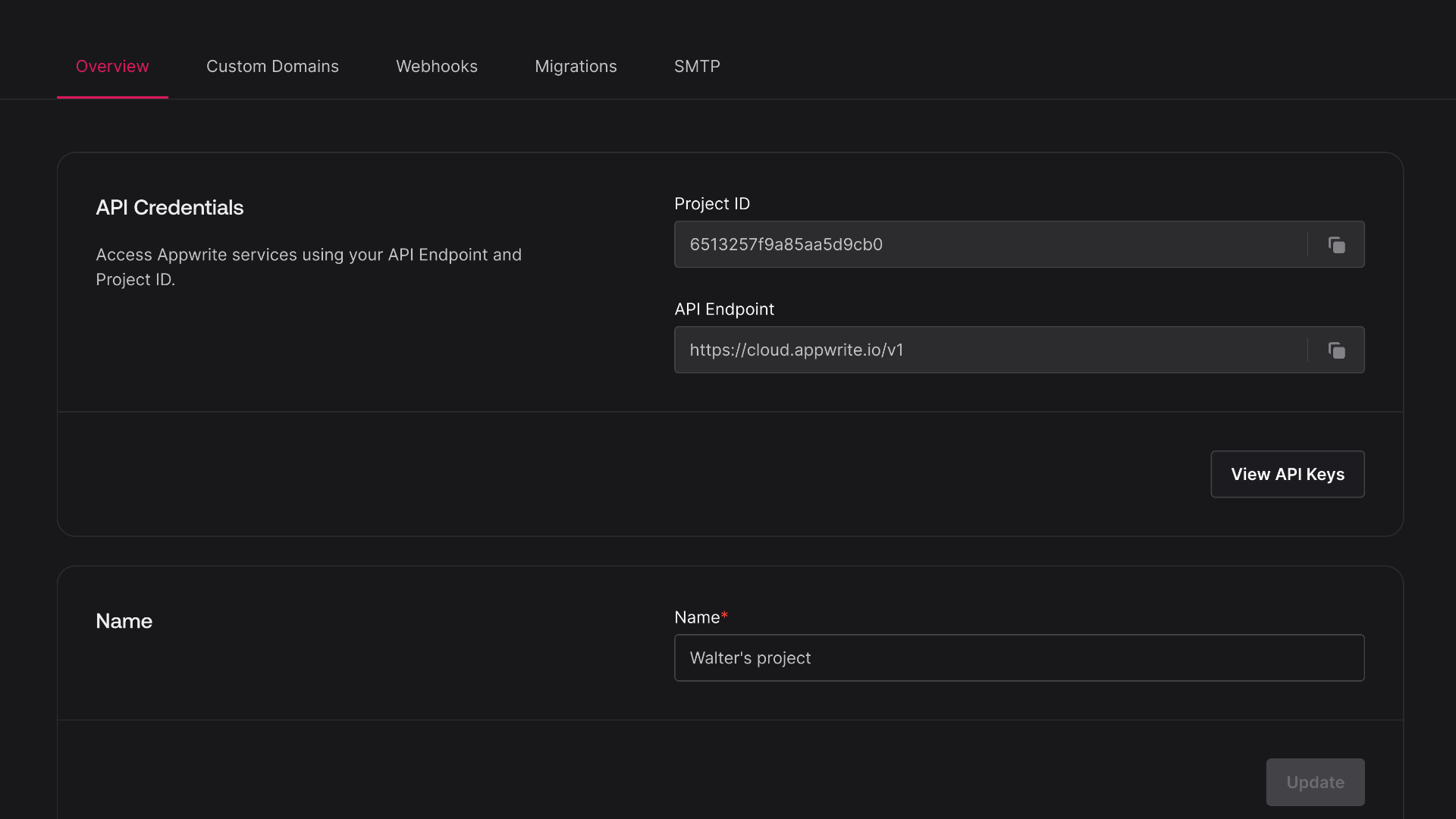
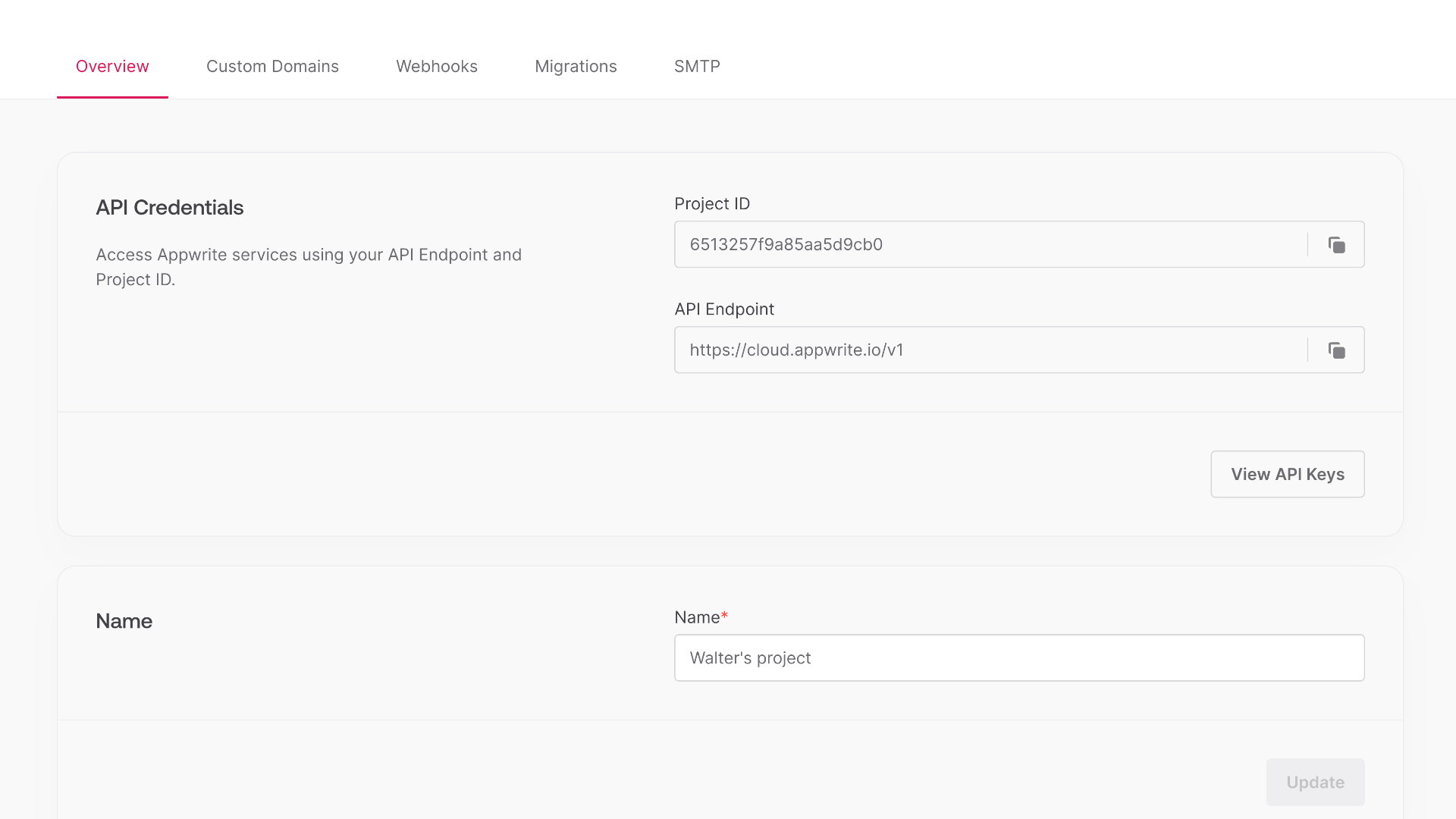
Create Kotlin project
Create a Kotlin application by opening IntelliJ IDEA > New Project and create a Kotlin application. This quick start will use Gradle as the build system, with the Kotlin DSL. You can follow with Maven or IntelliJ if you're more comfortable.
Follow the wizard and open your new project.
Install Appwrite
Open your build.gradle.kts file and implement the following dependency.
dependencies {
... other dependencies
implementation("io.appwrite:sdk-for-kotlin:5.0.1")
}
Import Appwrite
Find your project ID in the Settings page. Also, click on the View API Keys button to find the API key that was created earlier.
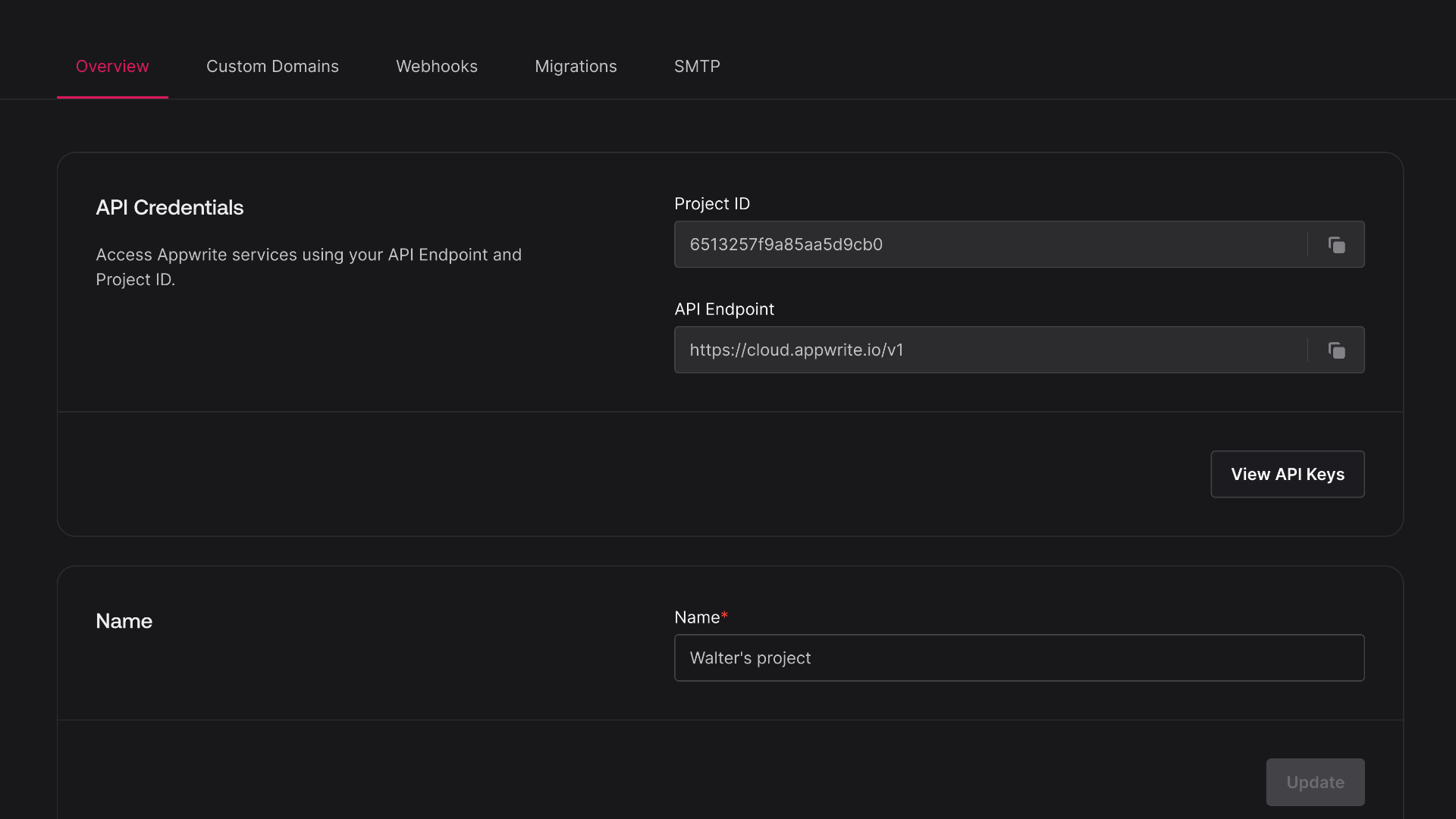
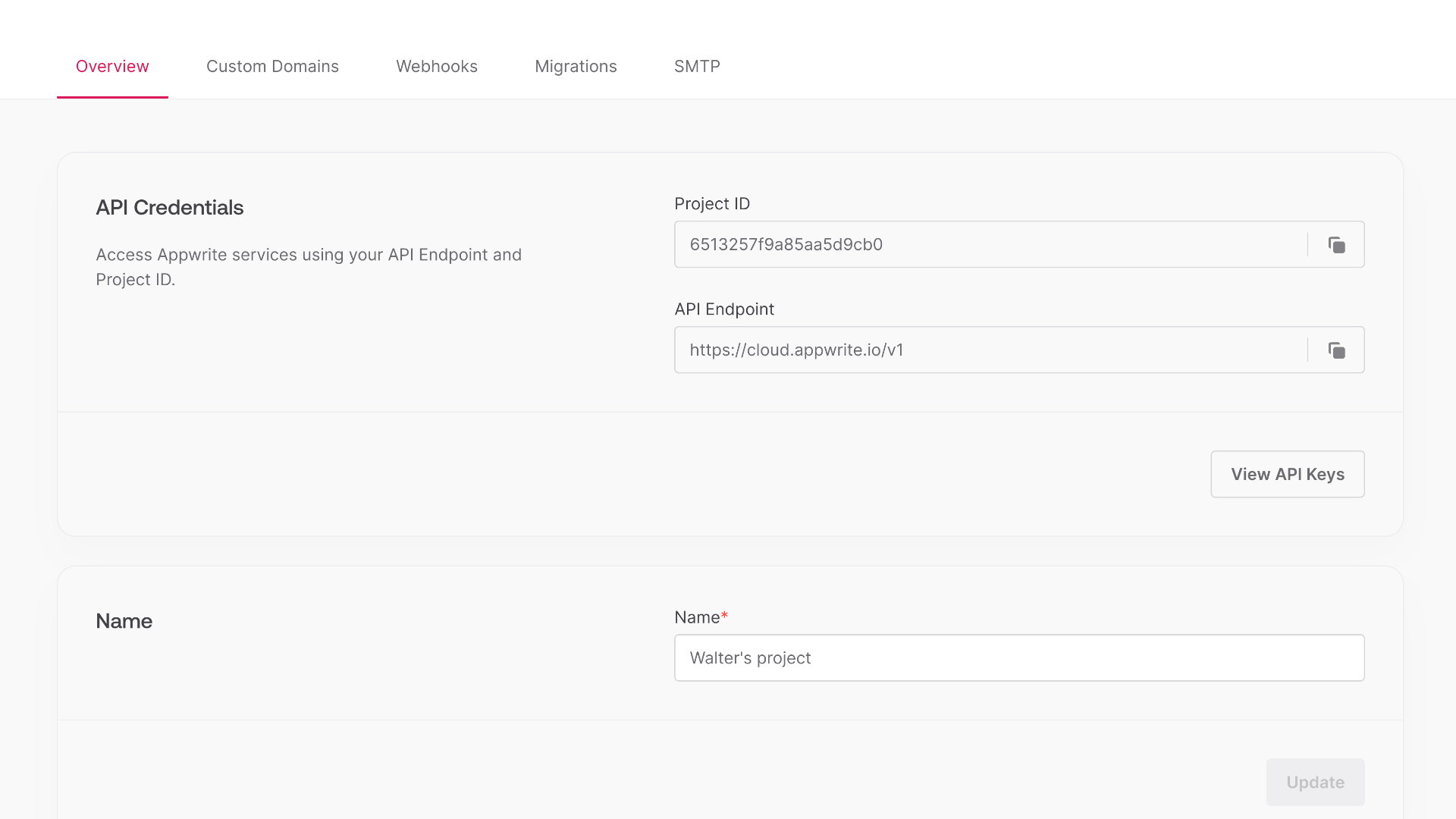
Open the file Main.kt and initialize the Appwrite Client. Replace <PROJECT_ID> with your project ID and <YOUR_API_KEY> with your API key.
import io.appwrite.Client
import io.appwrite.ID
import io.appwrite.services.Databases
import io.appwrite.models.Database
import io.appwrite.models.Collection
import kotlinx.coroutines.coroutineScope
val client = Client()
.setEndpoint("https://<REGION>.cloud.appwrite.io/v1")
.setProject("<PROJECT_ID>")
.setKey("<YOUR_API_KEY>");
Initialize database
Once the Appwrite Client is initialized, create a function to configure a todo collection.
val databases = Databases(client)
var todoDatabase: Database? = null
var todoCollection: Collection? = null
suspend fun prepareDatabase() {
todoDatabase = databases.create(ID.unique(), "TodosDB")
todoCollection = databases.createCollection(todoDatabase?.id!!, ID.unique(), "Todos")
databases.createStringAttribute(
databaseId = todoDatabase?.id!!,
collectionId = todoCollection?.id!!,
key = "title",
size = 255,
required = true
)
databases.createStringAttribute(
databaseId = todoDatabase?.id!!,
collectionId = todoCollection?.id!!,
key = "description",
size = 255,
required = false,
default = "This is a test description."
)
databases.createBooleanAttribute(
databaseId = todoDatabase?.id!!,
collectionId = todoCollection?.id!!,
key = "isComplete",
required = true
)
}
Add documents
Create a function to add some mock data into your new collection.
suspend fun seedDatabase() {
val testTodo1 = mapOf(
"title" to "Buy apples",
"description" to "At least 2KGs",
"isComplete" to true
)
val testTodo2 = mapOf(
"title" to "Wash the apples",
"isComplete" to true
)
val testTodo3 = mapOf(
"title" to "Cut the apples",
"description" to "Don't forget to pack them in a box",
"isComplete" to false
)
databases.createDocument(
databaseId = todoDatabase?.id!!,
collectionId = todoCollection?.id!!,
documentId = ID.unique(),
data = testTodo1
)
databases.createDocument(
databaseId = todoDatabase?.id!!,
collectionId = todoCollection?.id!!,
documentId = ID.unique(),
data = testTodo2
)
databases.createDocument(
databaseId = todoDatabase?.id!!,
collectionId = todoCollection?.id!!,
documentId = ID.unique(),
data = testTodo3
)
}
Retrieve documents
Create a function to retrieve the mock todo data.
suspend fun getTodos() {
val todos = databases.listDocuments(todoDatabase?.id!!, todoCollection?.id!!)
for (todo in todos.documents) {
println(
"""
Title: ${todo.data["title"]}
Description: ${todo.data["description"]}
Is Todo Complete: ${todo.data["isComplete"]}
""".trimIndent()
)
}
}
suspend fun main() = coroutineScope {
prepareDatabase()
seedDatabase()
getTodos()
}
All set
Run your project with IntelliJ and view the response in your console.