Learn how to setup your first Dart project powered by Appwrite.
Create project
Head to the Appwrite Console.
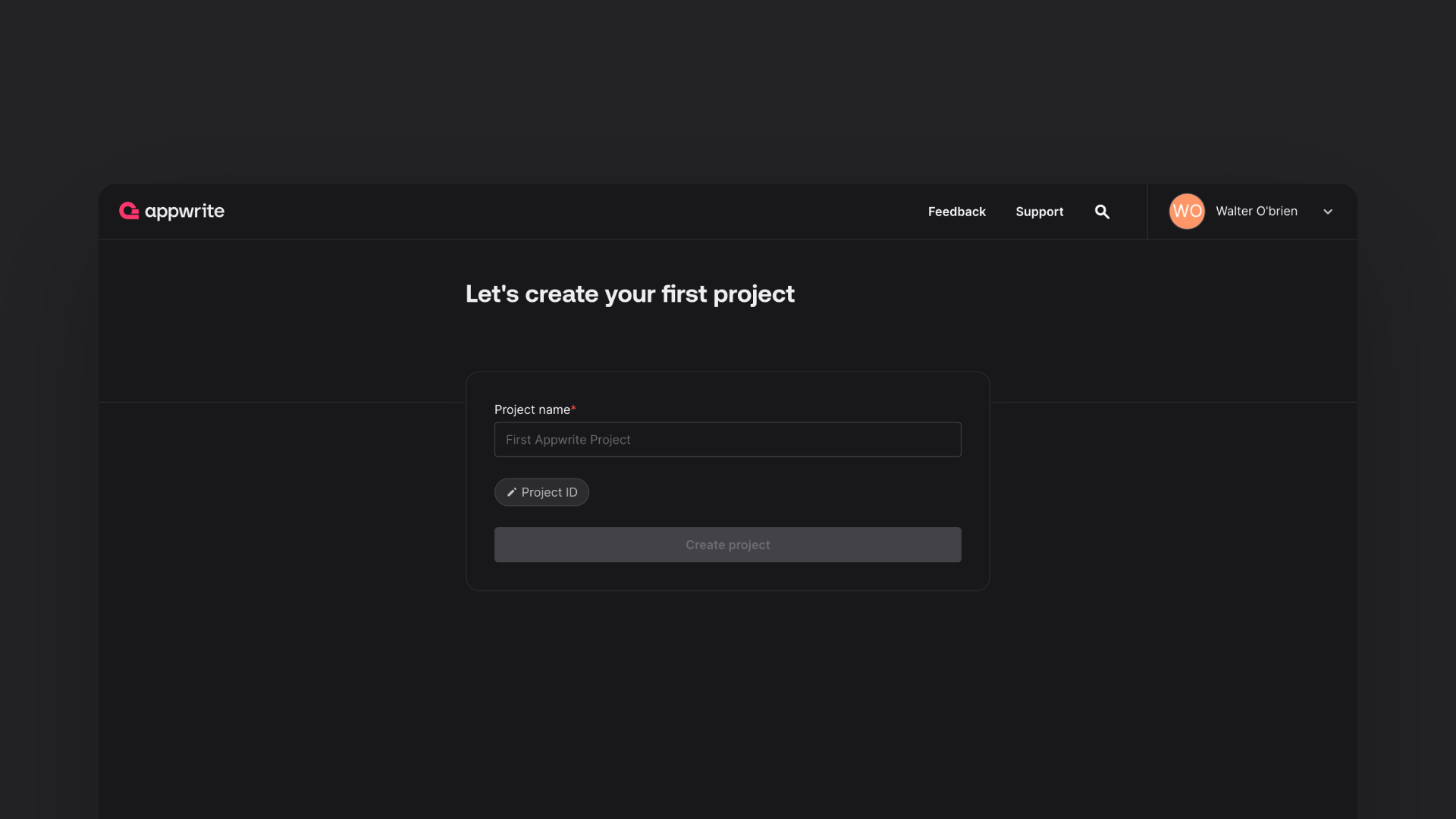
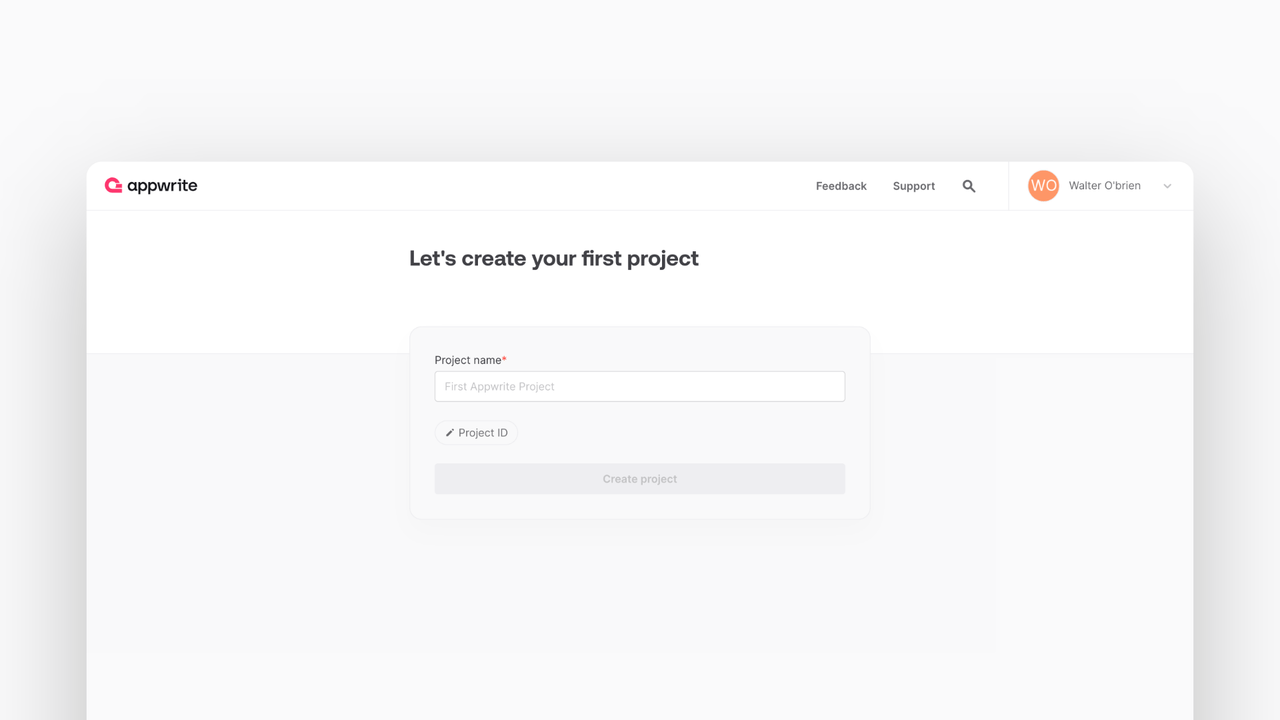
If this is your first time using Appwrite, create an account and create your first project.
Then, under Integrate with your server, add an API Key with the following scopes.
Category | Required scopes | Purpose |
Database | databases.write | Allows API key to create, update, and delete databases. |
collections.write | Allows API key to create, update, and delete collections. | |
attributes.write | Allows API key to create, update, and delete attributes. | |
documents.read | Allows API key to read documents. | |
documents.write | Allows API key to create, update, and delete documents. |
Other scopes are optional.
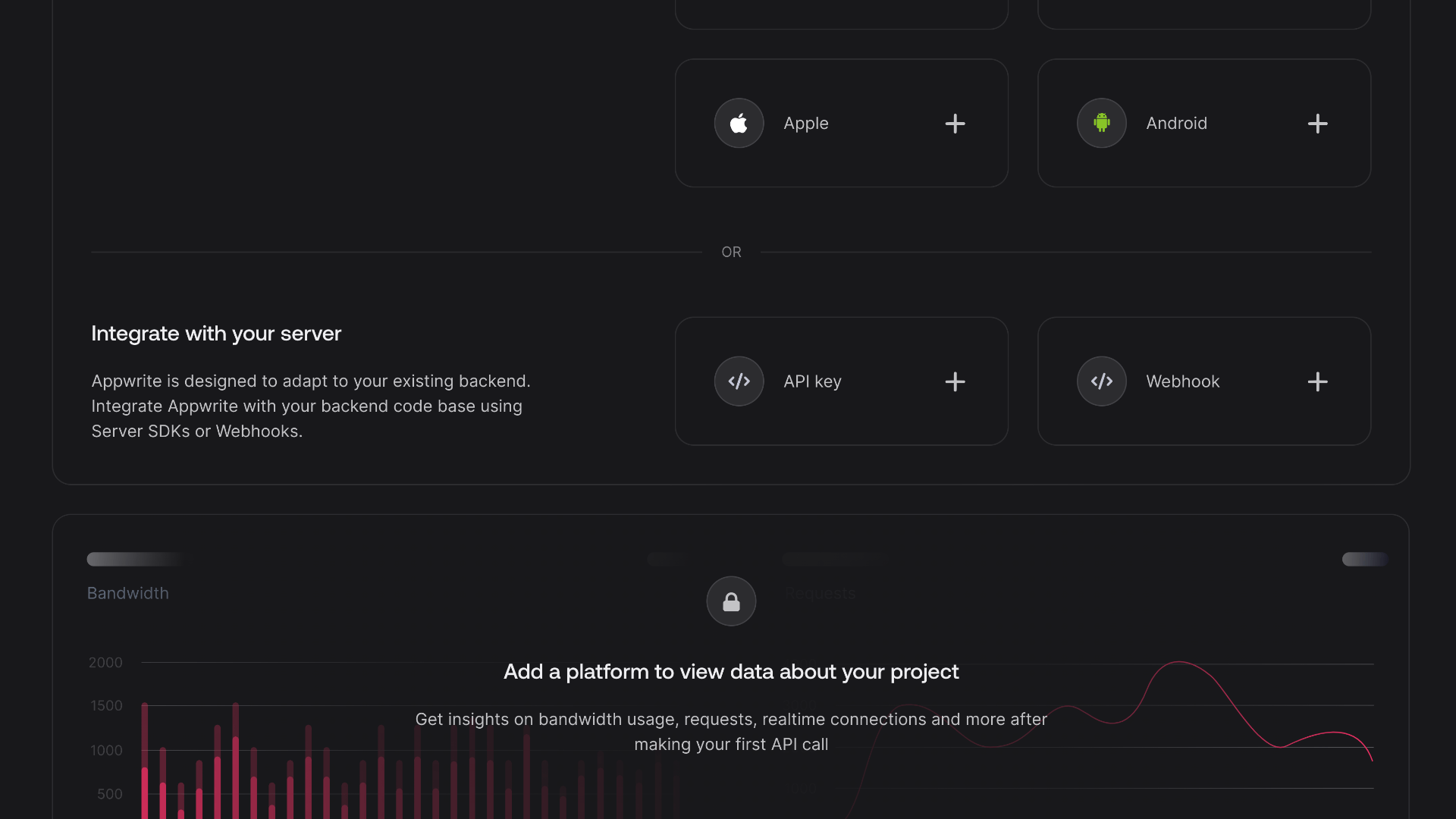
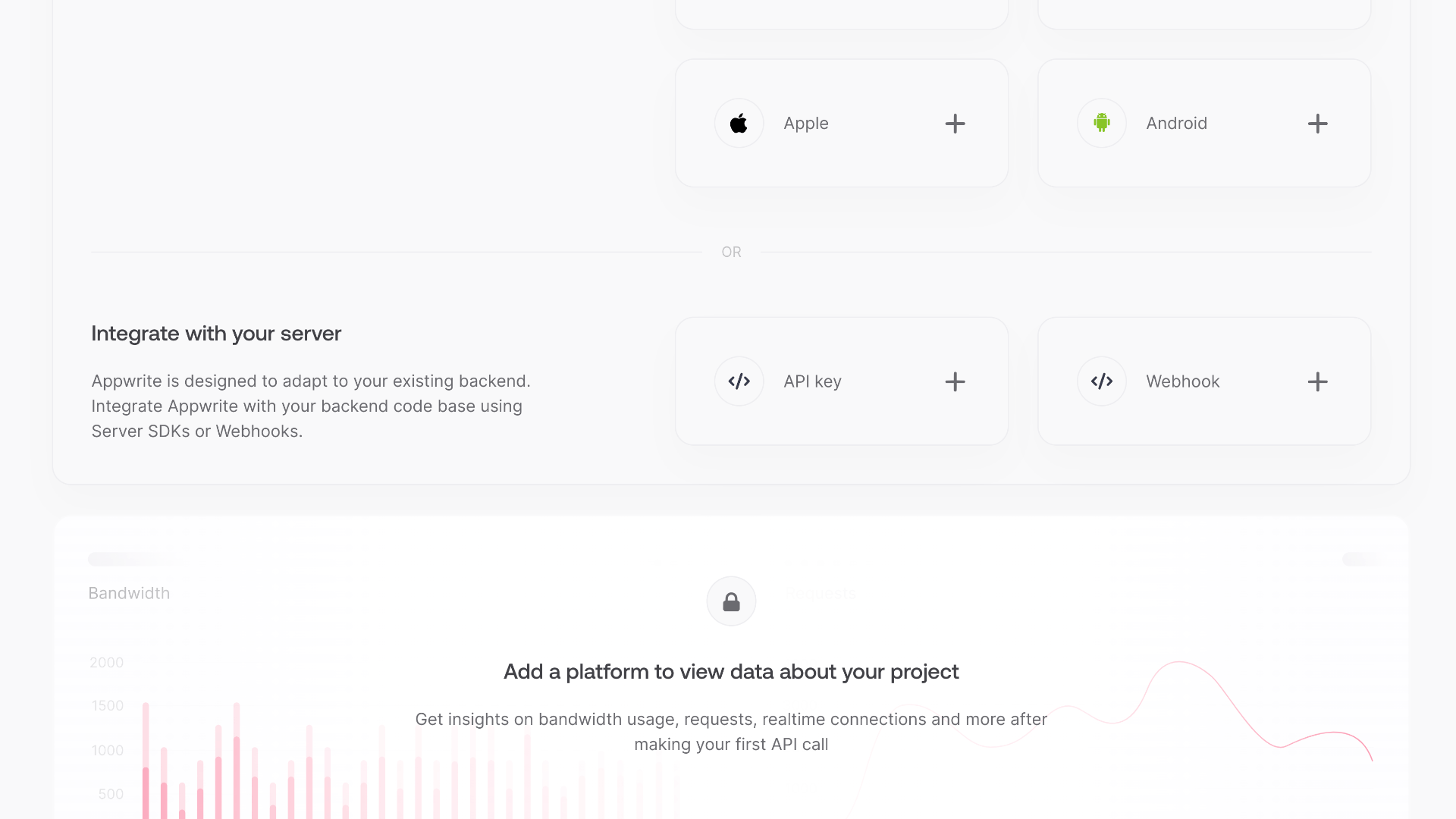
Create Dart project
Create a Dart CLI application.
dart create -t console my_app
cd my_app
After entering the project directory, remove the lib/ and test/ directories.
Install Appwrite
Install the Dart Appwrite SDK.
dart pub add dart_appwrite:16.0.0
Import Appwrite
Find your project ID in the Settings page.
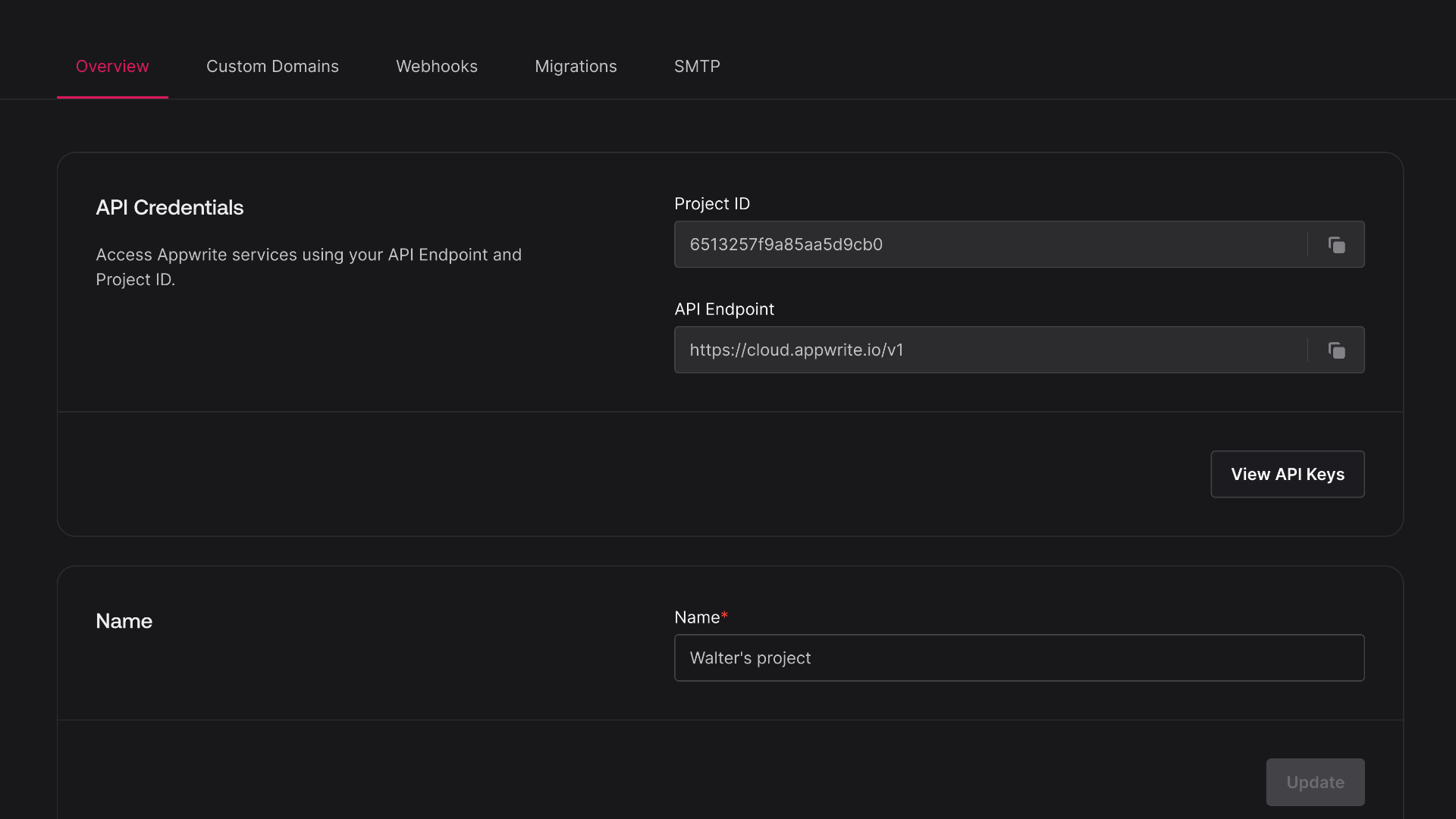
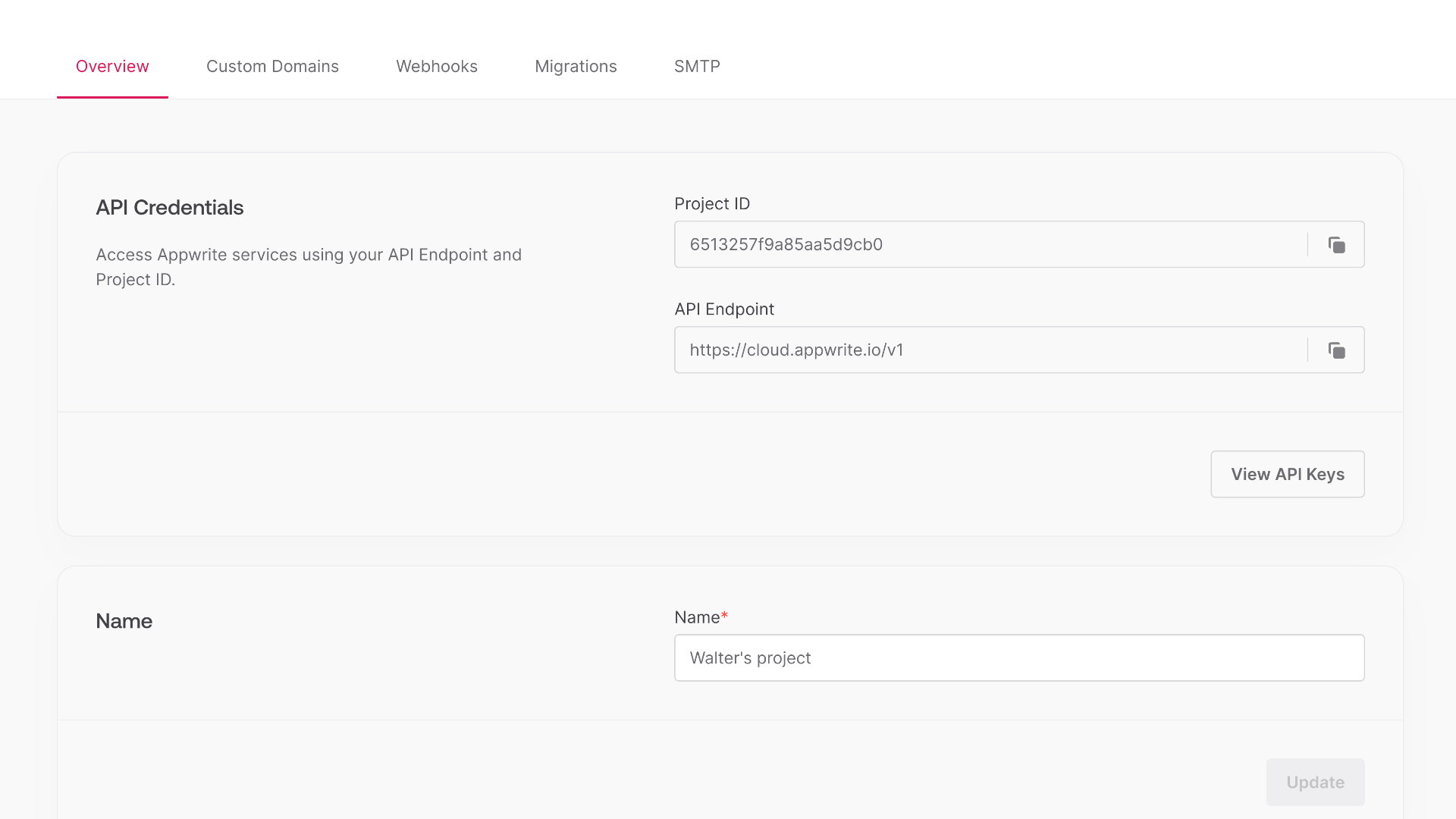
Also, click on the View API Keys button to find the API key that was created earlier.
Open bin/my_app.dart and initialize the Appwrite Client. Replace <PROJECT_ID> with your project ID and <YOUR_API_KEY> with your API key.
import 'package:dart_appwrite/dart_appwrite.dart';
var client = Client();
Future<void> main() async {
client
.setEndpoint("https://<REGION>.cloud.appwrite.io/v1")
.setProject("<PROJECT_ID>")
.setKey("<YOUR_API_KEY>");
}
Initialize database
Once the Appwrite Client is initialized, create a function to configure a todo collection.
var databases;
var todoDatabase;
var todoCollection;
Future<void> prepareDatabase() async {
databases = Databases(client);
todoDatabase = await databases.create(
databaseId: ID.unique(),
name: 'TodosDB'
);
todoCollection = await databases.createCollection(
databaseId: todoDatabase.$id,
collectionId: ID.unique(),
name: 'Todos'
);
await databases.createStringAttribute(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id,
key: 'title',
size: 255,
xrequired: true
);
await databases.createStringAttribute(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id,
key: 'description',
size: 255,
xrequired: false,
xdefault: 'This is a test description'
);
await databases.createBooleanAttribute(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id,
key: 'isComplete',
xrequired: true
);
}
Add documents
Create a function to add some mock data into your new collection.
Future<void> seedDatabase() async {
var testTodo1 = {
'title': 'Buy apples',
'description': 'At least 2KGs',
'isComplete': true
};
var testTodo2 = {
'title': 'Wash the apples',
'isComplete': true
};
var testTodo3 = {
'title': 'Cut the apples',
'description': 'Don\'t forget to pack them in a box',
'isComplete': false
};
await databases.createDocument(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id,
documentId: ID.unique(),
data: testTodo1
);
await databases.createDocument(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id,
documentId: ID.unique(),
data: testTodo2
);
await databases.createDocument(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id,
documentId: ID.unique(),
data: testTodo3
);
}
Retrieve documents
Create a function to retrieve the mock todo data.
Future<void> getTodos() async {
var todos = await databases.listDocuments(
databaseId: todoDatabase.$id,
collectionId: todoCollection.$id
);
todos.documents.forEach((todo) {
print('Title: ${todo.data['title']}\nDescription: ${todo.data['description']}\nIs Todo Complete: ${todo.data['isComplete']}\n\n');
});
}
Finally, revisit the main() function and call the functions created in previous steps.
Future<void> main() async {
client
.setEndpoint("https://<REGION>.cloud.appwrite.io/v1")
.setProject("<PROJECT_ID>")
.setKey("<YOUR_API_KEY>");
await prepareDatabase();
await Future.delayed(const Duration(seconds: 1));
await seedDatabase();
await getTodos();
}
All set
Run your project with dart run bin/my_app.dart and view the response in your console.