File tokens are a type of secret that allow you to share files publicly with anyone. By using file tokens, you can let any external user access your file without having to configure bucket or file permissions. File tokens can either be set to expire on a specific date or work indefinitely.
File tokens vs secure cookies
Currently, Appwrite uses secure cookies to manage sessions for users, which are essential for any Appwrite products with permissions configured. However, because the cookies sent to the users of apps consuming the Appwrite API are considered third-party cookies, certain browsers tend to block them due to their default privacy settings, creating a bad user experience.
One way to circumvent this issue in the past was to connect a custom domain to your Appwrite project, as browsers don't inherently block any cookies returned by subdomains of your app. File tokens offer an alternative, simpler path for file sharing in Appwrite Storage, as they don't depend on the session for authorization to share data.
Create file tokens
To create a file token, you must upload a file to a storage bucket.
Head to the Storage page, open a file inside a bucket, scroll down to the File tokens section, and click on the Create file token button.
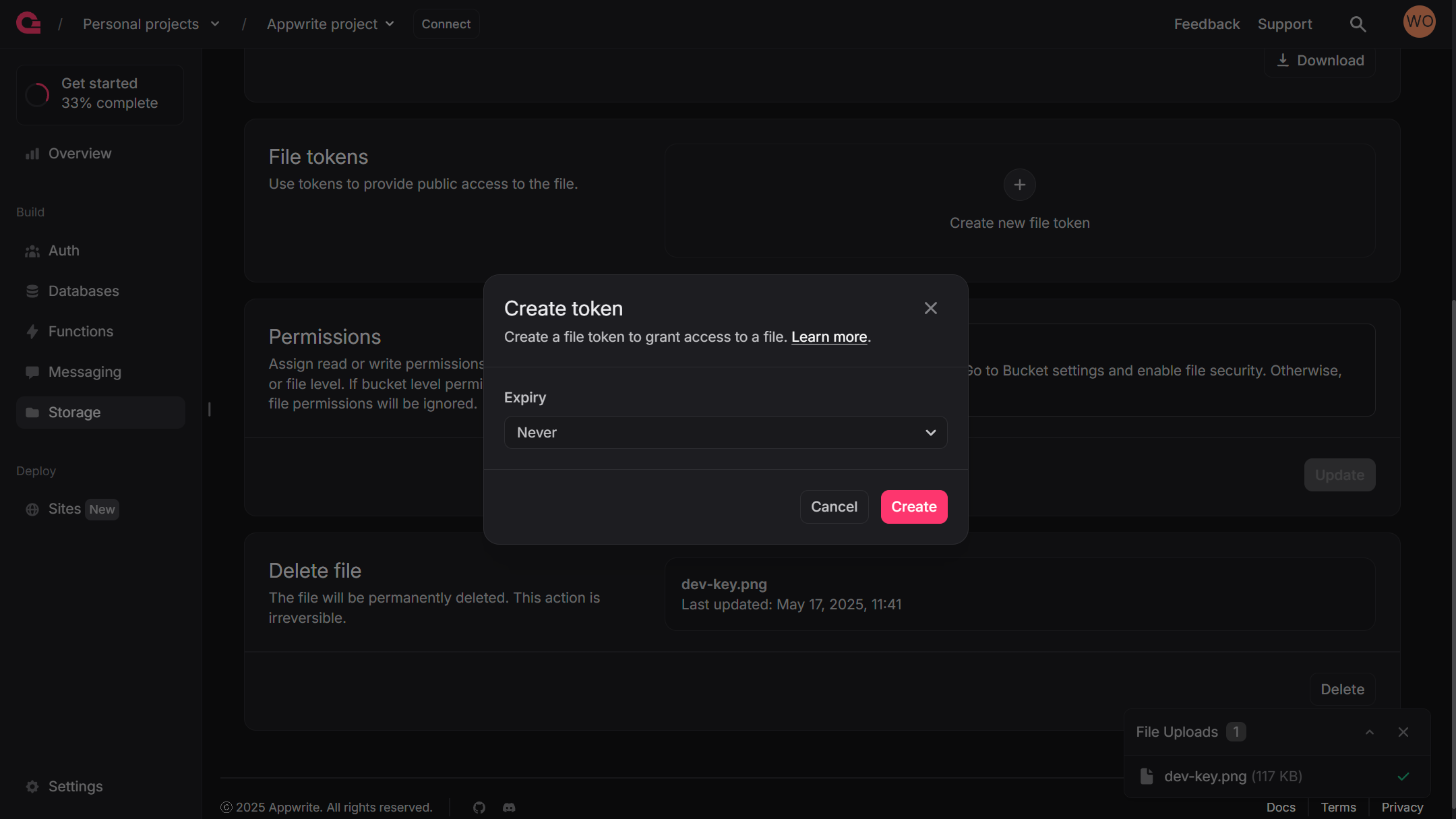
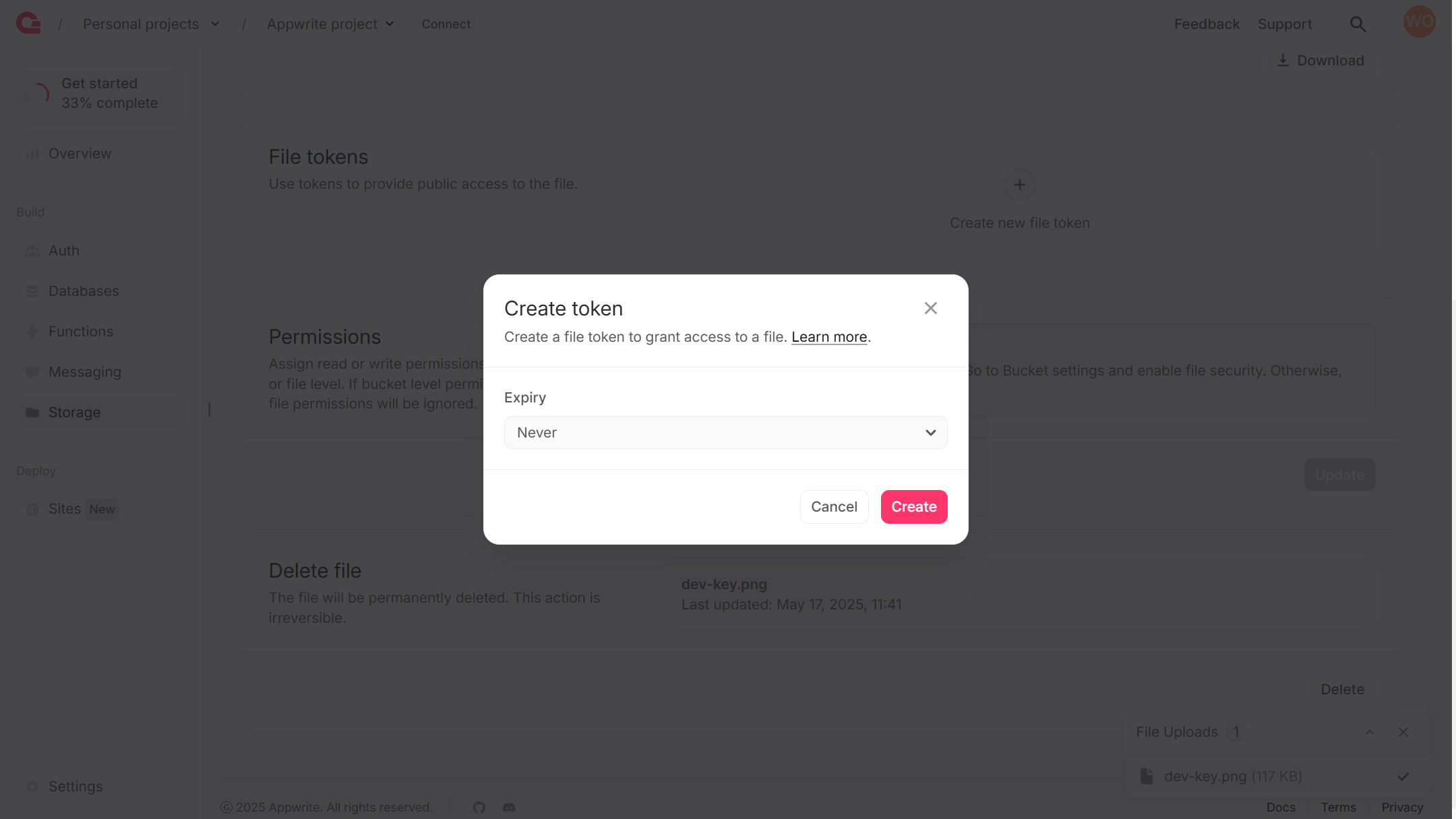
You can then click on the three-dots menu, click on Copy URL and get the token-based preview, view, and download URLs for the file.
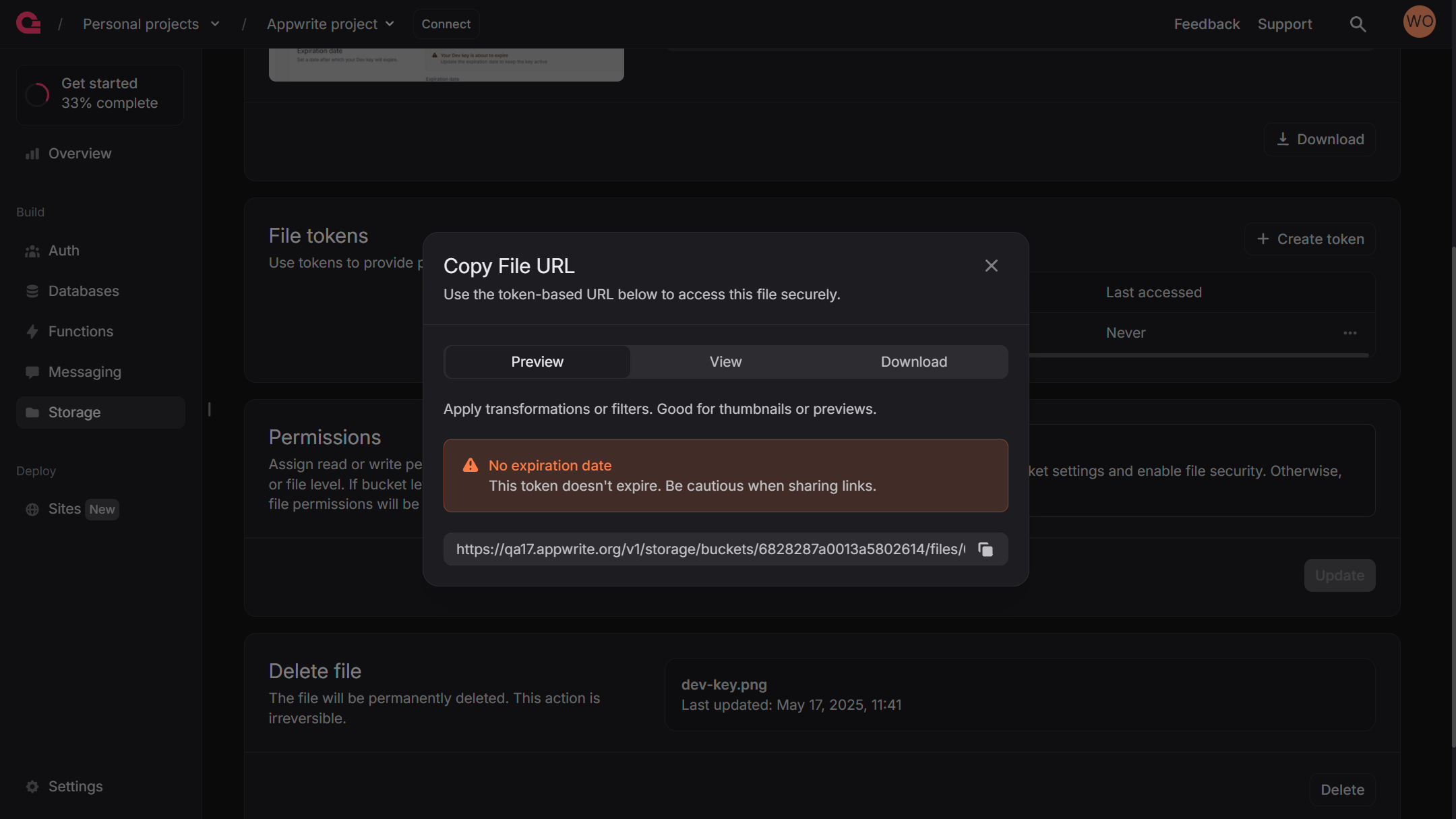
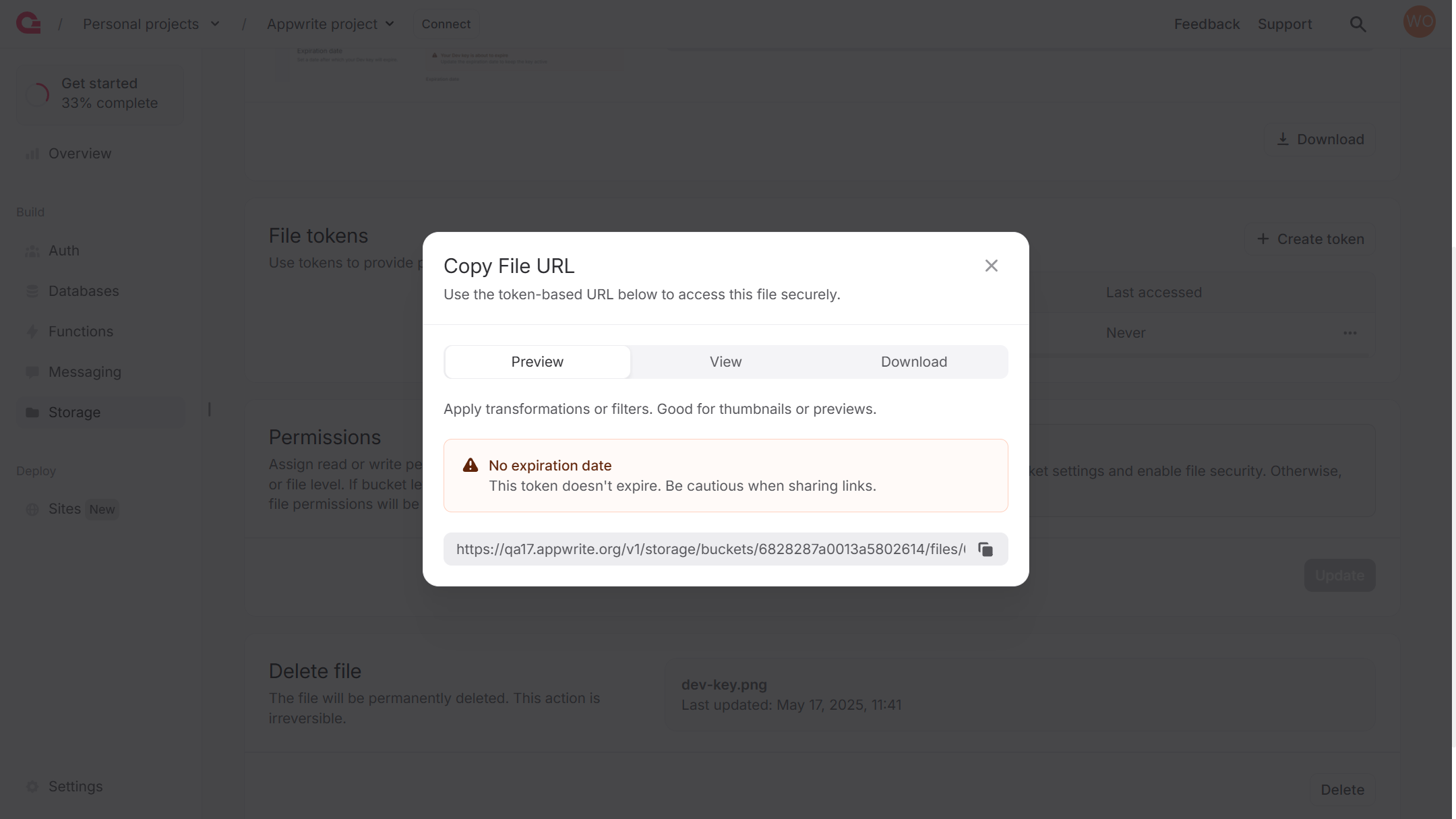
You can create file tokens programmatically using a Server SDK. Appwrite's Server SDKs require an API key with the tokens.write scope enabled.
The created token can then be used along with Appwrite Storage's view, preview, and download endpoints.
List all file tokens
You can use the Appwrite Console or one of the Server SDKs to view all created file tokens, their expiry dates and the time each token was last accessed at.
Head to the Storage page, open a file inside a bucket, and scroll down to the File tokens section.
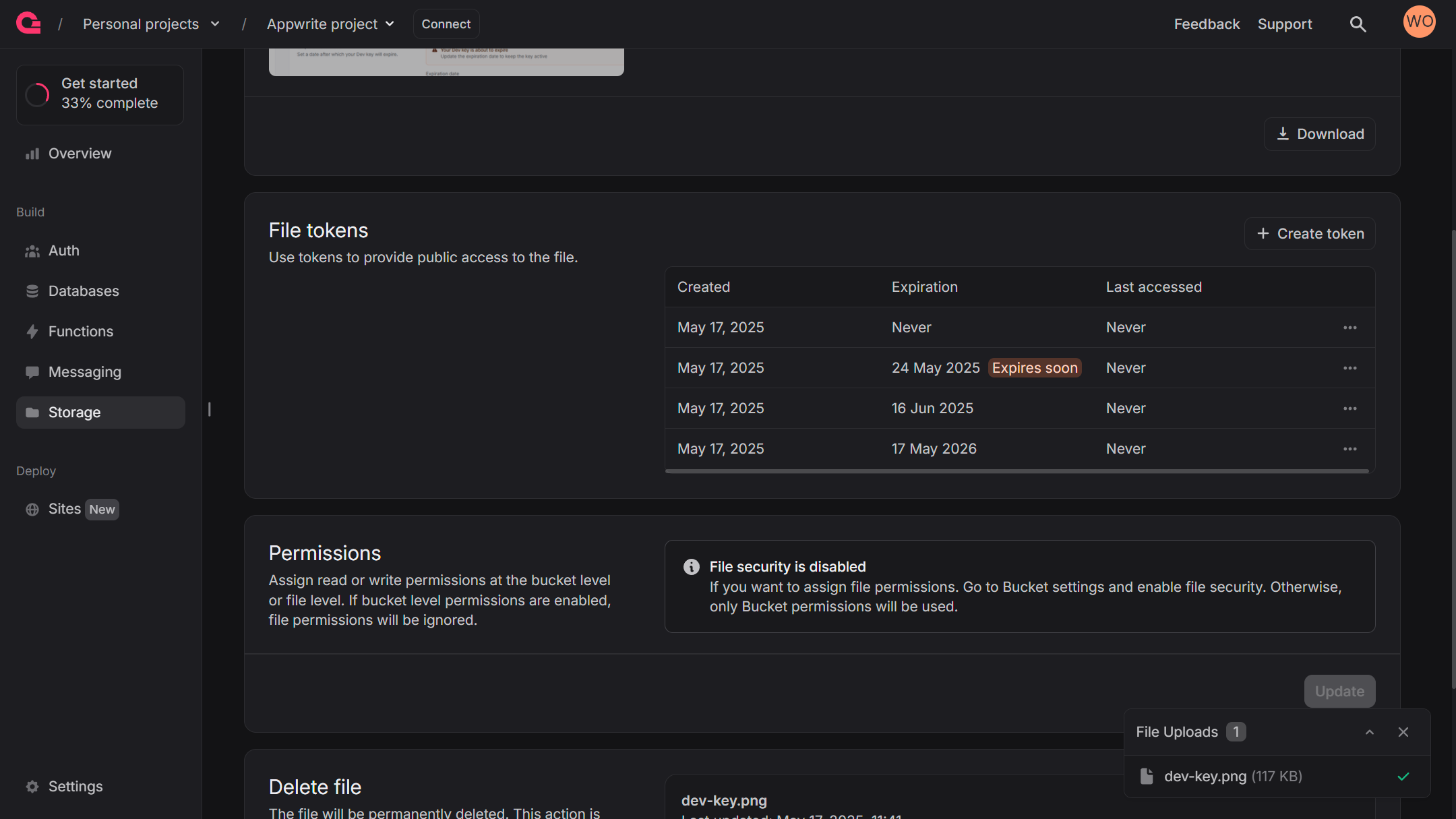
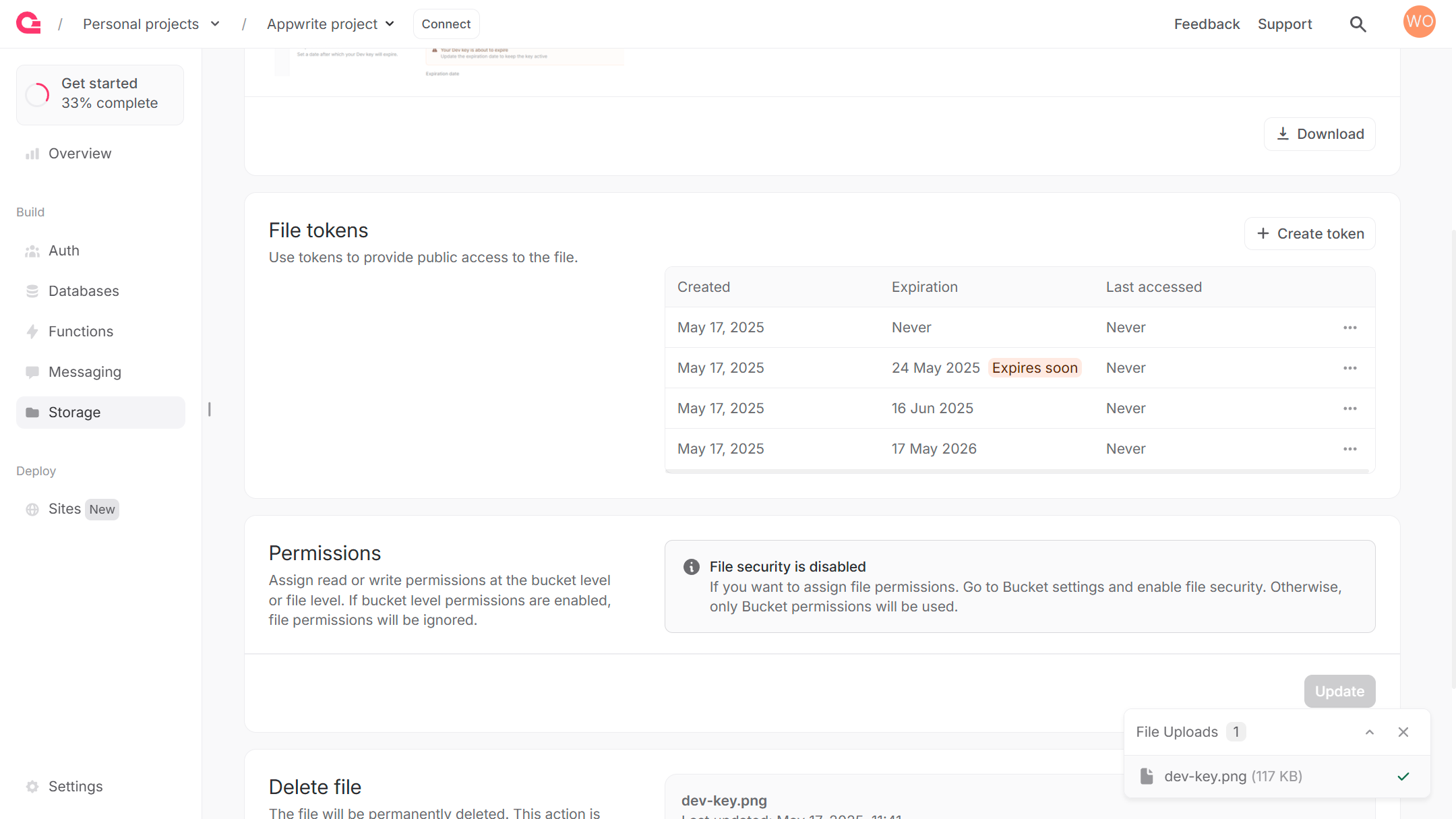
You can list all file tokens programmatically using a Server SDK. Appwrite's Server SDKs require an API key with the tokens.read scope enabled.
Using the token IDs, you can also get data pertaining to an individual file token.
Update file token expiry
File tokens can be set to expire on a specific date or stay active forever. This helps keep your files secure by making sure access ends after a set time.
While the expiry of a file token is set at the time of creation, you can update it later.
Head to the Storage page, open a file inside a bucket, and scroll down to the File tokens section. Click on the three-dots menu next to the created file token and click on Edit expiry.
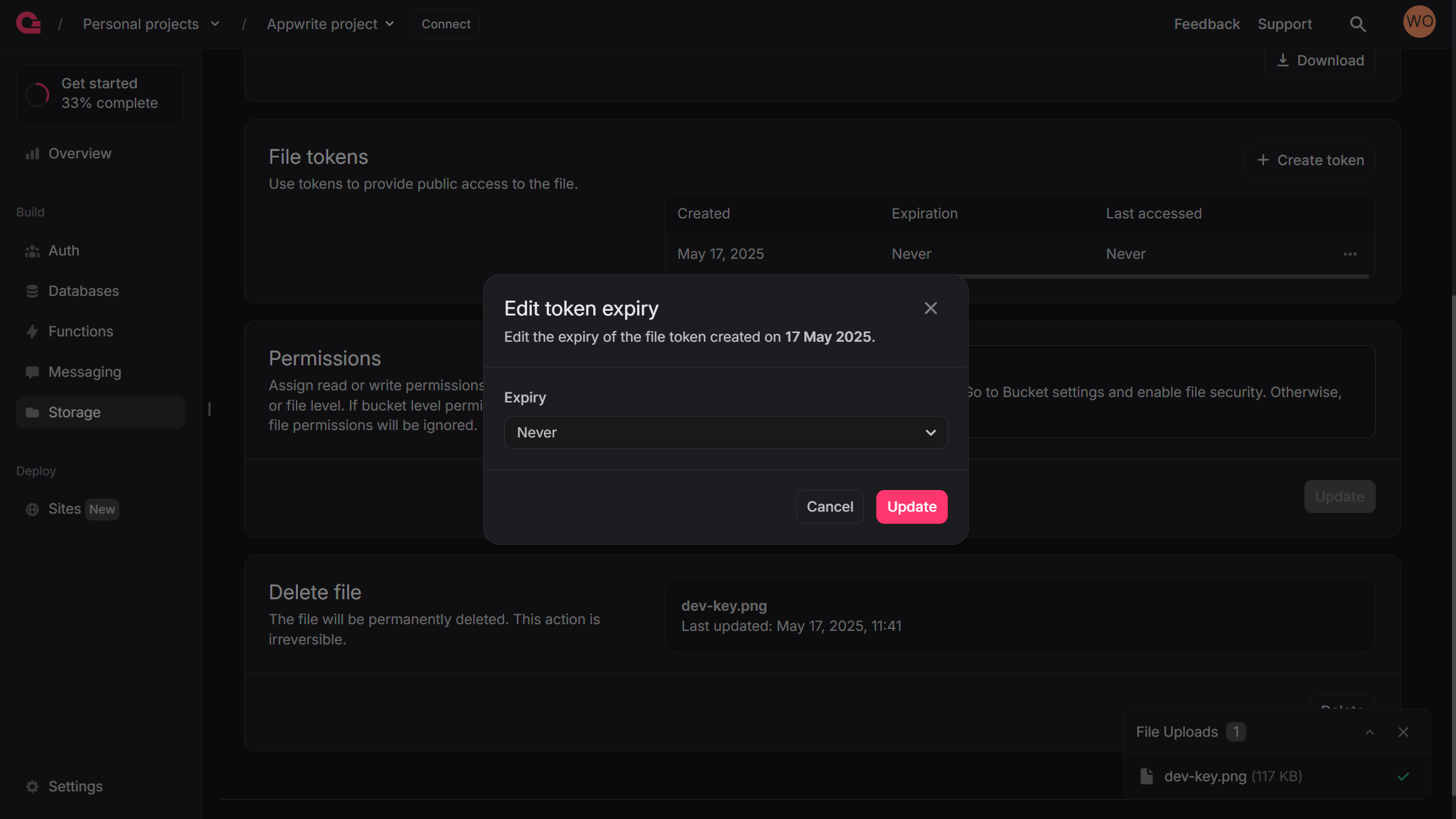
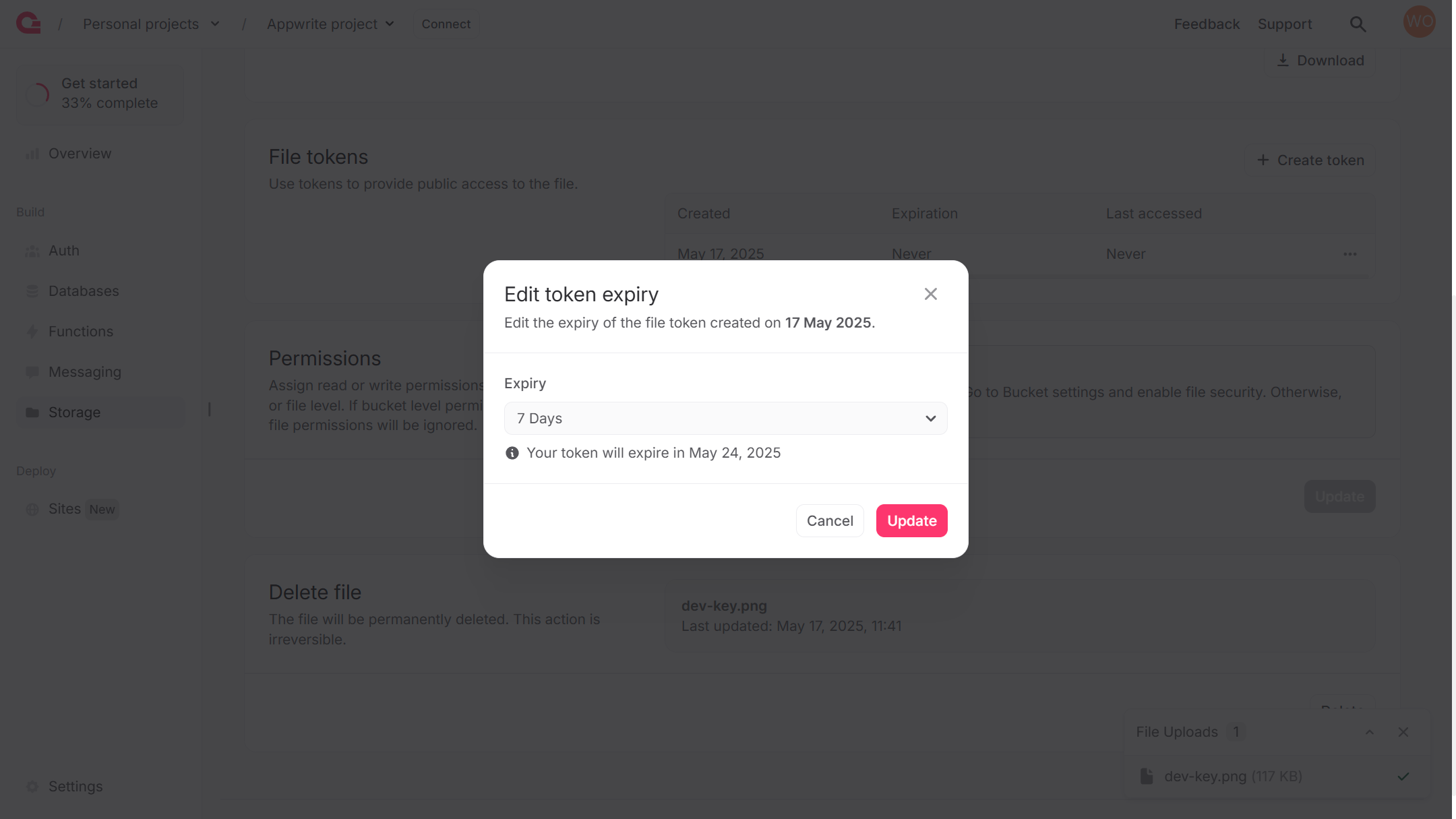
You can update file token expiry programmatically using a Server SDK. Appwrite's Server SDKs require an API key with the tokens.write scope enabled.
Delete file tokens
You can use the Appwrite Console or one of the Server SDKs to delete a file token.
Head to the Storage page, open a file inside a bucket, and scroll down to the File tokens section. Click on the three-dots menu next to the created file token and click on Delete.
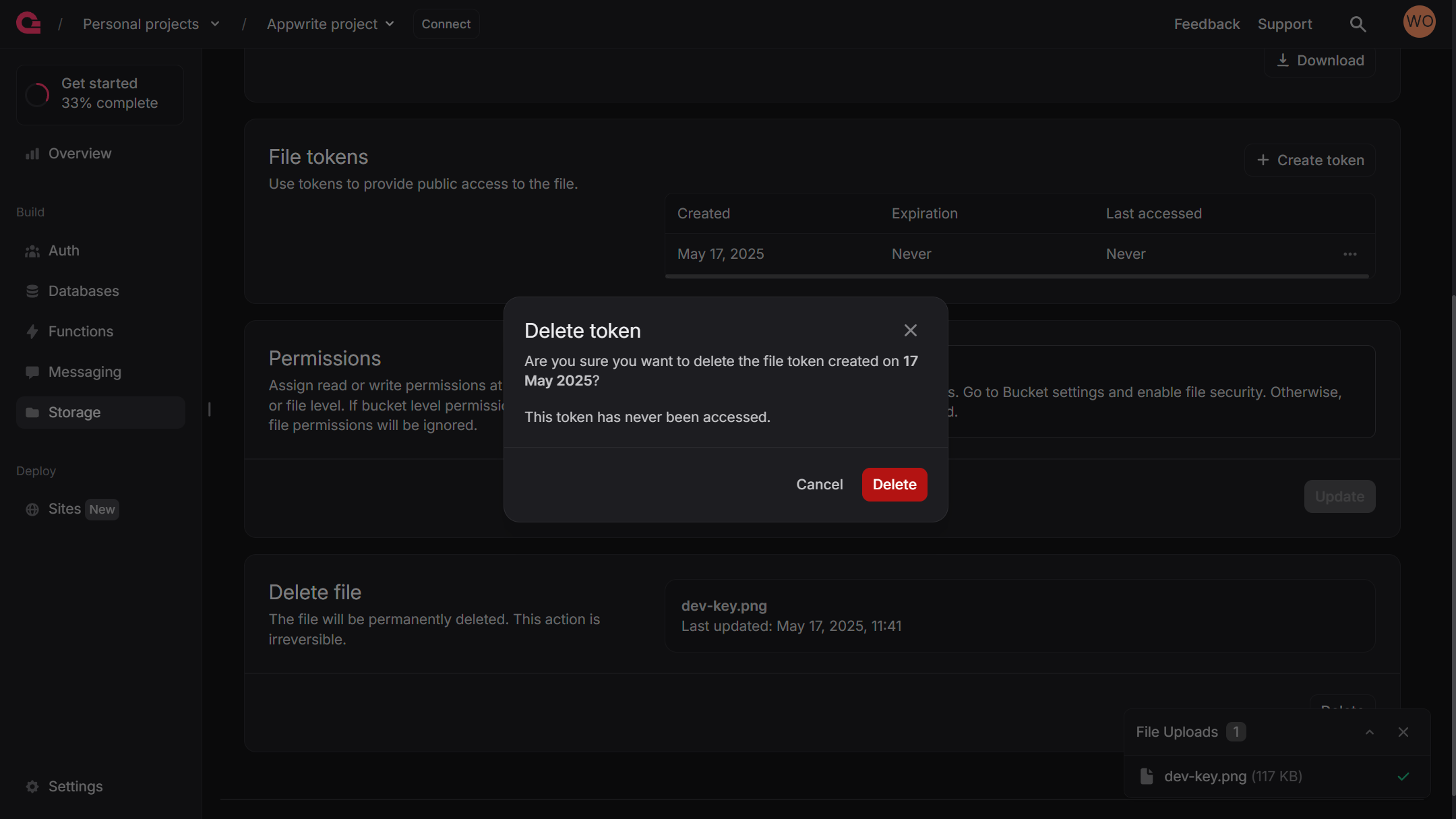
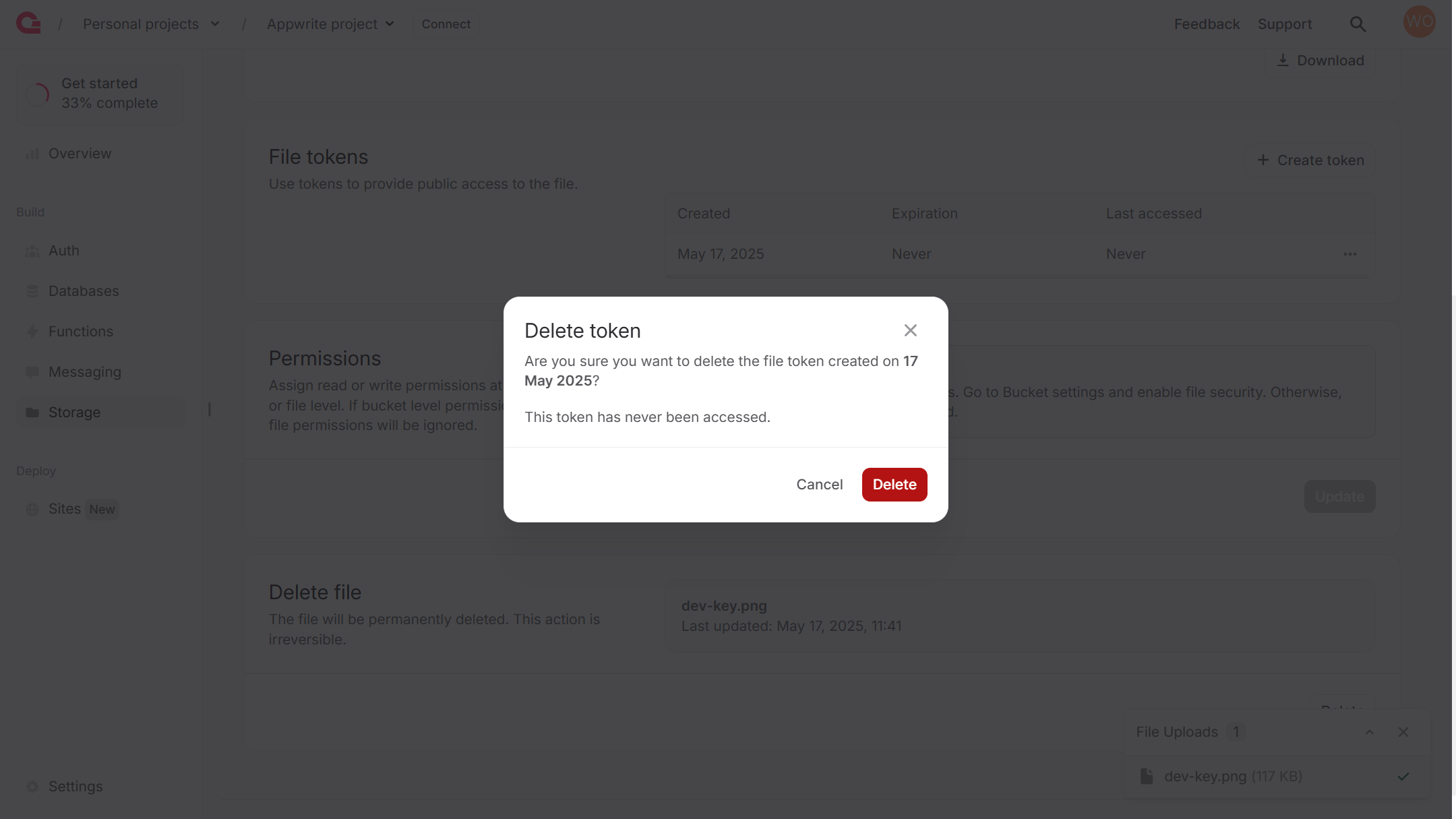
You can delete a file token programmatically using a Server SDK. Appwrite's Server SDKs require an API key with the tokens.write scope enabled.